The Complete Spring Boot Development Bootcamp
17h 59m 2s
English
Paid
Spring Boot is the best Java framework for developing web applications. It favors convention over configuration, making it super easy to develop stand-alone, production-grade web services and APIs. Become a Java Web Developer and Build Powerful APIs!
The Spring Boot Development Bootcamp offers support for Visual Studio Code and IntelliJ. The curriculum is as follows:
Read more about the course
- Setting up a Spring Boot Project: Create a Spring Boot Project using best practices.
- Model View Controller: Build a Web application that adheres to the Model View Controller design.
- Field Validation: Validate fields prior to form submission to prevent the storage of invalid data.
- Three-Layer Codebase: Refactor your code to make sure it's clean and scalable.
- Bean and Dependency Injection: Loosely couple your code using beans.
- Testing a Spring Boot Web Application: Write unit and integration tests to verify that your application is behaving correctly.
- React: Connect your Spring Boot application to a React Front-end.
- REST API: Develop a REST API that can process GET / POST / PUT / DELETE operations.
- OpenAPI: Document your application using the OpenAPI specification.
- Exception Handling: Make your API resilient by throwing and handling unchecked exceptions inside @ControllerAdvice.
- Spring Data JPA (SQL): Develop a REST API that interacts with an SQL database. Define relational tables that contain @OneToMany and @ManyToMany relationships.
- Spring Boot Security: Secure your application through Basic as well as Token-based Authentication (JWT).
Watch Online The Complete Spring Boot Development Bootcamp
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Introduction | 00:26 |
2 | Prerequisite for this course | 00:22 |
3 | Where to Ask Questions | 00:31 |
4 | Required Installation: Java 17 (Mac) | 02:06 |
5 | Required Installation: Java 17 (Windows) | 01:56 |
6 | Recommended Installation: Maven (Mac) | 04:41 |
7 | Recommended Installation: Maven (Windows) | 04:31 |
8 | Visual Studio Code Vs. IntellJ | 01:59 |
9 | Roadmap | 00:17 |
10 | Downloading Visual Studio Code | 01:44 |
11 | Creating a Spring Boot Project | 04:41 |
12 | Breaking Down a Spring Boot Project | 02:28 |
13 | Running a Spring Boot Application | 04:47 |
14 | The Client-Server Model | 05:51 |
15 | Workbook and Challenges | 01:40 |
16 | Workbook 1.1 | 00:43 |
17 | Launching an HTTP Server | 09:43 |
18 | Workbook 1.2 | 01:22 |
19 | Workbook 1.3 | 01:05 |
20 | Maven Dependencies | 04:18 |
21 | Spring Boot DevTools Dependency | 03:14 |
22 | Cheat Sheet | 00:16 |
23 | Challenge | 00:39 |
24 | Solution | 07:14 |
25 | Roadmap | 00:17 |
26 | Downloading IntelliJ | 02:25 |
27 | Creating a Spring Boot Project | 04:03 |
28 | Breaking Down a Spring Boot Project | 02:28 |
29 | Running a Spring Boot Application | 05:03 |
30 | The Client-Server Model | 05:51 |
31 | Workbooks and Challenges | 01:40 |
32 | Workbook 1.1 | 00:43 |
33 | Launching an HTTP Server | 10:00 |
34 | Workbook 1.2 | 01:22 |
35 | Workbook 1.3 | 01:05 |
36 | Maven Dependencies | 04:18 |
37 | Spring Boot DevTools Dependency | 03:05 |
38 | Cheat Sheet | 00:16 |
39 | Challenge | 00:39 |
40 | Solution | 08:04 |
41 | Roadmap | 01:01 |
42 | Starter Project | 01:15 |
43 | The MVC Design | 02:39 |
44 | The Controller | 05:17 |
45 | Path A: Breakpoint Session | 02:08 |
46 | Path B: Breakpoint Session | 02:08 |
47 | The View | 02:44 |
48 | Backend vs Front end | 00:46 |
49 | Path A: The Model | 06:40 |
50 | Path B: The Model | 06:42 |
51 | Path A: Breakpoint Session | 01:16 |
52 | Path B: Breakpoint Session | 01:27 |
53 | Thymeleaf: Combining Model and View | 05:56 |
54 | Selection Expression | 03:11 |
55 | Thymeleaf Conditionals | 09:01 |
56 | Utility Methods | 07:24 |
57 | Link Expression | 05:49 |
58 | Thymeleaf Loops | 08:53 |
59 | Path A: Breakpoint Session | 01:44 |
60 | Path B: Breakpoint Session | 01:54 |
61 | Creating a Form | 05:53 |
62 | Form Submission | 09:17 |
63 | Path A: Breakpoint Session | 05:22 |
64 | Path B: Breakpoint Session | 05:23 |
65 | Updating Student Grade | 14:52 |
66 | Path A: Breakpoint Session | 06:07 |
67 | Path B: Breakpoint Session | 06:17 |
68 | Updating Grade Based on Id | 07:28 |
69 | Path A: Breakpoint Session | 09:53 |
70 | Path B: Breakpoint Session | 10:01 |
71 | Final Touches | 03:27 |
72 | Challenge | 01:12 |
73 | Solution – Part 1 | 10:11 |
74 | Solution – Part 2 | 15:26 |
75 | Solution – Part 2 (Follow-up) | 12:39 |
76 | Solution – Part 3 (Tasks 1 - 4) | 14:06 |
77 | Solution – Part 3 (Remaining Tasks) | 12:22 |
78 | Roadmap | 00:22 |
79 | Field Validation | 11:47 |
80 | Breakpoint Session | 03:51 |
81 | Breakpoint Session 2 | 03:51 |
82 | Workbook 3.1 | 00:39 |
83 | Custom Constraints | 10:53 |
84 | Workbook 3.2 (incl. Cross Field Validation) | 00:31 |
85 | Workbook 3.3 | 00:27 |
86 | Breakpoint Session | 03:06 |
87 | Breakpoint Session 2 | 03:16 |
88 | Challenge | 00:45 |
89 | Solution | 14:01 |
90 | Three Layer Architecture | 04:17 |
91 | Repository | 08:21 |
92 | Service | 09:06 |
93 | Challenge | 00:18 |
94 | Solution | 12:24 |
95 | Beans | 01:58 |
96 | Dependency Injection | 05:09 |
97 | @Service and @Repository | 02:52 |
98 | @Bean | 03:46 |
99 | Workbook 5.1 | 00:45 |
100 | Breakpoint Session | 05:21 |
101 | Autowired Vs. Constructor | 01:37 |
102 | Solution | 05:41 |
103 | The Importance of Dependency Injection for Unit Testing | 03:53 |
104 | Setting up Testing Class | 05:34 |
105 | Unit Testing the Service Class | 19:07 |
106 | Breakpoint Session | 06:10 |
107 | Intro to Integration Testing | 04:11 |
108 | Integration Testing – Part 1 | 04:08 |
109 | Integration Testing – Part 2 | 12:57 |
110 | Breakpoint Session | 04:41 |
111 | React Front-end and Spring Boot Backend | 05:02 |
112 | Demo: React + Spring Boot | 04:36 |
113 | Module 2 | 00:38 |
114 | REST API | 03:49 |
115 | REST API: Getting Started | 07:00 |
116 | REST API: GET Operation | 13:25 |
117 | Postman | 01:37 |
118 | REST API: POST Operation | 08:41 |
119 | REST API: PUT Operation | 06:03 |
120 | REST API: DELETE Operation | 08:48 |
121 | Solution | 20:07 |
122 | Solution 2 | 19:28 |
123 | Roadmap | 00:44 |
124 | Getting Started: JPA and H2 | 05:22 |
125 | Object Relational Mapper | 08:18 |
126 | Saving a Student | 11:42 |
127 | Retrieving a Student | 03:18 |
128 | Deleting a Student | 03:17 |
129 | Lombok | 03:40 |
130 | Grade Entity | 05:15 |
131 | Unidirectional: Many to One | 09:23 |
132 | Read Grade from Student ID | 04:07 |
133 | Bidirectional: One to Many | 11:20 |
134 | Cascade | 02:13 |
135 | Autowired vs. AllArgsConstructor | 02:33 |
136 | Course Entity | 09:57 |
137 | Bidirectional: One to Many | 10:09 |
138 | Refactoring Around Optionals | 07:32 |
139 | Finalizing the GradeServiceImpl | 07:28 |
140 | Constraints: Preventing Duplicate Grades | 02:55 |
141 | Refactoring Around Optionals: Loose Ends | 05:14 |
142 | Solution | 23:23 |
143 | Solution 2 | 04:18 |
144 | Roadmap | 01:11 |
145 | Basic Auth: Authentication and Authorization | 03:54 |
146 | Basic Auth with Spring Security (Part 1) | 18:35 |
147 | Basic Auth with Spring Security (Part 2) | 08:54 |
148 | Getting Started: JWT Project | 06:52 |
149 | Token-based Authentication (JWT) | 10:08 |
150 | Token-based Authentication – Part 1 | 06:18 |
151 | Intermission: Protecting the Password | 01:29 |
152 | Token-based Authentication – Part 2 | 09:50 |
153 | Side Quest: Exception Handling + Dispatcher Servlet | 13:44 |
154 | Token-based Authentication – Part 3 | 17:00 |
155 | Token-based Authentication – Part 4 | 10:13 |
156 | Token-based Authentication – Part 5 | 29:07 |
157 | Final Recap | 03:44 |
158 | Path A: Customize your Editor | 02:48 |
159 | Path B: Customize your Editor | 01:49 |
160 | Path A: Breakpoints in Visual Studio Code | 05:31 |
161 | Path B: Breakpoints in IntelliJ | 05:18 |
162 | Workbook 1.1 | 01:17 |
163 | Path A: Workbook 1.2 | 04:41 |
164 | Path B: Workbook 1.2 | 04:08 |
165 | Path A: Workbook 1.3 | 03:58 |
166 | Path B: Workbook 1.3 | 04:03 |
167 | Workbook 2.1 | 05:42 |
168 | Workbook 2.2 | 09:04 |
169 | Workbook 2.3 | 03:51 |
170 | Workbook 2.4 | 02:10 |
171 | Workbook 2.5 | 05:44 |
172 | Workbook 2.6 | 05:07 |
173 | Workbook 2.7 | 04:50 |
174 | Workbook 2.10 | 04:30 |
175 | Workbook 2.11 | 09:24 |
176 | Workbook 3.1 | 22:02 |
177 | Workbook 3.2 | 03:42 |
178 | Workbook 3.3 | 13:00 |
179 | Workbook 5.1 | 04:40 |
180 | Workbook 8.1 | 09:17 |
181 | Workbook 8.2 | 11:18 |
182 | Workbook 8.3 | 09:19 |
183 | Workbook 9.1 | 10:37 |
184 | Workbook 10.1 | 07:22 |
Similar courses to The Complete Spring Boot Development Bootcamp
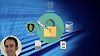
Web security: Injection Attacks with Java & Spring Bootudemy
Category: JavaScript, Spring Boot, Spring Data, Ethical Hacking / Penetration Testing
Duration 8 hours 44 minutes 36 seconds
Course
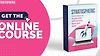
Stratospheric - From Zero to Production with Spring Boot and AWS + BOOKleanpub
Category: AWS, Spring Boot
Duration 7 hours 19 minutes 39 seconds
Course
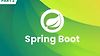
Spring Boot: Mastering REST API Developmentcodewithmosh (Mosh Hamedani)
Category: Spring Boot
Duration 8 hours 59 minutes 26 seconds
Course
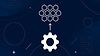
Event-Driven Microservices, CQRS, SAGA, Axon, Spring Bootudemy
Category: Spring Boot, Spring Cloud
Duration 8 hours 55 minutes 3 seconds
Course
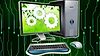
Java Spring Tutorial Masterclass - Learn Spring Framework 5udemy
Category: Spring Boot, Spring, Spring MVC
Duration 45 hours 18 minutes 33 seconds
Course
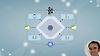
Microservices: Clean Architecture, DDD, SAGA, Outbox & Kafkaudemy
Category: Spring Boot, Others, Java
Duration 18 hours 2 minutes 34 seconds
Course
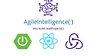
Full Stack HATEOAS: Spring Boot 2.1, ReactJS, Reduxudemy
Category: React.js, Spring Boot, Redux, Spring, Spring HATEOAS
Duration 5 hours 51 minutes 37 seconds
Course
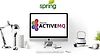
Java Messaging Service - Spring MVC, Spring Boot, ActiveMQudemy
Category: Spring Boot, Java
Duration 1 hour 47 minutes 44 seconds
Course
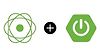
Build Reactive RESTFUL APIs using Spring Boot/WebFluxudemy
Category: Spring Boot
Duration 9 hours 34 minutes 2 seconds
Course
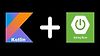
Build RESTFUL APIs using Kotlin and Spring Bootudemy
Category: Spring Boot, Others
Duration 8 hours 23 minutes 18 seconds
Course