The Complete Developers Guide to MongoDB
More
NodeJS focused? Yep. Test Driven Exercises? Absolutely! Advanced Features of MongooseJS? You know it.
This course will get you up and running with MongoDB quickly, and teach you the core knowledge you need to deeply understand and build apps centered around Mongo.
We'll start by mastering the fundamentals of Mongo, including collections, validations, and common record manipulation techniques. Source code is provided for each lecture, so you will always stay up-to-date with the course pacing. Special attention has been paid to creating reusable code that you'll be able to make use of on your own fantastic projects.
If you are new to MongoDB, or if you've been working to learn it but sometimes feel like you still don't quite 'get it', this is the MongoDB course for you! To learn MongoDB you have to understand it.
- Learn how to use the popular MongooseJS library to interface with Mongo
- Write tests around Mongo queries to ensure your code is working. You can reuse these tests on your own personal projects!
- Master the process of designing NoSQL schema
- Grasp the differences record associations and resource embedding
- Use the advanced features of Mongoose to save development time
- Develop apps that are fast and responsive thanks to Mongo's speed and flexibility
- Work on either Windows or OSX
- Master the integration of Mongo, Node, and Mocha in a modern development environment
I've built the course that I would have wanted to take when I was learning MongoDB. A course that explains the concepts and how they're implemented in the best order for you to learn and deeply understand them.
Requirements:
All you need is a Mac or a PC
- This course is for anyone learning MongoDB in the Node JS environment
What you'll learn:
- Understand how MongoDB stores data
- Gain mastery of the most popular MongoDB interface, Mongoose JS
- Write efficient queries for reading data
- Learn the purpose of each of Mongoose's functions
- Design effective NoSQL schema with both data nesting and lookups
Watch Online The Complete Developers Guide to MongoDB
# | Title | Duration |
---|---|---|
1 | How to Use This Course | 02:21 |
2 | MongoDB Setup on OSX | 11:22 |
3 | More OSX Setup - Robomongo | 04:02 |
4 | Windows Setup | 08:48 |
5 | RoboMongo Setup | 03:33 |
6 | A Refresher on Promises | 03:25 |
7 | Let's Play A Game | 07:40 |
8 | Winning the Game | 07:17 |
9 | Behind the Scenes with Promises | 06:26 |
10 | Where Do We Use Mongo? | 03:02 |
11 | Fundamentals of MongoDB | 04:15 |
12 | A Last Touch of Setup | 03:22 |
13 | Project Overview | 05:00 |
14 | The Test Helper File | 07:43 |
15 | Mongoose Connection Helper | 10:07 |
16 | Mongoose Models | 05:14 |
17 | More on Models | 06:28 |
18 | The Basics of Mocha | 06:04 |
19 | Running Mocha Tests | 06:22 |
20 | Creating Model Instances | 06:13 |
21 | Saving Users to Mongo | 07:49 |
22 | Dropping Collections | 04:49 |
23 | Mocha's Done Callback | 04:57 |
24 | Mongoose's isNew Property | 06:26 |
25 | Default Promise Implementation | 06:47 |
26 | Test Setup for Finding Users | 06:39 |
27 | Making Mongo Queries | 06:01 |
28 | The ID Property - A Big Gotcha | 06:25 |
29 | Automating Tests with Nodemon | 04:58 |
30 | Finding Particular Records | 05:06 |
31 | The Many Ways to Remove Records | 09:55 |
32 | Class Based Removes | 05:12 |
33 | More Class Based Removals | 05:47 |
34 | The Many Ways to Update Records | 04:00 |
35 | Set and Save for Updating Records | 08:22 |
36 | Model Instance Updates | 07:39 |
37 | Class Based Updates | 08:51 |
38 | Update Operators | 11:16 |
39 | The Increment Update Operator | 06:18 |
40 | Validation of Records | 05:04 |
41 | Requiring Attributes on a Model | 11:16 |
42 | Validation With a Validator Function | 06:42 |
43 | Handling Failed Inserts | 04:21 |
44 | Embedding Resources in Models | 05:18 |
45 | Nesting Posts on Users | 05:41 |
46 | Testing Subdocuments | 07:30 |
47 | Adding Subdocuments to Existing Records | 11:29 |
48 | Removing Subdocuments | 07:47 |
49 | Virtual Types | 08:00 |
50 | Defining a Virtual Type | 06:20 |
51 | ES6 Getters | 08:18 |
52 | Fixing Update Tests | 02:20 |
53 | Challenges of Nested Resources | 05:28 |
54 | Embedded Documents vs Separate Collections | 07:56 |
55 | BlogPosts vs Posts | 03:28 |
56 | Creating Associations with Refs | 08:54 |
57 | Test Setup for Associations | 05:13 |
58 | Wiring Up Has Many and Has One Relations | 08:41 |
59 | Promise.All for Parallel Operations | 06:45 |
60 | Populating Queries | 11:07 |
61 | Loading Deeply Nested Associations | 12:13 |
62 | Cleaning Up with Middleware | 04:43 |
63 | Dealing with Cyclic Requires | 05:08 |
64 | Pre-Remove Middleware | 06:00 |
65 | Testing Pre-Remove Middleware | 05:47 |
66 | Skip and Limit | 04:31 |
67 | Writing Skip and Limit Queries | 07:06 |
68 | Sorting Collections | 07:16 |
69 | Project Setup | 03:16 |
70 | Project Overview | 05:47 |
71 | First Step - Artist and Album Models | 04:30 |
72 | The Album Schema | 07:18 |
73 | The Artist Model | 09:12 |
74 | Finding Particular Records | 05:32 |
75 | FindOne vs FindById | 06:30 |
76 | The CreateArtist Operation | 02:49 |
77 | Solution to Creating Artists | 03:13 |
78 | Deleting Singular Records | 02:22 |
79 | Solution to Removing | 04:21 |
80 | Editing Records | 02:57 |
81 | How to Edit Single Artists | 03:15 |
82 | Minimum and Maximum Values in a Collection | 05:38 |
83 | Solution to Min and Max Queries | 14:59 |
84 | Challenge Mode - Search Query | 07:36 |
85 | Sorting, Limiting, and Skipping Together | 13:06 |
86 | Danger! Big Challenge Ahead | 04:31 |
87 | Filtering By Single Properties | 07:23 |
88 | Filtering with Multiple Props | 03:31 |
89 | Handling Text Search | 04:51 |
90 | Indexes and Text Search | 10:06 |
91 | Batch Updates | 05:29 |
92 | The Hidden 'Multi' Setting | 07:07 |
93 | Seeding Many Records | 06:32 |
94 | Counting the Result Set | 04:51 |
95 | App Overview | 02:42 |
96 | Designing API Routes | 06:02 |
97 | Project Setup | 06:49 |
98 | HTTP Request Methods | 03:55 |
99 | The Basics of Express | 04:41 |
100 | Express Boilerplate | 04:31 |
101 | Handling Requests with Express | 08:13 |
102 | Testing Express Apps with Mocha | 07:22 |
103 | Running Mocha | 04:34 |
104 | Project Structure | 02:58 |
105 | Refactoring for Controllers and Models | 09:40 |
106 | The Driver Model | 06:52 |
107 | The Create Drivers Route | 03:48 |
108 | The BodyParser Middleware | 07:04 |
109 | Testing Driver Creation | 07:16 |
110 | More on Testing Driver Creation | 09:08 |
111 | Additional Mongoose Setup | 05:28 |
112 | Driver Implementation | 04:34 |
113 | Testing Endpoints with Postman | 09:15 |
114 | Dev vs Test Environments | 03:41 |
115 | Separate Test Databases | 10:34 |
116 | Middlewares in Express | 15:04 |
117 | Handling Editing of Drivers | 09:10 |
118 | Testing Driver Updates | 07:45 |
119 | Handling Deletion of Drivers | 03:06 |
120 | Testing Driver Deletion | 05:28 |
121 | Geography with MongoDB | 05:33 |
122 | The GeoJSON Schema | 05:42 |
123 | GeoNear Queries | 10:13 |
124 | Testing a GeoNear Query | 07:02 |
125 | One Big Gotcha | 04:50 |
126 | Another Big Gotcha | 02:57 |
127 | Testing GeoQueries | 03:40 |
Similar courses to The Complete Developers Guide to MongoDB
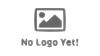
Node.js API - making it shine!udemy
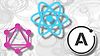
Full-Stack React with GraphQL and Apollo Boostudemy
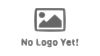
Node.js, Express, MongoDB & More The Complete Bootcamp 2023udemy
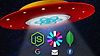
MERN Stack Web Development with Ultimate Authenticationudemy
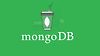
MongoDBcodeschool
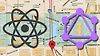
Build a Realtime App with React Hooks and GraphQLudemy
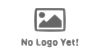
MongoDBamigoscode (Nelson Djalo)
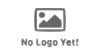
The Complete Node.js Developer Course (3rd Edition)udemy
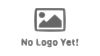
Web Development with Google’s Go (golang) Programming Languagegreatercommons.com
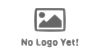