Node.js API Masterclass With Express & MongoDB
This is a project based course where we build an extensive, in-depth backend API for DevCamper, a bootcamp directory app. We will start from scratch and end up with a professional deployed API with documentation. We will dive deep into Node, Express and MongoDB. Here is some of what you will learn in this course and project...
Read more about the course
HTTP Essentials
Postman Client
RESTful APIs
Express Framework
Routing & Controller Methods
MongoDB Atlas & Compass
Mongoose ODM
Advanced Query (Pagination, filter, etc)
Models & Relationships
Middleware (Express & Mongoose)
MongoDB Geospatial Index / GeoJSON
Geocoding
Custom Error Handling
User Roles & Permissions
Aggregation
Photo Upload
Authentication With JWT & Cookies
Emailing Password Reset Tokens
Custom Database Seeder Using JSON Files
Password & Token Hashing
Security: NoSQL Injection, XSS, etc
Creating Documentation
Deployment With PM2, NGINX, SSL
- Modern JavaScript (ES6)
- Basic programming principles
- Basic knowledge of Node helps
- People that want to learn backend web development with Node
- React/Vue/Angular Frontend devs that want to be full stack devs
What you'll learn:
- Real World Backend RESTful API For Bootcamp Directory App
- HTTP Fundamentals (Req/Res Cycle, Status Codes, etc)
- Advanced Mongoose Queries
- JWT/Cookie Authentication
- Express & Mongoose Middleware (Geocoding, Auth, Error Handling, etc)
- API Security (NoSQL injection, XSS protection, Rate Limiting)
- API Documentation & Deployment
Watch Online Node.js API Masterclass With Express & MongoDB
# | Title | Duration |
---|---|---|
1 | Course Introduction | 03:36 |
2 | A Look At The Project | 06:38 |
3 | Environment Setup | 05:43 |
4 | HTTP & the Node Http Module | 10:04 |
5 | Installing Nodemon | 03:56 |
6 | Responding With Data | 08:04 |
7 | HTTP Status Codes | 07:40 |
8 | Sending Data To The Server | 05:35 |
9 | HTTP Methods & RESTful APIs | 12:45 |
10 | Project Specs & Resources | 06:47 |
11 | Basic Express Server, dotenv & Git | 10:10 |
12 | Creating Routes & Responses In Express | 10:13 |
13 | Using The Express Router | 05:01 |
14 | Creating Controller Methods | 09:58 |
15 | Intro To Middleware | 10:41 |
16 | Postman Environment & Collections | 08:52 |
17 | MongoDB Atlas & Compass Setup | 08:56 |
18 | Connecting To The Database With Mongoose | 11:58 |
19 | Colors In The Console | 02:49 |
20 | Creating Our First Model | 15:23 |
21 | Create Bootcamp - POST | 10:32 |
22 | Fetching Bootcamps - GET | 05:30 |
23 | Updating & Deleting Bootcamps - PUT & DELETE | 08:23 |
24 | Error Handler Middleware | 05:44 |
25 | Custom ErrorResponse Class | 05:34 |
26 | Mongoose Error Handling [1] | 07:25 |
27 | Mongoose Error Handling [2] | 09:13 |
28 | Async/Await Middleware | 06:46 |
29 | Mongoose Middleware & Slugify | 07:52 |
30 | GeoJSON Location & Geocoder Hook - MapQuest API | 15:39 |
31 | Database Seeder For Bootcamps | 10:23 |
32 | Geospatial Query - Get Bootcamps Within Radius | 14:08 |
33 | Advanced Filtering | 12:37 |
34 | Select & Sorting | 12:49 |
35 | Adding Pagination | 11:31 |
36 | Course Model & Seeding | 09:03 |
37 | Course Routes & Controller | 12:21 |
38 | Populate, Virtuals & Cascade Delete | 11:47 |
39 | Single Course & Add Course | 11:57 |
40 | Update & Delete Course | 07:39 |
41 | Aggregate - Calculating The Average Course Cost | 16:15 |
42 | Photo Upload For Bootcamp | 23:13 |
43 | Advanced Results Middleware | 12:15 |
44 | User Model | 11:35 |
45 | User Register & Encrypting Passwords | 09:55 |
46 | Sign & Get JSON Web Token | 09:10 |
47 | User Login | 12:40 |
48 | Sending JWT In a Cookie | 09:56 |
49 | Auth Protect Middleware | 16:47 |
50 | Storing The Token In Postman | 05:19 |
51 | Role Authorization | 07:08 |
52 | Bootcamp & User Relationship | 10:42 |
53 | Bootcamp Ownership | 08:33 |
54 | Course Ownership | 07:25 |
55 | Forgot Password - Generate Token | 11:35 |
56 | Forgot Password - Send Email | 16:17 |
57 | Reset Password | 09:42 |
58 | Update User Details | 10:44 |
59 | Admin Users CRUD | 18:38 |
60 | Review Model & Get Reviews | 10:52 |
61 | Get Single Review & Update Seeder | 09:03 |
62 | Add Review For Bootcamp | 10:28 |
63 | Aggregate - Calculate Average Rating | 07:30 |
64 | Update & Delete Reviews | 12:52 |
65 | Logout To Clear Token Cookie | 07:36 |
66 | Prevent NoSQL Injection & Sanitize Data | 05:19 |
67 | XSS Protection & Security Headers | 07:04 |
68 | Rate Limiting, HPP & CORS | 07:58 |
69 | Documentation With Postman & Docgen | 12:13 |
70 | Digital Ocean Droplet & Server Log In | 05:51 |
71 | Prepare & Push To Github | 06:24 |
72 | Clone Repo On Server | 08:42 |
73 | PM2 Process Manager Setup | 05:45 |
74 | NGINX Reverse Proxy Setup | 05:55 |
75 | Domain, SSL & Wrap Up | 11:39 |
Similar courses to Node.js API Masterclass With Express & MongoDB
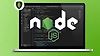
Node.js, Express, MongoDB Bootcamp 2020 - with Real Projectsudemy
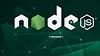
NodeJS: Beginner to Pro - APIs for Food Delivery & Ecommerceudemy
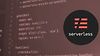
Serverless Framework Bootcamp: Node.js, AWS & Microservicesudemy
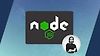
Understanding Node.js: Core Conceptsudemy
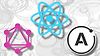
Full-Stack React with GraphQL and Apollo Boostudemy
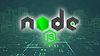
Complete Node.js Developer in 2023: Zero to Masteryzerotomastery.io
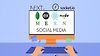