SocketIO v4, with websockets - the details.
This course is meant to alleviate that! It is not a quick start guide to socket.IO. There are loads of those all over the Internet. You should definitely look elsewhere if you are wanting a 10 minute intro to the 3-4 things you need to know to make something quick. On the other hand, if you are looking to really learn one of the most awesome JavaScript libraries in socket io, you should stick around. Like Express and other JavaScript/Node pieces, it's getting passed over in the wave to learn just enough to get to the term "full-stack developer."
Read more about the course
- You have a solid working knowledge of JavaScript and nodejs (Not a ninja, but you are not new).
- You have used the node ecosystem (npm, Express, etc.). This is NOT an intro to node class and I will NOT cover these in detail.
- You have basic command line knowledge (at least capable of navigating and running a program)
- You have a computer capable of running Nodejs.
Websockets are one of the coolest things to hit the web in ages. They allow the browser real-time communication bridging one of the last gaps in both human and web-based communication. Socket io is the king library that uses websockets under the hood. There's a good chance if you're reading this, you've heard about socket io. Maybe even done a tutorial on it. But how far did you get? In my experience, the vast majority of the material on the web goes no farther than a quick-start, instant chat app. You don't learn how anything works, never look at the docs, and are stuck at the end wondering what to do now. The remaining shred of material is waaaaay over everyone's head. The fact that the websocket API was standardized in 2011 and most developers still don't know how to use it is evidence of the gap.
My main goal is to help you figure out how to go from being a good developer to a great developer. Understanding... not just knowing a few methods... of socketio is part of that! It even trancends the browser and node with implementations in most other languages, and even mobile. This means as you grow, you have the power of sockets without having to learn more than the socket io library.
I first used socket.IO in 2013 for a tiny company directory app. I've been following since and have been frustrated that it hasn't gotten more mainstream notice because it opens the way for so many improvements to existing applications and obvious groundwork for new ones. Let's change that :) Prepare to for a detailed look at socketio and websockets and start going real-time.
Sections:
Environment Setup (skip if you have node installed already)
Before Socket.IO... - TCP, network sockets, & a native websockets app
Socketi.IO 101 - Why you'd want to use socketio and how it works (simple chat app)
Socket.IO 201 - Making the chat app into a slack clone with namespaces and rooms
Project 1 - real-time canvas game
Project 2 - Real-time performance data (uses React, Cluster, and Redis)
streaming videos & socket.io-stream - in development
Supplemental - HTTP (for those in need of a review)
- JS/Node developers who want to actually learn how to use Socketio for more than a chat app tutorial
- JS developers interested in how node & JS combine to make the networking happen
- Developers interested in building real time applications
What you'll learn:
- Socketio. That's (mostly) all we cover so when you finish, you'll know it!
- Setup a socketio application between the browser and an Express server and run real-time back and forth
- Understand the basics of websockets and how they work
- The basics of the transport layer and how a packet works
Watch Online SocketIO v4, with websockets - the details.
# | Title | Duration |
---|---|---|
1 | Welcome Video | 02:15 |
2 | Course Overview | 05:18 |
3 | It's 2023: native websockets or socket.io... what should we use? | 10:45 |
4 | Pre-socketio | 06:26 |
5 | Housekeeping - How I do node/express | 05:18 |
6 | TCP/UDP and networking 101 | 18:08 |
7 | Networking 201 - What is a socket and why should I care? | 15:37 |
8 | HTTP vs Websockets | 03:55 |
9 | A short overview of native websockets (finally some code!!) | 13:48 |
10 | A short overview of native websockets continued | 15:05 |
11 | Intro | 01:42 |
12 | Why Socket.io? This is why. | 08:10 |
13 | The basics of socket.io | 15:07 |
14 | An important pitfall - connect/reconnect | 07:44 |
15 | Small Chat app - in socket.io! | 17:00 |
16 | Docs - new Server | 10:04 |
17 | Docs - Server options | 09:12 |
18 | The big 3 - .emit, .on, .connect | 09:35 |
19 | Docs - The Client | 07:44 |
20 | Section Intro and folder setup | 04:15 |
21 | A quick illustration | 04:15 |
22 | Project Whiteboarding & Steps | 11:07 |
23 | Setup slack with sanity checks | 07:59 |
24 | Steps 1-3 - Populate spaces and rooms from the server | 09:58 |
25 | Rooms and Namespaces classes | 10:49 |
26 | Add rooms to DOM | 08:34 |
27 | UX Cleanup | 06:38 |
28 | Docs - Namespaces | 11:43 |
29 | Namespaces code review | 06:16 |
30 | Docs - Rooms | 11:38 |
31 | Step 4-6 | 09:23 |
32 | Whiteboarding - performance thinking Websockets | 05:36 |
33 | Implementing nsChange and Express route --> io.emit | 13:18 |
34 | Implementing nsChange continued... | 10:13 |
35 | Slack - Joining a Room- Steps 7-9 | 12:19 |
36 | Acknowledgement Functions, fetchSockets() - steps 7-9 continued | 15:17 |
37 | emitWithAck, init join room | 04:48 |
38 | emit messages to room - steps 7-9 continued | 14:20 |
39 | Slack - Sending the history - Steps 7-9 continued | 19:44 |
40 | Passing query data on connection - basic auth intro | 08:10 |
41 | Project Demo & setting expectations | 12:48 |
42 | Project Strategy - performance matters! | 10:32 |
43 | Socket.io App Organization | 16:06 |
44 | Getting the DOM setup | 14:14 |
45 | A few UI Loose ends | 07:13 |
46 | Draw the player | 13:24 |
47 | The ugly math of moving the right direction | 19:58 |
48 | Get, and draw, the game orbs (non-players) | 16:41 |
49 | Whiteboarding Player classes - What does the server NEED to send? | 07:30 |
50 | The Player Classes | 14:14 |
51 | Refactoring init for performance | 12:59 |
52 | Tick-Tock - send player data from the server to the clients and vice-versa | 08:46 |
53 | Step 1 of drawing the players from the server | 05:49 |
54 | Tock event - sending player direction from client to server | 08:16 |
55 | Clamping the camera (and a little cleanup) | 17:51 |
56 | Collision Testing (the Math part) | 08:48 |
57 | Collision Testing (the code part) | 14:20 |
58 | Leaderboard | 13:13 |
59 | Disconnect | 06:03 |
60 | Project Demo | 03:53 |
61 | Socket.io scaling options | 07:57 |
62 | Project Setup and dependencies | 06:58 |
63 | Architecture | 09:20 |
64 | Pulling performance data | 11:24 |
65 | Figuring CPU load | 20:02 |
66 | How the cluster module works | 10:18 |
67 | Using the cluster module | 08:29 |
68 | Connecting React to the socket.io server (for testing!) | 06:03 |
69 | Connecting nodeClient to the socket server | 05:11 |
70 | Fetch nodeClient macAddress | 08:12 |
71 | Start the ticking clock | 09:22 |
72 | Create React App Overview | 03:27 |
73 | Basic React Component Architecture | 04:43 |
74 | Connecting React to the socket server the correct way | 08:11 |
75 | Getting data to React, and setting up React components | 10:23 |
76 | Sending state to our <Widget /> | 06:24 |
77 | A little UI busywork - moving/copying files, classNames | 06:56 |
78 | CPU widget - canvas | 07:52 |
79 | Memory widget | 06:36 |
80 | Adding isAlive, disconnect, and final touches | 13:02 |
81 | Handling a nasty React bug... | 13:13 |
82 | Admin UI on Agar Clone | 12:47 |
83 | HTTP 101 | 14:13 |
84 | Namespaces | 23:29 |
85 | Rooms | 21:35 |
86 | Going Slack (project) - Overview & Steps | 15:53 |
87 | Folder structure & DOM overview | 08:19 |
88 | Setting up our data and classes | 10:57 |
89 | Slack: Steps 1-3 | 19:48 |
90 | Slack - Steps 4-6 | 12:49 |
91 | Refactoring and Reorganizing our code | 06:03 |
92 | Slack - Joining a Room- Steps 7-9 | 23:28 |
93 | Slack - Sending the history - Steps 7-9 continued | 18:30 |
94 | Slack - Linking up NS and Group, & Final Touches | 19:26 |
95 | Passing query data on connection | 06:35 |
96 | Line by line review of the project | 21:23 |
97 | Docs Checklist Update | 06:34 |
98 | Project Intro | 12:49 |
99 | Project Strategy | 10:31 |
100 | Socket.io App Organization | 17:12 |
101 | Getting the DOM setup | 15:46 |
102 | Time to draw! | 30:20 |
103 | Add Sockets (and orbs) | 14:36 |
104 | Wiring up the server for collisions | 21:32 |
105 | Connecting the client and server | 07:57 |
106 | Tick-Tock - 30FPS | 22:08 |
107 | Collision Testing (the Math part) | 11:00 |
108 | Collision Testing (The Socket Part) | 13:55 |
109 | Collision Testing (The Socket Part 2) | 10:56 |
110 | Updating the LeaderBoard | 10:18 |
111 | Disconnecting and broadcasting a message | 15:42 |
112 | Project Demo | 04:06 |
113 | Project Setup and dependencies | 05:22 |
114 | Installing Redis on Windows | 03:47 |
115 | Architecture | 18:11 |
116 | Getting performance data | 10:26 |
117 | Getting performance data - part 2 | 15:31 |
118 | How the cluster module works | 12:46 |
119 | Using the cluster module - Master | 08:16 |
120 | Using the cluster module - workers | 10:13 |
121 | Connecting nodeClient to the socket server | 06:12 |
122 | Start the ticking clock | 12:13 |
123 | Mongo/Mongoose Schema and a small fix | 15:49 |
124 | Adding a record | 07:48 |
125 | Create React App Overview | 03:38 |
126 | Connecting React to the socket server for re-useable | 07:38 |
127 | Basic React Component Architecture | 04:50 |
128 | Setup React Components | 05:49 |
129 | Setting our widget state | 16:31 |
130 | CPU widget canvas | 08:31 |
131 | Mem and Info widgets | 11:47 |
132 | Adding isActive, disconnect, and final touches | 23:08 |
Similar courses to SocketIO v4, with websockets - the details.
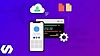
Redis: The Complete Developer's GuideudemyStephen Grider
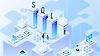
Complete SQL + Databases Bootcamp: Zero to Masteryzerotomastery.io
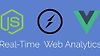
Real-time chat with Node.js, Socket.io and Vue.jsCodecourse
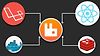
React and Laravel: Breaking a Monolith to Microservicesudemy
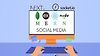
MERN Stack React, Socket io, Next.js Express,MongoDb, Nodejsudemy
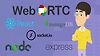
Discord Clone - Learn MERN Stack with WebRTC and SocketIOudemy
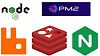
Node JS Cluster with PM2, RabbitMQ, Redis and Nginxudemy
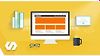
Node JS: Advanced ConceptsudemyStephen Grider
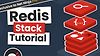