Node JS: Advanced Concepts
Node Internals: Here's one of the most common interview questions you'll face when looking for a Node job: "Can you explain Node's Event Loop?" There are two types of engineers: those who can describe the Event Loop and those who cannot! This course will ensure that you are incredibly well prepared to answer that most important question. Besides being critical for interviews, knowledge of the Event Loop will give you a better understanding of how Node works internally.
More
Many engineers know not to 'block' the Event Loop, but they don't necessarily understand why. You will be one of the engineers who can clearly articulate the performance profile of Node and its Event Loop.
Caching with Redis: We'll also supercharge the performance of database queries by implementing caching backed by Redis. No previous experience of Redis is required! Redis is an in-memory data store purpose built for solving caching needs. By adding caching to your application, you can decrease the amount of time that any given request takes, improving the overall response time of your app.
File Upload: There are many resources online that offer suggestions on how to handle file upload, but few show a solution that can truly scale. Hint: saving files directly on your server isn't a scalable solution! Learn how to leverage AWS S3 to implement file upload that can scale to millions of users with a few dozen lines of simple code. Plentiful discussions are included on security concerns with handling uploads, as well.
Continuous Integration Testing: This is a must have feature for any serious production app. We'll first learn how to test huge swaths of our codebase with just a few lines of code by using Puppeteer and Jest. After writing many effective tests, we'll enable continuous integration on Travis CI, a popular - and free - CI platform. Testing can sometimes be boring, so we'll use this section to brush up on some advanced Javascript techniques, including one of the only legitimate uses of ES2015 Proxies that you'll ever see!
Here's what we'll learn:
- Master the Node Event Loop - understand how Node executes your source code.
- Understand the purpose of Node, and how the code you write is eventually executed by C++ code in the V8 engine
- Add a huge boost to performance in your Node app through clustering and worker threads
- Turbocharge MongoDB queries by adding query caching backed by a lightning-fast Redis instance
- Scale your app to infinity with image and file upload backed by Amazon's S3 file service
- Implement a continuous integration testing pipeline so you always know your project functions properly
- Think you know everything there is about managing cookies and session? Well, you might, but learn even more!
- Ensure your app works the way you expect with automated browser testing using Jest and Puppeteer
- Bonus - learn advanced JS techniques along the way, including where to use ES2015 proxies!
I've built the course that I would have wanted to take when I was learning to Node. A course that explains the concepts and how they're implemented in the best order for you to learn and deeply understand them.
- Basic knowledge of Node, Express, and MongoDB
- Strong knowledge of Javascript
- Anyone who wants a deep mastery of Node
- Engineers looking to understand the internals of Node
- Programmers looking to improve Node's performance
What you'll learn:
- Absolutely master the Event Loop and understand each of its stages
- Utilize Worker Threads and Clustering to dramatically improve the performance of Node servers
- Speed up database queries with caching for MongoDB backed by Redis
- Add automated browser testing to your Node server, complete with continuous integration pipeline setup
- Apply scalable image and file upload to your app, utilizing AWS S3
Watch Online Node JS: Advanced Concepts
# | Title | Duration |
---|---|---|
1 | How to Get Help | 01:08 |
2 | Starting With Node Internals | 03:48 |
3 | Module Implementations | 08:24 |
4 | Node Backed by C++! | 06:34 |
5 | The Basics of Threads | 06:30 |
6 | The Node Event Loop | 06:35 |
7 | The Event Loop Implementation | 07:23 |
8 | Event Loop Ticks | 06:48 |
9 | Is Node Single Threaded? | 05:07 |
10 | Testing for Single Threads | 06:50 |
11 | The Libuv Thread Pool | 03:08 |
12 | Threadpools with Multithreading | 06:07 |
13 | Changing Threadpool Size | 05:26 |
14 | Common Threadpool Questions | 03:17 |
15 | Explaining OS Operations | 03:53 |
16 | Libuv OS Delegation | 03:20 |
17 | OS/Async Common Questions | 02:41 |
18 | Review | 02:49 |
19 | Crazy Node Behavior | 07:40 |
20 | Unexpected Event Loop Events | 11:24 |
21 | Enhancing Performance | 02:19 |
22 | Express Setup | 03:23 |
23 | Blocking the Event Loop | 07:08 |
24 | Clustering in Theory | 05:12 |
25 | Forking Children | 05:38 |
26 | Clustering in Action | 05:34 |
27 | Benchmarking Server Performance | 05:17 |
28 | Benchmark Refactor | 03:56 |
29 | Need More Children! | 16:43 |
30 | PM2 Installation | 02:54 |
31 | PM2 Configuration | 06:50 |
32 | Webworker Threads | 02:28 |
33 | Worker Threads in Action | 11:35 |
34 | Benchmarking Workers | 05:18 |
35 | The Next Phase | 02:13 |
36 | Project Walkthrough | 08:43 |
37 | Key Customization | 03:12 |
38 | MongoDB Creation | 06:24 |
39 | Routes Walkthrough | 04:19 |
40 | MongoDB Query Performance | 10:43 |
41 | Query Caching Layer | 07:56 |
42 | Redis Introduction | 03:23 |
43 | Installing Redis on MacOS | 03:20 |
44 | Getting and Setting Basic Values | 06:48 |
45 | Redis Hashes | 06:58 |
46 | One Redis Gotcha | 02:54 |
47 | Cache Keys | 07:48 |
48 | Promisifying a Function | 08:08 |
49 | Caching in Action | 08:00 |
50 | Caching Issues | 05:27 |
51 | The Ultimate Caching Solution | 21:00 |
52 | Patching Mongoose's Exec | 10:43 |
53 | Restoring Blog Routes Handler | 02:06 |
54 | Unique Keys | 06:01 |
55 | Key Creation | 03:10 |
56 | Restoring Redis Config | 01:29 |
57 | Cache Implementation | 04:28 |
58 | Resolving Values | 05:47 |
59 | Hydrating Models | 06:27 |
60 | Hydrating Arrays | 03:28 |
61 | Toggleable Cache | 08:42 |
62 | Cache Expiration | 02:31 |
63 | Forced Cache Expiration | 05:16 |
64 | Nested Hashes | 06:47 |
65 | Clearing Nested hashes | 04:37 |
66 | Automated Cache Clearing with Middlware | 06:40 |
67 | Testing Flow | 06:39 |
68 | Testing Challenges | 03:26 |
69 | Commands Around Testing | 03:14 |
70 | First Jest Test | 04:27 |
71 | Launching Chromium Instances | 09:51 |
72 | Chromium Navigation | 04:32 |
73 | Extracting Page Content | 05:04 |
74 | Puppeteer - Behind the Scenes | 04:45 |
75 | DRY Tests | 03:24 |
76 | Browser Termination | 01:35 |
77 | Asserting OAuth Flow | 07:31 |
78 | Asserting URL Domain | 03:00 |
79 | Issues with OAuth | 05:12 |
80 | Solving Authentication Issues with Automation Testing | 04:37 |
81 | The Google OAuth Flow | 07:17 |
82 | Inner Workings of Sessions | 12:07 |
83 | Sessions From Another Angle | 08:23 |
84 | Session Signatures | 11:34 |
85 | Generating Sessions and Signatures | 09:04 |
86 | Assembling the Pieces | 08:08 |
87 | WaitFor Statements | 09:18 |
88 | Factory Functions | 05:00 |
89 | The Session Factory | 08:49 |
90 | Assembling the Session Factory | 03:04 |
91 | Code Separation | 06:23 |
92 | Global Jest Setup | 07:01 |
93 | Testing Factory Tests! | 03:42 |
94 | Adding a Login Method | 05:02 |
95 | Extending Page | 07:59 |
96 | Introduction to Proxies | 07:47 |
97 | Proxies in Action | 12:11 |
98 | Combining Object Property Access | 08:18 |
99 | Combining the Page and Browser | 08:38 |
100 | Custom Page Implementation | 04:17 |
101 | Function Lookup Priority | 04:27 |
102 | Gee, I Hope This Works! | 05:33 |
103 | Reusable Functions on Page | 05:39 |
104 | Testing Blog Creation | 04:54 |
105 | Default Navigation | 05:32 |
106 | Asserting Form Display | 06:00 |
107 | Test Timeout | 05:24 |
108 | Common Test Setup | 05:08 |
109 | Nested Describes for Structure | 07:01 |
110 | Asserting Validation Errors | 07:40 |
111 | Asserting Form Confirmation | 06:26 |
112 | Asserting Blog Creation | 05:58 |
113 | Options for Testing Prohibited Actions | 03:33 |
114 | Direct API Requests | 11:45 |
115 | Executed Arbitrary JS in Chromium | 03:55 |
116 | Asserting Page Response | 07:25 |
117 | Get Restrictions | 02:45 |
118 | A Final 'GET' Test | 02:57 |
119 | Super Advanced Test Helpers | 31:46 |
120 | Introduction to CI | 07:25 |
121 | CI Providers | 06:22 |
122 | The Basics of YAML Files | 04:23 |
123 | Travis YAML Setup | 10:05 |
124 | More Travis YAML | 05:09 |
125 | Client Builds | 06:38 |
126 | Script Config | 06:19 |
127 | Using Travis Documentation | 05:45 |
128 | More Server Configuration | 13:27 |
129 | A Touch More Configuration | 03:12 |
130 | Git Repo Setup | 04:19 |
131 | Travis CI Setup | 02:16 |
132 | Triggering CI Builds | 02:49 |
133 | Build Success | 01:20 |
134 | Image Upload | 02:16 |
135 | Big Issues Around Image Upload | 04:41 |
136 | Alternate Image Upload | 03:34 |
137 | Chosen Storage Solution | 04:18 |
138 | Upload Constraints | 04:27 |
139 | Image File Transport | 06:05 |
140 | Upload Flow with AWS S3 | 05:23 |
141 | Details of the Presigned URL | 06:48 |
142 | Security Issues Solved with Presigned URL's | 04:16 |
143 | Adding an Image Picker | 03:32 |
144 | Handling File Changes | 04:40 |
145 | Recording Image Files | 04:39 |
146 | The SubmitBlog Function | 03:08 |
147 | AWS Credentials with IAM | 05:09 |
148 | Creating S3 Buckets | 03:17 |
149 | Allowing Actions with IAM Policies | 08:18 |
150 | Creating IAM Users | 04:42 |
151 | Upload Routes Files | 05:15 |
152 | Configuring the AWS SDK | 03:19 |
153 | GetSignedURL Arguments | 10:07 |
154 | Calling GetSignedURL | 09:36 |
155 | Viewing the Signed URL | 02:18 |
156 | Attempting Image Upload | 08:00 |
157 | Handling CORS Errors | 05:21 |
158 | Outstanding Issues | 01:40 |
159 | S3 Bucket Policies | 05:02 |
160 | Tying Uploads to Blogs | 04:19 |
161 | Ensuring Images get Tied | 02:02 |
162 | Displaying Images | 04:07 |
Similar courses to Node JS: Advanced Concepts
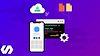
Redis: The Complete Developer's Guide
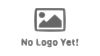
Complete Node.js Developer in 2023: Zero to Mastery
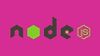
Node.js for Beginners - Become a NodeJs Developer + Project
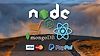
React Node FullStack - Ecommerce from Scratch to Deployment
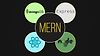
React, NodeJS, Express & MongoDB - The MERN Fullstack Guide
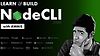
Node.js CLI | Build Node.js Command-line Automation Dev-tools
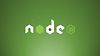
Learn and Understand NodeJS
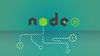
NodeJS - The Complete Guide (incl. MVC, REST APIs, GraphQL)
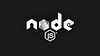