Rust Programming: The Complete Developer's Guide
26h 7m 19s
English
Paid
September 10, 2024
Learn the Rust programming language from scratch! Learn how to code & build real-world applications using Rust so that you can get hired and be recognized as a top programmer. No previous experience needed.
More
- Fundamentals of computer programming concepts such as conditional logic, loops, and data transformations
- Foundational computer science topics such as computer memory, program logic, and simple data structures
- Working with data: enums, structs, tuples, expressions, optional data and more
- Solid understanding of all core concepts of the Rust programming language such as: memory, mutability, traits, slices, and generics
- Reading and writing application code in the Rust programming language
- Utilization of the Rust ecosystem to develop applications more efficiently
- How to translate real-life requirements into working applications to solve real-world problems (and that you can add to your portfolio)
- How to make your programs reliable through the use of automated testing and by leveraging features of the Rust language
Watch Online Rust Programming: The Complete Developer's Guide
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Rust Programming: The Complete Developer's Guide | 02:47 |
2 | Intro | 02:27 |
3 | Data Types | 02:26 |
4 | Variables | 06:36 |
5 | Functions | 08:13 |
6 | println! | 03:05 |
7 | Control Flow with If | 08:39 |
8 | Repetition | 06:31 |
9 | Setup Rust | 04:50 |
10 | Comments | 03:37 |
11 | Activity: Functions | 07:09 |
12 | Numeric Types & Basic Arithmetic | 03:37 |
13 | Activity: Basic Math | 05:28 |
14 | Control Flow: If & Else | 02:34 |
15 | Activity: Logic with If & Else 1 | 04:00 |
16 | Activity: Logic with If & Else 2 | 05:01 |
17 | Match Expression | 04:26 |
18 | Demo: Basic Match | 03:12 |
19 | Activity: Basic Match 1 | 04:04 |
20 | Activity: Basic Match 2 | 05:12 |
21 | The Loop Expression | 04:49 |
22 | Activity: Loops | 04:59 |
23 | The While Loop | 03:23 |
24 | Activity: While Loops | 04:46 |
25 | Enums | 03:12 |
26 | Demo: Enums | 03:44 |
27 | Activity: Enums | 06:10 |
28 | Structs | 02:48 |
29 | Demo: Structs | 02:52 |
30 | Activity: Structs | 09:28 |
31 | Tuples | 03:32 |
32 | Demo: Tuples | 05:50 |
33 | Activity: Tuples | 05:56 |
34 | Expressions | 03:50 |
35 | Demo: Expressions | 05:10 |
36 | Activity: Expressions | 07:12 |
37 | Intermediate Memory Concepts | 03:35 |
38 | Ownership | 06:18 |
39 | Demo: Ownership | 06:07 |
40 | Activity: Ownership | 04:58 |
41 | Implementing Functionality | 09:21 |
42 | Activity: Implementing Functionality | 16:31 |
43 | The Vector Data Structure | 04:54 |
44 | Vector Basics & For Loops | 02:29 |
45 | Activity: Vectors & For Loops | 06:48 |
46 | About Strings | 04:01 |
47 | Demo: Strings | 04:29 |
48 | Activity: Strings | 07:24 |
49 | Deriving Functionality | 06:09 |
50 | Type Annotations | 04:08 |
51 | Enums Revisited | 04:00 |
52 | Demo: Advanced Match | 07:58 |
53 | Activity: Advanced match | 10:43 |
54 | The Option Type | 06:27 |
55 | Demo: Option | 04:27 |
56 | Activity: Option | 05:02 |
57 | Generating Documentation | 02:25 |
58 | Standard Library API docs | 03:34 |
59 | Activity: Standard Library API docs | 03:47 |
60 | The Result Type | 04:41 |
61 | Demo: Result | 14:17 |
62 | Activity: Result | 06:41 |
63 | Activity: Result & The Question Mark Operator | 07:52 |
64 | The HashMap Data Structure | 04:21 |
65 | Working With HashMaps | 05:33 |
66 | Activity: HashMap Basics | 08:27 |
67 | Basic Closures | 04:42 |
68 | Map Combinator | 05:02 |
69 | Activity: Map Combinator | 08:08 |
70 | Option Combinator Pattern | 07:50 |
71 | Activity: Option Combinators | 04:24 |
72 | Using Iterators | 09:13 |
73 | Activity: Using Iterators | 05:15 |
74 | Ranges | 01:59 |
75 | If..let..else | 02:59 |
76 | while..let | 02:07 |
77 | Inline Modules | 04:03 |
78 | Activity: Inline Modules | 10:18 |
79 | Testing | 06:53 |
80 | Activity: Testing | 07:43 |
81 | External Crates | 06:29 |
82 | Activity: Adding an External Crate | 04:46 |
83 | External Modules | 10:28 |
84 | Activity: External Modules | 09:55 |
85 | Gathering User Input | 14:22 |
86 | Activity: Gathering User Input | 22:48 |
87 | Mini Project: Introduction | 02:45 |
88 | Retrieve User Input | 03:17 |
89 | Creating The Main Menu Loop | 07:15 |
90 | Required Data Structures | 04:11 |
91 | Implementation: Adding & Viewing Bills | 13:01 |
92 | Implementation: Removing Bills | 06:51 |
93 | Implementation: Editing Bills | 09:16 |
94 | Traits | 04:55 |
95 | Demo: Traits | 04:59 |
96 | Activity: Traits | 05:59 |
97 | Implementing The "Default" Trait | 02:29 |
98 | Generics & Functions | 13:55 |
99 | Demo: Generics & Functions | 06:23 |
100 | Activity: Generics & Functions | 05:53 |
101 | Generic Structures | 10:07 |
102 | Generic Structures & impl Blocks | 07:59 |
103 | Demo: Generics & Structures | 06:39 |
104 | Activity: Generics & Structures | 10:19 |
105 | Advanced Memory Concepts | 08:51 |
106 | Trait Objects | 11:36 |
107 | Demo: Trait Objects | 07:27 |
108 | Activity: Trait Objects | 08:36 |
109 | Ownership & Lifetimes | 09:04 |
110 | Demo: Lifetimes | 08:33 |
111 | Activity: Lifetimes & Structures | 10:50 |
112 | Activity: Lifetimes & Functions | 04:48 |
113 | Custom Error Types | 08:52 |
114 | Demo: Custom Error Types | 08:54 |
115 | Activity: Creating a Custom Error | 09:02 |
116 | const | 02:21 |
117 | New Type Pattern | 05:08 |
118 | Activity: Utilizing The New Type Pattern | 08:09 |
119 | TypeState Pattern | 04:14 |
120 | Demo: TypeState Pattern | 11:49 |
121 | Activity: TypeState Pattern | 07:42 |
122 | Demo: Match Guards & Binding | 06:23 |
123 | Activity: Match Guards & Binding | 08:46 |
124 | Arrays & Slices | 07:49 |
125 | Slice Patterns | 05:54 |
126 | Activity: Slices | 05:42 |
127 | Type Aliases | 05:23 |
128 | Exercise: Imposter Syndrome | 02:56 |
129 | From/Into | 08:18 |
130 | TryFrom/TryInto | 03:57 |
131 | Demo: From/Into | 07:59 |
132 | Activity: TryFrom/TryInto | 10:16 |
133 | Numeric Limits & Numeric Type Casting | 08:00 |
134 | Passing Closures to Functions | 10:54 |
135 | Threads | 06:45 |
136 | Demo: Threads | 07:40 |
137 | Activity: Threads | 04:30 |
138 | Channels | 08:14 |
139 | Demo: Channels | 05:05 |
140 | Demo: Bidirectional Threaded Communication | 07:29 |
141 | Activity: Channels | 09:24 |
142 | Smart Pointers | 04:08 |
143 | Interior Mutability: Cell & RefCell | 08:07 |
144 | Demo: Smart Pointers & RefCell | 06:01 |
145 | Activity: Smart Pointers & ReffCell | 09:15 |
146 | Arc/Mutex | 08:39 |
147 | Threading: Deadlocks | 06:28 |
148 | Demo: Arc/Mutex | 04:16 |
149 | Activity: Arc/Mutex | 05:14 |
150 | Enum Equality & Ordering | 03:43 |
151 | Struct Equality & Ordering | 04:24 |
152 | Operator Overloading | 07:25 |
153 | Iterators: Implementing Iterator for a Struct | 03:32 |
154 | Implement IntoIterator | 07:59 |
155 | Demo: Implementing IntoIterator | 09:24 |
156 | Activity: Implementing Iterator | 05:46 |
157 | Iterators: Custom Iteration Logic | 08:08 |
158 | Helpful Macros | 07:49 |
159 | Managing Integer Overflow | 05:40 |
160 | Turbofish | 02:30 |
161 | Loop Labels | 02:45 |
162 | Loop Expressions | 02:35 |
163 | Struct Update Syntax | 02:48 |
164 | Escape Sequences & Raw Strings | 04:18 |
165 | rust-analyzer | 04:19 |
166 | clippy | 02:32 |
167 | error-lens | 01:28 |
168 | dotenvy | 02:33 |
169 | serde | 03:57 |
170 | rand | 04:21 |
171 | cached | 04:17 |
172 | regex | 05:18 |
173 | chrono | 07:32 |
174 | strum | 04:25 |
175 | derive_more | 06:33 |
176 | rayon | 04:01 |
177 | tracing | 06:30 |
178 | color-eyre | 04:16 |
179 | Async Primer | 07:52 |
180 | Introduction To The Project | 01:04 |
181 | Architecture | 03:39 |
182 | Walkthrough & Domain Structure Modules | 07:26 |
183 | Domain Errors | 06:03 |
184 | Domain Implementation: Content & Hits | 06:37 |
185 | Domain Implementation: Password | 06:28 |
186 | Domain Implementation: Shortcode | 07:33 |
187 | Domain Implementation: Title | 02:41 |
188 | Domain Implementation: Time | 06:49 |
189 | Domain Implementation: Expire & Posted | 05:04 |
190 | Domain Implementation: Dbld & Clipld | 06:01 |
191 | Recap & Error Correction | 05:19 |
192 | Database Type Aliases | 07:26 |
193 | Database Wrapper | 04:13 |
194 | Database Model | 11:57 |
195 | SQL Primer | 04:26 |
196 | sqlx cli | 01:43 |
197 | Database Query: Get Clip | 06:56 |
198 | Database Query: New Clip | 07:07 |
199 | Database Query: Update Clip | 03:52 |
200 | Recap & Next Steps | 04:19 |
201 | Service Layer: Errors | 07:35 |
202 | Service Layer: Get Clip | 11:23 |
203 | Service Layer: Add & Update Clip | 07:22 |
204 | Templates | 02:01 |
205 | Page Contexts | 06:16 |
206 | Template Renderer | 14:31 |
207 | Rocket Framework | 04:29 |
208 | Initial Web Setup | 03:47 |
209 | Web Forms | 09:20 |
210 | Homepage, Catchers, and Router | 05:46 |
211 | Rocket Configuration | 03:35 |
212 | Running the Server | 09:35 |
213 | Retrieving a Clip | 11:06 |
214 | Saving a Clip | 13:15 |
215 | Password Protected Clips | 07:13 |
216 | Raw Clips | 05:32 |
217 | Hit Counter | 01:40 |
218 | Hit Counter - Service & Data | 11:39 |
219 | Hit Counter Implementation part 1 | 12:28 |
220 | Hit Counter Implementation part 2 | 05:59 |
221 | Database Migration | 01:12 |
222 | API: Keys & Error Handling | 09:20 |
223 | API: Service & Queries | 07:11 |
224 | API: Request Guard | 06:44 |
225 | API: Routing | 12:07 |
226 | API Client: Cargo.toml & CLI Options | 08:39 |
227 | API Client: Get Clip & New Clip | 07:43 |
228 | API Client: Update Clip & Making Requests | 06:08 |
229 | Maintenance Tasks | 07:07 |
230 | Testing: Database | 09:15 |
231 | Testing: HTTP Routes | 10:01 |
232 | Overview | 06:55 |
233 | Detail | 15:40 |
234 | Demo: impl Blocks | 06:39 |
235 | Activity: Control Flow | 02:52 |
236 | Activity: impl Blocks | 02:35 |
237 | Repetitions | 08:48 |
238 | Demo: Repetitions | 03:15 |
239 | Activity: HashMap | 05:23 |
240 | Demo: Syntax Extension | 08:53 |
241 | Activity: Syntax Extension | 04:12 |
242 | Activity: Generating Tests | 06:32 |
243 | Activity: Function Tracer | 04:48 |
244 | Demo: Checked Config | 13:19 |
245 | Demo: Recursive tt Muncher | 06:41 |
246 | Thank You! | 01:18 |
Similar courses to Rust Programming: The Complete Developer's Guide
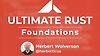
Ultimate Rust Foundations
Duration 17 hours 53 minutes 36 seconds
Course
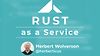
Rust as a Service
Duration 6 hours 13 minutes 47 seconds
Course
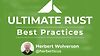
Ultimate Rust Best Practices
Duration 1 hour 39 minutes 25 seconds
Course
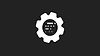
Rust: Building Reusable Code with Rust from Scratch
Duration 6 hours 17 minutes 32 seconds
Course
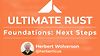
Ultimate Rust: Foundations - Next Steps
Duration 50 minutes 48 seconds
Course
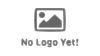
Rust from C/C++
Duration 5 hours 31 minutes 48 seconds
Course
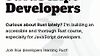
Rust for JavaScript Developers
Duration
Book
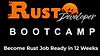
Rust Developer Bootcamp
Duration 9 hours 50 minutes 20 seconds
Course