Rust Developer Bootcamp
Who is this Bootcamp for? Experienced Developers trying to switch to Rust. Learn the language of the future.
Your time is valuable (literally, $148,688/yr). Learn Rust FAST with the Bootcamp, instead of digging through low-quality resources. Students/Graduates looking for a job. Learning Rust is a great resume builder. Stand out from other candidates. Become a competent programmer. Get access to a community that can help you land your first job. And... You! Rust enthusiast. Rust is an innovative language that will expand your way of thinking Cutting-edge technologies are built in Rust.
Read more about the course
You'll learn how to setup your development environment, configure your IDE and install the right plugins. Then you'll create your first Rust project!
- How to Setup Your Environment
- Setting Up Your IDE & Plugins
- Creating Rust Packages With Cargo
- Variables & Data Types in Rust
- Constant & Static Variables
- Functions and Control Flow
Understanding memory management is critical when learning Rust! In this section you'll learn about memory management from the ground up!
- Rust-Based CS Masterclass
- How Ownership Works
- How Borrowing Works
- What Slices Are
- String Types (str, &str, and String)
Rust doesn't have classes or objects! Instead, Rust uses Structs and Enums for custom data types. In this section you will learn about creating your own types!
- How To Create Structs & Enums
- How To Use Implementation Blocks
- Pattern Matching
- The Option and Result Enum
- Vectors
How To Structure Your Rust Projects
In this section I will explain how modules work in an easy to understand way. I'll also go over structuring larger projects and publishing your work to Crates.io.
- Project Structure Overview
- The Module System
- External Dependencies
- Conditional Compilation
- How to Structure Larger Projects
- Publishing Your Rust Projects
How Test & Document Your Code
Tests are a critical part of writing robust code. In this section you'll learn how to write and structure unit & integration tests in Rust. We'll also cover documentation.
- How To Write Unit Tests
- How to Write Integration Tests
- How To Structure Your Tests
- Documentation Comments
Polymorphism With Generics & Traits
Rust does not support classical inheritance! Instead, polymorphism is achieved through generics and traits. In this section you'll learn how both work!
- How To Define Generics
- The Trait System
- Traits Bounds & Trait Objects
- Supertraits
- Static Dispatch vs Dynamic Dispatch
- How To Derive Traits
- Traits in the Standard Library
Advanced Memory Management
Memory management doesn't end with ownership and borrowing! In this section you'll learn about lifetimes! We'll also cover various smart pointers in Rust.
- Concrete Lifetimes
- Generic Lifetimes Anotations
- Liftimes in Functions And Structs
- Smart Pointers & Why They Are Useful
- Implicit Deref Coericon
Understanding Error Handling In Rust
Understanding error handling is critical when designing your Rust applications. In this section we will cover how error handling works in Rust.
- How To Throw Unrecoverable Errors
- How To Return Recoverable Errors
- How To Propagate Errors
- The Result And Option Enums
- How To Handle Multiple Error Types
- How To Use anyhow & thiserror
Functional Features In Rust
One of the most powerful things about Rust is its functional features. In this section we will cover closures, function pointers, iterators, and more!
- Closures & Function Pointers
- The Iterator Pattern In Rust
- Implementing The Iterator Pattern
- How To Iterate Over Collections
- Combinators And How To Use Them
Concurrency & async/.await
This is probably the MOST IMPOTANT section. Understanding concurrency is critical, especially when using rust for web related projects.
- Basic Concurrency Concepts
- Creating Threads
- Message Passing With Channels
- Sharing State With Mutex
- The async/.await Model
- How Futures Work
- What Is An Async Runtime
- What Is Tokio And How Is It Used
- What Are Tokio Tasks
- Running CPU Blocking Code
- Streams
Rust's Powerful Macro System
Macros are an extremely powerful way to extend the syntax of a language. In this section you'll learn about the Macro system in Rust from the ground up!
- The Compilation Process
- What Macros Are
- How To Create Declarative Macros
- How To Create Precedural Macros
- Function-Like Procedural Macros
- Attribute-Like Procedural Macros
- Custom Derive Procedural Macros
Unsafe Rust & FFI
One huge benefit of Rust is its ability to interface with other languages through a foreign function interface. In this section you'll learn about unsafe Rust and the FFI!
- Unsafe Rust And When To Use It
- How To Dereference Raw Pointers
- What Are Unsafe Functions
- What Are Unsafe Traits
- How To Mutate Static Variables
- How To Write Inline Assembly In Rust
- How To Call C Code From Rust
- How To Call Rust Code From C
Watch Online Rust Developer Bootcamp
# | Title | Duration |
---|---|---|
1 | 001. Don't skip this video! | 07:21 |
2 | 002. How to use this Bootcamp effectively | 05:44 |
3 | 003. Hello World | 03:14 |
4 | 004. Variables | 03:56 |
5 | 005. Data Types | 03:34 |
6 | 006. Constants & Statics | 02:44 |
7 | 007. Functions | 03:27 |
8 | 008. Control Flow | 03:21 |
9 | 009. Comments | 00:50 |
10 | 010. Stack, Heap, and Static Memory | 07:18 |
11 | 011. Memory Management Strategies | 03:47 |
12 | 012. C++ RAII vs Rust OBRM - Part 1 | 10:53 |
13 | 013. C++ RAII vs Rust OBRM - Part 2 | 05:19 |
14 | 014. Ownership | 06:32 |
15 | 015. Ownership Continued | 07:18 |
16 | 016. Borrowing | 10:29 |
17 | 017. Slices | 09:03 |
18 | 018. BONUS Masterclass Strings in Rust. PART 1 | 10:09 |
19 | 019. BONUS Masterclass Strings in Rust. PART 2 | 10:39 |
20 | 020. Structs | 03:30 |
21 | 021. Implementation Blocks | 07:56 |
22 | 022. Tuple Structs | 02:07 |
23 | 023. Enums | 06:18 |
24 | 024. Matching | 08:42 |
25 | 025. Option | 05:54 |
26 | 026. Result | 04:48 |
27 | 027. Vectors | 07:22 |
28 | 028. Project Structure Overview | 05:19 |
29 | 029. Modules | 14:01 |
30 | 030. Modules Continued | 08:19 |
31 | 031. External Dependencies | 03:15 |
32 | 032. Publishing Your Package | 06:11 |
33 | 033. Cargo Features | 07:04 |
34 | 034. Cargo Workspaces | 05:39 |
35 | 035. Unit Tests | 12:25 |
36 | 036. Integration Tests | 03:49 |
37 | 037. Documentation | 02:26 |
38 | 038. BONUS Benchmark Testing | 04:32 |
39 | 039. Generics | 11:42 |
40 | 040. Traits | 05:48 |
41 | 041. Trait Bounds | 05:18 |
42 | 042. Supertraits | 01:39 |
43 | 043. Trait Objects | 06:48 |
44 | 044. Deriving Traits | 02:46 |
45 | 045. The Orphan Rule | 02:59 |
46 | 046. Concrete Lifetimes | 05:55 |
47 | 047. Generic Lifetimes | 09:35 |
48 | 048. Structs & Lifetime Elision | 08:06 |
49 | 049. Box Smart Pointer | 05:29 |
50 | 050. Rc Smart Pointer | 03:39 |
51 | 051. RefCell Smart Pointer | 04:57 |
52 | 052. Deref Coercion | 06:14 |
53 | 053. Unrecoverable Errors | 02:02 |
54 | 054. Recoverable Errors | 04:08 |
55 | 055. Propagating Errors | 04:08 |
56 | 056. Result and Option | 04:49 |
57 | 057. Multiple Error Types | 05:42 |
58 | 058. Overview of Error Handling | 04:47 |
59 | 059. Basic Error Handling | 11:50 |
60 | 060. Custom Errors 1 | 06:23 |
61 | 061. Custom Errors 2 | 08:42 |
62 | 062. Custom Errors 3 | 07:00 |
63 | 063. thiserror & anyhow | 05:14 |
64 | 064. BONUS error-stack | 23:20 |
65 | 065. Closures | 12:22 |
66 | 066. Closures Continued | 05:57 |
67 | 067. Function Pointers | 04:42 |
68 | 068. Iterator Pattern | 06:50 |
69 | 069. Iterator Pattern Continued | 05:19 |
70 | 070. Iterating Over Collections | 02:09 |
71 | 071. Combinators | 11:07 |
72 | 072. Intro to Concurrency | 08:19 |
73 | 073. Creating Threads | 03:29 |
74 | 074. Moving Values Into Threads | 01:02 |
75 | 075. Message Passing Between Threads | 04:50 |
76 | 076. Sharing State Between Threads | 02:49 |
77 | 077. Sharing State Between Threads Continued | 03:33 |
78 | 078. Send & Sync Traits | 02:07 |
79 | 079. async.await Basics | 08:38 |
80 | 080. Tokio Tasks | 04:47 |
81 | 081. CPU Intensive Code | 02:12 |
82 | 082. Streams | 02:10 |
83 | 083. Intro to Macros | 05:08 |
84 | 084. Declarative Macros | 03:00 |
85 | 085. Declarative Macros Continued | 06:39 |
86 | 086. Procedural Macros | 01:50 |
87 | 087. Procedural Macros - Function Like | 04:51 |
88 | 088. Procedural Macros - Custom Derive | 07:18 |
89 | 089. Procedural Macros - Attribute Like | 07:09 |
90 | 090. Procedural Macros - Attribute Continued | 07:11 |
91 | 091. Unsafe Basics | 01:46 |
92 | 092. Dereferencing a Raw Pointer | 02:48 |
93 | 093. Calling an Unsafe Function | 01:38 |
94 | 094. Implementing an Unsafe Trait | 01:10 |
95 | 095. Mutable Static Variables | 01:29 |
96 | 096. Inline Assembly | 01:10 |
97 | 097. FFI C from Rust | 03:31 |
98 | 098. FFI Rust from C | 01:45 |
99 | 099. BONUS FFI Rust from Python | 07:36 |
100 | 100. What are microservices | 06:19 |
101 | 101. Why Rust is great for microservices | 02:40 |
102 | 102. Communication with gRPC | 05:02 |
103 | 103. Containerization with Docker | 08:03 |
104 | 104. CICD with GitHub Actions | 02:19 |
105 | 105. Cloud Providers | 03:21 |
Similar courses to Rust Developer Bootcamp
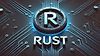
Rust: The Complete Developer's GuideudemyStephen Grider
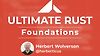
Ultimate Rust Foundationsardanlabs.com
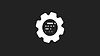
Rust: Building Reusable Code with Rust from Scratchudemy
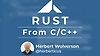
Rust from C/C++ardanlabs.com
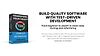
Transform Your Craft with TDD: Master clean code and testingDaniel Moka
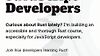
Rust for JavaScript DevelopersSidhartha Chatterjee
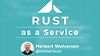
Rust as a Serviceardanlabs.com
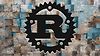
Rust Programming: The Complete Developer's Guidezerotomastery.io
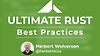