Testing Java with JUnit 5 & Mockito
Unit Testing is a must-have skill and this video course is about unit testing. If you take this video course, you will learn how to test your Java code using JUnit 5 and Mockito framework. This video course is for beginners and you do not need to have any prior Unit testing knowledge to enrol in this course. This video course teaches Unit and Integration testing with Java from the very beginning and covers many advanced topics as well.
Read more about the course
JUnit 5 in Different Java projects
There are different Java projects, build tools and development environments. In this course, you will learn how to create a new project and configure JUnit 5 support for different types of projects, using different development environments and build tools.
You will learn how to create a Unit test in:
IntelliJ IDEA and
Eclipse Development environments.
You will learn how to create a Unit test in:
Regular Java project,
Maven-based Java project,
Cradle-based Java project.
By the end of this course, you will learn:
JUnit 5 basics, and
JUnit 5 advanced topics.
Once you become familiar with JUnit 5, you will learn to use:
Test-Driven Development(TDD)
You will then learn to use another very popular Test framework for Java called:
Mockito
You will also learn how to write:
Spring Boot integration tests
By the end of this course you will learn and be able to use all of the following:
Create Unit Tests in IntelliJ,
Create Unit Tests in Eclipse,
Run unit tests using Gradle,
Run Unit Tests using Maven,
Use @DisplayName annotation,
Use JUnit assertions,
Test for Exceptions,
Use Lifecycle methods (@BeforeAll, @BeforeEach, @AfterEach, @AfterAll),
Run unit tests in any order you need: (Random, Order by Name, Order by Index),
Disable Unit test,
Repeated Tests with @RepeatedTest annotation,
Parameterized tests with @Parameterized annotation
@ValueSource,
@MethodSource,
@CsvSource,
@CsvFileSource
Change Test Instance lifecycle with @TestInstance (PER_CLASS, PER_METHOD)
Learn to Mock objects with Mockito's @Mock annotation,
Learn to user Mockito's argument matches,
Mockito method stubbing,
Mockito Exception stubbing,
Verify method call,
Call Real Method,
Do nothing when a method is called,
Write integration tests for Spring Boot applications,
and more...
Watch Online Testing Java with JUnit 5 & Mockito
# | Title | Duration |
---|---|---|
1 | Introduction | 03:25 |
2 | What is a Unit Test? | 08:20 |
3 | Why write Unit Test? | 02:29 |
4 | The F.I.R.S.T Principle | 03:54 |
5 | Testing Code in Isolation | 04:33 |
6 | Testing Pyramid | 02:20 |
7 | What is JUnit 5? | 02:10 |
8 | JUnit and Build Tools | 01:03 |
9 | Create new Maven project using IntelliJ IDEA | 02:35 |
10 | Add JUnit Dependencies | 06:13 |
11 | Maven Surefire Plugin | 04:48 |
12 | Creating a new project | 02:43 |
13 | Add JUnit Dependencies | 05:08 |
14 | Executing Unit Test | 02:23 |
15 | Introduction | 00:41 |
16 | Basic Java project with IntelliJ | 06:33 |
17 | Basic Java project with Eclipse | 06:51 |
18 | Creating First Unit Test method | 04:56 |
19 | Assertions and Assertion message | 01:58 |
20 | Other assertions | 02:59 |
21 | Exercise solution overview | 03:27 |
22 | Lazy Assert Messages | 04:41 |
23 | Naming Unit Tests | 04:22 |
24 | @DisplayName annotation | 02:34 |
25 | Test Method Code Structure. Arrange, Act, Assert. | 04:13 |
26 | JUnit Test Lifecycle | 03:37 |
27 | Lifecycle methods demo | 06:17 |
28 | Disable Unit Test | 02:48 |
29 | Assert an Exception | 05:41 |
30 | @ParameterizedTest. Multiple Parameters with @MethodSource. | 07:39 |
31 | @ParameterizedTest. Multiple parameters with @CsvSource. | 04:23 |
32 | @ParameterizedTest + CSV file | 04:45 |
33 | @ParameterizedTest + @ValueSource annotation. | 02:46 |
34 | Repeated Tests | 07:32 |
35 | Methods Order - Random order | 02:36 |
36 | Methods Order - Order by name | 01:56 |
37 | Methods Order - Random by order index | 03:48 |
38 | Order of Unit Test Classes | 08:19 |
39 | Test Instance Lifecycle - Introduction | 01:56 |
40 | Changing Test Instance Lifecycle - example 1 | 04:57 |
41 | Test Instance Lifecycle Demo project overview | 05:03 |
42 | Test Instance Lifecycle Demo Project Implementation | 09:01 |
43 | Introduction | 03:05 |
44 | New project, Class, Method | 02:18 |
45 | Creating UserService | 03:35 |
46 | Test Create User method | 06:42 |
47 | Test User object contains first name | 04:15 |
48 | Refactor Test method | 02:16 |
49 | Exercise | 00:56 |
50 | Solution overview | 01:08 |
51 | Check if user id is set | 04:03 |
52 | Assert throws Exception | 07:53 |
53 | Exercise | 01:57 |
54 | Introduction | 03:32 |
55 | Adding Mocking to a project | 02:26 |
56 | Method under test overview | 01:34 |
57 | Implementing UsersRepository | 03:15 |
58 | Injecting UsersRepository as Dependency | 04:19 |
59 | Creating a Mock object | 02:43 |
60 | Stubbing using built-in any() argument matcher | 05:02 |
61 | Verify method call | 06:35 |
62 | Exception stubbing | 05:36 |
63 | Creating EmailNotificationService class | 03:48 |
64 | Stub void method with Exception | 05:21 |
65 | Do nothing when method is called | 01:59 |
66 | Call real method | 04:01 |
67 | Introduction | 03:54 |
68 | Generating code coverage report | 05:12 |
69 | Export Code Coverage Report | 02:40 |
70 | Export Test Report using Maven | 06:54 |
71 | Jacoco - Maven Plugin for Code Coverage | 03:04 |
72 | Jacoco - Export Code Coverage Report in HTML format | 03:56 |
73 | Introduction to Unit Testing Spring Boot Application | 03:29 |
74 | Introduction to Integration Testing of Web Layer | 02:11 |
75 | Introduction to Integration Testing with All Layers | 01:48 |
76 | Adding Testing Support to Spring Boot Application | 04:55 |
77 | Existing Project overview + Source code | 08:11 |
78 | New Test Class. @WebMvcTest & @AutoConfigureMockMvc. | 05:24 |
79 | RequestBuilder - Building and Performing HTTP Request | 09:14 |
80 | @MockBean - Mocking Service Layer | 06:55 |
81 | @MockBean annotation - Trying how it works. | 04:25 |
82 | Assert for BAD_REQUEST | 07:36 |
83 | Practice exercise solution overview | 03:28 |
84 | Introduction | 01:00 |
85 | @SpringBootTest annotation | 02:03 |
86 | @SpringBootTest WebEnvironment MOCK | 01:38 |
87 | Defined Port Number | 03:43 |
88 | @TestPropertySource. Loading alternative configuration. | 06:35 |
89 | Random Port Number | 03:10 |
90 | Test Create User - User Details JSON | 02:19 |
91 | TestRestTemplate - Prepare & Perform HTTP Post Request | 07:37 |
92 | Trying how it works | 02:51 |
93 | Test JWT is Required | 06:04 |
94 | Test User Login Works | 10:10 |
95 | Order Test Methods | 03:49 |
96 | GET /users. Include JWT Token in the Request | 11:25 |
97 | Introduction to testing JPA Entities | 06:11 |
98 | JPA Entity Overview + Source Code | 03:49 |
99 | Test that UserEntity can be persisted | 08:09 |
100 | Test UserEntity cannot be persisted with invalid user's first name | 04:07 |
101 | Excersize - Test that UserId is Unique | 01:48 |
102 | Excersize solution + Source code | 03:44 |
103 | Introduction to testing JPA Repositories | 05:30 |
104 | Testing Find By Email Query Method | 05:35 |
105 | Excersize - Test Find By User Id Query Method | 01:15 |
106 | Excersize solution overview + Source code | 02:59 |
107 | Test JPQL Query + Source code | 05:53 |
Similar courses to Testing Java with JUnit 5 & Mockito
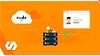
Machine Learning with JavascriptudemyStephen Grider
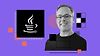
Java Data Structures & Algorithms + LEETCODE Exercisesudemy
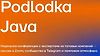
Java Crew #2podlodka.io
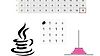
Algorithms in Java: Live problem solving & Design Techniquesudemy
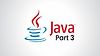
Ultimate Java Part 3: Advanced Topicscodewithmosh (Mosh Hamedani)
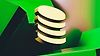
RESTful Web Services, Java, Spring Boot, Spring MVC and JPAudemy
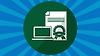
Oracle Java Certification - Pass the Associate 1Z0-808 Exam.udemy
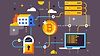
Learn Blockchain Technology & Cryptocurrency in Javaudemy
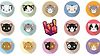
Catsrockthejvm.com
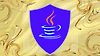