Mastering REST APIs in Node.js: Zero To Hero
Have you ever wondered about how REST APIs work? Have you tried to understand all the components that are required to create REST APIs? Are you a developer coming from a Java/PHP/Perl/Python background but now interested in Node.js? Did you want to know how relational databases work? Or how NoSQL works? How are they different - or even similar?
Read more about the course
Have you heard about JSON Web Tokens and token based authentication but wasn't sure how they work? Have you heard the latest buzzword GraphQL but wondered how it works?
Look no further -- this course answers all your questions! It teaches the very basics of REST APIs including its architecture and HTTP methods, to adding relational database and/or NoSQL support while also covering authentication via tokens as well as GraphQL. As a bonus, Section 10 (Appendix B) has an entire section on how to implement the REST API in an example application - including authentication for users.
The course is broken up into 10 distinctive sections.
SECTION 1 - Introduction to REST
An overview of the true basics of REST - including the architecture, HTTP methods and status codes and it also discusses why you'd want to develop a REST API at the first place.
SECTION 2 - Our first REST API in Node.js
This section takes a look at the basics around REST API and implements a simple API service using Node.js without using any external dependencies (yes, it only uses some of the built-in Node.js modules)
SECTION 3 - Express - a versatile web server for Node.js
In this section we learn about Express - the most popular web server framework for Node.js. We learn how to install it, how to handle routes, how to utilise the Router object for advanced routing. Later on we also take a look at how to work with static files, how to utilise query strings and query parameters - we'll implement these features later on when we create a REST API.
SECTION 4 - Relational Database Management Systems
It is crucial to understand data storage before we can create a functional REST API. This section shows us how to work with Relational Database Management Systems (MySQL, to be more precise) and also teaches us how SQL queries work, and how to manipulate data.
SECTION 5 - Create a REST API using a Relational Database
In this section of the course we learn about creating a REST API instance where we utilise the previously gathered knowledge about relational databases. The course uses MySQL, the native MySQL driver but later on we change to Knex.js which is a SQL Query Builder for Node.js
SECTION 6 - Creating a REST API using NoSQL
This part of the course takes a look at NoSQL - it introduces the student to NoSQL databases, compares them with Relational Databases. During this section we learn how to use MongoDB (the most popular NoSQL database) and utilise it to create another REST API.
SECTION 7 - Securing a REST API
Security plays an important factor in REST APIs. During this section we learn how token based authentication works, we get an introduction to JSON Web Tokens (JWT) and we take the previously created two REST APIs - one that uses a relational database, and the other that uses a NoSQL database - and we add JWT support for both.
SECTION 8 - GraphQL
GraphQL is gaining popularity and often times it is seen as a competitor for REST APIs. During this section we take a look at the basics of GraphQL and see how it can complement an existing REST API. The videos in this section not only show us the basics but it also shows us how to add the previously implemented authentication to GraphQL.
Section 9 (Appendix A) - Tooling
This section has a single video that introduces Insomnia - a tool that can be seen throughout the course for testing the REST API
Section 10 (Appendix B) - Application creation
Although not strictly related to REST APIs - this section teaches us how to create an application to consume the REST API created throughout the previous sections of the course. It walks us through CORS and its importance, describes environment variables and finally creates a rudimentary application that allows us to register users, login/logout and check a secret profile - it essentially implements our API including JWT authentication.
- JavaScript knowledge (including ES2015)
- Basic NodeJS knowledge
- Junior developers wishing to gather knowledge about REST APIs
- Senior Developers who want to create REST APIs using NodeJS and a pure JavaScript environment
- Developers interested in REST API design and GraphQL
- Developers wanting to understand REST APIs and Relational Databases
- Developers wanting to understand REST APIs and NoSQL
What you'll learn:
- Architecting and designing a REST API from scratch
- HTTP methods, HTTP verbs and HTTP status codes along with their use cases
- Introduction to NoSQL (MongoDB) - including queries
- Introduction to Relational Databases and SQL (MySQL)
- ExpressJS - including route handling and middleware
- How to secure a REST API using JWT
- GraphQL essentials
- Create an application to consume a REST API
Watch Online Mastering REST APIs in Node.js: Zero To Hero
# | Title | Duration |
---|---|---|
1 | Introduction to REST | 27:04 |
2 | HTTP Methods and Status Codes | 08:04 |
3 | Why develop (and use) a REST API? | 01:51 |
4 | Create a REST API without external dependencies | 17:20 |
5 | Introduction to Express | 01:38 |
6 | Install Express | 01:55 |
7 | Basic route handling | 05:26 |
8 | Advanced routing via the Router object | 03:30 |
9 | Working with Static Files | 02:16 |
10 | Utilising Query Strings | 02:16 |
11 | Utilising Query Parameters | 03:48 |
12 | Introduction to Relational Databases | 15:21 |
13 | Introduction to SQL | 05:17 |
14 | Install Workbench | 05:31 |
15 | Create a database and a table | 07:01 |
16 | Insert data | 02:37 |
17 | Update data | 01:45 |
18 | Delete data | 00:43 |
19 | Advanced SQL statements | 11:42 |
20 | SQL JOINs - joining information in two tables | 06:30 |
21 | Reviewing the final setup | 03:17 |
22 | Designing our REST API | 07:35 |
23 | Using the MySQL Native Driver | 06:59 |
24 | Select data via the MySQL Native Driver (Using SQL's SELECT statement) | 04:19 |
25 | Introduction to Knex.js | 05:21 |
26 | Extend the REST API by using Knex.js | 04:13 |
27 | Using Express Middlewares | 05:34 |
28 | Posting data to the database via Knex.js | 09:10 |
29 | Patching data in the database with Knex.js | 06:37 |
30 | Deleting data from the database with Knex.js | 03:21 |
31 | Handling missing routes | 02:43 |
32 | Student Challenge: Create the route handlers for Departments | 01:52 |
33 | Use JOINs with Knex.js | 11:02 |
34 | Utilise Query Strings | 06:56 |
35 | Return an object instead of an array | 01:42 |
36 | Introduction to NoSQL | 11:18 |
37 | Install and load data to MongoDB | 10:48 |
38 | Basic queries in MongoDB | 17:31 |
39 | Updates and Aggregates | 10:37 |
40 | Create a blank application | 02:13 |
41 | Display all data from MongoDB | 08:28 |
42 | Display a single Document from MongoDB | 06:47 |
43 | Insert data in NoSQL | 04:32 |
44 | insert() vs insertOne() in MongoDB | 02:41 |
45 | HTTP Patch for MongoDB | 03:59 |
46 | Delete data from MongoDB | 01:35 |
47 | Handling errors | 06:18 |
48 | Loading additional documents to MongoDB | 07:36 |
49 | Query Parameters for NoSQL | 06:12 |
50 | Introduction to JWT (JSON Web Tokens) | 08:05 |
51 | Implement JWT for a SQL based REST API | 23:47 |
52 | Implement JWT for a NoSQL based REST API | 11:48 |
53 | Introduction to GraphQL | 14:16 |
54 | Query that returns a collection | 20:29 |
55 | Query that returns a single data object | 04:34 |
56 | Execute subqueries using GraphQL | 10:34 |
57 | Execute subqueries for nested data using GraphQL | 04:20 |
58 | A note on performance for RDBMS and GraphQL | 05:58 |
59 | Authentication for GraphQL and our REST API | 06:34 |
60 | Using GraphQL with NoSQL | 20:06 |
61 | Introduction to Insomnia | 05:11 |
62 | CORS | 05:35 |
63 | Using environment variables the smart way | 04:36 |
64 | Create an application to consume our REST API - part 1 | 06:55 |
65 | Create an application to consume our REST API - part 2 | 13:55 |
66 | Create an application to consume our REST API - part 3 | 31:55 |
Similar courses to Mastering REST APIs in Node.js: Zero To Hero
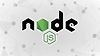
Mastering NodeJS with Interview Questions 2024udemy
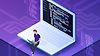
Build a Full-Stack Chrome Extension with NodeJS and MongoDBudemy
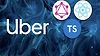
Uber Clone - Typescript, NodeJS, GraphQL, React, ApolloNomad Coders
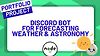
Create a Discord Bot with Node.jszerotomastery.io
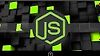
Node.js, Express & MongoDB Dev to Deploymentudemy
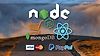
React Node FullStack - Ecommerce from Scratch to Deploymentudemy
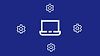
Microservices with NodeJS, React, Typescript and Kubernetesudemy
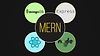