NestJS Masterclass - NodeJS Framework Backend Development
NestJS is a framework for Node.js designed for creating efficient, reliable, and scalable server-side applications. Its architecture is inspired by Angular, and thanks to this approach, NestJS has become popular, with more than 3 million downloads on NPM weekly.
When I was learning NestJS, I had to overcome a steep learning curve. I lacked a detailed and structured course that would simplify this process. That is why I created the "NestJS Masterclass": so that other developers wouldn't have to face such difficulties when learning NestJS.
Read more about the course
I guarantee that the "NestJS Masterclass" is the most detailed, comprehensive, and structured course on NestJS on the market! Nothing compares to this course, I promise you!
Practical Training in NestJS
Throughout the course, we create a server-side REST API for a blog, studying various NestJS features through real-world examples and delving into its internal mechanisms.
Thoughtful Structure and Curriculum
In creating the course curriculum, I invested a lot of time and effort to make learning NestJS as accessible as possible. The course covers all the key topics necessary for a deep understanding of the framework.
By the end of the course, you will confidently master NestJS and be ready to create complex server-side applications using this powerful framework.
Course Topics Overview:
- NestJS Modules: Understanding the principles of how NestJS modules work, their internal structure and schemas, including services and providers.
- Validation and Pipes: Learn how to use the class-validator package and Pipes for validating incoming data in a NestJS application.
- Dependency Injection: Dive into the DI (Dependency Injection) system, ensuring the modularity of the application, including addressing cyclic dependencies.
- Code Documentation: Explore NestJS capabilities for documenting APIs using Open API Specification and source code documentation with Compodoc.
- TypeORM and Relational Databases: Use PostgreSQL and TypeORM integration for creating a REST API application, learning the intricacies of working with databases.
- Database Relations: Master relationships between tables in SQL: one-to-one, one-to-many, and many-to-many, clarifying possible misconceptions on these topics.
- Environment Configuration: Learn how NestJS manages configurations for different environments.
- Exception Handling: Elegant management of exceptions to enhance user and developer experience.
- Database Transactions: Study TypeORM transactions for safely executing related operations.
- User Authentication with JWT: Build a JWT-based authentication system for application security.
- Guards and Decorators: Use Guards for request filtering and decorators for metadata and data transmission.
- Google Authentication: Use Google OAuth to simplify registration.
- File Upload: Implement a file upload mechanism to the server using Interceptors.
- Unit Testing and End-to-End Testing: Create unit tests to ensure high code quality.
- Mongoose and MongoDB: Work with NoSQL databases like MongoDB using Mongoose.
- Deployment to AWS: A complete guide to deploying a production application on AWS, including using CloudFront and S3 for media file storage.
Watch Online NestJS Masterclass - NodeJS Framework Backend Development
# | Title | Duration |
---|---|---|
1 | NestJS Masterclass Introduction | 03:16 |
2 | What Will We Build in This Course | 01:15 |
3 | What is NestJS | 09:13 |
4 | Setting Up The Development Enviroment | 02:30 |
5 | Installing NestJS CLI | 05:19 |
6 | Creating Our First NestJs Application | 09:22 |
7 | Boilerplate Code In NestJs | 01:23 |
8 | Best Learning Path For The Course | 01:55 |
9 | What are Modules? | 08:00 |
10 | How NestJS Bootstraps (main.ts) | 03:59 |
11 | Understanding the app Module | 06:03 |
12 | Creating a New users Module | 09:57 |
13 | What is a REST API | 16:28 |
14 | Setting Up Postman and httpYac | 06:49 |
15 | Creating Controllers | 08:27 |
16 | Working With Routing Decorators | 05:02 |
17 | Params, Query and Body | 13:38 |
18 | Additional Request Components | 04:53 |
19 | Providers in NestJS | 03:55 |
20 | What are Pipes? | 06:03 |
21 | Validation and Transformation Needs | 03:39 |
22 | Validating Params with Built-in Pipes | 05:46 |
23 | Validating Query Params | 05:04 |
24 | Introduction to DTO | 02:59 |
25 | Creating Our First DTO | 10:13 |
26 | Connecting DTO to Route Method | 07:42 |
27 | Global Pipes and Avoiding Malicious Request | 05:31 |
28 | Converting to an Instance of DTO | 03:02 |
29 | Using DTOs with Params | 05:53 |
30 | Using Mapped Types To Avoid Code Duplication | 07:06 |
31 | Introduction To Inversion Of Control | 10:22 |
32 | Dependency Injection In NestJS | 09:18 |
33 | Create a users Service | 04:48 |
34 | findall Users Method | 05:23 |
35 | findOneById Users Method | 01:27 |
36 | Practice: Create a Posts Module | 00:52 |
37 | Solution: Create a Posts Module | 05:30 |
38 | Types Of Dependencies | 02:07 |
39 | Create a GET Posts Route With DTO | 04:03 |
40 | Return Posts From Posts Service | 01:51 |
41 | Use Users Service Inside Posts Service | 04:28 |
42 | Practice: Create an Auth Module | 00:34 |
43 | Solution: Create an Auth Module | 02:06 |
44 | Circular Dependency | 08:57 |
45 | Documentation With NestJS | 05:40 |
46 | Open API Specification | 01:34 |
47 | Enabling Swagger in NestJS | 04:28 |
48 | Adding Configuration Methods to Swagger | 07:19 |
49 | Documenting GET users | 09:47 |
50 | Practice: POST Endpoint and DTO For Posts Controller | 03:05 |
51 | Solution: POST Endpoint and DTO For Posts Controller | 07:05 |
52 | Adding Validations To CreatePostDto | 07:19 |
53 | Working With Nested DTOs | 06:08 |
54 | Testing Validation | 07:38 |
55 | Using Swagger For Documenting CreatePostDto | 13:19 |
56 | Mapped Types Using Swagger | 11:06 |
57 | Getting Started With Compodoc | 04:45 |
58 | Compodoc Coverage And JSDocs | 05:48 |
59 | Working with Databases In NestJS | 04:37 |
60 | What is an ORM? | 06:54 |
61 | Installing PostgreSQL Locally | 05:26 |
62 | Adding psql to PATH | 03:07 |
63 | Connecting NestJS to PostgreSQL | 07:27 |
64 | Using Async Configuration | 03:30 |
65 | Theoretical Understanding of the Repository Pattern | 06:17 |
66 | Creating Our First Entity - user.entity | 06:55 |
67 | Expanding Entity Definition | 08:19 |
68 | Creating First Repository | 16:16 |
69 | Practice: Creating Post Entity | 00:34 |
70 | Solution: Creating Post Entity | 10:29 |
71 | Relationships in SQL Database | 04:56 |
72 | Creating the Tags Entity | 09:22 |
73 | Creating The Meta Options Entity | 04:14 |
74 | Updating DTO Files | 07:21 |
75 | Autoloading Entities | 03:51 |
76 | One to One Relationships | 01:48 |
77 | Uni-directional One To One Relationship | 04:53 |
78 | Creating MetaOptions Service | 09:13 |
79 | Creating Post With Relationships | 15:22 |
80 | Cascade Creation with Relationships | 05:31 |
81 | Querying with Eager Loading | 04:28 |
82 | Deleting Related Entities | 08:52 |
83 | Bi-Directional One To One Relationship | 02:12 |
84 | Creating A Bi-Directional Relationship | 07:11 |
85 | Cascade Delete With Bi-Directional Relationship | 06:12 |
86 | One To Many Relationships | 01:50 |
87 | Creating One To Many Relationship | 03:36 |
88 | Create Post With Author | 10:34 |
89 | Eager Loading Author | 02:51 |
90 | Many To Many Relationships | 01:51 |
91 | Practice: Service To Create Tags | 00:50 |
92 | Solution: TagsService | 05:14 |
93 | Testing Tags Service | 02:32 |
94 | Uni-Directional Many To Many Relationship | 09:21 |
95 | Querying Many To Many Relationship | 02:33 |
96 | Updating Post With New Tags | 16:12 |
97 | Deleting Post and Relationship | 02:44 |
98 | Bi-Directional Many To Many Relationship | 02:27 |
99 | Cascade Delete With Many To Many | 07:44 |
100 | Soft Delete Tags | 07:03 |
101 | Introduction To Environments | 02:48 |
102 | Installing Config Module | 03:41 |
103 | Using Config Service | 04:11 |
104 | Confirming NODE_ENV While Testing | 05:47 |
105 | Conditionally Loading Environments | 07:42 |
106 | Inject Database Details | 05:57 |
107 | Custom configuration Files | 11:54 |
108 | Config Files With Namespaces | 04:31 |
109 | Module Configuration and Partial Registration | 07:12 |
110 | Validating Environment Variables | 06:46 |
111 | Introduction To Exception Handling | 02:29 |
112 | Built-in Http Exceptions | 01:06 |
113 | Identifying Points of Failure | 03:52 |
114 | Exception Handling Model Constraints | 11:29 |
115 | Create User Exception Handling | 04:55 |
116 | Throw a Custom Exception | 05:59 |
117 | Practice: Exception Handling Post Update | 00:50 |
118 | Solution: Exception Handling Post Update | 07:01 |
119 | Understanding Transactions | 04:07 |
120 | TypeORM QueryRunner | 03:14 |
121 | Creating First Transaction | 13:27 |
122 | Why Create Post Is Not a Transaction | 02:29 |
123 | Creating Multiple Providers | 08:16 |
124 | Updating The DTO | 10:19 |
125 | Practice: Handle Exceptions For CreateManyUsers | 00:37 |
126 | Solution: Handle Exceptions For CreateManyUsers | 06:15 |
127 | Introduction To Pagination | 04:15 |
128 | Creating Pagination Query DTO | 13:16 |
129 | Adding Pagination To Query | 06:25 |
130 | Pagination Module And Interface | 09:11 |
131 | Using paginateQuery | 04:11 |
132 | Building Response Object | 10:38 |
133 | Complete Paginated Response | 09:36 |
134 | Introduction To Authentication | 03:39 |
135 | Hashing And Salting Passwords | 05:21 |
136 | Create Hashing Providers | 06:37 |
137 | Implementing Hashing Provider | 03:36 |
138 | User Signup | 12:51 |
139 | User SignIn Controller | 12:22 |
140 | Completing the signIn Method | 08:30 |
141 | Custom Response Code | 03:52 |
142 | Understanding JWTs | 07:26 |
143 | Adding JWT Configuration | 09:34 |
144 | Generating JWT | 05:55 |
145 | JWT Token Signatures | 03:59 |
146 | Introducing Guards | 02:11 |
147 | Creating AccessTokenGuard | 09:22 |
148 | Completing AccessTokenGuard Implementation | 05:43 |
149 | Testing the AccessTokenGuard | 06:46 |
150 | Applying AccessTokenGuard Globally | 05:25 |
151 | Practice: Validations For JWT env Variables | 00:31 |
152 | Solution: Validations For JWT env Variables | 01:37 |
153 | What Are Decorators | 05:23 |
154 | Our First Decorator | 09:43 |
155 | Authentication Guard Strategy | 03:16 |
156 | Create AuthenticationGuard | 11:54 |
157 | AuthenticationGuard Implemenation | 18:23 |
158 | Understanding User Payload | 04:53 |
159 | Create Active User Decorator | 09:34 |
160 | Practice: Refactor createPostDto | 01:11 |
161 | Solution: Refactor createPostDto | 10:02 |
162 | Introduction To Refresh Tokens | 03:57 |
163 | Refresh Token Configuration | 04:58 |
164 | Generate Tokens Provider | 06:10 |
165 | Generate Tokens Method | 05:26 |
166 | Generate Refresh Token On SignIn | 02:51 |
167 | Generate Access Token Using Refresh Token | 09:53 |
168 | Create Refresh Token Endpoint | 04:55 |
169 | Google Authentication Strategy | 03:36 |
170 | Create Google Project | 05:27 |
171 | Setting The Configuration | 08:40 |
172 | Initialize Google Auth Client | 05:35 |
173 | Implementation Strategy Google Authentication | 07:23 |
174 | Implement Authentication With Google Token | 05:31 |
175 | React App In Front-End | 10:39 |
176 | createGoogleUser Method | 06:12 |
177 | Complete Google Authentication | 03:24 |
178 | Testing Google Authentication | 01:19 |
179 | Introducing Interceptors and Serialization | 03:42 |
180 | Serializing User Entity | 04:38 |
181 | Global Data Interceptor | 06:06 |
182 | Adding API Version | 06:51 |
183 | Introduction To File Uploads | 03:16 |
184 | Setup S3 And Cloudfront | 09:46 |
185 | Uploads Module And Configuration | 10:25 |
186 | Create Upload Entity | 06:37 |
187 | Upload File Service And Controller | 08:04 |
188 | UploadToAwsProvider | 14:08 |
189 | Complete Uploads Service | 12:02 |
190 | Testing File Uploads | 06:02 |
191 | Introduction To Notification Emails | 01:49 |
192 | Setup A Mailtrap Account | 01:24 |
193 | Configuration For Notification Emails | 06:29 |
194 | Configure NestJS Mailer | 09:12 |
195 | Creating MailService | 06:22 |
196 | Testing Email Service | 07:10 |
197 | Enabling Inline CSS | 02:09 |
198 | Introduction To Testing | 03:25 |
199 | Test Settings | 01:53 |
200 | Understanding Unit Tests | 09:37 |
201 | Testing UsersService | 04:46 |
202 | Mocking Providers | 06:22 |
203 | Testing Service Method | 10:41 |
204 | New Spec File For CreateUserProvider | 06:15 |
205 | Mocking Repositories | 07:35 |
206 | Mocking Other Providers | 04:16 |
207 | Using Mock Repository To Test | 10:03 |
208 | Running Tests | 02:31 |
209 | What Is End To End Testing | 03:52 |
210 | Test Database And Configuration | 02:03 |
211 | Encapsulate App Creation Logic | 04:50 |
212 | Creating First E2E Test | 06:36 |
213 | Completing App Loading Lifecycle | 10:13 |
214 | Encapsulate Application Bootstrap | 04:10 |
215 | Introduction To Faker | 05:28 |
216 | Testing Validations | 03:23 |
217 | Completing All Test Cases | 05:34 |
218 | Introduction To MongoDB With Mongoose | 06:02 |
219 | Creating MongoDB Account | 08:10 |
220 | MongoDB Configuration | 06:28 |
221 | First Schema - User | 09:42 |
222 | Post Schema | 07:55 |
223 | Create Using Model | 06:28 |
224 | Mongoose Sub Documents | 03:10 |
225 | Single Sub Document | 07:54 |
226 | Practice: Tags Module | 01:08 |
227 | Solution: Tags Module | 05:02 |
228 | Practice: Tags Service + Controller | 01:07 |
229 | Solution: Tags Service + Controller | 06:00 |
230 | Array Of Sub Documents | 06:33 |
231 | Querying Sub Documents | 04:32 |
232 | Deployment Strategy | 04:35 |
233 | Creating An EC2 Instance | 05:12 |
234 | Installing Node, NPM and GIT | 07:47 |
235 | Installing PostgreSQL | 03:52 |
236 | Installing and Configuring NGINX | 07:58 |
237 | Git Clone And Install | 03:34 |
238 | Create The .env file | 02:50 |
239 | Understanding Migrations | 04:27 |
240 | Creating and Running Migrations | 09:29 |
241 | Testing Migration On EC2 | 07:22 |
242 | Running with PM2 | 05:42 |
Similar courses to NestJS Masterclass - NodeJS Framework Backend Development
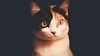
NestJS Authentication and Authorizationlearn.nestjs.com
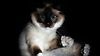
NestJS Advanced Conceptslearn.nestjs.com
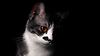
NestJS GraphQL - Code-first approachlearn.nestjs.com
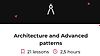
NestJS. Architecture and Advanced Patternslearn.nestjs.com
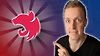
NestJS - Building Real Project API From ScratchudemyMonsterLessons
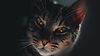
NestJS Fundamentalslearn.nestjs.com
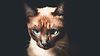
NestJS GraphQL - Schema-first approachlearn.nestjs.com
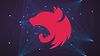
NestJS Microservices: Build & Deploy a Scaleable Backendudemy
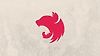
Master NestJS 9 - Node.js Framework 2023udemy
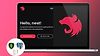