Master NestJS 9 - Node.js Framework 2023
NestJS is one of the most modern Node.js frameworks out there. This course will teach you everything you need to know to get you started ASAP! Don't get fooled by other longer courses - which basically contain more fluff and chit-chat than any meaningful lessons. I value your time most, and make sure that every minute of the course is filled with what you really need! We will cover basics, like routing and controllers.
Read more about the course
Then all the database topics using the TypeORM framework, like repository pattern, query building, and relations. You can use MySQL or Postgres with the course.
We'll be using Docker to run the database, as that's the most modern and up-to-date real-life way, you'll be running your databases at work. We'll take time to understand what Docker is and how it works.
I'll take time to explain how NestJS works - including tough topics like Providers and Dependency Injection. Believe me, though it's pretty tough to understand and crack at first, it's a powerful feature and needs to be understood well - you can count on me here!
There we get into authentication & authorization. Every app needs user accounts and related security, making sure users can only do what we want them to do. I'll explain how to do that with NestJS, with plenty of examples. I'll also take time to deep-dive into JWT Tokens - something other courses will skip!
You'll use Passport.js - a standard Node library to handle authentication.
Finally, I got you covered with Unit testing & End to End testing. You will learn all the ins and outs of how to do that properly with Nest, including a lot of examples and real-world scenarios you'll run into for sure!
Let's not forget we'll learn how to use Postman to work with our API.
You won't be building yet another boring example taken straight out of the documentation, instead, you'll build an API for an existing frontend project (made in Vue 3) - that's included in the course! Your job is to build an API for an Event attending application. You'll have a lot of fun doing it!
Some of the topics we'll cover, include:
Routing and controllers
Databases including TypeORM (Repository, Query Builder, Relations)
Using Docker in your local development workflow
Data validation and serialization
All about NestJS modules, Dependency Injection, and Providers
Configuring, logging, error handling
Authentication including Passport.js, Bcrypt
JSON Web Tokens (JWT) tokens explained, generation and usage
Authorization (making sure the user has privileges)
Using Postman (including collections, environments, automating Postman)
Unit testing
End to End testing (including connecting to a database)
The course comes with full source code included and is available on GitHub at all times. Including a separate branch for every lecture with code changes.
I've made sure everything is as clear and simple as possible, so there are a lot of diagrams and visual aids included (and available for download too!).
Join the community in the Q&A forums and on our Discord channel - talk to other students, share your progress, questions and insights!
I made extra effort to organize the topics in a way that would make you enjoy the process of learning. The course is short and to the point but covers plenty of topics in surprising detail!
Watch Online Master NestJS 9 - Node.js Framework 2023
# | Title | Duration |
---|---|---|
1 | About Node, Express, Nest and Backend Development | 05:46 |
2 | How to Get Help? | 02:00 |
3 | Getting the Source Code! | 01:57 |
4 | Don't Know TypeScript or JavaScript? | 02:10 |
5 | Software You Need (Node, Docker, Postman) | 01:43 |
6 | Using Postman | 01:19 |
7 | Using Visual Studio Code | 04:14 |
8 | Front-End Application (OPTIONAL!) | 04:48 |
9 | Introduction to NestJS - Section Intro | 00:40 |
10 | Installing and Using Nest CLI | 02:30 |
11 | NestJS Project Structure | 04:57 |
12 | Controllers, Routing, Requests - Section Intro | 02:29 |
13 | Controllers | 05:15 |
14 | Resource Controller | 06:35 |
15 | Route Parameters | 03:14 |
16 | Request Body | 02:07 |
17 | Responses and Status Codes | 05:21 |
18 | Request Payload - Data Transfer Objects | 03:06 |
19 | The Update Payload | 04:24 |
20 | A Working API Example | 12:22 |
21 | Database Basics - Section Introduction | 03:06 |
22 | Adding Docker to the Stack | 03:13 |
23 | Running the Database with Docker Compose | 06:57 |
24 | Introduction to ORMs | 02:18 |
25 | Connecting to the Database | 03:59 |
26 | The Entity (Primary Key & Columns) | 07:51 |
27 | Repository Pattern | 02:33 |
28 | TypeORM 3 UPGRADE GUIDE! | 03:06 |
29 | Repository in Practice | 06:35 |
30 | Repository Querying Criteria and Options | 08:41 |
31 | Data Validation - Section Introduction | 03:29 |
32 | Introduction to Pipes | 02:33 |
33 | Input Validation | 06:06 |
34 | Validation Groups and Options | 05:33 |
35 | Modules, Providers, Dependency Injection - Section Introduction | 07:25 |
36 | Introduction to Modules, Providers and Dependency Injection | 03:37 |
37 | Creating a Custom Module | 02:47 |
38 | Static Modules and Dynamic Modules | 04:32 |
39 | Standard & Custom Providers | 07:27 |
40 | Application Config and Environments | 05:21 |
41 | Custom Configuration Files and Options | 06:17 |
42 | Logging | 03:28 |
43 | Exception Filters | 02:17 |
44 | Understanding Relations | 01:42 |
45 | One To Many Relation | 05:41 |
46 | Loading Related Entities | 03:18 |
47 | Associating Related Entities | 06:11 |
48 | Many To Many Relation | 06:48 |
49 | Query Builder Introduction | 07:42 |
50 | Joins And Aggregation with Query Builder | 08:52 |
51 | Filtering Data Using Query Builder | 08:57 |
52 | Pagination Using Query Builder | 08:46 |
53 | Updating, Deleting, Modifying Relations using QB | 12:29 |
54 | One to One Relation | 04:15 |
55 | Introduction to Authentication | 03:39 |
56 | Local Passport Strategy | 07:33 |
57 | Logging In - Passport Strategy with a Nest Guard | 06:29 |
58 | JWT - JSON Web Tokens Introduction | 04:22 |
59 | JWT - Generating Token | 07:15 |
60 | JWT - Strategy & Guard - Authenticating with JWT Token | 06:00 |
61 | Hashing Passwords with Bcrypt | 02:27 |
62 | Custom CurrentUser Decorator | 03:51 |
63 | User Registration | 11:17 |
64 | Only Authenticated Users Can Create Events | 08:49 |
65 | Only The Owners Can Edit or Delete Events | 06:22 |
66 | Interceptors and Serialization | 01:51 |
67 | Serializing Data | 05:33 |
68 | Serializing Nested Objects | 03:38 |
69 | (Practical) Building Full Events API | 01:54 |
70 | Relations Between Entities | 05:44 |
71 | Getting Event Attendees | 06:34 |
72 | Getting Events Organized by User | 07:51 |
73 | Current User Event Attendance - the Business Logic | 07:37 |
74 | Current User Event Attendance - the Controller | 09:19 |
75 | Events Refactoring | 14:44 |
76 | Manual Testing with Postman | 06:48 |
77 | Introduction to Automated Testing | 02:00 |
78 | Introduction to Jest | 04:34 |
79 | Basic Unit Test and Code Coverage | 06:39 |
80 | Test Grouping, Spies, Mocks, Setup and Teardown | 20:59 |
81 | Nest Testing Utilities | 04:57 |
82 | Complex Unit Tests Part 1 | 06:15 |
83 | Complex Unit Tests Part 2 | 09:43 |
84 | Complex Unit Tests Part 3 | 06:07 |
85 | Introduction to E2E Testing | 07:33 |
86 | E2E Tests with Data Fixtures | 08:50 |
87 | Exploring E2E Tests | 09:03 |
88 | Dealing with Big E2E Test Suites | 09:23 |
89 | Upgrade Guide for Existing Students Nest 7 to Nest 9 | 06:50 |
90 | What is GraphQL? | 02:39 |
91 | How Does GraphQL API Work? | 04:49 |
92 | REST vs GraphQL APIs! | 03:49 |
93 | Setting Up and Configuring a GraphQL Module | 07:10 |
94 | First Resolver, Query and Type! | 14:06 |
95 | Nullable Fields & Nested Objects on Types | 08:30 |
96 | Query Arguments | 07:39 |
97 | Handling Errors Using Exception Filter | 07:49 |
98 | Mutations & Input Types (How to Make Changes Using GQL) | 10:04 |
99 | Input Types & Data Validation | 06:02 |
100 | Resolving Nested Objects & Lazy Database Relations | 08:35 |
101 | Field Resolvers | 07:05 |
102 | Working with Enums in GraphQL | 05:03 |
103 | Editing Data in GraphQL (Edit Input Types) | 08:23 |
104 | Not All Fields Should Change - Using Partial/Omit | 02:51 |
105 | Deleting in GraphQL | 08:56 |
106 | Course, Subject and Teacher Resolvers | 15:11 |
107 | Authorization in GraphQL | 09:21 |
108 | Authentication Refactoring Visualized | 04:55 |
109 | Extract Authentication Logic Into the AuthService (Reusability) | 05:35 |
110 | Authentication Resolver - Logging In using GraphQL | 08:31 |
111 | Getting Current User in GQL & Me Resolver | 08:32 |
112 | Refactoring User Registration Introduction | 01:40 |
113 | Moving of User Creation into the UserService | 08:31 |
114 | Creating an "Identical Password" Custom Validator | 10:46 |
115 | Checking if the User Already Exists Using a Validator | 14:50 |
116 | User Registration Mutation in GraphQL | 06:39 |
117 | Refactoring Pagination For GraphQL using Tests & Advanced TS Types | 12:55 |
118 | Paginated GraphQL Query Results | 05:37 |
Similar courses to Master NestJS 9 - Node.js Framework 2023
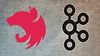
NestJS Microservices: Breaking a Monolith to Microservicesudemy
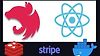
React, NextJS and NestJS: A Rapid Guide - Advancedudemy
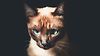
NestJS GraphQL - Schema-first approachlearn.nestjs.com
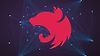
NestJS Microservices: Build & Deploy a Scaleable Backendudemy
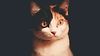
NestJS Authentication and Authorizationlearn.nestjs.com
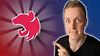
NestJS - Building Real Project API From ScratchudemyMonsterLessons
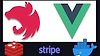
Vue 3, Nuxt.js and NestJS: A Rapid Guide - Advancedudemy
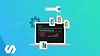
NestJS: The Complete Developer's GuideudemyStephen Grider
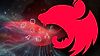
Mastering NestJS - 2024udemy
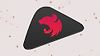