NestJS: The Complete Developer's Guide
Congratulations! You've found the complete guide on how to build enterprise-ready apps with NestJS. NestJS is a backend framework used to create scalable and reliable APIs. It is a "battery-included" framework; it includes tools to handle just about every possible use case, from data persistence, to validation, to config management, to testing, and much, much more.
More
This course will help you master Nest. By the time you complete this course, you will have the confidence to build any app you can imagine.
Throughout this course you will build a series of apps with growing complexity. We use as few libraries and tools as possible. Instead, you will write many custom systems to better understand how every piece of Nest works together. Each application you build includes discussion on data modeling and persistence. We will first save records in a simple file-based data store (built from scratch) and eventually work our way up to saving data in a production-grade Postgres instance.
Testing is a fundamental topic in Nest. A tremendous amount of functionality in Nest is dedicated to making sure your project is easy to test. This course follows Nest's testing recommendations, and you will write both integration and unit tests to ensure your project is working as expected. Although testing can sometimes be confusing and boring, I have put special care into making sure the tests we write are expressive, fast, and effective. You will be able to use this knowledge on your own projects, even those that don't use Nest!
Typescript is used throughout this course to make sure we are writing clean and correct code. Don't know Typescript? Not a problem! A free appendix is included at the end of the course to get you up to speed on Typescript. Once you're familiar with it, Typescript will help you catch errors and bugs in your code before you even run it. If you've never used Typescript before you are in for a treat :)
Everything in this course is designed to make your learning process as easy as possible.
At every step, I will teach you what Nest is doing internally, and help you understand how to twist and bend Nest to better suit your application's needs.
Every single video in the course has an attached ZIP file containing up-to-date code, just in case you ever get stuck.
Full-time teaching assistants are standing by to help answer your questions
Access private live chat server is included. Live help whenever you need it!
Here's a partial list of the topics included in this course:
Securely deploy your app to production
Write automated integration and unit tests to make sure your code is working
Build an authentication system from scratch to log users in
Allow users to perform certain actions with a permissions system
Store and retrieve data with complex queries using TypeORM
Understand how TypeORM handles data relationships
Write declarative code using property, method, and parameter decorators
Master the concept of dependency injection to write reusable code
Implement automatic validation of incoming requests
Format outgoing response data with a custom DTO system
Handle incoming requests and outgoing responses using Guards and Interceptors
Segment your code into reusable Nest Modules
Add structure to your database using migrations
I had a tough time learning NestJS. There are a tremendous number of outdated tutorials around it, the documentation is sometimes unclear, and Nest itself is just plain hard to understand. I made this course to save you time and money - a course to show you exactly what you need to know about every topic in Nest. You will find learning Nest to be a delightful experience and pick up a tremendous amount of knowledge along the way.
Watch Online NestJS: The Complete Developer's Guide
# | Title | Duration |
---|---|---|
1 | How to Get Help | 00:52 |
2 | Project Setup | 04:05 |
3 | TypeScript Configuration | 05:37 |
4 | Creating a Controller | 07:04 |
5 | Starting Up a Nest App | 05:51 |
6 | File Naming Conventions | 04:36 |
7 | Routing Decorators | 02:48 |
8 | App Setup | 07:43 |
9 | Using the Nest CLI to Generate Files | 06:11 |
10 | More on Generating Files | 04:12 |
11 | Adding Routing Logic | 04:05 |
12 | [Optional] Postman Setup | 04:48 |
13 | [Optional] VSCode REST Client Extension | 05:12 |
14 | Accessing Request Data with Decorators | 05:39 |
15 | Using Pipes for Validation | 03:03 |
16 | Adding Validation Rules | 07:38 |
17 | Behind the Scenes of Validation | 07:22 |
18 | How Type Info is Preserved | 06:15 |
19 | Services and Repositories | 06:12 |
20 | Implementing a Repository | 06:39 |
21 | Reading and Writing to a Storage File | 04:40 |
22 | Implementing a Service | 05:02 |
23 | Manual Testing of the Controller | 07:13 |
24 | Reporting Errors with Exceptions | 05:27 |
25 | Understanding Inversion of Control | 11:18 |
26 | Introduction to Dependency Injection | 09:26 |
27 | Refactoring to Use Dependency Injection | 07:37 |
28 | Few More Notes on DI | 05:50 |
29 | Project Overview | 03:07 |
30 | Generating a Few Files | 04:43 |
31 | Setting Up DI Between Modules | 05:58 |
32 | More on DI Between Modules | 04:40 |
33 | Consuming Multiple Modules | 05:20 |
34 | Modules Wrapup | 01:54 |
35 | App Overview | 02:19 |
36 | API Design | 04:09 |
37 | Module Design! | 03:03 |
38 | Generating Modules, Controllers, and Services | 02:28 |
39 | Persistent Data with Nest | 02:51 |
40 | Setting Up a Database Connection | 07:29 |
41 | Creating an Entity and Repository | 07:14 |
42 | Viewing a DB's Contents | 06:55 |
43 | Understanding TypeORM Decorators | 07:15 |
44 | One Quick Note on Repositories | 03:54 |
45 | A Few Extra Routes | 03:09 |
46 | Setting Up Body Validation | 07:16 |
47 | Manual Route Testing | 03:53 |
48 | Creating and Saving a User | 08:59 |
49 | Quick Breather and Review | 05:55 |
50 | More on Create vs Save | 07:37 |
51 | Querying for Data | 04:42 |
52 | Updating Data | 11:25 |
53 | Removing Users | 03:45 |
54 | Finding and Filtering Records | 05:51 |
55 | Removing Records | 02:52 |
56 | Updating Records | 06:00 |
57 | A Few Notes on Exceptions | 06:49 |
58 | Excluding Response Properties | 05:51 |
59 | Solution to Serialization | 04:38 |
60 | How to Build Interceptors | 12:14 |
61 | Serialization in the Interceptor | 08:21 |
62 | Customizing the Interceptor's DTO | 03:03 |
63 | Wrapping the Interceptor in a Decorator | 03:08 |
64 | Controller-Wide Serialization | 02:49 |
65 | A Bit of Type Safety Around Serialize | 03:31 |
66 | Authentication Overview | 08:22 |
67 | Reminder on Service Setup | 04:17 |
68 | Implementing Signup Functionality | 03:45 |
69 | [Optional] Understanding Password Hashing | 18:50 |
70 | Salting and Hashing the Password | 08:05 |
71 | Creating a User | 03:23 |
72 | Handling User Sign In | 08:29 |
73 | Setting up Sessions | 06:03 |
74 | Changing and Fetching Session Data | 05:46 |
75 | Signing in a User | 04:48 |
76 | Getting the Current User | 02:02 |
77 | Signing Out a User | 03:47 |
78 | Two Automation Tools | 01:58 |
79 | Custom Param Decorators | 05:35 |
80 | Why a Decorator and Interceptor | 06:13 |
81 | Communicating from Interceptor to Decorator | 07:08 |
82 | Connecting an Interceptor to Dependency Injection | 04:44 |
83 | Globally Scoped Interceptors | 03:53 |
84 | Preventing Access with Authentication Guards | 06:47 |
85 | Testing Overview | 04:43 |
86 | Testing Setup | 07:17 |
87 | Yes, Testing is Confusing | 06:50 |
88 | Getting TypeScript to Help With Mocks | 06:24 |
89 | Improving File Layout | 04:03 |
90 | Ensuring Password Gets Hashed | 05:57 |
91 | Changing Mock Implementations | 07:33 |
92 | Testing the Signin Flow | 02:03 |
93 | Checking Password Comparison | 08:38 |
94 | More Intelligent Mocks | 07:57 |
95 | Refactoring to Use Intelligent Mocks | 03:25 |
96 | Unit Testing a Controller | 06:49 |
97 | More Mock Implementations | 06:01 |
98 | Not Super Effective Tests | 08:11 |
99 | Testing the Signin Method | 06:29 |
100 | Getting Started with End to End Testing | 03:32 |
101 | Creating an End to End Test | 06:42 |
102 | App Setup Issues in Spec Files | 08:27 |
103 | Applying a Globally Scoped Pipe | 05:03 |
104 | Applying a Globally Scoped Middleware | 04:41 |
105 | Solving Failures Around Repeat Test Runs | 04:47 |
106 | Creating Separate Test and Dev Databases | 04:45 |
107 | Understanding Dotenv | 05:26 |
108 | Applying Dotenv for Config | 06:49 |
109 | Specifying the Runtime Environment | 04:45 |
110 | Solving a SQLite Error | 02:54 |
111 | It Works! | 04:36 |
112 | A Followup Test | 05:23 |
113 | Back to Reports | 01:01 |
114 | Adding Properties to Reports | 02:53 |
115 | A DTO for Report Creation | 03:51 |
116 | Receiving Report Creation Requests | 07:08 |
117 | Saving a Report with the Reports Service | 04:12 |
118 | Testing Report Creation | 04:08 |
119 | Building Associations | 03:36 |
120 | Types of Associations | 06:40 |
121 | The ManyToOne and OneToMany Decorators | 05:46 |
122 | More on Decorators | 08:29 |
123 | Setting up the Association | 06:39 |
124 | Formatting the Report Response | 03:39 |
125 | Transforming Properties with a DTO | 05:05 |
126 | Adding in Report Approval | 05:59 |
127 | Testing Report Approval | 05:49 |
128 | Authorization vs Authentication | 04:18 |
129 | Adding an Authorization Guard | 04:36 |
130 | The Guard Doesn't Work?! | 02:19 |
131 | Middlewares, Guards, and Interceptors | 03:50 |
132 | Assigning CurrentUser with a Middleware | 08:16 |
133 | Fixing a Type Definition Error | 02:34 |
134 | Validating Query String Values | 07:28 |
135 | Transforming Query String Data | 03:49 |
136 | How Will We Generate an Estimate | 03:32 |
137 | Creating a Query Builder | 05:15 |
138 | Writing a Query to Produce the Estimate | 08:33 |
139 | Testing the Estimate Logic | 03:46 |
140 | The Path to Production | 02:09 |
141 | Providing the Cookie Key | 03:36 |
142 | Understanding the Synchronize Flag | 03:44 |
143 | The Dangers of Synchronize | 02:23 |
144 | The Theory Behind Migrations | 03:21 |
145 | Headaches with Config Management | 04:49 |
146 | TypeORM and Nest Config is Great | 24:05 |
147 | Env-Specific Database Config | 06:40 |
148 | Installing the TypeORM CLI | 04:39 |
149 | Generating and Running Migrations | 07:08 |
150 | Running Migrations During E2E Tests | 02:50 |
151 | Production DB Config | 04:03 |
152 | Heroku Specific Project Config | 02:43 |
153 | Deploying the App - (Final Lecture) | 02:52 |
154 | How to Get Help | 01:05 |
155 | TypeScript Overview | 06:20 |
156 | Environment Setup | 08:00 |
157 | A First App | 04:44 |
158 | Executing TypeScript Code | 05:04 |
159 | One Quick Change | 03:36 |
160 | Catching Errors with TypeScript | 07:23 |
161 | Catching More Errors! | 05:16 |
162 | Course Overview | 03:37 |
163 | Types | 05:13 |
164 | More on Types | 05:54 |
165 | Examples of Types | 04:50 |
166 | Where Do We Use Types? | 00:50 |
167 | Type Annotations and Inference | 02:04 |
168 | Annotations with Variables | 04:54 |
169 | Object Literal Annotations | 06:54 |
170 | Annotations Around Functions | 05:57 |
171 | Understanding Inference | 03:52 |
172 | The Any Type | 07:48 |
173 | Fixing the "Any" Type | 01:50 |
174 | Delayed Initialization | 03:06 |
175 | When Inference Doesn't Work | 04:38 |
176 | More Annotations Around Functions | 04:57 |
177 | Inference Around Functions | 06:09 |
178 | Annotations for Anonymous Functions | 01:43 |
179 | Void and Never | 02:50 |
180 | Destructuring with Annotations | 03:36 |
181 | Annotations Around Objects | 07:06 |
182 | Arrays in TypeScript | 05:06 |
183 | Why Typed Arrays? | 04:31 |
184 | Multiple Types in Arrays | 02:58 |
185 | When to Use Typed Arrays | 00:55 |
186 | Tuples in TypeScript | 04:06 |
187 | Tuples in Action | 05:29 |
188 | Why Tuples? | 03:21 |
189 | Interfaces | 01:27 |
190 | Long Type Annotations | 04:43 |
191 | Fixing Annotations with Interfaces | 04:37 |
192 | Syntax Around Interfaces | 03:32 |
193 | Functions In Interfaces | 04:47 |
194 | Code Reuse with Interfaces | 04:16 |
195 | General Plan with Interfaces | 03:13 |
196 | Classes | 03:48 |
197 | Basic Inheritance | 03:04 |
198 | Class Method Modifiers | 06:42 |
199 | Fields in Classes | 06:19 |
200 | Fields with Inheritance | 04:19 |
201 | Where to Use Classes | 01:11 |
202 | App Overview | 02:46 |
203 | Bundling with Parcel | 04:56 |
204 | Project Structure | 03:20 |
205 | Generating Random Data | 05:30 |
206 | Type Definition Files | 05:18 |
207 | Using Type Definition Files | 06:21 |
208 | Export Statements in TypeScript | 05:07 |
209 | Defining a Company | 04:44 |
210 | Adding Google Maps Support | 07:39 |
211 | Google Maps Integration with TypeScript | 04:07 |
212 | Exploring Type Definition Files | 12:47 |
213 | Hiding Functionality | 06:29 |
214 | Why Use Private Modifiers? Here's Why | 08:26 |
215 | Adding Markers | 09:19 |
216 | Duplicate Code | 02:46 |
217 | One Possible Solution | 06:39 |
218 | Restricting Access with Interfaces | 05:36 |
219 | Implicit Type Checks | 03:27 |
220 | Showing Popup Windows | 06:24 |
221 | Updating Interface Definitions | 07:12 |
222 | Optional Implements Clauses | 06:07 |
223 | App Wrapup | 08:09 |
Similar courses to NestJS: The Complete Developer's Guide
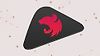
NestJS Zero to Hero - Modern TypeScript Back-end Development
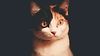
NestJS Authentication and Authorization
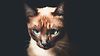
NestJS GraphQL - Schema-first approach
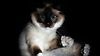
NestJS Advanced Concepts
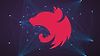
NestJS Microservices: Build & Deploy a Scaleable Backend
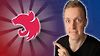
NestJS - Building Real Project API From Scratch
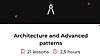
NestJS. Architecture and Advanced Patterns
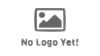
Master NestJS 9 - Node.js Framework 2023
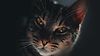
NestJS Fundamentals
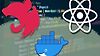