Build a Python REST API with the Django Rest Framework
10h 8m 56s
English
Paid
How does Apple Maps have Yelp listings? How does Tinder get Facebook user profiles? How does Amazon Alexa know the latest news? These questions get to the core of how powerful REST APIs can be: it allows for websites to communicate with each other without any human interaction.
Read more about the course
Building a REST API isn't just about connecting with third party services, it's also about:
- Adding Authentication, Registration, Databases, and more to your Mobile App, IOT Device, TV app, Car app, and more
- Connecting your own web apps with each other
- Creating micro-services
- Adding a backend for Angular, React, iOS Apps, Android apps, and more
- Simplifying the process of separating your backend from your frontend
- Making it easier to build additional services with new developers
This course will teach you step-by-step exactly how to build a REST API
We'll cover the concepts first, then we'll go into build a PURE DJANGO REST API, then we'll use the Django REST Framework to build a REST API.
Requirements:
- Some Python Experience
- Some Django Experience
Who this course is for:
- Anyone looking to connect their apps (mobile, web, iot, TV, etc) to a REST API
- Anyone looking to get better at building web applications
- Anyone looking to add Users, Security, Permissions, and a Database to anything that can connect to the internet
- Pythonistas and Beginner Python learners
- Djangonauts and Beginner Django learners
What you'll learn:
- What is an API?
- What is a REST API?
- How to build your own REST API
- A deeper understanding of Django
- A deep understanding of the Django Rest Framework
- A deep understanding of JWT and authentication with the Django Rest Framework
- Building an REST API with Python
Watch Online Build a Python REST API with the Django Rest Framework
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Welcome | 01:43 |
2 | Welcome | 00:26 |
3 | Behind the API | 09:40 |
4 | The Magic of REST APIs | 27:34 |
5 | Requirements | 01:29 |
6 | Blank Django Project | 04:51 |
7 | The Updates App and Model | 06:42 |
8 | A JSON Response | 08:53 |
9 | HttpResponse with Json Data | 02:30 |
10 | JSON CBV | 08:12 |
11 | Serialize Data | 08:31 |
12 | Managers & Methods to Produce Serialized Data | 08:23 |
13 | The Dot Values Method | 05:26 |
14 | Practical API Module | 08:07 |
15 | Practical API Module Part 2 | 10:14 |
16 | Use the API with Python Requests | 10:24 |
17 | Handling Errors | 12:16 |
18 | Http Status Codes | 07:00 |
19 | Validating Data with a Model Form | 14:42 |
20 | Update & Delete | 12:26 |
21 | Validate JSON | 07:36 |
22 | Update & Delete Part 2 | 15:06 |
23 | One Endpoint to Rule the Model | 19:59 |
24 | Introduction | 03:09 |
25 | Install Django Rest Framework | 04:21 |
26 | Status Model & App | 05:04 |
27 | Model Form for Validation | 05:53 |
28 | Creating a Serializer | 12:19 |
29 | Create & Update through Serializers | 11:12 |
30 | Validation & Fields | 08:43 |
31 | API Endpoints Overview | 05:34 |
32 | List & Search API View | 09:26 |
33 | Create API View | 02:34 |
34 | Detail API View | 03:14 |
35 | Update & Delete API Views | 03:15 |
36 | Mixins to Power Http Methods | 09:47 |
37 | One API Endpoint for CRUDL | 12:45 |
38 | One API Endpoint for CRUDL Part 2 | 16:33 |
39 | Uploading & Handling Images | 10:23 |
40 | 2 Views for CRUDL | 08:07 |
41 | Authentication & Permissions | 11:22 |
42 | Global Settings for Authentication & Permissions | 05:28 |
43 | Permission Tests with Python Requests | 07:06 |
44 | Implement JWT Authentication | 10:45 |
45 | JWT Authorization Header | 10:16 |
46 | Custom JWT Response Payload Handler | 06:10 |
47 | Custom Authentication View | 15:08 |
48 | Register API View | 09:14 |
49 | User Register Serializer | 10:23 |
50 | Serializer Method Field | 05:04 |
51 | Get Context Data | 05:54 |
52 | Custom Permissions | 06:56 |
53 | Is Owner or Read Only Permission | 12:51 |
54 | Nested Serializer Part 1 | 08:52 |
55 | Nested Serializer Part 2 | 07:33 |
56 | Nested Serializer Part 3 | 16:21 |
57 | Pagination to Manage Request Load | 08:38 |
58 | Search Filter & Ordering | 11:20 |
59 | Reverse URLs with DRF | 10:27 |
60 | Serializer related fields | 11:58 |
61 | Automated Testing | 07:48 |
62 | Testing User API | 19:05 |
63 | Testing API | 20:45 |
64 | Testing with a Temporary Image | 20:19 |
65 | Django Rest API Wrap Up | 03:34 |
66 | Thank you and next steps | 01:10 |
Similar courses to Build a Python REST API with the Django Rest Framework
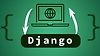
Python Django - The Practical GuideAcademind Pro
Category: Python, Django
Duration 22 hours 54 minutes 38 seconds
Course
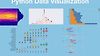
Python Data VisualizationTalkpython
Category: Python
Duration 4 hours 36 minutes 12 seconds
Course
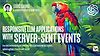
Responsive LLM Applications with Server-Sent Eventsfullstack.io
Category: TypeScript, React.js, Python
Duration 1 hour 18 minutes 18 seconds
Course
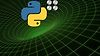
Python 3: Deep Dive (Part 4 - OOP)udemy
Category: Python
Duration 35 hours 15 minutes 32 seconds
Course
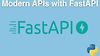
Modern APIs with FastAPI and Python CourseTalkpython
Category: Python
Duration 3 hours 53 minutes 18 seconds
Course
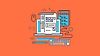
30 Days of Python | Unlock your Python Potentialudemy
Category: Python
Duration 9 hours 22 minutes 38 seconds
Course
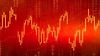
Time Series Analysis, Forecasting, and Machine Learningudemy
Category: Python, Data processing and analysis
Duration 22 hours 47 minutes 45 seconds
Course
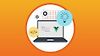
The Complete Guide to Django REST Framework and Vue JSudemy
Category: Python, Vue, Django
Duration 13 hours 40 minutes 40 seconds
Course
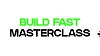
Build Fast MasterclassBuildFast Academy
Category: Python, Data processing and analysis
Duration 7 hours 22 minutes 11 seconds
Course
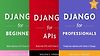
Django for Beginners/APIs/Professionalsleanpub
Category: Python, Django
Duration
Book