Python Mega Course: Learn Python in 60 Days, Build 20 Apps
In this intensive 60-day course, you will go from a complete beginner with no programming experience to a skilled Python developer capable of creating real applications and applying for entry-level positions in the IT industry. The curriculum, based on practical projects, will allow you to acquire the practical skills and experience that employers are looking for.
"Mega Course on Python" is designed to provide a practical, immersive learning experience. You will gain hands-on skills by creating 20 diverse applications, ranging from simple scripts to complex web applications. Each project is crafted to teach you essential programming concepts, best practices, and problem-solving methods necessary in a professional environment.
More
Below are the 20 applications that you will create during the course. The curriculum is designed for 60 days, but you can work through the material at your own pace:
- A "To-do" application (both a desktop GUI and a web application), covering all the basics of Python
- A website for showcasing projects, built with Python, for publishing your Python projects
- Task automation: creating PDF receipts with Python
- Extracting data from Excel and generating reports
- Automatic daily news email dispatch using Python
- Creating an API that provides historical weather data
- A weather forecast data dashboard
- Natural Language Processing (NLP) for e-books
- A webcam monitoring application with email alerts
- Web scraping sites
- A hotel booking application using Object-Oriented Programming (OOP) in Python
- Understanding programs written by others: studying the code of the Mario game
- A student management system - desktop application with GUI, SQLite, and PyQt
- A student management system - desktop application with GUI, MySQL, and PyQt
- An intelligent chatbot using ChatGPT and PyQt
- A job application web application with Flask
- A job application web application with Django
- A restaurant kitchen web application
- A movie recommendation system
- Creating and publishing a third-party Python package
These 20 applications have been carefully selected to cover all major areas of Python, including the basics of Python, task automation, data analysis and visualization, APIs, SQL databases, object-oriented programming, desktop GUIs, web development, data science and machine learning, as well as third-party package development using Python.
Additional benefits of the course:
Comprehensive learning:
This course covers both the theoretical foundations and practical aspects of programming in Python. You will gain a deep understanding of Python concepts and immediately apply them to create 20 practical applications. The course includes everything from basic syntax to advanced topics such as web development, data analysis, and machine learning.
Hands-on experience:
Learning by doing is the core principle of this course. You will start coding from day one, creating real-world applications alongside the instructor. With each project, you will strengthen your skills, experiment with different techniques, and build confidence in writing code independently.
Portfolio creation:
As you progress through the course, you will create an impressive portfolio of 20 Python applications, hosted on your GitHub account. This portfolio will serve as proof of your programming skills, showcasing your abilities to potential employers or clients.
Multimodal learning approach:
Our proven multimodal learning approach ensures complete mastery of Python concepts. You will benefit from video lectures, hands-on exercises, code reviews, quizzes, and independent projects. This comprehensive approach caters to different learning styles and ensures thorough understanding and retention of the material.
Career preparation:
Whether you want to change careers or advance to a new level in your current role, this course will prepare you for success. You will develop the skills necessary to confidently apply for junior developer positions. Additionally, you will learn important tools like Git and GitHub, which are essential for collaborating with other developers and showcasing your work to potential employers.
Watch Online Python Mega Course: Learn Python in 60 Days, Build 20 Apps
# | Title | Duration |
---|---|---|
1 | Welcome to Module 1 | 04:59 |
2 | The Four Types of Computer Programs | 03:26 |
3 | Today's Goals #print #variables #functions #lists | 01:08 |
4 | Installing Python and PyCharm | 11:19 |
5 | Create and Run Your First Python Program #print #strings | 07:55 |
6 | Getting User Input #input #variables | 10:20 |
7 | Storing User Input #lists | 07:45 |
8 | Coding Experiments | 07:56 |
9 | Bonus Example | 04:30 |
10 | Programming Tool/Concept of the Day: The Python Console | 06:21 |
11 | Recap of Yesterday | 01:34 |
12 | Getting User Input Repeatedly #while-loop | 06:35 |
13 | Storing User Input Repeatedly #methods | 09:36 |
14 | Coding Experiments | 06:04 |
15 | Bonus Example | 07:37 |
16 | Programming Tool/Concept of the Day: How to Find the Code You Need | 08:47 |
17 | Recap of Yesterday | 01:07 |
18 | Todo List View and Program Exit #match-case | 07:20 |
19 | Improving the Program Output #for-loops | 06:30 |
20 | Code Experiments | 06:46 |
21 | Bonus Example | 04:08 |
22 | Programming Tool/Concept of the Day: What Surprisingly is Python | 03:38 |
23 | Recap of Yesterday | 01:44 |
24 | Add an "Edit" Feature #type-conversion #list-indexing | 15:05 |
25 | Coding Experiments | 12:00 |
26 | Bonus Example #tuples | 13:13 |
27 | Programming Tool/Concep of the Day: Text Editors, Code Editors, IDE, PyCharm | 15:24 |
28 | Recap of Yesterday | 01:52 |
29 | Numbered Todos #enumerate | 04:45 |
30 | Improving the Program Output в–¶пёЏ #f-strings #formatting-strings | 03:39 |
31 | Add a "Complete Todo" Feature | 09:11 |
32 | Code Experiments | 10:27 |
33 | Bonus Example | 06:20 |
34 | Programming Tool/Concept of the Day: How to Ask Good Programming Questions | 09:50 |
35 | Recap of Yesterday | 02:41 |
36 | Storing Items in Text Files #text-files #read #write #writelines #readlines | 19:42 |
37 | Getting Todo Items from Text Files #read-text-files | 03:58 |
38 | How Data Types are Created #types | 03:05 |
39 | Code Experiments | 09:19 |
40 | Bonus Example | 12:39 |
41 | Programming Tool/Concept of the Day: Online Python Communities | 05:17 |
42 | Recap of Yesterday | 02:44 |
43 | Improving the Program Output #list-comprehensions | 10:17 |
44 | Code Experiments | 06:03 |
45 | Bonus Example | 03:22 |
46 | Programming Tool/Concept of the Day: Usages of Python in Real Life | 11:32 |
47 | Recap of Yesterday | 01:46 |
48 | Optimising the Code #with-context-manager | 04:51 |
49 | Editing and Completing Todo Items #with-context-manager | 11:20 |
50 | Code Experiments | 07:49 |
51 | Bonus Example | 07:56 |
52 | Programming Tool/Concept of the Day: Steps of Creating and Maintaining a Program | 03:55 |
53 | Recap of Yesterday | 02:52 |
54 | Improving the "Add" Feature #if-conditionals #slicing | 14:37 |
55 | Optimising the Program #elif #else | 11:03 |
56 | Code Experiments | 09:11 |
57 | Bonus Example | 24:00 |
58 | Programming Tool/Concept of the Day: High vs. Low Level Programming Languages | 04:58 |
59 | Recap of Yesterday | 03:02 |
60 | Fixing Two Bugs in the Program #bugs | 09:24 |
61 | Anticipating Program Errors #try-except #continue | 11:13 |
62 | Code Experiments | 06:09 |
63 | Bonus Example | 07:43 |
64 | Programming Tool/Concept of the Day: Cloud IDEs | 08:11 |
65 | Recap of Yesterday | 01:32 |
66 | Avoiding Repetitive Code #custom-functions | 16:52 |
67 | Code Experiments | 07:42 |
68 | Bonus Example | 09:52 |
69 | Programming Tool/Concept of the Day: Python vs. Other Languages | 03:28 |
70 | Recap of Yesterday | 01:12 |
71 | Optimising the Code #function-arguments | 09:40 |
72 | Optimising the Code Further #multiple-arguments | 14:14 |
73 | Code Experiments | 06:55 |
74 | Bonus Example | 11:54 |
75 | Programming Tool/Concept of the Day: The Learn-to-Program Curve | 03:26 |
76 | Recap of Yesterday | 01:19 |
77 | Optimising the Code #default-arguments | 12:55 |
78 | Documenting the Code #doc-strings | 07:41 |
79 | Code Experiments | 04:10 |
80 | Bonus Example | 10:07 |
81 | Programming Tool/Concept of the Day: Python Versions Explained | 07:56 |
82 | Recap of Yesterday | 01:26 |
83 | Organising the Code in Modules #modules #import | 13:04 |
84 | Anatomy of Python | 15:03 |
85 | Code Experiments | 09:59 |
86 | Bonus Examples | 08:21 |
87 | Programming Tool/Concept of the Day: What is Version Control and Git? #git | 10:22 |
88 | Recap of Yesterday | 01:57 |
89 | Add a "Date" Feature #standard-modules #time #constants #if-name-equal-main | 16:33 |
90 | Code Experiments | 22:56 |
91 | Bonus Example | 34:44 |
92 | Programming Tool/Concept of the Day: Using Git #git #commit #checkout #reset | 19:33 |
93 | Recap of Yesterday | 02:21 |
94 | Difference Between Frontend and Backend #frontend #backend | 04:34 |
95 | Create a Desktop Graphical User Interface (GUI) #desktop-gui #freesimplegui | 28:32 |
96 | Code Experiments | 05:22 |
97 | Bonus Example | 09:29 |
98 | Programming Tool of the Day: Using Github #github | 10:41 |
99 | Recap of Yesterday | 01:48 |
100 | Implementing an "Add Todo" Button #gui-buttons | 20:36 |
101 | Implementing an "Edit" Button #gui-buttons | 24:21 |
102 | Code Experiments | 07:51 |
103 | Bonus Example | 27:48 |
104 | Programming Tool/Concept of the Day: Clone a Remote Github Repository #git-clone | 13:13 |
105 | Recap of Yesterday | 01:09 |
106 | Implement "Complete" and "Exit" Buttons #gui-buttons | 07:59 |
107 | Final Touches | 15:53 |
108 | Code Experiments | 06:11 |
109 | Bonus Example | 21:37 |
110 | Programming Tool/Concept of the Day: Creating a Standalone Executable | 24:26 |
111 | Recap of Yesterday | 01:26 |
112 | Create a Web App #streamlit #title #subheader #checkbox | 16:22 |
113 | Adding New Todo Items on the Web App | 13:23 |
114 | Completing Todo Items on the Web App | 10:05 |
115 | Deploying the Web App to the Cloud | 13:42 |
116 | Code Experiments | 13:29 |
117 | Bonus Example | 12:36 |
118 | Programming Tool/Concept of the Day: Web App Deployment - Deploy to Heroku | 16:10 |
119 | Today | 01:35 |
120 | PyCharm Review | 04:32 |
121 | Objects, Variables, Functions | 13:09 |
122 | Methods, Lists, Tuples, Dictionaries | 17:51 |
123 | Code Blocks, f-strings | 06:49 |
124 | External Files, List Comprehensions | 07:26 |
125 | Errors, Comments, Modules, Libraries, Web and Desktop GUIs | 15:12 |
126 | Programming Tool/Concept of the Day: Using the Command Line | 15:19 |
127 | Today | 02:50 |
128 | Programming Tool/Concept of the Day: Prototyping with Figma | 22:52 |
129 | Designing the App | 01:43 |
130 | Setting up the PyCharm Project and Git | 07:17 |
131 | The App Data Source | 07:35 |
132 | Create a Multi-Column Webpage | 08:01 |
133 | Student Project: Add Content to the Website | 04:02 |
134 | Student Project: Solution | 03:58 |
135 | Today | 01:13 |
136 | Add Thumbnail Titles to the Webpage | 13:29 |
137 | Add Descriptions, Images, and Links to the Webpage | 08:51 |
138 | Student Project: Create a Company Website | 05:11 |
139 | Student Project: Solution | 06:33 |
140 | Programming Tool/Concept of the Day: Coding without an IDE | 11:23 |
141 | Today | 01:09 |
142 | Multipage Web Apps | 05:05 |
143 | Webforms | 09:09 |
144 | Send Email | 13:33 |
145 | Send Email Via the Web Form | 12:27 |
146 | Student Project: Contact Us Email Form | 03:59 |
147 | Student Project: Solution | 03:33 |
148 | Programming Tool/Concept of the Day: Secure Passwords in Environment Variables | 08:06 |
149 | Today | 03:47 |
150 | Create a Multipage PDF | 15:48 |
151 | From CSV to PDF #pandas | 15:34 |
152 | Add More Pages to PDF | 09:30 |
153 | Add Footer | 08:43 |
154 | Student Project: Lined PDF | 01:12 |
155 | Student Project: Solution | 04:10 |
156 | Programming Tool/Concept of the Day: PEP 8 Style Guide | 16:32 |
157 | Today | 03:38 |
158 | Setting up the Project | 06:50 |
159 | Load a Data from Excel to Python | 06:39 |
160 | Create a PDF for Each Excel File | 10:17 |
161 | Student Project: From Text Files to PDF | 02:17 |
162 | Student Project: Solution | 03:59 |
163 | Programming Tool/Concept of the Day: Zen of Python | 12:59 |
164 | Today | 00:25 |
165 | Add Date to PDF | 05:44 |
166 | Add Table from Excel to PDF | 16:49 |
167 | Add Table Header and Total Price | 07:47 |
168 | Student Project: Adding Multiline Text to PDF | 00:46 |
169 | Student Project: Solution | 02:11 |
170 | Programming Tool/Concept of the Day: Using the PyCharm Debugger | 24:19 |
171 | Today | 00:35 |
172 | Programming Tool/Concept of the Day: API Explained | 05:46 |
173 | URL Requests with Python | 08:46 |
174 | Getting Data from an API | 09:55 |
175 | Student Project: Email the API Data | 01:57 |
176 | Student Project: Solution | 07:38 |
177 | Today | 00:48 |
178 | Five Program Refinements | 09:34 |
179 | Download a File from the Web | 05:30 |
180 | Student Project: Astronomy Image of the Day | 01:39 |
181 | Student Project: Solution | 03:05 |
182 | Programming Tool/Concept of the Day: Using PythonAnywhere PaaS | 09:43 |
183 | Today | 03:04 |
184 | Programming Tool/Concept of the Day: HTML Tutorial | 13:00 |
185 | Build a Website with Flask | 12:53 |
186 | Build a REST API | 15:03 |
187 | Running Multiple Apps | 01:48 |
188 | Student Project: Build a Dictionary API (Part 1) | 03:46 |
189 | Student Project: Solution | 01:57 |
190 | Today | 02:08 |
191 | Programming Tool of the Day: Jupyter Lab Tutorial | 13:33 |
192 | Pandas In-Depth: Exploring 200-years of European Weather Data | 40:05 |
193 | API that Returns Weather Temperature Data | 15:01 |
194 | Student Project: Build a Thesaurus API (Part 2) | 01:19 |
195 | Student Project: Solution | 03:40 |
196 | Today | 00:43 |
197 | Programming Tool/Concept of the Day: Data Analysis and Visualization Theory | 05:24 |
198 | Show Data Table on the Webpage | 08:45 |
199 | URL Endpoints for All Data and Annual Data | 15:07 |
200 | Student Project: Analyze a Wine Tasting Dataset | 05:57 |
201 | Student Project: Solution | 04:26 |
202 | Today #streamlit #webapp | 03:16 |
203 | Coding the User Interface | 11:49 |
204 | Plotting Data Dynamically | 13:28 |
205 | Student Project: Build a Happiness Data App | 04:01 |
206 | Student Project: Solution | 04:52 |
207 | Today | 01:10 |
208 | Getting Raw Forecast Data | 13:34 |
209 | Filtering Forecast Data | 14:07 |
210 | Add Sky Conditions | 17:19 |
211 | Student Project: Fix the Weather Forecast App | 01:34 |
212 | Student Project: Solution | 02:36 |
213 | Today | 01:28 |
214 | Programming Tool/Concept of the Day: Regular Expressions (Regex) | 03:18 |
215 | Get Number of Chapters | 15:58 |
216 | Extract Certain Sentences | 11:19 |
217 | Most Common Words | 12:17 |
218 | Student Project: Regular Expressions | 03:19 |
219 | Student Project: Solution | 06:21 |
220 | Today | 01:02 |
221 | Most Used Non-Stop Words | 08:32 |
222 | Most Positive/Negative Chapters | 11:01 |
223 | Student Project: Visualise Your Mood Across Days | 02:30 |
224 | Student Project: Solution | 04:12 |
225 | Programming Tool/Concept of the Day: Applications of NLP | 05:26 |
226 | Today | 02:20 |
227 | Programming Tool/Concept of the Day: Images from a Computer Science Perspective | 11:02 |
228 | Capture Webcam Video | 13:47 |
229 | Program the Webcam to Detect Moving Objects | 27:29 |
230 | Trigger Action when the Webcam Detects an Object | 11:20 |
231 | Student Project: Add Live Timestamp to Webcam Video | 05:21 |
232 | Student Project: Solution | 02:21 |
233 | Today | 00:40 |
234 | Extract Images from Webcam Video | 08:40 |
235 | Send Email with Attachment | 14:11 |
236 | Send Email Attachment when Webcam Detects an Object | 05:35 |
237 | Threading in Python | 11:07 |
238 | Programming Tool/Concept of the Day: Applications of Image Processing | 02:56 |
239 | Today | 02:04 |
240 | Programming Tool/Concept of the Day: Web Scraping | 03:48 |
241 | Scraping a Webpage | 09:46 |
242 | Extracting Data from the Scraped Content | 10:37 |
243 | Storing the Extracted Data in Text Files | 12:04 |
244 | Send Email when New Event is Found on the Website | 04:55 |
245 | Running the Program Non-Stop | 02:26 |
246 | Student Project: Scrape Temperature Data | 02:49 |
247 | Student Project: Solution | 04:30 |
248 | Today | 01:08 |
249 | Programming Tool/Concept of the Day: SQL Databases | 17:24 |
250 | Select, Add, and Delete SQL Data | 12:25 |
251 | Storing the App Data in an SQL Database | 17:14 |
252 | Student Project: Store Temperature Data in SQL Database | 03:00 |
253 | Student Project: Solution | 05:05 |
254 | Today | 02:51 |
255 | Classes and Object Oriented Programming #classes #oop | 14:31 |
256 | Class init and self | 14:16 |
257 | Today | 01:16 |
258 | Programming Tool/Concept of the Day: Class vs Instance | 04:02 |
259 | Planning the App in OOP Style | 03:39 |
260 | Code the Classes | 03:43 |
261 | Create Instances | 09:24 |
262 | Implement the Methods of the Hotel Class | 11:39 |
263 | Implement the Methods of the Reservation Ticket Class | 08:35 |
264 | Student Project | 02:50 |
265 | Student Project: Solution | 04:20 |
266 | Today | 01:29 |
267 | Credit Card feature #extending #new-feature | 17:26 |
268 | Secure Credit Card feature #inheritance #new-feature | 13:40 |
269 | Overwriting Parent Methods | 03:51 |
270 | Student Project - Spa Hotel Class | 03:43 |
271 | Student Project: Solution | 02:53 |
272 | Today | 01:00 |
273 | Instance Variables Vs. Class Variables | 07:23 |
274 | Instance Methods Vs. Class Methods | 06:11 |
275 | Properties | 06:36 |
276 | Static Methods | 03:13 |
277 | Magic Methods | 12:41 |
278 | Abstract Classes and Abstract Methods | 07:32 |
279 | Today | 01:44 |
280 | пёЏ Programming Tool of the Day - Collaborating #github #fork #pull-request | 10:56 |
281 | Setting up the Project Locally | 05:22 |
282 | Inspecting the Project Structure | 09:32 |
283 | Inspecting the Code | 15:29 |
284 | Today | 02:53 |
285 | Project Requirements | 02:14 |
286 | Sketching a Prototype | 05:17 |
287 | Introduction to PyQt6 | 31:22 |
288 | Student Project - Speed Calculator GUI | 01:33 |
289 | Solution | 02:58 |
290 | Today | 00:34 |
291 | Add a Menu Bar | 08:42 |
292 | Creating a Table Structure | 04:20 |
293 | Populate Table with Data | 12:43 |
294 | Inserting New Records | 19:20 |
295 | Student Project - Implement the Search Functionality | 01:34 |
296 | Solution | 08:04 |
297 | Today | 00:52 |
298 | Add the Toolbar | 07:52 |
299 | Add the Status Bar | 11:44 |
300 | Create an Edit Dialog | 20:39 |
301 | Create a Delete Dialog | 12:28 |
302 | Create an About Dialog | 04:41 |
303 | Programming Skill of the Day - Refactoring | 09:44 |
304 | Today | 01:20 |
305 | MySQL vs SQLite | 06:00 |
306 | Installing MySQL on Windows | 08:07 |
307 | Installing MySQL on Mac | 09:13 |
308 | Installing MySQL on Linux | 04:18 |
309 | пёЏ Programming Skill of the Day - MySQL Command Line Tutorial | 11:57 |
310 | Implement MySQL in Python | 12:03 |
311 | Today #chatbot #chatgpt | 01:28 |
312 | Creating the Chatbot GUI | 11:48 |
313 | Create the GPT Chatbot | 08:51 |
314 | Connect the Chatbot to the GUI | 15:22 |
315 | Today | 02:32 |
316 | Creating the App Structure | 06:14 |
317 | Connecting the Python Backend to the HTML Part | 05:24 |
318 | в–¶Building the Form | 16:32 |
319 | Bootstrap Style | 13:12 |
320 | Today | 00:50 |
321 | Getting the User Data | 14:31 |
322 | Creating a Database | 11:04 |
323 | Storing the User Data in the Database | 07:26 |
324 | Showing Submission Notification | 07:03 |
325 | Sending a Confirmation Email | 09:54 |
326 | Today | 01:35 |
327 | Setting up a Django Project and an App | 09:24 |
328 | Setting up Database Models | 12:58 |
329 | Creating a View and a Template | 10:35 |
330 | Today | 01:27 |
331 | Code the HTML of the Application Form | 08:10 |
332 | Creating a Form Model | 12:28 |
333 | Store the Data in the Database | 04:58 |
334 | Send an Email with Django | 09:09 |
335 | Today | 00:36 |
336 | Creating an Admin Interface | 07:09 |
337 | Customizing the Admin Interface | 06:31 |
338 | Creating a Base Template | 11:54 |
339 | Adding the Navigation Bar | 06:54 |
340 | Today | 03:37 |
341 | Creating the App QR Code | 03:45 |
342 | Setup a Django Project and App | 04:07 |
343 | Creating the Database Model | 19:01 |
344 | Today | 01:23 |
345 | Creating Class-Based Views | 22:40 |
346 | Context in Django | 05:54 |
347 | Today | 00:40 |
348 | Creating an Admin Interface and Adding Data | 09:38 |
349 | Jinja For Loops | 12:49 |
350 | Jinja If Conditionals | 08:53 |
351 | Adding Dynamic Links | 10:25 |
352 | Adding Bootstrap | 12:40 |
353 | Today | 01:39 |
354 | Three Types of Recommendation Systems | 04:04 |
355 | The Movies Dataset | 05:33 |
356 | Project Setup on Deepnote | 07:30 |
357 | Popularity-Based Recommendation Model | 19:50 |
358 | Today | 00:56 |
359 | Term Frequency and Inverse Document Frequency | 16:49 |
360 | Similarity Matrix | 07:25 |
361 | Extracting Most Similar Movies | 19:07 |
362 | Collaborative Filtering with Machine Learning | 04:04 |
363 | Training and Using the Model to Predict | 14:17 |
364 | Today | 01:13 |
365 | Description of the Package | 02:17 |
366 | Preparing the Code of the Package | 11:11 |
367 | Testing the Package | 12:15 |
368 | Uploading the Package to PyPi | 13:01 |
Similar courses to Python Mega Course: Learn Python in 60 Days, Build 20 Apps
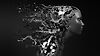
Deep Learning A-Z™: Hands-On Artificial Neural Networksudemy
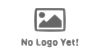
Build an LLM-powered Q&A App using LangChain, OpenAI and Pythonzerotomastery.io
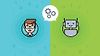
Create Telegram Bot with Pythonudemy
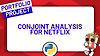
Conduct a Choice-Based Conjoint Analysis for Netflix with Pythonzerotomastery.io
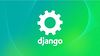
The Ultimate Django Series: Part 2codewithmosh (Mosh Hamedani)
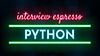
Python Interview Espressointerviewespresso (Aaron Jack)
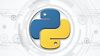
Complete Python Developer in 2023: Zero to Masteryudemyzerotomastery.io
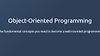
Object-Oriented Programmingprogrammingexpert.io
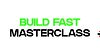
Build Fast MasterclassBuildFast Academy
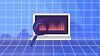