Automated Software Testing with Python
Testing automation doesn't have to be painful. Software testing is an essential skill for any developer, and I'm here to help you truly understand all types of test automation with Python. I'm Jose, a software engineer and founder of Teclado. The focus of this course is on testing for the web—we'll be working with REST APIs and web applications, and technologies such as unittest, Postman, and Selenium WebDriver.
More
Fear not though, after going through this course, you'll be able to take your new testing knowledge and apply it to any project, even non-web projects!
What will you work with?
This course is jam-packed with all the latest technologies for you to use professionally and in personal projects:
The unittest library, Python's standard automated software testing library;
Mocking and patching, two essential tools to reduce dependencies when testing;
unit, integration, system, and acceptance testing—all types of testing to have you fully covered;
Postman for easy collaboration and testing while developing;
Selenium WebDriver for automated browser tests;
Git and Travis for continuous integration of your project.
Fundamental Software Testing Skills
We will cover every fundamental software testing skill that you need to know in order to get a job testing or to apply these skills in your existing projects.
From things like mocking and patching using the unittest library, which reduce dependencies and turn complex tests to simple ones; to looking at all types of testing: simple unit tests to large system tests and even customer acceptance tests.
The Testing Pyramid
The Testing Pyramid says you should have a lot of unit tests, slightly fewer integration tests, even fewer system tests, and as few acceptance tests as possible.
Throughout the course we work on this concept, making sure that we have full coverage of every component of our system with unit tests. Then we test the dependencies using integration tests. Finally, we cover the entire system and its assumptions using system tests. Of course, we'll also look at what acceptance testing is, how we come up with acceptance tests, and some of the best ways to write acceptance tests for a web application using Behavior-Driven Development and Selenium WebDriver.
Automated Browser Testing with Selenium WebDriver
Selenium WebDriver is extremely powerful, particularly when coupled with the efficient and tried-and-tested approach recommended in this course. We'll design our acceptance tests professionally—just the way you'd do at a software testing job. We'll use page models, locators, and step definitions to structure the automated tests in a reusable way. The customers will be able to come up with acceptance tests that you can easily translate to code.
We'll also learn about implicit and explicit waits with Selenium WebDriver and Python, a key concept to speed up the runtime of your acceptance tests.
Continuous Integration
We also cover how you can take automated testing much further in your projects.
By implementing a Continuous Integration pipeline that runs your tests whenever you make any changes, you'll have much higher quality in your projects and not let any pesky bugs pass you by. We'll look at putting our projects in GitHub and liking the CI pipeline with them.
Watch Online Automated Software Testing with Python
# | Title | Duration |
---|---|---|
1 | Introduction to this section | 01:02 |
2 | Variables in Python | 08:27 |
3 | Solution to coding exercise: Variables | 02:01 |
4 | String formatting in Python | 06:27 |
5 | Getting user input | 05:17 |
6 | Writing our first Python app | 03:20 |
7 | Lists, tuples, and sets | 06:32 |
8 | Advanced set operations | 04:40 |
9 | Solution to coding exercise: lists, tuples, sets | 04:41 |
10 | Booleans in Python | 05:01 |
11 | If statements | 08:18 |
12 | The 'in' keyword in Python | 02:03 |
13 | If statements with the 'in' keyword | 08:19 |
14 | Loops in Python | 11:08 |
15 | Solution to coding exercise: flow control | 03:09 |
16 | List comprehensions in Python | 07:25 |
17 | Dictionaries | 08:32 |
18 | Destructuring variables | 08:29 |
19 | Functions in Python | 10:42 |
20 | Function arguments and parameters | 07:41 |
21 | Default parameter values | 03:55 |
22 | Functions returning values | 07:20 |
23 | Solution to coding exercise: Functions | 02:31 |
24 | Lambda functions in Python | 07:53 |
25 | Dictionary comprehensions | 04:02 |
26 | Solution to coding exercise: dictionaries | 06:17 |
27 | Unpacking arguments | 10:25 |
28 | Unpacking keyword arguments | 08:45 |
29 | Object-Oriented Programming in Python | 15:53 |
30 | Magic methods: __str__ and __repr__ | 06:26 |
31 | Solution to coding exercise: classes and objects | 05:05 |
32 | @classmethod and @staticmethod | 14:04 |
33 | Solution to coding exercise: @classmethod and @staticmethod | 05:55 |
34 | Class inheritance | 08:33 |
35 | Class composition | 06:09 |
36 | Type hinting in Python 3.5+ | 05:09 |
37 | Imports in Python | 09:34 |
38 | Relative imports in Python | 08:54 |
39 | Errors in Python | 12:48 |
40 | Custom error classes | 05:05 |
41 | First-class functions | 07:53 |
42 | Simple decorators in Python | 07:13 |
43 | The 'at' syntax for decorators | 03:34 |
44 | Decorating functions with parameters | 02:25 |
45 | Decorators with parameters | 04:51 |
46 | Mutability in Python | 06:04 |
47 | Mutable default parameters (and why they're a bad idea) | 04:28 |
48 | Conclusion of this section | 00:38 |
49 | Introduction to this section | 00:25 |
50 | Setting up our project | 06:43 |
51 | Writing our first test | 11:11 |
52 | Testing dictionary equivalence | 05:26 |
53 | Writing blog tests and PyCharm run configurations | 06:44 |
54 | The __repr__ method, and intro to TDD | 08:50 |
55 | Integration tests and finishing the blog | 11:58 |
56 | Mocking, patching, and system tests | 16:38 |
57 | Patching the input method and returning values | 07:30 |
58 | Taking our patching further | 16:04 |
59 | The last few patches! | 07:08 |
60 | The TestCase setUp method | 04:37 |
61 | Conclusion of this section | 00:28 |
62 | Introduction to this section | 00:27 |
63 | Setting our project up | 04:39 |
64 | Creating our Flask app | 06:51 |
65 | Our first System test | 09:57 |
66 | Refactoring our System Tests | 06:33 |
67 | Conclusion of this section | 00:34 |
68 | Introduction to this section | 00:36 |
69 | A look at a REST API with Flask | 18:19 |
70 | Unit testing a REST API | 08:16 |
71 | Setting up our generic BaseTest | 09:37 |
72 | Integration testing a REST API | 06:48 |
73 | Conclusion of this section | 00:26 |
74 | Introduction to this section | 00:21 |
75 | Setting up our project | 08:46 |
76 | Testing foreign key constraints with Python | 05:26 |
77 | Unit testing models and SQLAlchemy mappers | 11:04 |
78 | Finishing our Store tests | 11:39 |
79 | Conclusion of this section | 00:25 |
80 | Introduction to this section | 00:29 |
81 | Setting project up and creating User model | 03:59 |
82 | Allowing users to log in | 04:11 |
83 | Writing our User tests | 04:15 |
84 | The setUpClass method in the BaseTest | 05:00 |
85 | Testing user registration | 06:42 |
86 | Finalising user System tests | 07:32 |
87 | Writing Store System tests | 13:36 |
88 | Writing our Item System tests and testing authentication | 22:36 |
89 | Conclusion of this section | 00:27 |
90 | Introduction to this section | 00:41 |
91 | Introduction to Postman | 09:02 |
92 | Our first Posman tests | 05:51 |
93 | Setting and clearing environment variables in Postman | 07:05 |
94 | Running a test folder in Postman | 07:54 |
95 | Advanced PyCharm run configurations | 06:09 |
96 | Installing Node and Newman | 04:44 |
97 | Multirun in PyCharm—Running app and tests together | 03:02 |
98 | Conclusion of this section | 00:37 |
99 | Introduction to this section | 00:39 |
100 | Installing Git | 04:13 |
101 | What is a Git repository? | 05:28 |
102 | A local Git workflow | 04:32 |
103 | GitHub and remote repositories | 05:18 |
104 | Adding our project to GitHub | 04:52 |
105 | What is Travis CI? | 02:30 |
106 | Adding our repository to Travis | 01:39 |
107 | The Travis config file and running tests | 10:34 |
108 | Adding our test badge to the Readme | 02:48 |
109 | Conclusion of this section | 00:39 |
110 | Introduction to this section | 00:41 |
111 | What is acceptance testing? | 05:09 |
112 | Introduction to our project | 03:11 |
113 | Our first acceptance test step | 10:47 |
114 | Getting the Chrome webdriver | 03:39 |
115 | Verifying everything works | 05:45 |
116 | Finishing our first test | 14:52 |
117 | Re-using steps with the regular expression matcher | 04:01 |
118 | Our first content test | 09:19 |
119 | Page locators and models | 18:37 |
120 | The blog page | 07:31 |
121 | Using pages in navigation | 06:46 |
122 | Don't over-generalise tests! | 02:26 |
123 | Waits and timeouts with Selenium | 07:34 |
124 | Debugging acceptance tests in PyCharm | 03:33 |
125 | Our final complex scenario | 02:47 |
126 | Filling in forms with Selenium | 13:02 |
127 | Conclusion of this section | 00:42 |
Similar courses to Automated Software Testing with Python
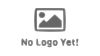
PHP for Beginners - Become a PHP Master udemy
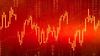
Time Series Analysis, Forecasting, and Machine Learningudemy
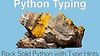
Rock Solid Python with Python Typing CourseTalkpython
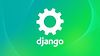
The Ultimate Django Series: Part 1codewithmosh (Mosh Hamedani)
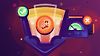
Test with Jestvueschool.io
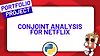
Conduct a Choice-Based Conjoint Analysis for Netflix with Pythonzerotomastery.io
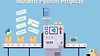
Modern Python ProjectsTalkpython
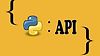
API Testing with Python 3 & PyTest, Backend Automation 2022udemy
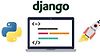
Python and Django Full Stack Web Developer Bootcampudemy
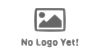