3D Computer Graphics Programming
This course is a complete immersion into the fundamentals of computer graphics! You'll learn how a software 3D engine works under the hood, and use the C programming language to write a complete software rasterizer from scratch; including textures, camera, clipping, and loading complex OBJ files. Pixel per pixel, triangle per triangle, mesh per mesh.
More
We'll review all the beautiful math that makes 3D computer graphics possible as we tackle all concepts from first principles. We'll also write a comprehensive software renderer that can display complex 3D objects on the screen without the help of any graphics API. No GPU, no OpenGL, no DirectX! Just a C compiler and a little bit of linear algebra is all we need to create a final project that is nothing short of amazing!
The tools you'll need
We'll simply use the command-line, a code editor, and a C compiler. All these tools are multi-platform, so you'll be able to code along on either Windows, macOS, or Linux!
Also, make sure you have pen and paper ready for the lectures. This course will probably be a little bit different than other programming courses you took before. We will take our sweet time and make sure we understand every formula we find along the way!
Is this course for you?
3d programming tutorial This is a self-contained course with no prerequisites. However, you will probably get the most out of it if you already know the basics of coding (if-else, loops, functions). If you never programmed in C before, don't worry! Many successful students come from different languages like Java, Python, JavaScript, Swift, and others. We'll learn to work with the C language together.
Watch Online 3D Computer Graphics Programming
# | Title | Duration |
---|---|---|
1 | 1.01 Introduction and Learning Outcomes | 12:24 |
2 | 1.02 How to Take this Course | 02:59 |
3 | 1.03 Words of Encouragement | 02:37 |
4 | 2.01 Project Dependencies | 10:00 |
5 | 2.02 A Quick Note for Windows Users | 01:56 |
6 | 2.03 Project Folder Structure | 02:02 |
7 | 2.04 The Compilation Flow | 06:19 |
8 | 2.05 Working with Makefiles | 08:21 |
9 | 2.06 Configuring Visual Studio on Windows | 14:46 |
10 | 3.01 Creating an SDL Window | 19:16 |
11 | 3.02 Rendering an SDL Window | 19:54 |
12 | 3.03 Declaring a Color Buffer | 20:51 |
13 | 3.04 Allocating Memory and Freeing Resources | 07:42 |
14 | 3.08 SDL Texture | 17:14 |
15 | 3.09 Fullscreen Window | 08:31 |
16 | 3.10 Exercise. Drawing a Background Grid | 02:59 |
17 | 3.11 Drawing a Background Grid | 05:43 |
18 | 3.13 Exercise. Drawing Rectangles | 03:09 |
19 | 3.14 Creating a Function to Draw Rectangles | 06:00 |
20 | 4.01 Defining Header Files | 11:20 |
21 | 4.02 Coding New Header Files | 13:01 |
22 | 5.01 The Draw Pixel Function | 07:48 |
23 | 5.02 Vectors | 14:50 |
24 | 5.03 Declaring a Vector Type | 03:40 |
25 | 5.04 Review of C Structs | 06:24 |
26 | 5.05 Array of Points | 09:31 |
27 | 6.01 Orthographic Projection | 16:13 |
28 | 6.03 Perspective Projection | 15:34 |
29 | 6.04 Implementing the Perspective Divide | 04:14 |
30 | 6.05 Coordinate System Handedness | 03:12 |
31 | 7.01 Vector Transformations | 10:06 |
32 | 7.02 Review of Sine Cosine and Tangent | 08:58 |
33 | 7.04 Rotating Vectors | 22:10 |
34 | 7.05 Vector Rotation Function | 11:18 |
35 | 7.06 Proof of Angle Sine Addition | 16:10 |
36 | 7.08 Proof of Angle Cosine Addition | 07:05 |
37 | 8.01 Fixing our Game Loop Time Step | 14:25 |
38 | 8.02 Using a Delay Function | 08:14 |
39 | 9.01 Triangles and Meshes | 12:36 |
40 | 9.02 Vertices and Triangle Faces | 24:40 |
41 | 9.03 Triangle Edges | 02:49 |
42 | 10.01 Line Equation | 15:19 |
43 | 10.02 DDA Line Drawing Algorithm | 22:39 |
44 | 10.03 Coding a Function to Draw Lines | 21:51 |
45 | 11.01 Dynamic Arrays | 20:55 |
46 | 11.03 Dynamic Mesh Vertices and Faces | 22:26 |
47 | 12.01 OBJ Files | 20:17 |
48 | 12.02 Exercise. Loading OBJ File Content | 08:31 |
49 | 12.03 Loading OBJ File Content | 26:23 |
50 | 13.01 Back-face Culling Motivation | 08:37 |
51 | 13.03 Vector Magnitude | 13:49 |
52 | 13.04 Vector Addition and Subtraction | 09:31 |
53 | 13.05 Vector Scalar Multiplication and Division | 04:24 |
54 | 13.06 Vector Cross Product | 08:59 |
55 | 13.07 Finding the Normal Vector | 08:34 |
56 | 13.08 Dot Product | 12:24 |
57 | 13.10 Back-face Culling Algorithm | 04:33 |
58 | 13.11 Back-face Culling Code | 26:26 |
59 | 13.13 Vector Normalization | 20:09 |
60 | 14.01 Triangle Fill | 06:38 |
61 | 14.02 Flat-Bottom & Flat-Top Technique | 07:20 |
62 | 14.03 Activity. Find Triangle Midpoint | 04:39 |
63 | 14.04 Solution to the Triangle Midpoint | 09:10 |
64 | 14.05 Coding the Triangle Midpoint Computation | 14:42 |
65 | 14.06 Flat-Bottom Triangle Algorithm | 09:49 |
66 | 14.07 Flat-Bottom Triangle Code | 14:22 |
67 | 14.08 Flat-Top Triangle Algorithm | 05:10 |
68 | 14.09 Flat-Top Triangle Code | 09:34 |
69 | 14.10 Avoiding Division by Zero | 07:25 |
70 | 14.12 Different Rendering Options Solution | 16:29 |
71 | 14.15 Colored Triangle Faces | 14:35 |
72 | 15.01 Painter's Algorithm | 11:35 |
73 | 15.03 Coding a Sorting Function | 14:07 |
74 | 16.01 Matrices Overview | 13:48 |
75 | 16.02 Matrix Operations | 11:50 |
76 | 16.03 Properties of Matrix Multiplication | 08:43 |
77 | 16.04 Examples of Matrix Multiplication | 07:19 |
78 | 16.06 2D Rotation Matrix | 07:09 |
79 | 17.01 3D Matrix Transformations | 09:15 |
80 | 17.02 3D Scale Matrix | 08:55 |
81 | 17.03 Matrix Typedef | 09:41 |
82 | 17.04 Scale Matrix Code | 18:35 |
83 | 17.05 3D Translation Matrix | 07:54 |
84 | 17.06 Translation Matrix Code | 06:09 |
85 | 17.07 3D Rotation Matrices | 09:40 |
86 | 17.08 Rotation Matrix Code | 09:23 |
87 | 17.10 The World Matrix | 18:07 |
88 | 17.11 Order of Transformations | 04:43 |
89 | 17.12 Translation is Not a Linear Transformation | 09:22 |
90 | 18.01 Defining a Projection Matrix | 18:46 |
91 | 18.02 Populating our Perspective Projection Matrix | 13:03 |
92 | 18.03 Coding the Perspective Projection Matrix | 21:27 |
93 | 18.04 Exercise. Projecting Negative Values | 03:10 |
94 | 18.05 Projecting Negative Values | 06:16 |
95 | 18.07 Row-major and Column-major Orders | 07:46 |
96 | 19.01 Flat Shading | 14:28 |
97 | 19.02 Coding Flat Shading & Light | 28:09 |
98 | 19.04 Smooth Shading Techniques | 07:47 |
99 | 19.06 Inverted Vertical Screen Values | 07:54 |
100 | 20.01 Texture Mapping | 14:33 |
101 | 20.02 Representing Textures in Memory | 12:41 |
102 | 20.03 Texture Typedef | 28:15 |
103 | 20.04 Textured Triangles | 05:52 |
104 | 20.05 Textured Flat-Bottom Triangle | 30:18 |
105 | 20.06 Textured Flat-Top Triangle | 09:14 |
106 | 20.07 Barycentric Coordinates | 18:16 |
107 | 20.08 Barycentric Weights (О±, ОІ, Оі) | 18:58 |
108 | 20.09 Function to Compute (О±, ОІ, Оі) | 13:13 |
109 | 20.10 Visualizing Textured Triangles | 25:43 |
110 | 21.01 Perspective Correct Interpolation | 28:55 |
111 | 21.02 PS1 Games and Affine Texture Mapping | 03:52 |
112 | 21.03 Perspective Correct Interpolation Code | 35:46 |
113 | 21.06 Inverted Cube UV Coordinates | 06:05 |
114 | 22.01 Decoding PNG Files | 10:08 |
115 | 22.03 Loading PNG File Content | 21:18 |
116 | 22.04 Freeing PNG Textures | 01:43 |
117 | 23.01 Loading OBJ Texture Attributes | 25:44 |
118 | 23.02 Preventing Texture Buffer Overflow | 10:54 |
119 | 23.04 Visualizing Textured OBJ Models | 07:42 |
120 | 24.01 Z-Buffer | 13:55 |
121 | 24.02 Z-Buffer Code | 22:35 |
122 | 24.03 Exercise. Z-Buffer for Filled Triangles | 05:00 |
123 | 24.04 Implementing a Z-Buffer for Filled Triangles | 10:37 |
124 | 24.05 A Discussion on Dynamic Memory Allocation | 18:01 |
125 | 25.01 Camera Space | 15:30 |
126 | 25.02 Look At Camera Model | 10:34 |
127 | 25.03 Look At Transformations | 17:40 |
128 | 25.04 The LookAt Function | 11:47 |
129 | 25.05 Coding the LookAt Function | 24:16 |
130 | 25.07 Variable Delta-time | 11:45 |
131 | 25.08 A Simple FPS Camera Movement | 06:07 |
132 | 25.09 Coding a Simple FPS Camera Movement | 35:56 |
133 | 26.01 Frustum Clipping | 09:03 |
134 | 26.02 Planes | 07:17 |
135 | 26.03 Exercise. Right Frustum Plane Point & Normal | 03:25 |
136 | 26.04 Defining Frustum Planes Points & Normals | 19:12 |
137 | 26.05 Initializing an Array of Frustum Planes | 12:00 |
138 | 26.06 Defining Points Inside and Outside Planes | 06:26 |
139 | 26.07 Intersection Between Line & Plane | 18:22 |
140 | 26.08 Clipping a Polygon Against a Plane | 14:28 |
141 | 26.09 Polygon Typedef | 23:51 |
142 | 26.10 A Function to Clip Polygon Against Planes | 24:27 |
143 | 26.11 Coding the Function to Clip Polygons Against Planes | 38:37 |
144 | 26.12 Converting Polygons Back Into Triangles | 06:57 |
145 | 26.13 Visualizing Clipped Triangles | 24:08 |
146 | 26.14 Horizontal & Vertical FOV Angles | 21:03 |
147 | 26.15 Clipping Texture UV Coordinates | 37:16 |
148 | 26.16 Clipping Space | 18:35 |
149 | 27.01 Working with Static Variables | 08:52 |
150 | 27.02 Refactoring SDL Globals | 30:28 |
151 | 27.03 Simulating Low-Resolution Displays | 12:30 |
152 | 27.04 Refactoring Light Globals | 07:16 |
153 | 27.05 Exercise. Camera Pitch Rotation | 04:40 |
154 | 27.06 Implementing the Camera Pitch Rotation | 14:41 |
155 | 28.01 Declaring Multiple Meshes | 17:30 |
156 | 28.02 Implementing Multiple Meshes | 28:55 |
157 | 28.03 Implementing Multiple Textures | 16:18 |
158 | 28.04 Finishing our Implementation | 20:06 |
159 | 28.05 Handedness & Orientation | 23:12 |
160 | 29.01 Dedicated Graphics Cards | 10:06 |
161 | 29.02 Modern Graphics APIs & Shaders | 14:17 |
162 | 29.03 A Parallel Rasterization Algorithm | 19:22 |
163 | 29.04 Determining Point Inside Triangle | 16:21 |
164 | 29.05 Top-Left Rasterization Rule | 17:38 |
165 | 29.06 Edge Function & Barycentric Weights | 14:04 |
166 | 29.07 Edge Function & Constant Increments | 19:23 |
167 | 29.08 Subpixel Rasterization | 23:15 |
168 | 30.03 Next Steps | 10:26 |
Similar courses to 3D Computer Graphics Programming
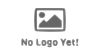
Communication Masterclass 2.0
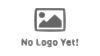
Macroeconomics Made Clear
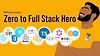
Zero to Full Stack Hero
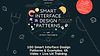
Smart Interface Design Patterns
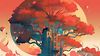
Algorithms and Data Structures
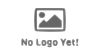
How to Survive in Space
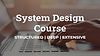
System Design Course
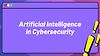