The Software Designer Mindset (COMPLETE)
"The Software Designer Mindset" is a course that teaches all aspects of software architecture and offers practical advice on creating scalable software products.
Did you know that the salary difference between a junior and a senior developer in the USA is about $65,000 per year? This shows how important it is to invest in yourself—the potential benefits are enormous!
To become a senior developer, you need to be an excellent programmer. This means you have to be able to solve complex problems that no one else can solve. You need to be able to structure problems logically in order to translate them into software.
Read more about the course
How to Bridge the Gap Between Junior and Senior Level?
The Internet offers a wealth of useful material, but it is also full of outdated information and harmful practices that are still being promoted.
You can try to climb this ladder on your own, but it will take a lot of time, and you risk learning the wrong things, which you will then have to unlearn. This can only keep you further from your goal of becoming a senior developer, depriving you of thousands of dollars a month that you could be earning.
But why complicate your life if there is another way? This way is conscious investment in yourself, learning from experienced colleagues, and following advanced software development practices. This path is available to you right now.
Presenting "Software Developer Mindset"
This online course is a modern look at software design. It includes everything I know about software design, structured in such a way that you can:
- Consistently make better design decisions
- Gain the technical knowledge necessary to advance in your career
- Design complex programs
- Significantly improve your existing code
- Create software that is easy to modify and scale
The goal of the course is to help you unlock your full potential.
Important Information
In the course, I use Python for all code examples. The advantage of Python is that it is flexible: it supports both object-oriented programming and functional programming.
This makes it an ideal language for learning software design. However, although the course is based on Python, the principles you master can be applied to any programming language and projects.
This course is for you if:
- You have basic programming skills in Python and want to take them to the next level
- You want to become a better software developer and are ready to invest in yourself and work on it
- You are striving for a senior developer position but want to do it efficiently
- You enjoy writing clean and elegant code that perfectly solves tasks
- You want to communicate with like-minded people from around the world and are not afraid to ask questions
This course is NOT for you if:
- You have no programming experience in Python at all. To take the course, you need to have basic Python knowledge. However, if you can follow my videos on YouTube, you can also take the course.
- You expect results without effort. You need to take seriously the goal of becoming a senior developer and be prepared to spend time and adhere to principles.
- You expect the course to give you ready-made solutions for specific tasks. This course focuses on the principles underlying the solutions and the mindset that helps you create your own solutions.
- You hate Python.
Watch Online The Software Designer Mindset (COMPLETE)
# | Title | Duration |
---|---|---|
1 | Course Introduction | 05:34 |
2 | Type Hints | 26:55 |
3 | Data Types | 19:57 |
4 | Data Structures | 24:50 |
5 | Classes and Dataclasses | 22:50 |
6 | The Mighty Function | 29:35 |
7 | Inheritance and Abstractions | 22:24 |
8 | Mixins | 11:00 |
9 | Final Thoughts | 03:47 |
10 | Favor Composition Over Inheritance | 22:05 |
11 | High Cohesion | 22:37 |
12 | Low Coupling | 22:22 |
13 | Start With The Data | 17:38 |
14 | Depend On Abstractions | 23:17 |
15 | Separate Creation From Use | 21:19 |
16 | Keep Things Simple | 18:12 |
17 | Project Scaffolding | 15:21 |
18 | Organizing Your Code | 25:42 |
19 | Error Handling | 27:41 |
20 | Course Wrap Up | 01:51 |
21 | Domain Modelling And Levels Of Software Design | 12:11 |
22 | History of Computing, Data Vs Processing | 40:42 |
23 | Mixins And Composition | 47:48 |
24 | How To Do Great Code Reviews | 01:16:07 |
25 | A Code Review Of Python Fire | 01:00:26 |
26 | May 2022 Q&A Session | 28:59 |
27 | VS Code Extensions | 04:04 |
28 | Vim Editor Plugin | 02:45 |
29 | AI Tools And Autoformatting | 02:27 |
30 | VS Code And Pylint Settings | 04:38 |
31 | Pyenv | 03:17 |
32 | Introduction To Domain Modeling | 06:57 |
33 | Domain Building Blocks | 11:44 |
34 | Domain Modeling | 13:02 |
35 | Implementing Effective Domain Models | 07:42 |
36 | Bonus: Collaboration and Communication Strategies | 09:05 |
37 | Why Understanding The Domain Is Important | 02:17 |
38 | Modelling The Domain | 05:16 |
39 | What Is An API? | 03:21 |
40 | Creating An API Server | 01:58 |
41 | Setting Up The Database Models | 06:09 |
42 | Relationships Between Models | 02:25 |
43 | Creating The Database System | 03:00 |
44 | Patching Everything Up | 01:51 |
45 | Why Scaffolding Is Useful | 01:45 |
46 | What Is The Layered Architecture? | 02:13 |
47 | Creating A Layered Architecture | 04:55 |
48 | Analysis | 03:07 |
49 | Developing A Basic Customer API | 02:35 |
50 | Creating A New Customer | 04:09 |
51 | Converting Database Query Results | 02:50 |
52 | Updating An Existing Customer | 03:07 |
53 | Adding Booking Operations | 04:55 |
54 | Adding The Booking Router | 03:08 |
55 | Analysis | 01:17 |
56 | Introduction | 01:45 |
57 | Creating An Abstract Data Interface | 04:12 |
58 | Database Implementation | 04:52 |
59 | Updating The Booking Operation | 04:13 |
60 | Updating The Booking Router | 02:10 |
61 | Writing Unit Tests | 07:12 |
62 | Final Thoughts | 02:46 |
63 | Introduction | 02:10 |
64 | Exploratory Questions | 04:58 |
65 | Organizational Questions | 03:12 |
66 | Architecture and Design Questions | 02:28 |
67 | Personal Development Questions | 01:48 |
68 | Avoid Type Abuse | 03:00 |
69 | Use Built-In Constructs | 01:51 |
70 | Use Clear Names | 01:44 |
71 | Avoid Flags | 02:33 |
72 | Don't Use Too Many Arguments | 05:30 |
73 | Use Shallow Nesting | 02:33 |
74 | Avoid Deeply Nested Conditionals | 03:05 |
75 | No Wildcard Imports | 02:31 |
76 | Write Symmetrical Code | 03:24 |
77 | Only Use Self If Needed | 02:35 |
78 | Keep Classes Small | 04:59 |
79 | Tell Don’t Ask | 03:11 |
80 | Use Meaningful Instance Variables | 05:11 |
81 | Avoid Redundancy | 03:20 |
82 | Don't Redefine Programming Concepts | 04:44 |
83 | BONUS #1: Protocol Segregation | 01:53 |
84 | BONUS #2: Function Composition | 01:59 |
Read Book The Software Designer Mindset (COMPLETE)
# | Title |
---|---|
1 | cheatsheet |
Similar courses to The Software Designer Mindset (COMPLETE)
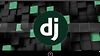
Python Django Dev To DeploymentudemyBrad Traversy
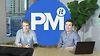
Become a Product Manager | Learn the Skills & Get the Jobudemy
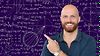
Mathematical Foundations of Machine Learningudemy
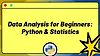
Data Analysis for Beginners: Python & Statisticszerotomastery.io
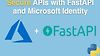
Secure APIs with FastAPI and the Microsoft Identity PlatformTalkpython
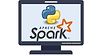
Spark and Python for Big Data with PySparkudemy
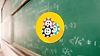
Essential Poker Math for No Limit Holdemudemy
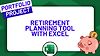
Create a Retirement Planning Tool with Excelzerotomastery.io
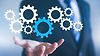
Master System Design and Design Patternudemy
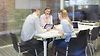