Go Bootcamp: Master Golang with 1000+ Exercises and Projects
Read more about the course
Get a Real In-Depth Understanding of Go and its Internal Mechanisms by:
Ultra-detailed, entertaining, intuitive, and easy to understand animations.
Learn by doing:
Write a log parser, file scanner, spam masker and more.
Solve 1000+ hands-on exercises.
Learn a lot of tips and tricks that you can't find easily anywhere else.
What's included?
Go OOP: Interfaces and Methods
Internals of Methods and Interfaces
Functions and Pointers: Program design, pass by value, and addressability.
Implicit interface satisfaction
Type assertion and Type Switch
Empty interface: []interface{} vs interface{}
Value, Pointer, and Nil Receivers
Promoted Methods
Famous Interfaces
Tips about when to use interfaces
fmt.Stringer, sort.Sort, json.Marshaler, json.Unmarshaler, and so on.
Composite Types: Arrays, Slices, Maps, and Structs
Internals of Slices and Maps
Backing array, slice header, capacity, and map header
JSON encoding and decoding, field tags, embedding
Make, copy, full Slice expressions and append mechanics
UTF-8 encoding and decoding
Go Type System Mechanics
Type inference, underlying, predeclared, and unnamed types.
Untyped constants and iota.
Blank Identifier, short declaration, redeclaration, scopes, naming conventions
I/O
Process Command-Line Arguments, printf, working with files, bufio.Scanner, ...
How to create your own Go packages
How to run multiple Go files, and how to use third-party packages
Go tools
Debugging Go code, go doc, and others.
...and more.
Why Go?
Go is one of the most desired, easy to learn, and the highest paying programming languages. There are 1+ million Go programmers around the world, and the number is increasing each day exponentially. It's been used by Google, Facebook, Twitter, Uber, Docker, Kubernetes, Heroku, and many others.
Go is Efficient like C, C++, and Java, and Easy to use like Python and Javascript. It's Open-Source, Simple, Powerful, Efficient, Cross-Platform (OS X, Windows, Linux, ...), Compiled, Garbage-Collected, and Concurrent.
Go is best for Command-line Tools, Web APIs, Distributed Network Applications like Microservices, Database Engines, Big-Data Processing Pipelines, and so on.
Go has been designed by one of the most influential people in the industry:
Unix: Ken Thompson
UTF-8, Plan 9: Rob Pike
Hotspot JVM (Java Virtual Machine): Robert Griesemer
- Access to a computer with an internet connection.
- Beginners who have never programmed before.
- Programmers switching languages to Go.
- Intermediate Go programmers who want to level up their skills!
- Intermediate Go programmers who want to learn the internals of slices, maps, interfaces, and so on.
What you'll learn:
- Watch the free videos to see how I teach Go programming in depth.
- Practice with 1000+ Exercises (with included solutions)
- Pass Interviews: Master Go Internals In-Depth
- Master Interfaces and Internals
- Master Slice Internals: Slice Header and Memory Allocations
- Master Map Internals: Map Header
- Encode and Decode JSON
- Create a log file parser, spam masker, retro led clock, console animations, dictionary programs and so on.
Watch Online Go Bootcamp: Master Golang with 1000+ Exercises and Projects
# | Title | Duration |
---|---|---|
1 | Introduction to Variables | 08:06 |
2 | Example: Path Separator | 05:32 |
3 | When to use a short declaration? | 06:12 |
4 | Let's convert a value! | 07:10 |
5 | Learn the basics of os.Args | 04:07 |
6 | Naming Things: Recommendations | 08:26 |
7 | What is a Raw String Literal? | 06:36 |
8 | How to get the length of a utf-8 string? | 04:37 |
9 | Example: Banger: Yell it back! | 04:43 |
10 | Constants and iota | 09:54 |
11 | Println vs Printf | 07:45 |
12 | What is an Escape Sequence? | 04:09 |
13 | How to print using Printf? | 07:48 |
14 | The verbs can be type-safe too! | 05:10 |
15 | If Statement | 05:13 |
16 | Else and Else If | 03:52 |
17 | Tiny Challenge: Validate a single user | 02:35 |
18 | Solution: Validate a single user | 07:51 |
19 | Tiny Challenge: Validate multiple users | 01:41 |
20 | Solution: Validate multiple users | 06:48 |
21 | What is a nil value? | 04:28 |
22 | What is an error value? | 06:31 |
23 | Error handling example | 03:44 |
24 | Challenge: Feet to Meter | 00:54 |
25 | Solution: Feet to Meter | 03:13 |
26 | What is a Simple Statement? | 04:17 |
27 | Scopes of simple statements | 06:14 |
28 | Famous Shadowing Gotcha | 05:13 |
29 | Learn the Switch Statement Basics | 09:26 |
30 | What is a default clause? | 03:27 |
31 | Use multiple values in case conditions | 02:26 |
32 | Use bool expressions in case conditions | 03:52 |
33 | How does the fallthrough statement work? | 06:53 |
34 | What is a short switch? | 03:01 |
35 | Tiny Challenge: Parts of a Day | 04:04 |
36 | Solution: Parts of a Day | 03:37 |
37 | If vs Switch: Which one to use? | 05:59 |
38 | There is only one loop statement in Go | 06:03 |
39 | How to break from a loop? | 05:24 |
40 | How to continue a loop? (+BONUS: Debugging) | 05:26 |
41 | Create a multiplication table | 05:41 |
42 | How to loop over a slice? | 05:23 |
43 | For Range: Learn the easy way! | 07:27 |
44 | Randomization and Go | 07:09 |
45 | Seed the randomizer with time | 04:12 |
46 | Write the Game Logic | 07:31 |
47 | Build the Word Finder Program | 07:36 |
48 | Labeled Break and Continue | 09:09 |
49 | Break from a Switch using Labels | 04:10 |
50 | Yes there is a "goto" statement in Go | 05:20 |
51 | Introduction and Roadmap | 06:10 |
52 | What is an array in Go? | 09:20 |
53 | Let's create an array | 04:58 |
54 | Learn the gotcha when using a for range on arrays | 07:34 |
55 | What is a composite literal? | 05:07 |
56 | Refactor the Hipster's Love Bookstore to array literals | 06:20 |
57 | Tiny Challenge #1: Moodly | 02:28 |
58 | Can you compare array values? | 07:49 |
59 | Can you assign an array value to another one? | 06:48 |
60 | How to use multi-dimensional arrays? | 08:46 |
61 | Tiny Challenge #2: Moodly | 04:15 |
62 | Learn the rarely known feature of Go: The Keyed Elements | 08:47 |
63 | Learn the relation between composite and unnamed types | 10:44 |
64 | Recap: Arrays | 04:10 |
65 | Challenge: Retro Led Clock | 06:43 |
66 | Let's print the digits | 07:27 |
67 | Let's print the clock | 06:51 |
68 | It's time to animate the clock! | 08:18 |
69 | Introduction and Roadmap | 03:10 |
70 | Learn the differences between slices and arrays | 06:01 |
71 | Can you compare a slice to another one? | 10:16 |
72 | Create a unique number generator | 07:15 |
73 | Append: Let's grow a slice! | 07:27 |
74 | Slicing: Let's cut that slice! | 09:34 |
75 | How to create pagination using slices? (+ Sprintf) | 04:48 |
76 | What is a Backing Array? | 10:46 |
77 | What's a slice header? | 05:29 |
78 | What does a slice header look like in the actual Go runtime code? | 07:29 |
79 | What is the capacity of a slice? | 05:10 |
80 | Extend a slice using its capacity | 06:04 |
81 | When does the append function create a new backing array? | 07:17 |
82 | Animate: When the backing array of a slice grows? | 06:18 |
83 | Full Slice Expressions: Limit the capacity of a slice | 06:04 |
84 | make(): Preallocate the backing array | 09:23 |
85 | copy(): Copy elements between slices | 07:40 |
86 | How to use multi-dimensional slices? | 09:19 |
87 | Fetch the Files | 05:28 |
88 | Write to a file | 04:29 |
89 | Optimize! | 04:17 |
90 | Challenge | 04:11 |
91 | Step #1: Create and Draw the Board | 06:27 |
92 | Step #2: Optimize by adding a Buffer | 07:03 |
93 | Step #3: Animate the Ball | 06:22 |
94 | Introduction and Roadmap | 01:23 |
95 | Let's learn the basics of bytes, runes and strings | 03:37 |
96 | Let's write a character-set program | 06:45 |
97 | Let's convert, index, and slice bytes, runes and strings | 11:28 |
98 | How can you decode a string? | 07:50 |
99 | String Header: Why strings are immutable? | 08:16 |
100 | Recap: Strings Revisited | 01:41 |
101 | Challenge | 03:05 |
102 | Detect the link patterns | 05:04 |
103 | Mask the links | 06:18 |
104 | Let's build a Unicode text wrapper | 06:02 |
105 | Create an English to Turkish dictionary | 08:29 |
106 | Populate the dictionary | 08:14 |
107 | Map Internals: How maps work behind the scenes? | 10:46 |
108 | Scan user input using bufio.Scanner | 07:08 |
109 | Use maps as sets | 09:23 |
110 | Create a Log Parser using maps and bufio.Scanner | 07:40 |
111 | What is a struct? | 04:34 |
112 | Let's create a struct! | 07:41 |
113 | When can you compare struct values? | 08:12 |
114 | Go OOP: Struct Embedding | 06:47 |
115 | Rewrite: Log Parser to Structs | 05:46 |
116 | Encode values to JSON | 09:12 |
117 | Decode values from JSON | 06:43 |
118 | Learn the function basics | 09:36 |
119 | Confine variables to a function | 10:07 |
120 | Rewrite: Log Parser using functions | 08:12 |
121 | Learn the Pass By Value Semantics | 07:59 |
122 | What is a pointer? | 10:56 |
123 | Learn the pointer mechanics | 10:16 |
124 | Learn how to work with pointers to composite types | 08:02 |
125 | Rewrite the Log Parser using Pointers | 06:38 |
126 | Pointers or Values? Be Consistent | 07:25 |
127 | Methods: Enhance types with additional behavior | 11:03 |
128 | Pointer Receivers: Change the received value | 10:29 |
129 | Non-Structs: Attach methods to almost any type | 07:55 |
130 | Interfaces: Be dynamic! | 11:38 |
131 | Type Assertion: Extract the dynamic value! | 11:49 |
132 | Empty Interface: Represent any type of value | 10:26 |
133 | Type Switch: Detect and extract multiple values | 06:28 |
134 | Promoted Methods: Let's make a little bit of refactoring | 09:33 |
135 | Don't interface everything! | 11:46 |
136 | Stringer: Grant a type the ability to represent itself as a string | 09:47 |
137 | Sorter: Let a type know how to sort itself | 09:51 |
138 | Marshalers: Customize JSON encoding and decoding of a type | 08:40 |
Similar courses to Go Bootcamp: Master Golang with 1000+ Exercises and Projects
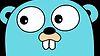
Introduction to industry REST microservices in Golang (Go)udemy
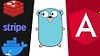
Angular and Golang: A Rapid Guide - Advancedudemy
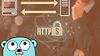
How to develop a productive HTTP client in Golang (Go)udemy
![Golang + Lambda Masterclass [EARLY-ACCESS]](https://cdn.courseflix.net/courses/100x56/golang-lambda-masterclass-early-access.jpg?d=1737536570995)
Golang + Lambda Masterclass [EARLY-ACCESS]Gourav Kumar
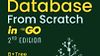
Build Your Own Database in Go From ScratchJames Smith
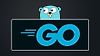
Go - The Complete GuideAcademind Pro
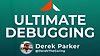