Typescript: The Complete Developer's Guide
Every other course online teaches you the basic syntax and features of Typescript, but only this course will show you how to apply Typescript on real projects, instructing you how to build large, successful projects through example.
Read more about the course
This is the best course online for mastering Typescript.
Typescript is a 'super-set' of Javascript. That means that if you already know Javascript, you are ready to take this course. Typescript adds in several important features to Javascript, including a type system. This type system is designed to help you catch errors during development, rather than when you are running your code. That means you'll be twice as productive by catching bugs earlier in development. But besides the type system, Typescript also provides several tools for structuring large codebases and writing truly reusable code.
Mastering Typescript by reading the documentation alone is challenging. Although you might know what a 'typed array' or an 'interface' is, the documentation (and other courses!) don't show you where to use this features, or how to use them effectively. The goal of this course is to help you understand why each feature of Typescript exists, and exactly where to use them.
Top companies are hungry for Typescript developers. Some of the largest web apps today are being built with Typescript. Employers are scrambling to find engineers who are fluent with this cutting edge system. Solid knowledge of Typescript will make you far more employable, by giving you a unique skill that few other engineers possess.
Planning on building your own apps? Using Typescript will help you structure your project correctly from day one, ensuring that you won't be crushed under technical debt at some critical stage of your company. In this course, you'll learn how to write reusable code, with a tremendous emphasis on leveraging classes and interfaces to make swappable 'widgets'. You will be able to reconfigure your apps on the fly to build wildly different features with only a minimum amount of effort
Learn from someone who has worked on one of the largest Typescript projects around. On professional projects of my own, I have developed plugins for an open-source, browser-based code editor called Theia. The Theia project is absolutely gargantuan in scale, encompassing hundreds of thousands of lines of code, all written in Typescript. This project works only thanks to the power of Typescript.
But don't just take my word for it - check out the reviews for this course! You'll see that other engineers, just like yourself, have had great success and acquired a new understanding of how to build scalable web applications.
There is just too much content in this course to summarize in a few short words, but here is a partial listing of the different skills you'll master:
Understand why Composition vs Inheritance is the most mis-understood topic in the Javascript community
Master the fundamental features of Typescript by working on real world projects
We'll build a custom front-end framework from scratch that you can use in place of React or Angular on your own personal projects
Comprehend the complex interplay between classes and interfaces, and why these two pieces of Typescript enable you to build incredibly reusable code
Dive deeper into Typescript with decorators, which we'll use to build a custom integration between Typescript and Express
Structure your React and Redux applications more confidently by understanding how to couple them with Typescript
Skip the documentation for third party libraries by fluently reading type definition files
Learn just how smart Typescript is by experiencing how deeply it analyzes your code for errors
Watch Online Typescript: The Complete Developer's Guide
# | Title | Duration |
---|---|---|
1 | How to Get Help | 01:05 |
2 | Typescript Overview | 06:20 |
3 | Environment Setup | 08:00 |
4 | A First App | 04:44 |
5 | Executing Typescript Code | 05:04 |
6 | One Quick Change | 03:36 |
7 | Catching Errors with Typescript | 07:23 |
8 | Catching More Errors! | 05:16 |
9 | Do Not Skip - Course Overview | 03:37 |
10 | Types | 05:13 |
11 | More on Types | 05:54 |
12 | Examples of Types | 04:50 |
13 | Where Do We Use Types? | 00:50 |
14 | Type Annotations and Inference | 02:04 |
15 | Annotations with Variables | 04:54 |
16 | Object Literal Annotations | 06:54 |
17 | Annotations Around Functions | 05:57 |
18 | Understanding Inference | 03:52 |
19 | The 'Any' Type | 07:48 |
20 | Fixing the 'Any' Type | 01:50 |
21 | Delayed Initialization | 03:06 |
22 | When Inference Doesn't Work | 04:38 |
23 | More on Annotations Around Functions | 04:57 |
24 | Inference Around Functions | 06:09 |
25 | Annotations for Anonymous Functions | 01:43 |
26 | Void and Never | 02:50 |
27 | Destructuring with Annotations | 03:36 |
28 | Annotations Around Objects | 07:06 |
29 | Arrays in Typescript | 05:06 |
30 | Why Typed Arrays? | 04:31 |
31 | Multiple Types in Arrays | 02:58 |
32 | When to Use Typed Arrays | 00:55 |
33 | Tuples in Typescript | 04:06 |
34 | Tuples in Action | 05:29 |
35 | Why Tuples? | 03:21 |
36 | Interfaces | 01:27 |
37 | Long Type Annotations | 04:43 |
38 | Fixing Long Annotations with Interfaces | 04:37 |
39 | Syntax Around Interfaces | 03:32 |
40 | Functions in Interfaces | 04:47 |
41 | Code Reuse with Interfaces | 04:16 |
42 | General Plan with Interfaces | 03:13 |
43 | Classes | 03:48 |
44 | Basic Inheritance | 03:04 |
45 | Instance Method Modifiers | 06:42 |
46 | Fields in Classes | 06:19 |
47 | Fields with Inheritance | 04:19 |
48 | Where to Use Classes | 01:11 |
49 | App Overview | 02:46 |
50 | Bundling with Parcel | 04:55 |
51 | Project Structure | 03:20 |
52 | Generating Random Data | 05:30 |
53 | Type Definition Files | 05:18 |
54 | Using Type Definition Files | 06:21 |
55 | Export Statements in Typescript | 05:07 |
56 | Defining a Company | 04:44 |
57 | Adding Google Maps Support | 07:39 |
58 | Google Maps Integration | 04:07 |
59 | Exploring Type Definition Files | 12:47 |
60 | Hiding Functionality | 06:29 |
61 | Why Use Private Modifiers? Here's Why | 08:26 |
62 | Adding Markers | 09:19 |
63 | Duplicate Code | 02:46 |
64 | One Possible Solution | 06:39 |
65 | Restricting Access with Interfaces | 05:36 |
66 | Implicit Type Checks | 03:27 |
67 | Showing Popup Windows | 06:48 |
68 | Updating Interface Definitions | 07:12 |
69 | Optional Implements Clauses | 06:07 |
70 | App Wrapup | 08:09 |
71 | App Overview | 01:35 |
72 | Configuring the TS Compiler | 07:41 |
73 | Concurrent Compilation and Execution | 05:06 |
74 | A Simple Sorting Algorithm | 04:48 |
75 | Sorter Scaffolding | 03:11 |
76 | Sorting Implementation | 05:18 |
77 | Two Huge Issues | 07:38 |
78 | Typescript is Really Smart | 09:35 |
79 | Type Guards | 09:15 |
80 | Why is This Bad? | 02:23 |
81 | Extracting Key Logic | 07:30 |
82 | Separating Swapping and Comparison | 13:59 |
83 | The Big Reveal | 04:39 |
84 | Interface Definition | 04:49 |
85 | Sorting Arbitrary Collections | 11:09 |
86 | Linked List Implementation | 24:16 |
87 | Just...One...More...Fix... | 04:04 |
88 | Integrating the Sort Method | 02:45 |
89 | Issues with Inheritance | 06:55 |
90 | Abstract Classes | 06:26 |
91 | Why Use Abstract Classes? | 04:31 |
92 | Solving All Issues with Abstract Classes | 04:01 |
93 | Interfaces vs Abstract Classes | 03:24 |
94 | Project Overview | 01:46 |
95 | Project Setup | 05:58 |
96 | Type Definition Files - Again! | 06:46 |
97 | Reading CSV Files | 05:06 |
98 | Running an Analysis | 04:23 |
99 | Losing Dataset Context | 05:13 |
100 | Using Enums | 06:20 |
101 | When to Use Enums | 07:05 |
102 | Extracting CSV Reading | 08:51 |
103 | Data Types | 02:34 |
104 | Converting Date Strings to Dates | 05:03 |
105 | Converting Row Values | 03:38 |
106 | Type Assertions | 03:58 |
107 | Describing a Row with a Tuple | 07:29 |
108 | Not Done with FileReader Yet! | 03:15 |
109 | Understanding Refactor #1 | 04:36 |
110 | Creating Abstract Classes | 04:22 |
111 | Variable Types with Generics | 11:29 |
112 | Applying a Type to a Generic Class | 04:12 |
113 | Alternate Refactor | 04:21 |
114 | Interface-Based Approach | 02:05 |
115 | Extracting Match References - Again! | 02:34 |
116 | Transforming Data | 02:37 |
117 | Updating Reader References | 03:40 |
118 | Inheritance vs Composition | 03:21 |
119 | More on Inheritance vs Composition | 07:23 |
120 | A Huge Misconception Around Composition | 14:57 |
121 | Goal Moving Forward | 05:17 |
122 | A Composition-Based Approach | 06:27 |
123 | Implementing an Analyzer Class | 07:25 |
124 | Building the Reporter | 05:27 |
125 | Putting It All Together | 03:36 |
126 | Generating HTML Reports | 05:10 |
127 | One Last Thing! | 05:35 |
128 | Oops, My Bad | 03:15 |
129 | App Wrapup | 03:34 |
130 | More on Generics | 05:05 |
131 | Type Inference with Generics | 02:50 |
132 | Function Generics | 06:06 |
133 | Generic Constraints | 05:51 |
134 | App Overview | 02:46 |
135 | Parcel Setup | 02:43 |
136 | Framework Structure | 08:06 |
137 | Designing the User | 02:49 |
138 | Retrieving User Properties | 06:00 |
139 | Optional Interface Properties | 06:38 |
140 | An Eventing System | 02:45 |
141 | Listener Support | 04:24 |
142 | Storing Event Listeners | 05:25 |
143 | Dynamic Array Creation | 05:28 |
144 | Triggering Event Callbacks | 03:58 |
145 | Adding JSON Server | 07:15 |
146 | Understanding REST Conventions | 08:53 |
147 | Adding Fetch Functionality | 05:13 |
148 | Successfully Fetching Model Data | 04:51 |
149 | Saving User Data | 08:58 |
150 | Refactoring with Composition | 03:49 |
151 | Re-Integrating Eventing | 12:50 |
152 | Composition with Nested Objects | 02:46 |
153 | A More Complicated Extraction | 02:49 |
154 | Options for Adapting Sync | 09:33 |
155 | Refactoring Sync | 10:42 |
156 | Generic Constraints Around Sync | 03:31 |
157 | Connecting Sync Back to User | 04:31 |
158 | Optional Properties | 07:01 |
159 | Extracting an Attributes Class | 03:13 |
160 | The Get Method's Shortcoming | 06:19 |
161 | Two Important Rules | 07:07 |
162 | An Advanced Generic Constraint | 09:37 |
163 | Re-Integrating Attributes | 03:19 |
164 | Composition is Delegation | 04:40 |
165 | Reminder on Accessors | 05:08 |
166 | Passthrough Methods | 06:56 |
167 | A Context Issue | 08:38 |
168 | Setting Data While Triggering | 03:23 |
169 | Fetching User Data | 06:27 |
170 | Saving Data | 05:39 |
171 | Composition vs Inheritance...Again! | 09:03 |
172 | Extracting a Model Class | 07:30 |
173 | Extending the User | 05:25 |
174 | Final User Refactor | 03:49 |
175 | Model Wrapup | 03:39 |
176 | Shortened Passthrough Methods | 05:40 |
177 | Users Collection | 03:27 |
178 | Implementing a Users Collection | 03:02 |
179 | Parsing User JSON | 07:13 |
180 | Generic User Collection | 06:23 |
181 | A Class Method for Collections | 02:15 |
182 | View Classes | 04:04 |
183 | Building the UserForm | 04:46 |
184 | The UserForm's Render Method | 03:04 |
185 | Rendering HTML | 02:07 |
186 | Defining an Events Map | 06:14 |
187 | Binding Event Handlers | 07:07 |
188 | Adding Model Properties | 04:16 |
189 | Binding Events on Class Name | 05:09 |
190 | Adding Methods to the User | 04:31 |
191 | Re-Rendering on Model Change | 04:57 |
192 | Reading Input Text | 04:40 |
193 | Strict Null Checks | 06:13 |
194 | Reusable View Logic | 12:26 |
195 | Extracting a View Class | 04:11 |
196 | Extending with Generic Constraints | 12:16 |
197 | Saving Data From a View | 03:58 |
198 | UserEdit and UserShow | 06:13 |
199 | Nesting with Regions | 04:49 |
200 | Mapping Regions | 05:41 |
201 | Testing Region Mapping | 04:02 |
202 | View Nesting | 06:54 |
203 | Collection Views | 02:21 |
204 | CollectionView Implementation | 16:53 |
205 | App Wrapup | 03:36 |
206 | Typescript with JS Libraries | 05:35 |
207 | App Overview | 03:20 |
208 | Project Setup | 03:32 |
209 | Basic Routes with Express | 06:32 |
210 | Using an Express Router | 03:47 |
211 | Parsing Form Bodies | 05:56 |
212 | Why Doesn't Express Play Nicely with TS? | 13:56 |
213 | Issues with Type Definition Files | 10:17 |
214 | Dealing with Poor Type Defs | 06:29 |
215 | Wiring Up Sessions | 08:37 |
216 | Checking Login Status | 03:20 |
217 | Logging Out | 01:10 |
218 | Protecting Routes | 04:52 |
219 | A Closer Integration | 06:34 |
220 | The Refactoring Process | 03:15 |
221 | Prototypes Reminder | 08:44 |
222 | Decorators in Typescript | 06:49 |
223 | Details on Decorators | 07:41 |
224 | Property Descriptors | 06:12 |
225 | Wrapping Methods with Descriptors | 02:08 |
226 | Decorator Factories | 02:47 |
227 | Decorators Around Properties | 03:58 |
228 | More on Decorators | 07:33 |
229 | A Quick Disclaimer | 00:59 |
230 | Project Overview | 08:18 |
231 | Why is This Hard? | 07:07 |
232 | Solution Overview | 05:52 |
233 | Basics of Metadata | 08:47 |
234 | Practical Metadata | 11:09 |
235 | Let's Refactor! | 04:52 |
236 | The 'Get' Decorator | 04:26 |
237 | The Controller Decorator | 04:21 |
238 | Proof of Concept | 05:13 |
239 | A Few Fixups | 08:10 |
240 | Defining a RouteBinder | 05:05 |
241 | Closed Method Sets with Enums | 07:39 |
242 | Metadata Keys | 03:44 |
243 | The 'Use' Decorator | 08:02 |
244 | Testing Use | 03:23 |
245 | Body Validators | 05:56 |
246 | Automated Validation | 03:26 |
247 | Testing Automated Validation | 05:37 |
248 | Fixing Routes | 06:04 |
249 | Using Property Descriptors for Type Checking | 05:19 |
250 | App Wrapup | 01:23 |
251 | React and Redux Overview | 06:06 |
252 | App Overview | 02:07 |
253 | Generating the App | 01:08 |
254 | Simple Components | 03:36 |
255 | Interfaces with Props | 03:26 |
256 | Handling Component State | 02:31 |
257 | Confusing Component State! | 10:41 |
258 | Functional Components | 03:16 |
259 | Redux Setup | 07:32 |
260 | Action Creators with Typescript | 06:46 |
261 | Action Types Enum | 04:52 |
262 | The Generic Dispatch Function | 04:49 |
263 | A Reducer with Enums | 06:47 |
264 | Validating Store Structure | 05:07 |
265 | Connecting a Component to Redux | 06:11 |
266 | Rendering a List | 04:37 |
267 | Adding in Delete Functionality | 02:55 |
268 | Breaking Out Action Creators | 03:22 |
269 | Expressing Actions as Type Union | 04:08 |
270 | Type Guards in Reducers | 02:49 |
271 | Again, Type Definition Files | 03:02 |
272 | Tracking Loading with Component State | 04:07 |
273 | App Wrapup | 03:39 |
274 | Generating TypeScript Enabled Projects | 01:23 |
275 | File Extensions and Starting Up React | 04:35 |
276 | Changes with TypeScript | 02:34 |
277 | The Big Difference with Props | 03:55 |
278 | Explicit Component Type Annotations | 05:51 |
279 | Annotations with Children | 05:48 |
280 | State with TypeScript | 04:42 |
281 | Type Inference with State | 05:42 |
282 | Quick Finish to the Example | 00:56 |
283 | More on State | 04:20 |
284 | Type Unions in State | 05:42 |
285 | Inline Event Handlers | 03:19 |
286 | Typing Standalone Event Handlers | 02:41 |
287 | Handling Drag Events Too! | 03:45 |
288 | Applying Types to Refs | 05:36 |
289 | More on Refs | 04:31 |
290 | App Overview | 02:05 |
291 | Project Setup | 01:50 |
292 | Redux Store Design | 08:54 |
293 | Reducer Setup | 08:17 |
294 | Annotating the Return Type | 01:27 |
295 | Typing an Action | 01:55 |
296 | Separate Interfaces for Actions | 04:22 |
297 | Applying Action Interfaces | 04:23 |
298 | Adding an Action Type Enum | 05:42 |
299 | A Better Way to Organize Code | 03:38 |
300 | Adding Action Creators | 04:28 |
301 | Adding Request Logic | 03:16 |
302 | Applying Typings to Dispatch | 02:47 |
303 | Setting Up Exports | 04:20 |
304 | Wiring Up to React | 03:25 |
305 | Oops... Initial State! | 01:04 |
306 | Reminder on Event Types | 03:01 |
307 | Calling an Action Creator | 03:06 |
308 | Binding Action Creators | 04:22 |
309 | Selecting State | 04:17 |
310 | Awkward Typings Around React-Redux | 04:47 |
311 | Creating a Typed Selector | 04:01 |
312 | Consuming Store State | 03:03 |
313 | Quick Wrapup - Final Lecture | 03:19 |
Similar courses to Typescript: The Complete Developer's Guide
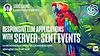
Responsive LLM Applications with Server-Sent Eventsfullstack.io
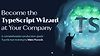
Professional TypeScript Training by Matt Pocock | Total TypeScriptMatt Pocock
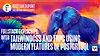
Fullstack Typescript with TailwindCSS and tRPC Using Modern Features of PostgreSQLfullstack.io
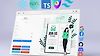
FULL Authentication WITH REACT JS NEXT JS TYPESCRIPTudemy
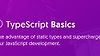
TypeScript Basicsultimatecourses.com
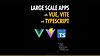
Course: Large Scale Apps with Vue, Vite and TypeScriptDamiano Fusco
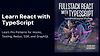
Fullstack React with Typescriptfullstack.io
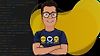
TypeScript Design Patterns And SOLID Principlesudemy
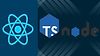