TypeScript Design Patterns And SOLID Principles
Welcome to this one-of-a-kind course specifically designed to transform your TypeScript programming skills by diving deep into the world of Gang Of Four Design Patterns, SOLID Design principles, and Object-Oriented Programming (OOP) concepts. Are you an aspiring or intermediate programmer looking to level up your game? Or are you an advanced programmer and need a refresher on the Gang Of Four Design Patterns and SOLID Design Principles? Do you have a grasp of TypeScript and now want to focus on architectural excellence and code reusability? If so, you've come to the right place!
This course isn't just another tutorial; it's your passport to becoming an advanced TypeScript developer. Throughout more than 140 high-definition videos, totaling over 10 hours of content, we'll delve into the nuances of effective software design and programming. We go beyond theory by providing practical, hands-on coding exercises and quizzes that reinforce your learning and provide the skills you need for the real world. With this course, you don't just learn; you practice, implement, and master the art of writing clean, efficient, and robust TypeScript code using the SOLID Design Principles and Gang Of For Design Patterns using TypeScript.
Watch Online TypeScript Design Patterns And SOLID Principles
# | Title | Duration |
---|---|---|
1 | Welcome | 03:23 |
2 | How To Make The Most Out Of This Course | 02:49 |
3 | Note About Resource | 02:15 |
4 | Intro To Design Patterns and Their History | 04:10 |
5 | Why We Need Design Patterns | 04:50 |
6 | Cautions and Considerations | 07:06 |
7 | Classification Of Design Patterns | 02:36 |
8 | Intro To UML | 07:31 |
9 | Setup Development Environment | 09:43 |
10 | What is OOP | 04:50 |
11 | OOP Lingo | 07:42 |
12 | Abstraction | 12:02 |
13 | Abstraction Implementation | 04:06 |
14 | Abstraction Real World Use case | 04:44 |
15 | Abstraction Advantages | 03:05 |
16 | Encapsulation | 13:45 |
17 | Encapsulation Implementation | 02:24 |
18 | Encapsulation Advantages | 02:37 |
19 | Polymorphism (Subtype) | 04:43 |
20 | Polymorphism Use Cases | 04:09 |
21 | Polymorphism Advantages | 06:46 |
22 | Inheritance | 06:01 |
23 | Inheritance Implementation | 09:29 |
24 | Inheritance Advantages | 02:11 |
25 | SOLID Design Principles Introduction | 03:40 |
26 | Single Responsibility Principle (SRP) Intro | 05:29 |
27 | Real World Application Of SRP | 03:55 |
28 | Advantages Single Responsibility Principle | 04:55 |
29 | Open Closed Principle (OCP) Intro | 05:07 |
30 | Real World Application Open Closed Principle | 06:46 |
31 | Advantages Of Open Closed Principle | 08:58 |
32 | Liskov Substitution Principle (LSP) | 07:47 |
33 | Real World Application LSP | 11:01 |
34 | Advantages Of The Liskov Substitution Principle | 03:39 |
35 | Interface Segregation Principle (ISP) | 08:09 |
36 | Real World Application of ISP | 07:43 |
37 | Advantages Of Interface Segregation Principle | 02:46 |
38 | Dependency Inversion Principle (DIP) | 07:40 |
39 | Implementation Of Dependency Inversion Principle (DIP) | 07:54 |
40 | Advantages Of Dependency Inversion Principle | 02:24 |
41 | Introduction To Creational Design Patterns | 02:11 |
42 | Singleton Pattern | 10:57 |
43 | When To Use Singleton Pattern | 03:33 |
44 | Singleton Real World Implementation | 07:04 |
45 | Singleton Advantages | 02:48 |
46 | Caveats or Criticism Of Singleton Pattern | 09:36 |
47 | Singleton Use Cases | 04:46 |
48 | Prototype Pattern | 11:04 |
49 | When To Use Prototype Pattern | 03:38 |
50 | Prototype Real World Implementation | 12:07 |
51 | Prototype Advantages | 04:14 |
52 | Caveats or Criticism Of Prototype Pattern | 05:30 |
53 | Prototype Use Cases | 03:10 |
54 | Builder Pattern | 21:55 |
55 | When To Use Builder Pattern | 04:13 |
56 | Builder Real World Implementation | 22:01 |
57 | Builder Pattern Advantages | 06:35 |
58 | Caveats or Criticism Of Builder Pattern | 06:01 |
59 | Builder Use Cases | 02:55 |
60 | Factory Pattern | 14:05 |
61 | When To Use Factory Pattern | 04:12 |
62 | Factory Real World Implementation | 09:35 |
63 | Factory Pattern Advantages | 04:12 |
64 | Caveats or Criticism Of Factory Pattern | 05:09 |
65 | Factory Pattern Use Cases | 02:24 |
66 | Abstract Factory Pattern | 14:23 |
67 | When To Use Abstract Factory Pattern | 02:57 |
68 | Abstract Factory Real World Implementation | 16:32 |
69 | Abstract Factory Advantages | 04:52 |
70 | Caveats or Criticism Of Abstract Factory Pattern | 05:39 |
71 | Abstract Factory Pattern Use Cases | 04:45 |
72 | Introduction To Structural Design Patterns | 02:39 |
73 | Facade Pattern | 09:22 |
74 | When To Use Facade Pattern | 05:02 |
75 | Facade Real World Implementation | 13:35 |
76 | Facade Pattern Advantages | 04:06 |
77 | Caveats or Criticism Of Facade Pattern | 04:58 |
78 | Facade Pattern Use Cases | 04:08 |
79 | Bridge Pattern | 13:20 |
80 | When To Use Bridge Pattern | 05:30 |
81 | Bridge Real World Implementation | 10:01 |
82 | Bridge Pattern Advantages | 03:01 |
83 | Caveats or Criticism Of Bridge Pattern | 02:26 |
84 | Bridge Pattern Use Cases | 03:52 |
85 | Composite Pattern | 19:44 |
86 | When To Use Composite Pattern | 03:13 |
87 | Composite Real World Implementation | 16:09 |
88 | Composite Pattern Advantages | 01:53 |
89 | Caveats or Criticism Of Composite Pattern | 05:31 |
90 | Composite Pattern Use Cases | 02:15 |
91 | Decorator Pattern | 12:59 |
92 | When To Use Decorator Pattern | 03:45 |
93 | Decorator Real World Implementation | 12:06 |
94 | Decorator Pattern Advantages | 03:36 |
95 | Caveats or Criticism Of Decorator Pattern | 05:23 |
96 | Decorator Pattern Use Cases | 02:43 |
97 | Adapter Pattern | 11:01 |
98 | When To Use Adapter Pattern | 04:33 |
99 | Adapter Real World Implementation | 11:52 |
100 | Adapter Pattern Advantages | 03:29 |
101 | Caveats or Criticism Of Adapter Pattern | 04:21 |
102 | Adapter Pattern Use Cases | 04:27 |
103 | Introduction To Behavioural Design Patterns | 01:50 |
104 | Observer Pattern | 24:13 |
105 | When To Use Observer Pattern | 03:34 |
106 | Observer Real World Implementation | 22:44 |
107 | Observer Pattern Advantages | 04:12 |
108 | Caveats Or Criticism Of Observer Pattern | 04:15 |
109 | Observer Patterns Use Cases | 03:28 |
110 | Iterator Pattern | 12:54 |
111 | When To Use Iterator Pattern | 06:48 |
112 | Iterator Real World Implementation | 20:58 |
113 | Iterator Pattern Advantages | 03:57 |
114 | Caveats Or Criticism Of Iterator Pattern | 03:25 |
115 | Iterator Patterns Use Cases | 02:42 |
116 | Strategy Design Pattern | 12:59 |
117 | When To Use The Strategy Pattern | 03:41 |
118 | Strategy Real World Implementation | 08:57 |
119 | Strategy Pattern Advantages | 04:02 |
120 | Caveats Or Criticism Of The Strategy Pattern | 04:07 |
121 | Strategy Pattern Use Cases | 02:33 |
122 | Template Method Pattern | 15:23 |
123 | When To Use The Template Pattern | 04:32 |
124 | Template Pattern Real World Implementation | 10:37 |
125 | Template Pattern Advantages | 05:15 |
126 | Caveats Or Criticism Of The Template Pattern | 06:34 |
127 | Template Pattern Use Cases | 03:09 |
128 | Command Design Pattern | 19:57 |
129 | When To Use The Command Pattern | 05:34 |
130 | Command Pattern Real World Implementation | 21:07 |
131 | Command Pattern Advantages | 04:06 |
132 | Caveats Or Criticism Of The Command Pattern | 03:58 |
133 | Command Pattern Use Cases | 03:53 |
134 | State Design Pattern | 11:10 |
135 | When To Use The State Design Pattern | 04:40 |
136 | State Design Pattern Real World Implementation | 09:06 |
137 | State Design Pattern Advantages | 03:49 |
138 | Caveats Or Criticism Of The State Design Pattern | 03:04 |
139 | State Design Pattern Use Cases | 02:51 |
140 | Chain of Responsibility Pattern | 18:57 |
141 | When To Use Chain of Responsibility | 05:44 |
142 | Chain of Responsibility Real World Implementation | 15:50 |
143 | Chain of Responsibility Advantages | 04:18 |
144 | Caveats Or Criticism Of The Chain of Responsibility | 04:29 |
145 | Chain of Responsibility Use Cases | 04:39 |
Similar courses to TypeScript Design Patterns And SOLID Principles
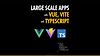
Course: Large Scale Apps with Vue, Vite and TypeScriptDamiano Fusco
![React & TypeScript Chrome Extension Development [2021]](https://cdn.courseflix.net/courses/100x56/react-typescript-chrome-extension-development-2021.jpg?d=1750848188190)
React & TypeScript Chrome Extension Development [2021]udemy
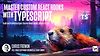
Master Custom React Hooks with TypeScriptfullstack.io
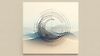
Practical Typescriptudemy
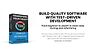
Transform Your Craft with TDD: Master clean code and testingDaniel Moka
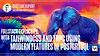
Fullstack Typescript with TailwindCSS and tRPC Using Modern Features of PostgreSQLfullstack.io
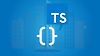
Understanding TypeScript - 2023 EditionudemyAcademind Pro
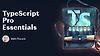