NFT Marketplace in React, Typescript & Solidity - Full Guide
The course covers everything you need for a decentralized NFT application according to the ERC721 standard. Students of this course will learn about NFTs by creating a real-world application. Students will learn what ERC721 smart contract is and how to create one. The frontend for dApps covered in the course is created with React JS library and Next JS framework. CSS and design are built with the Tailwind framework.
Read more about the course
Next.js gives you the best developer experience with all the features you need for production: hybrid static & server rendering, TypeScript support, smart bundling, route pre-fetching, etc. No config is necessary.
Ethereum is a technology that lets you send cryptocurrency to anyone, but in the first place, It also powers applications that everyone can use and no one can takedown. It's the world's programmable blockchain.
Solidity is an object-oriented, high-level language for implementing smart contracts. Smart contracts are programs that govern the behavior of accounts within the Ethereum state.
Pinata is a pinning service that allows users to host files on the IPFS network. The InterPlanetary File System (IPFS) is a protocol and peer-to-peer network for storing and sharing data in a distributed file system.
NFT Marketplace Application:
This central part of the course covers the creation of an interactive NFT marketplace. Clients of this platform will be able to purchase NFTs with Ether digital currency.
The application is created in the Next JS framework, built on top of the React JS.
The first part of the course covers the setup of the pages layouts, creation of the first pages, and components. The Tailwind CSS framework covers the styling part of the application.
Students will explore how to provide Web3 JS code into the application, code responsible for communication with a crypto wallet and, therefore, with the blockchain.
We will use the Provider/Consumer concept to provide this layer, which is very common in React JS. This will guarantee that all of the components and pages will be able to access functionalities responsible for communication to the blockchain.
The application's state and data management are handled through the SWR(stale while revalidate) library. This will provide a reactive feel to the application and a reactive rendering of components upon receiving new data.
After all, necessary communication with blockchain is achieved, the students will start to work on smart contract implementation.
The course follows the recognized ERC721 token standard.
The smart contract is an essential part of the project. It serves as the blockchain "storage" of NFTs and provides functionality to verify ownership of an NFT and linkage to the media storage.
The last part of the course covers preparing and storing data related to NFT. So-called NFT Metadata.
NFT metadata data will be stored on Pinata (IPFS) based storage. Students will learn to gather data from the form and later submit it to Pinata storage. The following important part is to link this metadata and create an NFT.
Watch Online NFT Marketplace in React, Typescript & Solidity - Full Guide
# | Title | Duration |
---|---|---|
1 | Introduction | 06:22 |
2 | Ask For Help | 00:35 |
3 | How to resolve issues | 05:52 |
4 | Init Project | 05:32 |
5 | Tailwind | 05:19 |
6 | Navbar | 08:34 |
7 | Cleanup | 02:00 |
8 | BaseLayout | 07:53 |
9 | Listing Page | 06:52 |
10 | Nft Components | 09:51 |
11 | Nft props | 06:05 |
12 | Nft Type | 05:37 |
13 | Display nft item values | 06:29 |
14 | Links and create page | 09:51 |
15 | Profile Link | 02:43 |
16 | Active Link | 05:34 |
17 | Create Page Layout | 06:39 |
18 | Profile Layout | 03:54 |
19 | Paths refactor | 08:24 |
20 | Web3Provider | 09:54 |
21 | Planning | 13:12 |
22 | Web3 Types | 05:21 |
23 | Web3 State | 08:02 |
24 | Window Ethereum | 10:13 |
25 | Provider | 04:54 |
26 | Ganache Install | 06:48 |
27 | Base smart contract | 11:00 |
28 | ERC721 | 08:51 |
29 | Truffle Console | 05:36 |
30 | Load Contract | 11:59 |
31 | Call name, symbol | 07:50 |
32 | Use Account | 09:52 |
33 | Hook Types | 08:23 |
34 | Generic Types | 08:34 |
35 | Setup Hooks | 12:05 |
36 | Create web3 state | 06:59 |
37 | Use Account Abstraction | 05:28 |
38 | Recap | 05:00 |
39 | Conditional swr | 06:32 |
40 | Account data | 09:05 |
41 | Connect button | 06:07 |
42 | Connect Type Definition | 04:02 |
43 | Handle accounts changed | 06:06 |
44 | Mutate account | 04:44 |
45 | Is loading and validating | 07:48 |
46 | Handle error when no wallet | 03:59 |
47 | Walletbar | 05:24 |
48 | Wallet conditionals | 11:18 |
49 | Use Network | 06:20 |
50 | Network Name | 05:58 |
51 | Network label | 01:36 |
52 | Target network | 06:03 |
53 | Handle Network Loading | 03:20 |
54 | Reload on network change | 09:03 |
55 | Handle Wallet Lock Out | 06:23 |
56 | Fix Loading and undefined Ethereum | 04:53 |
57 | Mint Token | 07:17 |
58 | AI Mint Token | 11:43 |
59 | Prepare test | 09:20 |
60 | Mint token test | 08:55 |
61 | TokenURI test | 06:12 |
62 | Check if token uri exists | 04:27 |
63 | Duplicate URI Test | 07:31 |
64 | Create NFT item | 09:50 |
65 | Listing Price Test | 07:53 |
66 | NFT item test | 06:11 |
67 | Listing price | 04:51 |
68 | Buy NFT | 10:29 |
69 | Buy NFT test | 07:05 |
70 | Add token to all enumeration | 09:54 |
71 | AI Add token to all enumerations | 08:01 |
72 | Test token transfers | 06:33 |
73 | Get all nfts on sale | 05:01 |
74 | AI get all nfts on sale | 09:20 |
75 | Test get all listed nfts | 06:24 |
76 | Add token to owner enums | 06:26 |
77 | AI add token to owner enums | 06:06 |
78 | Get owned Nfts | 07:55 |
79 | Test owned Nfts | 04:22 |
80 | Remove token from owned enums | 06:05 |
81 | AI Remove token from owned enums | 13:49 |
82 | Test Remove token from owned enums | 07:45 |
83 | Remove token from all enums | 04:48 |
84 | AI Remove token from all enums | 06:26 |
85 | Test Burn token | 10:14 |
86 | Place nft on sale | 09:28 |
87 | Test listing of nfts | 09:08 |
88 | Use listed Nfts | 10:56 |
89 | Upload Images to Pinata | 09:17 |
90 | Mint Token in Truffle | 08:23 |
91 | Get Nft Data | 05:04 |
92 | Contract Type | 09:31 |
93 | Get Complete Nfts | 08:04 |
94 | Display Nfts | 05:03 |
95 | Sign Contract | 04:50 |
96 | Use owned nfts | 07:31 |
97 | Active Nft | 03:51 |
98 | Set Active Nft | 05:02 |
99 | Buy Nft in Hook | 11:56 |
100 | List Nft | 08:48 |
101 | UI changes active nft | 02:46 |
102 | Use Callback | 06:43 |
103 | Handle part of form | 10:11 |
104 | Handle attribute change | 04:41 |
105 | Introduce Verification architecture | 15:46 |
106 | With iron session | 10:26 |
107 | Create message in server | 12:34 |
108 | Fix reload of the browser | 04:45 |
109 | Sign message | 08:18 |
110 | Verify form data | 07:26 |
111 | Get session back | 11:31 |
112 | Get contract server side | 07:19 |
113 | Verify Signature | 11:56 |
114 | Upload Metadata to Pinata | 14:50 |
115 | Get image bytes | 04:52 |
116 | Get signed data | 03:47 |
117 | Image upload endpoint | 11:28 |
118 | Fix image select issue | 03:29 |
119 | Upload image success | 09:27 |
120 | Store image to state | 05:38 |
121 | Check Nft Structure | 11:14 |
122 | Get Price | 03:26 |
123 | Create a Nft | 06:08 |
124 | Handle promises | 08:34 |
125 | Handle rest of promises | 04:31 |
126 | Display wrong network | 04:53 |
127 | Course Done! | 04:22 |
128 | Ropsten preparation | 08:26 |
129 | Deploy to Ropsten | 12:11 |
130 | Testing app on Ropsten | 09:11 |
131 | Github Repo | 09:15 |
132 | Deploy to Vercel | 10:18 |
Similar courses to NFT Marketplace in React, Typescript & Solidity - Full Guide

MERN Stack Web Development with Ultimate Authenticationudemy
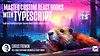
Master Custom React Hooks with TypeScriptfullstack.io
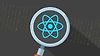
React - The Complete GuideudemyAcademind Pro
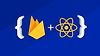
The essential guide to Firebase with React.udemy
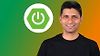
Master Spring Boot 3 & Spring Framework 6 with Javaudemy
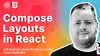
Composing Layouts in Reactfullstack.io
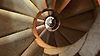
Testing React with Jest and Testing Libraryudemy
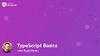
TypeScript Fundamentalsultimatecourses.com
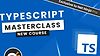
TypeScript MasterclassNet Ninja
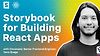