Python 3: Deep Dive (Part 1 - Functional)
This is Part 1 of a series of courses intended to dive into the inner mechanics and more complicated aspects of Python 3. This is not a beginner course - if you've been coding Python for a week or a couple of months, you probably should keep writing Python for a bit more before tackling this series.
Read more about the course
On the other hand, if you're now starting to ask yourself questions like:
I wonder how this works?
is there another way of doing this?
what's a closure? is that the same as a lambda?
I know how to use a decorator someone else wrote, but how does it work? Can I write my own?
why isn't this boolean expression returning a boolean value?
what does an import actually do, and why am I getting side effects?
and similar types of question...
then this course is for you.
Please make sure you review the pre-requisites for this course - although I give a brief refresh of basic concepts at the beginning of the course, those are concepts you should already be very comfortable with as you being this course.
In this course series, I will give you a much more fundamental and deeper understanding of the Python language and the standard library.
Python is called a "batteries-included" language for good reason - there is a ton of functionality in base Python that remains to be explored and studied.
So this course is not about explaining my favorite 3rd party libraries - it's about Python, as a language, and the standard library.
In particular this course is based on the canonical CPython. You will also need Jupyter Notebooks to view the downloadable fully-annotated Python notebooks.
It's about helping you explore Python and answer questions you are asking yourself as you develop more and more with the language.
In Python 3: Deep Dive (Part 1) we will take a much closer look at:
Variables - in particular that they are just symbols pointing to objects in memory
Namespaces and scope
Python's numeric types
Python boolean type - there's more to a simple or statement than you might think!
Run-time vs compile-time and how that affects function defaults, decorators, importing modules, etc
Functions in general (including lambdas)
Functional programming techniques (such as map, reduce, filter, zip, etc)
Closures
Decorators
Imports, modules and packages
Tuples as data structures
Named tuples
To get the most out of this course, you should be prepared to pause the coding videos, and attempt to write code before I do! Sit back during the concept videos, but lean in for the code videos!
And after you have seen a code video, pause the course, and try things out yourself - explore, experiment, play with code, and see how things work (or don't work! - that's also a great way to learn!)
- Basic introductory knowledge of Python programming (variables, conditional statements, loops, functions, lists, tuples, dictionaries, classes).
- You will need Python 3.6 or above, and a development environment of your choice (command line, PyCharm, Jupyter, etc.)
- Anyone with a basic understanding of Python that wants to take it to the next level and get a really deep understanding of the Python language and its data structures.
- Anyone preparing for an in-depth Python technical interview.
What you'll learn:
- An in-depth look at variables, memory, namespaces and scopes
- A deep dive into Python's memory management and optimizations
- In-depth understanding and advanced usage of Python's numerical data types (Booleans, Integers, Floats, Decimals, Fractions, Complex Numbers)
- Advanced Boolean expressions and operators
- Advanced usage of callables including functions, lambdas and closures
- Functional programming techniques such as map, reduce, filter, and partials
- Create advanced decorators, including parametrized decorators, class decorators, and decorator classes
- Advanced decorator applications such as memoization and single dispatch generic functions
- Use and understand Python's complex Module and Package system
- Idiomatic Python and best practices
- Understand Python's compile-time and run-time and how this affects your code
- Avoid common pitfalls
Watch Online Python 3: Deep Dive (Part 1 - Functional)
# | Title | Duration |
---|---|---|
1 | Course Overview | 18:09 |
2 | Introduction | 01:44 |
3 | The Python Type Hierarchy | 05:52 |
4 | Multi-Line Statements and Strings | 21:50 |
5 | Variable Names | 11:01 |
6 | Conditionals | 07:39 |
7 | Functions | 12:28 |
8 | The While Loop | 14:26 |
9 | Break, Continue and the Try Statement | 10:26 |
10 | The For Loop | 17:22 |
11 | Classes | 40:18 |
12 | Introduction | 02:55 |
13 | Variables are Memory References | 08:22 |
14 | Reference Counting | 14:22 |
15 | Garbage Collection | 26:40 |
16 | Dynamic vs Static Typing | 05:29 |
17 | Variable Re-Assignment | 04:49 |
18 | Object Mutability | 15:23 |
19 | Function Arguments and Mutability | 17:30 |
20 | Shared References and Mutability | 09:37 |
21 | Variable Equality | 14:23 |
22 | Everything is an Object | 13:59 |
23 | Python Optimizations: Interning | 09:15 |
24 | Python Optimizations: String Interning | 19:12 |
25 | Python Optimizations: Peephole | 20:10 |
26 | Introduction | 02:59 |
27 | Integers: Data Types | 18:08 |
28 | Integers: Operations | 24:26 |
29 | Integers: Constructors and Bases - Lecture | 29:35 |
30 | Integers: Constructors and Bases - Coding | 20:24 |
31 | Rational Numbers - Lecture | 14:28 |
32 | Rationals Numbers - Coding | 12:34 |
33 | Floats: Internal Representations - Lecture | 19:53 |
34 | Floats: Internal Representations - Coding | 04:57 |
35 | Floats: Equality Testing - Lecture | 18:43 |
36 | Floats: Equality Testing - Coding | 14:41 |
37 | Floats: Coercing to Integers - Lecture | 09:40 |
38 | Floats: Coercing to Integers - Coding | 05:04 |
39 | Floats: Rounding - Lecture | 25:23 |
40 | Floats: Rounding - Coding | 13:34 |
41 | Decimals - Lecture | 16:50 |
42 | Decimals - Coding | 10:28 |
43 | Decimals: Constructors and Contexts - Lecture | 10:07 |
44 | Decimals: Constructors and Contexts - Coding | 10:29 |
45 | Decimals: Math Operations - Lecture | 09:33 |
46 | Decimals: Math Operations - Coding | 13:31 |
47 | Decimals: Performance Considerations | 10:30 |
48 | Complex Numbers - Lecture | 11:29 |
49 | Complex Numbers - Coding | 14:17 |
50 | Booleans | 21:01 |
51 | Booleans: Truth Values - Lecture | 09:09 |
52 | Booleans: Truth Values - Coding | 14:48 |
53 | Booleans: Precedence and Short-Circuiting - Lecture | 21:11 |
54 | Booleans: Precedence and Short-Circuiting - Coding | 13:39 |
55 | Booleans: Boolean Operators - Lecture | 18:01 |
56 | Booleans: Boolean Operators - Coding | 14:46 |
57 | Comparison Operators | 20:54 |
58 | Introduction | 01:06 |
59 | Argument vs Parameter | 03:44 |
60 | Positional and Keyword Arguments - Lecture | 13:06 |
61 | Positional and Keyword Arguments - Coding | 06:22 |
62 | Unpacking Iterables - Lecture | 13:02 |
63 | Unpacking Iterables - Coding | 21:10 |
64 | Extended Unpacking - Lecture | 17:51 |
65 | Extended Unpacking - Coding | 29:05 |
66 | *args - Lecture | 06:01 |
67 | *args - Coding | 11:48 |
68 | Keyword Arguments - Lecture | 09:24 |
69 | Keyword Arguments - Coding | 14:19 |
70 | **kwargs | 10:29 |
71 | Putting it all Together - Lecture | 13:26 |
72 | Putting it all Together - Coding | 17:26 |
73 | Application: A Simple Function Timer | 19:09 |
74 | Parameter Defaults - Beware!! | 18:45 |
75 | Parameter Defaults - Beware Again!! | 19:23 |
76 | Introduction | 04:06 |
77 | Docstrings and Annotations - Lecture | 15:59 |
78 | Docstrings and Annotations - Coding | 15:03 |
79 | Lambda Expressions - Lecture | 12:11 |
80 | Lambda Expressions - Coding | 15:00 |
81 | Lambdas and Sorting | 15:57 |
82 | Challenge - Randomize an Iterable using Sorted!! | 02:56 |
83 | Function Introspection - Lecture | 19:31 |
84 | Function Introspection - Coding | 28:37 |
85 | Callables | 14:47 |
86 | Map, Filter, Zip and List Comprehensions - Lecture | 21:44 |
87 | Map, Filter, Zip and List Comprehensions - Coding | 21:15 |
88 | Reducing Functions - Lecture | 25:53 |
89 | Reducing Functions - Coding | 21:11 |
90 | Partial Functions - Lecture | 11:13 |
91 | Partial Functions - Coding | 25:33 |
92 | The operator Module - Lecture | 15:36 |
93 | The operator Module - Coding | 32:44 |
94 | Introduction | 01:32 |
95 | Global and Local Scopes - Lecture | 34:55 |
96 | Global and Local Scopes - Coding | 15:41 |
97 | Nonlocal Scopes - Lecture | 22:18 |
98 | Nonlocal Scopes - Coding | 14:38 |
99 | Closures - Lecture | 38:36 |
100 | Closures - Coding | 32:06 |
101 | Closure Applications - Part 1 | 15:39 |
102 | Closure Applications - Part 2 | 18:41 |
103 | Decorators (Part 1) - Lecture | 21:07 |
104 | Decorators (Part 1) - Coding | 21:00 |
105 | Decorator Application (Timer) | 35:17 |
106 | Decorator Application (Logger, Stacked Decorators) | 23:48 |
107 | Decorator Application (Memoization) | 29:15 |
108 | Decorators (Part 2) - Lecture | 11:45 |
109 | Decorators (Part 2) - Coding | 25:58 |
110 | Decorator Application (Decorator Class) | 09:41 |
111 | Decorator Application (Decorating Classes) | 48:24 |
112 | Decorator Application (Dispatching) - Part 1 | 31:46 |
113 | Decorator Application (Dispatching) - Part 2 | 35:46 |
114 | Decorator Application (Dispatching) - Part 3 | 26:51 |
115 | Introduction | 03:19 |
116 | Tuples as Data Structures - Lecture | 19:02 |
117 | Tuples as Data Structures - Coding | 25:25 |
118 | Named Tuples - Lecture | 27:50 |
119 | Named Tuples - Coding | 35:15 |
120 | Named Tuples - Modifying and Extending - Lecture | 14:26 |
121 | Named Tuples - Modifying and Extending - Coding | 21:47 |
122 | Named Tuples - DocStrings and Default Values - Lecture | 13:31 |
123 | Named Tuples - DocStrings and Default Values - Coding | 15:47 |
124 | Named Tuples - Application - Returning Multiple Values | 06:23 |
125 | Named Tuples - Application - Alternative to Dictionaries | 28:46 |
126 | Introduction | 03:02 |
127 | What is a Module? | 24:31 |
128 | How does Python Import Modules? | 49:33 |
129 | Imports and importlib | 27:40 |
130 | Import Variants and Misconceptions - Lecture | 14:01 |
131 | Import Variants and Misconceptions - Coding | 27:04 |
132 | Reloading Modules | 18:30 |
133 | Using __main__ | 27:02 |
134 | Modules Recap | 13:03 |
135 | What are Packages? - Lecture | 20:25 |
136 | What are Packages ? - Coding | 27:12 |
137 | Why Packages? | 13:08 |
138 | Structuring Packages - Part 1 | 36:42 |
139 | Structuring Packages - Part 2 | 27:28 |
140 | Namespace Packages | 10:39 |
141 | Importing from Zip Archives | 03:29 |
142 | Introduction | 03:41 |
143 | Additional Resources | 12:54 |
144 | Python 3.6 Highlights | 07:50 |
145 | Python 3.6 - Dictionary Ordering | 19:46 |
146 | Python 3.6 - Preserved Order of kwargs and Named Tuple Application | 05:33 |
147 | Python 3.6 - Underscores in Numeric Literals | 03:39 |
148 | Python 3.6 - f-Strings | 09:20 |
149 | Random: Seeds | 17:27 |
150 | Random Choices | 26:09 |
151 | Random Samples | 07:03 |
152 | Timing code using *timeit* | 16:18 |
153 | Don't Use *args and **kwargs Names Blindly | 07:36 |
154 | Command Line Arguments | 01:00:08 |
155 | Sentinel Values for Parameter Defaults | 11:03 |
156 | Simulating a simple switch in Python | 19:01 |
Similar courses to Python 3: Deep Dive (Part 1 - Functional)
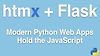
HTMX + Flask: Modern Python Web Apps, Hold the JavaScriptTalkpython
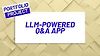
Build an LLM-powered Q&A App using LangChain, OpenAI and Pythonzerotomastery.io
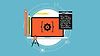
The Ultimate Flask Courseudemy
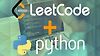
Python & LeetCode | The Ultimate Interview BootCampkaeducation.com
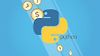
Python - The Practical Guideudemy
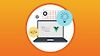
The Complete Guide to Django REST Framework and Vue JSudemy
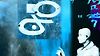
OpenAI API with Python Bootcamp: ChatGPT API, GPT-4, DALL·Eudemy
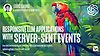
Responsive LLM Applications with Server-Sent Eventsfullstack.io
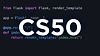
CS50's Web Programming with Python and JavaScriptHarvardX (Harvard University)
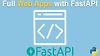