The Coding Interview Bootcamp: Algorithms + Data Structures
Data Structures? They're here. Algorithms? Covered. Lots of questions with well-explained solutions? Yep! If you're nervous about your first coding interview, or anxious about applying to your next job, this is the course for you. I got tired of interviewers asking tricky questions that can only be answered if you've seen the problem before, so I made this course! This video course will teach you the most common interview questions that you'll see in a coding interview, giving you the tools you need to ace your next whiteboard interview.
More
Coding interviews are notoriously intimidating, but there is one method to become a better interviewer - and that is practice! Practicing dozens of interview questions is what makes the difference between a job offer for a $120k USD and another rejection email. This course is going to not only give you dozens of questions to practice on, but it will also make sure you understand the tricks behind solving each question, so you’ll be able to perform in a real interview.
I have spent many hours combing through interview questions asked at Google, Facebook, and Amazon to make sure you know how to answer questions asked by the most well-paying companies out there. No stone is left unturned, as we discuss everything from the simplest questions all the way to the most complex algorithm questions.
In this course, you'll get:
- Clear, well-diagramed explanations for every single problem to make sure you understand the solution
- An overview of the most important data structures to know about. These are presented for people without a CS degree.
- A huge collection of common algorithm questions, including everything from 'reversing a string' to 'determine the width of a BST'
- Sensible strategies for tackling systems design problems
- Insider tips on answering what interviewers area really looking for
- Constant support on the Udemy Q&A forums from me!
My goal in this course is to help you defeat those interviewers who ask nasty algorithm questions. Sign up today, and be the cutting edge engineer who will be prepared to get a high paying job.
- Basic understanding of Javascript
- Anyone preparing for an interview that will involve coding challenges
What you'll learn:
- Master commonly asked interview questions
- Tackle common data structures used in web development
- Practice dozens of different challenges
- Use Javascript to solve challenging algorithms
Watch Online The Coding Interview Bootcamp: Algorithms + Data Structures
# | Title | Duration |
---|---|---|
1 | How to Get Help | 01:08 |
2 | The All Important Coding Interview | 04:06 |
3 | Getting Better at Coding Questions | 05:42 |
4 | Environment Setup | 01:55 |
5 | Repo Test Setup | 05:02 |
6 | First Question! Reverse String. | 02:56 |
7 | String Reversal, Solution #1 | 04:55 |
8 | String Reversal, Solution #2 | 05:56 |
9 | String Reversal, Solution #3 | 05:46 |
10 | Debugger Statements | 09:28 |
11 | Palindromes | 02:58 |
12 | Palindromes, Solution #1 | 02:52 |
13 | Palindromes, Alternate Solution | 08:55 |
14 | Reversing an Int | 06:51 |
15 | Reversing an Int Solution | 06:59 |
16 | Max Chars Problem | 05:34 |
17 | Max Chars Character Map | 05:08 |
18 | Max Chars Solution | 02:47 |
19 | Max Chars Solution Continued | 04:19 |
20 | FizzBuzz Problem Statement | 05:39 |
21 | Solving FizzBuzz with Style | 07:15 |
22 | Array Chunk Problem Statement | 03:16 |
23 | Chunk Solution #1 | 05:51 |
24 | More on Chunk | 04:13 |
25 | Chunk Solution #2 | 04:52 |
26 | Even More on Chunk! | 03:05 |
27 | What Are Anagrams? | 08:24 |
28 | Solving Anagrams | 11:45 |
29 | Another Way to Tackle Anagrams | 08:20 |
30 | Understanding Capitalization | 04:02 |
31 | Capitalization Solution #1 | 03:56 |
32 | How Else Can We Capitalize? | 06:28 |
33 | The Steps Question | 04:19 |
34 | Steps Solution #1 | 06:31 |
35 | Steps Solution #1 Continued | 04:59 |
36 | Step Up Your Steps Game | 07:49 |
37 | More on Steps | 15:51 |
38 | Pyramids Vs Steps | 04:51 |
39 | Pyramid Solution #1 | 09:56 |
40 | Pyramid Solution #2 | 08:39 |
41 | Get Your Vowels | 01:48 |
42 | Finding Vowels | 05:24 |
43 | Another Way to Find Vowels | 03:45 |
44 | General Matrix Spirals | 03:27 |
45 | Spiral Solution | 05:17 |
46 | More on Spiral | 19:19 |
47 | What is Runtime Complexity? | 05:40 |
48 | Determining Complexity | 09:22 |
49 | More on Runtime Complexity | 12:00 |
50 | The Fibonacci Series | 02:37 |
51 | Fibonacci Series Iterative Solution | 05:11 |
52 | Fibonacci Series Recursive Solution | 10:03 |
53 | Memoi-....Mem-...Memoization! | 11:01 |
54 | I Believe Its Memoization! | 11:28 |
55 | What's a Data Structure? | 03:42 |
56 | The Queue Data Structure | 06:39 |
57 | Implementing a Queue | 06:33 |
58 | What's a Weave? | 04:46 |
59 | How to Weave | 05:26 |
60 | Stack Data Structure | 04:46 |
61 | Implementing a Stack | 03:54 |
62 | Queue From Stack Question | 03:26 |
63 | Creating a Queue From Stacks | 07:10 |
64 | More on Queue From Stack | 10:16 |
65 | What's a Linked List? | 06:17 |
66 | Exercise Setup | 05:28 |
67 | Node Implementation | 06:35 |
68 | Linked List's Constructor | 04:43 |
69 | Linked Lists's InsertFirst | 05:12 |
70 | Solving Insert First | 07:05 |
71 | Sizing a List | 02:37 |
72 | Solve for Size | 05:15 |
73 | Get Over Here, GetFirst! | 00:56 |
74 | Building GetFirst | 00:57 |
75 | Find Your Tail with GetLast | 01:50 |
76 | GetLast Implementation | 03:21 |
77 | Clear that List | 01:19 |
78 | Clear Solution | 01:57 |
79 | Where's My Head, RemoveFirst? | 01:36 |
80 | Building RemoveFirst | 02:34 |
81 | Bye-Bye Tail with RemoveLast | 05:06 |
82 | RemoveLast Implementation | 06:18 |
83 | A New Tail to Tell with InsertLast | 03:21 |
84 | Adding InsertLast | 03:43 |
85 | Pick Em Out with GetAt | 02:55 |
86 | GetAt Solution | 06:19 |
87 | Remove Anything with RemoveAt | 06:01 |
88 | RemoveAt Solution | 09:47 |
89 | Insert Anywhere with InsertAt | 03:57 |
90 | InsertAt Solution | 08:26 |
91 | Code Reuse in Linked Lists | 04:16 |
92 | List Traversal Through ForEach | 02:27 |
93 | Brushup on Generators | 20:15 |
94 | Linked Lists with Iterators | 04:42 |
95 | Midpoint of a Linked List | 10:07 |
96 | Midpoint Solution | 03:28 |
97 | Detecting Linked Lists Loops | 07:29 |
98 | Loop Solution | 04:13 |
99 | From Last Question | 06:16 |
100 | From Last Solution | 02:36 |
101 | Trees Overview | 08:21 |
102 | Node Implementation | 06:34 |
103 | More on Nodes | 06:06 |
104 | Tree Implementation | 04:15 |
105 | Traverse By Breadth | 08:41 |
106 | Solving for Breadth-First Traversal | 05:25 |
107 | Depth First Traversal | 04:41 |
108 | Solving for Depth-First Traversal | 02:38 |
109 | Level Width Declaration | 13:00 |
110 | Measuring Level Width | 06:14 |
111 | What's a Binary Search Tree? | 07:08 |
112 | Binary Search Tree Implementation | 04:43 |
113 | BST Insertion | 04:41 |
114 | Do You Contain This? | 02:42 |
115 | Solving Contains | 03:07 |
116 | How to Validate a Binary Search Tree | 05:27 |
117 | More on Validation | 04:20 |
118 | Solution to Validation | 12:52 |
119 | What's an Eventing System? | 07:11 |
120 | A Tip on Events | 02:10 |
121 | Events Solution | 05:09 |
122 | How to Build Twitter | 15:34 |
123 | Sorting Algorithm Overview | 05:08 |
124 | BubbleSort Implementation | 07:13 |
125 | BubbleSort Solution | 04:33 |
126 | How SelectionSort Works | 05:23 |
127 | Selection Sort Solution | 04:15 |
128 | MergeSort Overview | 06:22 |
129 | The Merge Function | 06:56 |
130 | More on MergeSort | 05:56 |
131 | I Don't Like Recursion, But Let's Do This Anyways | 09:50 |
Similar courses to The Coding Interview Bootcamp: Algorithms + Data Structures
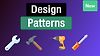
Object-Oriented Design Patterns
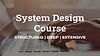
System Design Course
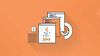
Recursion, Backtracking and Dynamic Programming in Java
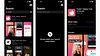
Ultimate SwiftUI Mock Interview AppStore
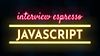
JavaScript Interview Espresso
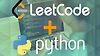
Python & LeetCode | The Ultimate Interview BootCamp
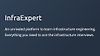
InfraExpert
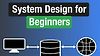
System Design for Beginners
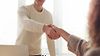