Master the Coding Interview Data Structures Algorithms
Want to land a job at a great tech company like Google, Microsoft, Facebook, Netflix, Amazon, or other companies but you are intimidated by the interview process and the coding questions? Do you find yourself feeling like you get "stuck" every time you get asked a coding question? This course is your answer. Using the strategies, lessons, and exercises in this course, you will learn how to land offers from all sorts of companies.
Read more about the course
Many developers who are "self taught", feel that one of the main disadvantages they face compared to college educated graduates in computer science is the fact that they don't have knowledge about algorithms, data structures and the notorious Big-O Notation. Get on the same level as someone with computer science degree by learning the fundamental building blocks of computer science which will give you a big boost during interviews. You will also get access to our private online chat community with thousands of developers online to help you get through the course.
Here is what you will learn in this course:
Technical:
1. Big O notation
2. Data structures:
* Arrays
* Hash Tables
* Singly Linked Lists
* Doubly Linked Lists
* Queues
* Stacks
* Trees (BST, AVL Trees, Red Black Trees, Binary Heaps)
* Tries
* Graphs
3. Algorithms:
* Recursion
* Sorting
* Searching
* Tree Traversal
* Breadth First Search
* Depth First Search
* Dynamic Programming
Non Technical:
- How to get more interviews
- What to do during interviews
- What do do after the interview
- How to answer interview questions
- How to handle offers
- How to negotiate your salary
- How to get a raise
Unlike most instructors, I am not a marketer or a salesperson. I am a senior developer and programmer who has worked and managed teams of engineers and have been in these interviews both as an interviewee as well as the interviewer.
My job as an instructor will be successful if I am able to help you become better at interviewing and land more jobs. This one skill can really change the course of your career and I hope you sign up today to see what it can do for your career!
Taught by:
Andrei is the instructor of the highest rated Web Development course on Udemy as well as one of the fastest growing. His graduates have moved on to work for some of the biggest tech companies around the world like Apple, Google, JP Morgan, IBM, etc... He has been working as a senior software developer in Silicon Valley and Toronto for many years, and is now taking all that he has learned, to teach programming skills and to help you discover the amazing career opportunities that being a developer allows in life.
Having been a self taught programmer, he understands that there is an overwhelming number of online courses, tutorials and books that are overly verbose and inadequate at teaching proper skills. Most people feel paralyzed and don't know where to start when learning a complex subject matter, or even worse, most people don't have $20,000 to spend on a coding bootcamp. Programming skills should be affordable and open to all. An education material should teach real life skills that are current and they should not waste a student's valuable time. Having learned important lessons from working for Fortune 500 companies, tech startups, to even founding his own business, he is now dedicating 100% of his time to teaching others valuable software development skills in order to take control of their life and work in an exciting industry with infinite possibilities.
Andrei promises you that there are no other courses out there as comprehensive and as well explained. He believes that in order to learn anything of value, you need to start with the foundation and develop the roots of the tree. Only from there will you be able to learn concepts and specific skills(leaves) that connect to the foundation. Learning becomes exponential when structured in this way.
Taking his experience in educational psychology and coding, Andrei's courses will take you on an understanding of complex subjects that you never thought would be possible.
Requirements:
- No experience with data structures or algorithms needed
- Basic understanding of one programming language
- No previous computer science knowledge necessary
- Any engineer, developer, programmer, who wants to improve their interviewing skills
- Anyone interested in improving their whiteboard coding skills
- Anyone who wants to become a better developer
- Any self taught programmer who missed out on a computer science degree
What you'll learn:
- Ace coding interviews given by some of the top tech companies
- Become more confident and prepared for your next coding interview
- Learn, implement, and use different Data Structures
- Learn, implement and use different Algorithms
- Get more interviews
- Professionally handle offers and negotiate raises
- Become a better developer by mastering computer science fundamentals
Watch Online Master the Coding Interview Data Structures Algorithms
# | Title | Duration |
---|---|---|
1 | How To Succeed In This Course | 05:05 |
2 | Join Our Online Classroom! | 04:02 |
3 | ZTM Resources | 04:24 |
4 | Section Overview | 05:40 |
5 | Resume | 05:10 |
6 | Exercise: Resume Walkthrough | 17:06 |
7 | Resume Review | 02:38 |
8 | What If I Don't Have Enough Experience? | 15:04 |
9 | 08:23 | |
10 | Portfolio | 03:24 |
11 | 08:25 | |
12 | Where To Find Jobs? | 06:03 |
13 | When Should You Start Applying? | 03:35 |
14 | Section Summary | 02:18 |
15 | Setting Up Your Environment | 02:54 |
16 | Section Overview | 02:25 |
17 | What Is Good Code? | 06:58 |
18 | Big O and Scalability | 11:09 |
19 | O(n) | 05:40 |
20 | O(1) | 06:11 |
21 | Solution: Big O Calculation | 05:55 |
22 | Solution: Big O Calculation 2 | 02:30 |
23 | Simplifying Big O | 01:51 |
24 | Big O Rule 1 | 04:29 |
25 | Big O Rule 2 | 06:38 |
26 | Big O Rule 3 | 03:14 |
27 | O(n^2) | 07:14 |
28 | Big O Rule 4 | 06:48 |
29 | Big O Cheat Sheet | 03:20 |
30 | What Does This All Mean? | 05:33 |
31 | O(n!) | 01:19 |
32 | 3 Pillars Of Programming | 03:33 |
33 | Space Complexity | 02:23 |
34 | Exercise: Space Complexity | 06:25 |
35 | Exercise: Twitter | 07:14 |
36 | Optional: Javascript Loops | 03:28 |
37 | Section Summary | 04:44 |
38 | Section Overview | 05:05 |
39 | What Are Companies Looking For? | 03:06 |
40 | What We Need For Coding Interviews | 03:28 |
41 | Exercise: Google Interview | 02:32 |
42 | Exercise: Interview Question | 20:34 |
43 | Exercise: Interview Question 2 | 23:00 |
44 | Review Google Interview | 01:31 |
45 | Section Summary | 03:37 |
46 | Section Overview | 01:59 |
47 | What Is A Data Structure? | 05:54 |
48 | How Computers Store Data | 12:34 |
49 | Data Structures In Different Languages | 03:29 |
50 | Operations On Data Structures | 03:06 |
51 | Arrays Introduction | 13:52 |
52 | Static vs Dynamic Arrays | 06:41 |
53 | Optional: Classes In Javascript | 24:53 |
54 | Implementing An Array | 17:20 |
55 | Strings and Arrays | 01:05 |
56 | Exercise: Reverse A String | 01:36 |
57 | Solution: Reverse A String | 10:32 |
58 | Exercise: Merge Sorted Arrays | 00:45 |
59 | Solution: Merge Sorted Arrays | 14:13 |
60 | Arrays Review | 03:29 |
61 | Hash Tables Introduction | 04:11 |
62 | Hash Function | 05:57 |
63 | Hash Collisions | 09:44 |
64 | Hash Tables In Different Languages | 03:31 |
65 | Exercise: Implement A Hash Table | 03:52 |
66 | Solution: Implement A Hash Table | 17:26 |
67 | keys() | 06:12 |
68 | Hash Tables VS Arrays | 02:02 |
69 | Exercise: First Recurring Character | 01:19 |
70 | Solution: First Recurring Character | 16:12 |
71 | Hash Tables Review | 06:10 |
72 | Linked Lists Introduction | 02:27 |
73 | What Is A Linked List? | 04:37 |
74 | Exercise: Imposter Syndrome | 02:57 |
75 | Exercise: Why Linked Lists? | 02:06 |
76 | Solution: Why Linked Lists? | 05:36 |
77 | What Is A Pointer? | 05:46 |
78 | Our First Linked List | 08:39 |
79 | Solution: append() | 05:26 |
80 | Solution: prepend() | 02:29 |
81 | Node Class | 02:42 |
82 | insert() | 05:13 |
83 | Solution: insert() | 13:09 |
84 | Solution: remove() | 05:22 |
85 | Doubly Linked Lists | 03:19 |
86 | Solution: Doubly Linked Lists | 08:51 |
87 | Singly VS Doubly Linked Lists | 02:41 |
88 | Exercise: reverse() | 01:31 |
89 | Solution: reverse() | 07:39 |
90 | Linked Lists Review | 05:09 |
91 | Stacks + Queues Introduction | 02:59 |
92 | Stacks | 03:29 |
93 | Queues | 03:31 |
94 | Exercise: Stacks VS Queues | 03:07 |
95 | Solution: Stacks VS Queues | 03:40 |
96 | Optional: How Javascript Works | 24:13 |
97 | Exercise: Stack Implementation (Linked Lists) | 02:24 |
98 | Solution: Stack Implementation (Linked Lists) | 08:59 |
99 | Exercise: Stack Implementation (Array) | 00:54 |
100 | Solution: Stack Implementation (Array) | 03:57 |
101 | Exercise: Queue Implementation | 01:49 |
102 | Solution: Queue Implementation | 07:51 |
103 | Queues Using Stacks | 02:06 |
104 | Stacks + Queues Review | 02:20 |
105 | Trees Introduction | 06:24 |
106 | Binary Trees | 05:46 |
107 | O(log n) | 07:01 |
108 | Binary Search Trees | 06:13 |
109 | Balanced VS Unbalanced BST | 03:43 |
110 | BST Pros and Cons | 02:27 |
111 | Exercise: Binary Search Tree | 03:58 |
112 | Solution: insert() | 10:11 |
113 | Solution: lookup() | 04:55 |
114 | Extra Exercise: remove() | 02:29 |
115 | Solution: remove() | 07:42 |
116 | AVL Trees + Red Black Trees | 02:57 |
117 | Binary Heaps | 05:37 |
118 | Quick Note on Heaps | 01:09 |
119 | Priority Queue | 05:28 |
120 | Trie | 03:17 |
121 | Tree Review | 00:52 |
122 | Graphs Introduction | 02:29 |
123 | Types Of Graphs | 03:33 |
124 | Exercise: Guess The Graph | 02:46 |
125 | Graph Data | 05:59 |
126 | Exercise: Graph Implementation | 04:07 |
127 | Solution: Graph Implementation | 04:52 |
128 | Graphs Review | 02:05 |
129 | Data Structures Review | 01:53 |
130 | What Else Is Coming Up? | 01:54 |
131 | Introduction to Algorithms | 03:52 |
132 | Recursion Introduction | 05:37 |
133 | Stack Overflow | 06:18 |
134 | Anatomy Of Recursion | 10:28 |
135 | Exercise: Factorial | 03:26 |
136 | Solution: Factorial | 06:21 |
137 | Exercise: Fibonacci | 02:00 |
138 | Solution: Fibonacci | 11:22 |
139 | Recursive VS Iterative | 04:17 |
140 | When To Use Recursion | 04:01 |
141 | Recursion Review | 02:48 |
142 | Sorting Introduction | 07:02 |
143 | The Issue With sort() | 06:52 |
144 | Sorting Algorithms | 03:39 |
145 | Bubble Sort | 03:47 |
146 | Solution: Bubble Sort | 05:07 |
147 | Selection Sort | 02:40 |
148 | Solution: Selection Sort | 02:24 |
149 | Dancing Algorithms | 01:37 |
150 | Insertion Sort | 02:39 |
151 | Solution: Insertion Sort | 02:07 |
152 | Merge Sort and O(n log n) | 09:00 |
153 | Solution: Merge Sort | 04:45 |
154 | Quick Sort | 07:41 |
155 | Which Sort Is Best? | 04:41 |
156 | Radix Sort + Counting Sort | 04:18 |
157 | Exercise: Sorting Interview | 01:28 |
158 | Solution: Sorting Interview | 07:08 |
159 | Sorting In Your Language | 01:28 |
160 | Sorting Review | 02:54 |
161 | Searching + Traversal Introduction | 01:35 |
162 | Linear Search | 03:38 |
163 | Binary Search | 06:06 |
164 | Graph + Tree Traversals | 03:57 |
165 | BFS Introduction | 02:46 |
166 | DFS Introduction | 03:24 |
167 | BFS vs DFS | 03:21 |
168 | Exercise: BFS vs DFS | 00:45 |
169 | Solution: BFS vs DFS | 03:17 |
170 | breadthFirstSearch() | 09:16 |
171 | breadthFirstSearchRecursive() | 05:34 |
172 | PreOrder, InOrder, PostOrder | 05:22 |
173 | depthFirstSearch() | 12:04 |
174 | Graph Traversals | 03:54 |
175 | BFS in Graphs | 02:08 |
176 | DFS in Graphs | 02:51 |
177 | Dijkstra + Bellman-Ford Algorithms | 05:12 |
178 | Searching + Traversal Review | 04:22 |
179 | Dynamic Programming Introduction | 01:52 |
180 | Memoization 1 | 07:48 |
181 | Memoization 2 | 03:57 |
182 | Fibonacci and Dynamic Programming | 05:34 |
183 | Dynamic Programming | 05:48 |
184 | Implementing Dynamic Programming | 09:13 |
185 | Dynamic Programming Review | 04:46 |
186 | Section Overview | 02:39 |
187 | During The Interview | 09:05 |
188 | Tell Me About Yourself | 05:31 |
189 | Why Us? | 05:07 |
190 | Tell Me About A Problem You Have Solved | 05:19 |
191 | What Is Your Biggest Weakness | 02:20 |
192 | Any Questions For Us? | 03:25 |
193 | Secret Weapon | 08:12 |
194 | After The Interview | 05:25 |
195 | Section Summary | 03:57 |
196 | Section Overview | 02:11 |
197 | Handling Rejection | 04:08 |
198 | Negotiation 101 | 09:41 |
199 | Handling An Offer | 09:04 |
200 | Handling Multiple Offers | 07:30 |
201 | Getting A Raise | 07:42 |
202 | Section Summary | 02:10 |
203 | Thank You. | 00:53 |
204 | Coding Problems | 01:26 |
205 | Contributing To Open Source | 14:45 |
206 | Contributing To Open Source 2 | 09:43 |
207 | From JTS: Learn to Learn | 02:00 |
208 | From JTS: Start With Why | 02:44 |
209 | How To Use Leetcode | 02:28 |
210 | AMA - 100,000 Students!! | 38:31 |
Similar courses to Master the Coding Interview Data Structures Algorithms
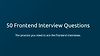
50 Frontend Interview Questionsalgoexpert
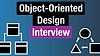
Object-Oriented Design Interviewneetcode.io
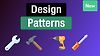
Object-Oriented Design Patternsneetcode.io
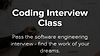
Coding Interview Class (Back To Back SWE)backtobackswe.com
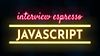
JavaScript Interview Espressointerviewespresso (Aaron Jack)
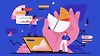
Python Data Analysis & Visualization Masterclassudemy
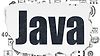
Java Puzzles to Eliminate Code Fearudemy
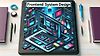
Frontend System DesignLearnersBucket | Prashant Yadav
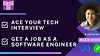