Python & LeetCode | The Ultimate Interview BootCamp
I know LeetCode questions are meant to be difficult, but do not worry! I made it a priority to present each problem in the most simplistic and direct way possible. You will benefit from my painless and easy-to-understand format, as I walk you through each problem, step-by-step. I cover everything from practical application of algorithms, to data structures, to time and space complexity. By the time you complete this course, you will be an expert in all the tricks, techniques, and patterns needed to solve even the most challenging of interview problems. A
Read more about the course
re you ready to supercharge your next technical interview and land that awesome dream job?! I believe we learn best by doing, so throughout this course you will have access to quizzes and challenges to test what you just learned. This format will allow you to apply and internalize new concepts, and not just follow along like a robot! And if you do get stuck, you will benefit from extremely fast and friendly support (both via direct messaging and/or Q&A discussion). We use basic Python in this course, and even if you are new to Python, do not worry! I have included a bonus crash-course in Python at the start of the course. No prior Python experience is required! Course material is regularly refreshed to include all of the newest updates and information, and since you are granted lifetime access upon registering, you can rely on this course to keep your technical interviewing skills on the cutting edge. There is no need to waste your time scouring the internet, frantically trying to piece together ways to solve coding challenges the night before a big, important interview. Invest in yourself, and allow me to show you the easiest ways to ace it!
Watch Online Python & LeetCode | The Ultimate Interview BootCamp
# | Title | Duration |
---|---|---|
1 | Welcome to the Course! | 01:56 |
2 | Development Environment Setup - Windows | 02:51 |
3 | Development Environment Setup - macOS | 04:21 |
4 | Development Environment Setup - Ubuntu | 02:22 |
5 | Course GitHub Repository and How to Run Tests | 06:18 |
6 | Python Crash Course (Optional) | 13:04 |
7 | How to use this Course | 00:15 |
8 | Strings Section Introduction | 00:15 |
9 | Valid Palindrome (LC #125) | 07:31 |
10 | Longest Palindromic Substring (LC #5) | 08:06 |
11 | Longest Substring Without Repeating Characters - Part 1 (LC #3) | 05:08 |
12 | Longest Substring Without Repeating Characters - Part 2 (LC #3) | 03:44 |
13 | Valid Anagram (LC #242) | 05:32 |
14 | Group Anagrams (LC #49) | 05:29 |
15 | Valid Parentheses (LC #20) | 05:29 |
16 | [Now You Try] Backspace String Compare (LC #844) | 05:35 |
17 | Encode and Decode Strings (LC #271) | 07:55 |
18 | Dynamic Programming Section Introduction | 00:28 |
19 | Climbing Stairs (LC #70) | 05:35 |
20 | House Robber - Part 1 (LC #198) | 05:08 |
21 | House Robber - Part 2 (LC #198) | 04:20 |
22 | Jump Game - Part 1 (LC #55) | 03:22 |
23 | Jump Game - Part 2 (LC #55) | 04:41 |
24 | Jump Game - Part 3 (LC #55) | 02:40 |
25 | Longest Increasing Subsequence - Part 1 (LC #300) | 03:53 |
26 | Longest Increasing Subsequence - Part 2 (LC #300) | 04:33 |
27 | Coin Change - Part 1 (LC #322) | 04:59 |
28 | Coin Change - Part 2 (LC #322) | 05:05 |
29 | Unique Paths (LC #62) | 04:58 |
30 | Arrays Section Introduction | 00:31 |
31 | Contains Duplicate (LC #217) | 02:57 |
32 | Product of Array Except Self (LC #238) | 05:58 |
33 | Container With Most Water (LC #11) | 05:13 |
34 | Best Time to Buy and Sell Stock (LC #121) | 04:27 |
35 | Two Sum (LC #1) | 04:02 |
36 | 3Sum - Part 1 (LC #15) | 04:48 |
37 | 3Sum - Part 2 (LC #15) | 05:33 |
38 | Longest Consecutive Sequence (LC #128) | 05:59 |
39 | Maximum Subarray - Part 1 (LC #53) | 02:46 |
40 | Maximum Subarray - Part 2 (LC #53) | 02:59 |
41 | Maximum Product Subarray - Part 1 (LC #152) | 03:10 |
42 | Maximum Product Subarray - Part 2 (LC #152) | 04:03 |
43 | Find Minimum in Rotated Sorted Array - Part 1 (LC #153) | 04:57 |
44 | Find Minimum in Rotated Sorted Array - Part 2 (LC #153) | 04:11 |
45 | Intervals Section Introduction | 00:39 |
46 | Meeting Rooms (LC #252) | 05:04 |
47 | Non-overlapping Intervals - Part 1 (LC #435) | 02:46 |
48 | Non-overlapping Intervals - Part 2 (LC #435) | 04:28 |
49 | Merge Intervals (LC #56) | 04:54 |
50 | Meeting Rooms II - Part 1 (LC #253) | 04:21 |
51 | Meeting Rooms II - Part 2 (LC #253) | 05:25 |
52 | Matrix Section Introduction | 00:28 |
53 | Spiral Matrix - Part 1 (LC #54) | 02:41 |
54 | Spiral Matrix - Part 2 (LC #54) | 05:40 |
55 | Set Matrix Zeroes - Part 1 (LC #73) | 03:49 |
56 | Set Matrix Zeroes - Part 2 (LC #73) | 06:11 |
57 | Word Search (LC #79) | 08:25 |
58 | Design (Practical Data Structures) Section Introduction | 00:13 |
59 | Shuffle an Array (LC #384) | 07:54 |
60 | Moving Average from Data Stream (LC #346) | 05:22 |
61 | Range Sum Query - Immutable - Part 1 (LC #303) | 05:24 |
62 | Range Sum Query - Immutable - Part 2 (LC #303) | 05:02 |
63 | Min Stack - Part 1 (LC #155) | 03:43 |
64 | Min Stack - Part 2 (LC #155) | 05:14 |
65 | Implement Queue using Stacks - Part 1 (LC #232) | 03:51 |
66 | Implement Queue using Stacks - Part 2 (LC #232) | 05:54 |
67 | Insert Delete GetRandom O(1) - Part 1 (LC #380) | 03:23 |
68 | Insert Delete GetRandom O(1) - Part 2 (LC #380) | 07:33 |
69 | Linked List Section Introduction | 00:26 |
70 | Reverse Linked List (LC #206) | 03:22 |
71 | Linked List Cycle (LC #141) | 03:23 |
72 | [Now You Try] Middle of the Linked List (LC #876) | 05:02 |
73 | Remove Nth Node From End of List (LC #19) | 05:07 |
74 | [Now You Try] Remove Linked List Elements (LC #203) | 04:56 |
75 | Merge Two Sorted Lists (LC #21) | 05:00 |
76 | Reorder List (LC #143) | 06:39 |
77 | Copy List with Random Pointer (LC #138) | 06:52 |
78 | Introduction - Trees and Graphs | 02:05 |
79 | Number of Islands (LC #200) | 02:25 |
80 | Invert Binary Tree (LC #226) | 05:13 |
81 | Maximum Depth of Binary Tree (LC #104) | 05:56 |
82 | [Now You Try] Maximum Depth of Binary Tree, *Iterative* (LC #104) | 04:51 |
83 | Binary Tree Level Order Traversal (LC #102) | 12:24 |
84 | Same Tree (LC #100) | 13:15 |
85 | Subtree of Another Tree (LC #572) | 07:46 |
86 | Validate Binary Search Tree (LC #98) | 11:49 |
87 | Lowest Common Ancestor of a Binary Search Tree - Part 1 (LC# 235) | 00:25 |
88 | Lowest Common Ancestor of a Binary Search Tree - Part 2 (LC# 235) | 06:43 |
89 | Kth Smallest Element in a BST (LC #230) | 03:28 |
90 | Verify Preorder Serialization of a Binary Tree - Part 1 (LC #331) | 04:00 |
91 | Verify Preorder Serialization of a Binary Tree - Part 2 (LC #331) | 04:43 |
92 | Implement Trie (Prefix Tree) (LC #208) | 03:51 |
93 | Add and Search Word - Data structure design (LC #211) | 04:12 |
94 | Number of Connected Components in an Undirected Graph (LC #323) | 06:40 |
95 | [Now You Try] Detect Cycle in Directed Graph | 06:07 |
96 | Course Schedule (LC #207) | 04:22 |
97 | [Now You Try] Detect Cycle in an Undirected Graph | 11:32 |
98 | Graph Valid Tree (LC #261) | 06:41 |
99 | Clone Graph (LC #133) | 09:13 |
100 | Heaps Section Introduction | 00:20 |
101 | Kth Largest Element in an Array (LC #215) | 07:07 |
102 | K Closest Points to Origin (LC #973) | 07:06 |
103 | Top K Frequent Elements (LC #347) | 07:22 |
104 | Merge k Sorted Lists (LC #23) | 09:19 |
105 | Congratulations! | 00:20 |
Similar courses to Python & LeetCode | The Ultimate Interview BootCamp
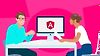
Angular Interview HackingDmytro Mezhenskyi (decodedfrontend.io)
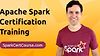
Apache Spark Certification TrainingFlorian Roscheck
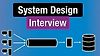
System Design Interviewneetcode.io
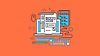
30 Days of Python | Unlock your Python Potentialudemy
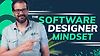
The Software Designer Mindset (COMPLETE)ArjanCodes
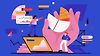
Python Data Analysis & Visualization Masterclassudemy
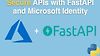
Secure APIs with FastAPI and the Microsoft Identity PlatformTalkpython
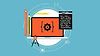
The Ultimate Flask Courseudemy
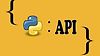