Grokking Dynamic Programming Patterns: Coding Interviews
This course on Dynamic Programming Coding Interview Algorithms will teach you the advanced algorithms and data structures needed for coding interviews and technical interviews. You’ll learn how to solve dynamic programming questions, and you’ll master the fundamentals of data structures and algorithms. You’ll also get an in-depth understanding of Grokking Dynamic Programming Interview Patterns for Technical Interviews, and you’ll learn the skills needed to solve the toughest coding interview questions. Finally, you’ll get hands-on experience with Java Dynamic Programming questions and Algorithms for Coding Interviews, and you’ll Master Dynamic Programming Coding Interview Algorithms and ace your next job interview. This course will teach you the fundamentals of dynamic programming and how to use them to solve complex coding interview questions quickly and confidently. You will learn the fundamentals of data structures and algorithms, as well as how to apply them to coding interview questions. You will also learn to use Java and dynamic programming techniques to solve dynamic programming questions related to Google, LeetCode, and other technical interviews. You will also learn the best practices for mastering the coding interview data structures and algorithms, as well as how to review and apply them in the real world.
Read more about the course
If you often struggle with dynamic programming problems despite your understanding of data structures and algorithms, this course is designed to bridge that gap. It provides a comprehensive understanding of critical Dynamic Programming concepts, empowering you to excel in competitive coding and interviews.
In addition to the mentioned problems, the "Dynamic Programming Algorithms Coding Interviews" course covers several more essential dynamic programming problems. Through detailed explanations, code implementations, and step-by-step walkthroughs, you'll gain a deep understanding of each problem's solution.
Watch Online Grokking Dynamic Programming Patterns: Coding Interviews
# | Title | Duration |
---|---|---|
1 | Introduction | 00:43 |
2 | 0/1 Knapsack Problem - Top Down | 24:52 |
3 | 0/1 Knapsack Problem - Bottom UP (2D Tabulation) | 24:28 |
4 | 0/1 Knapsack Problem - Bottom UP (1D Tabulation) | 21:28 |
5 | Target Sum - Top Down | 29:14 |
6 | Target Sum - Bottom UP | 47:43 |
7 | Count of Subset Sum - Top Down | 32:54 |
8 | Count of Subset Sum - Bottom Up [2D Tabulation] | 20:53 |
9 | Count of Subset Sum - Bottom UP [1D Tabulation] | 26:53 |
10 | Minimum Sum Partition - Top Down | 28:32 |
11 | Minimum Sum Partition - Bottom UP [1D Tabulation] | 20:24 |
12 | Minimum Number of Refuelling Stops - Top Down | 33:39 |
13 | Minimum Number of Refuelling Stops - Bottom UP [1D Tabulation] | 25:28 |
14 | Partition Equal Subset Sum - Top Down | 20:41 |
15 | Partition Equal Subset Sum - Bottom UP [1D Tabulation] | 20:03 |
16 | Count Square Submatrices with All Ones - Top Down | 23:46 |
17 | Count Square Submatrices with All Ones - Bottom Up | 16:31 |
18 | Unbounded Knapsack - Top Down | 27:33 |
19 | Unbounded Knapsack - Bottom UP [2D Tabulation] | 17:35 |
20 | Unbounded Knapsack - Bottom UP [1D Tabulation] | 13:03 |
21 | Maximum Ribbon Cut - Top Down | 17:48 |
22 | Maximum Ribbon Cut - Bottom UP [2D Tabulation] | 18:13 |
23 | Rod Cutting - Top Down | 20:09 |
24 | Rod Cutting - Bottom UP [2D Tabulation] | 15:57 |
25 | Coin Change - Top down | 15:42 |
26 | Coin Change - Bottom UP [1D Tabulation] | 28:44 |
27 | Coin Change II - Top Down | 17:04 |
28 | Coin Change II - Bottom UP [2D Tabulation] | 15:14 |
29 | Coin Change II - Bottom UP [1D Tabulation] | 15:45 |
30 | Fibonacci Number - Top Down | 24:25 |
31 | Fibonacci Number - Bottom UP [1D Tabulation] | 08:52 |
32 | Fibonacci Number - Bottom UP [Constant Space] | 08:47 |
33 | Climbing Stairs - Top Down | 16:22 |
34 | Climbing Stairs - Bottom UP | 22:21 |
35 | Decode Ways - Top Down | 24:42 |
36 | Decode Ways - Bottom UP [1D Tabulation] | 28:58 |
37 | Decode Ways - Bottom UP [Space Optimized] | 17:51 |
38 | House Robber - Top Down | 17:51 |
39 | House Robber - Bottom UP | 20:47 |
40 | Number Factors - Top Down | 15:57 |
41 | Number Factors - Bottom UP | 10:42 |
42 | Count Ways to Score in a Game - Top Down | 16:37 |
43 | Count Ways to Score in a Game - Bottom UP | 08:01 |
44 | Unique Paths to Goal - Top Down | 19:48 |
45 | Unique Paths to Goal - Bottom UP | 18:01 |
46 | Nth Tribonacci Number - Top Down | 20:11 |
47 | Nth Tribonacci Number - Bottom UP | 12:35 |
48 | The Catalan Numbers - Top Down | 22:17 |
49 | The Catalan Numbers - Bottom UP | 12:04 |
50 | Minimum Jumps to Reach the End - Top Down | 15:56 |
51 | Minimum Jumps to Reach the End - Bottom UP | 13:44 |
52 | Minimum Jumps With Fee - Top Down | 23:31 |
53 | Minimum Jumps With Fee - Bottom UP | 17:50 |
54 | Matrix Chain Multiplication - Top Down | 42:05 |
55 | Matrix Chain Multiplication - Bottom UP | 31:38 |
56 | Longest Common Substring - Top Down | 22:30 |
57 | Longest Common Substring - Bottom UP | 13:29 |
58 | Longest Common Subsequence - Top Down | 22:53 |
59 | Longest Common Subsequence - Bottom UP [2D Tabulation] | 17:25 |
60 | Shortest Common Supersequence - Top Down | 21:14 |
61 | Shortest Common Supersequence - Bottom UP | 19:20 |
62 | Minimum Number of Deletions and Insertions - Top Down | 27:33 |
63 | Minimum Number of Deletions and Insertions - Bottom UP | 15:10 |
64 | Edit Distance -- Top Down | 35:08 |
65 | Edit Distance -- Bottom UP [2D Tabulation] | 27:55 |
66 | Longest Repeating Subsequence - Top Down | 21:47 |
67 | Longest Repeating Subsequence - Bottom UP | 09:11 |
68 | Distinct Subsequence Pattern Matching - Top Down | 15:39 |
69 | Distinct Subsequence Pattern Matching - Bottom UP | 14:22 |
70 | Interleaving String - Top Down | 27:50 |
71 | Interleaving String - Bottom UP | 19:29 |
72 | Word Break - Bottom UP [1D Tabulation] | 16:24 |
73 | Word Break II - Top Down | 21:02 |
74 | Word Break II - Bottom UP | 17:26 |
75 | Longest Increasing Subsequence - Top Down | 20:06 |
76 | Longest Increasing Subsequence - Bottom UP [1D Tabulation] | 25:00 |
77 | Number of Longest Increasing Subsequence - Bottom UP | 19:52 |
78 | Minimum Deletions to Make a String Sorted - Top Down | 18:37 |
79 | Minimum Deletions to Make a String Sorted - Bottom UP | 16:46 |
80 | Maximum Sum Increasing Subsequence - Top Down | 16:01 |
81 | Maximum Sum Increasing Subsequence - Bottom UP | 07:34 |
82 | Longest Bitonic Subsequence - Bottom UP | 20:07 |
83 | Longest Alternating Subsequence - Bottom UP | 13:27 |
84 | Building Bridges - Bottom UP | 22:46 |
85 | Solution (i): Longest Palindromic Subsequence - Top Down | 22:14 |
86 | Solution (ii): Longest Palindromic Subsequence - Bottom UP [2D Tabulation] | 23:17 |
87 | Minimum Deletions to Make a String Palindrome - Top Down | 15:18 |
88 | Minimum Deletions to Make a String Palindrome - Bottom UP [2D] | 13:42 |
89 | Longest Palindromic Substring - Top Down | 22:52 |
90 | Longest Palindromic Substring - Bottom UP | 21:25 |
91 | Count of Palindromic Substrings - Top Down | 28:04 |
92 | Count of Palindromic Substrings - Bottom UP | 15:37 |
93 | Palindrome Partitioning - Top Down | 19:27 |
94 | Palindrome Partitioning - Bottom UP | 24:41 |
95 | Regular Expression Matching [2D Tabulation] | 32:47 |
96 | Range Sum Query 2D - Immutable [2D Tabulation] | 15:54 |
Similar courses to Grokking Dynamic Programming Patterns: Coding Interviews
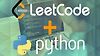
Python & LeetCode | The Ultimate Interview BootCampkaeducation.com
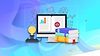
Master the Coding Interview: Big Tech (FAANG) Interviewszerotomastery.io
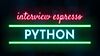
Python Interview Espressointerviewespresso (Aaron Jack)
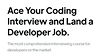
Hack the Tech Interview (The Pro Package)Randall Kanna
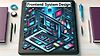
Frontend System DesignLearnersBucket | Prashant Yadav
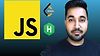
LeetCode & JavaScript Complete Course for Web Developer 2022udemy
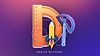
The Ultimate Design Patterns: Part 1codewithmosh (Mosh Hamedani)
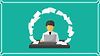
JavaScript & LeetCode | The Ultimate Interview Bootcampudemy
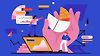