Ultimate Java Part 3: Advanced Topics
6h 28m 19s
English
Paid
Want to level-up your Java skills and reach the advanced level? Want to become an in-demand Java developer for exciting software companies? This course is exactly what you need. I’ll help you expand your programming skills and equip you with techniques that you can immediately put into practice.
Read more about the course
You’ll be able to:
- Write Java code with confidence
- Master advanced Java constructs
- Stay up-to-date with the modern Java features
- Become a better Java developer
What You'l Learn...
- Exception handling
- Generics
- Collections framework
- Lambda expressions
- Functional interfaces
- Streams
- Multi-threading
- Asynchronous programming
- And much, much more...
Watch Online Ultimate Java Part 3: Advanced Topics
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | 1- Introduction | 00:39 |
2 | 1- Introduction | 00:43 |
3 | 2- What are Exceptions | 03:46 |
4 | 3- Types of Exceptions | 03:41 |
5 | 4- Exceptions Hierarchy | 02:06 |
6 | 5- Catching Exceptions | 03:52 |
7 | 6- Catching Multiple Types of Exceptions | 04:29 |
8 | 7- The finally Block | 04:11 |
9 | 8- The try-with-resources Statement | 02:27 |
10 | 9- Throwing Exceptions | 04:42 |
11 | 10- Re-throwing Exceptions | 03:08 |
12 | 11- Custom Exceptions | 04:19 |
13 | 12- Chaining Exceptions | 04:57 |
14 | 13- Summary | 00:56 |
15 | 1- Introduction | 00:28 |
16 | 2- The Need for Generics | 03:33 |
17 | 3- A Poor Solution | 03:23 |
18 | 4- Generic Classes | 04:28 |
19 | 5- Generics and Primitive Types | 02:25 |
20 | 6- Constraints | 03:26 |
21 | 7- Type Erasure | 04:05 |
22 | 8- Comparable Interface | 05:39 |
23 | 9- Generic Methods | 04:49 |
24 | 10- Multiple Type Parameters | 02:33 |
25 | 11- Generic Classes and Inheritance | 04:18 |
26 | 12- Wildcards | 05:28 |
27 | 13- Summary | 00:56 |
28 | 1- Introduction | 00:35 |
29 | 2- Overview of Collections Framework | 03:09 |
30 | 3- The Need for Iterables | 03:55 |
31 | 4- The Iterable Interface | 05:25 |
32 | 5- The Iterator Interface | 05:27 |
33 | 6- The Collection Interface | 09:36 |
34 | 7- The List Interface | 03:52 |
35 | 8- The Comparable Interface | 04:29 |
36 | 9- The Comparator Interface | 03:52 |
37 | 10- The Queue Interface | 04:48 |
38 | 11- The Set Interface | 05:34 |
39 | 12- Hash Tables | 03:45 |
40 | 13- The Map Interface | 06:27 |
41 | 14- Summary | 00:45 |
42 | 1- Introduction | 00:48 |
43 | 2- Functional Interfaces | 03:52 |
44 | 3- Anonymous Inner Classes | 01:24 |
45 | 4- Lambda Expressions | 03:44 |
46 | 5- Variable Capture | 01:57 |
47 | 6- Method References | 03:47 |
48 | 7- Built-in Functional Interfaces | 01:43 |
49 | 8- The Consumer Interface | 04:07 |
50 | 9- Chaining Consumer | 04:29 |
51 | 10- The Supplier Interface | 02:31 |
52 | 11- The Function Interface | 03:01 |
53 | 12- Composing Functions | 04:20 |
54 | 13- The Predicate Interface | 02:03 |
55 | 14- Combining Predicates | 02:11 |
56 | 15- The BinaryOperator Interface | 03:16 |
57 | 16- The UnaryOperator Interface | 01:14 |
58 | 17- Summary | 00:41 |
59 | 1- Introduction | 00:42 |
60 | 2- Imperative vs Functional Programming | 06:25 |
61 | 3- Creating a Stream | 05:02 |
62 | 4- Mapping Elements | 05:25 |
63 | 5- Filtering Elements | 03:00 |
64 | 6- Slicing Streams | 04:22 |
65 | 7- Sorting Streams | 05:13 |
66 | 8- Getting Unique Elements | 02:10 |
67 | 9- Peeking Elements | 03:20 |
68 | 10- Simple Reducers | 03:38 |
69 | 11- Reducing a Stream | 04:13 |
70 | 12- Collectors | 06:04 |
71 | 13- Grouping Elements | 04:52 |
72 | 14- Partitioning Elements | 02:11 |
73 | 15- Primitive Type Streams | 01:26 |
74 | 16- Summary | 00:40 |
75 | 1- Introduction | 00:56 |
76 | 2- Processes and Threads | 03:08 |
77 | 3- Starting a Thread | 03:15 |
78 | 4- Pausing a Thread | 02:36 |
79 | 5- Joining a Thread | 02:28 |
80 | 6- Interrupting a Thread | 02:55 |
81 | 7- Concurrency Issues | 02:09 |
82 | 8- Race Conditions | 05:54 |
83 | 9- Strategies for Thread Safety | 02:51 |
84 | 10- Confinement | 03:41 |
85 | 11- Locks | 03:18 |
86 | 12- The synchronized Keyword | 05:14 |
87 | 13- The volatile Keyword | 06:34 |
88 | 14- Thread Signalling with wait() and notify() | 03:36 |
89 | 15- Atomic Objects | 03:26 |
90 | 16- Adders | 02:10 |
91 | 17- Synchronized Collections | 03:25 |
92 | 18- Concurrent Collections | 02:38 |
93 | 19- Summary | 01:19 |
94 | 1- Introduction | 00:39 |
95 | 2- Thread Pools | 01:47 |
96 | 3- Executors | 06:51 |
97 | 4- Callables and Futures | 05:01 |
98 | 5- Asynchronous Programming | 01:51 |
99 | 6- Completable Futures | 01:39 |
100 | 7- Creating a Completable Future | 03:43 |
101 | 8- Implementing an Asynchronous API | 04:19 |
102 | 9- Running Code on Completion | 04:05 |
103 | 10- Handling Exceptions | 04:16 |
104 | 11- Transforming a Completable Future | 04:35 |
105 | 12- Composing Completable Futures | 06:09 |
106 | 13- Combining Completable Futures | 04:33 |
107 | 14- Waiting for Many Tasks to Complete | 02:49 |
108 | 15- Waiting for the First Task | 02:14 |
109 | 16- Handling timeouts | 02:25 |
110 | 17- Project- Best Price Finder | 01:19 |
111 | 18- Solution- Getting a Quote | 04:16 |
112 | 19- Solution- Getting Many Quotes | 04:50 |
113 | 20- Solution- Random Delays | 04:28 |
Similar courses to Ultimate Java Part 3: Advanced Topics
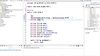
Building Your First App with Spring Boot and Angularpluralsight
Category: Angular, Spring Boot, Java
Duration 2 hours 22 minutes 15 seconds
Course
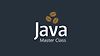
Java Master Classamigoscode (Nelson Djalo)
Category: Java
Duration 24 hours 40 minutes 37 seconds
Course
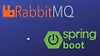
RabbitMQ : Messaging with Java, Spring Boot And Spring MVCudemy
Category: Spring Boot, Java, Spring Cloud, Spring MVC
Duration 4 hours 3 minutes 11 seconds
Course
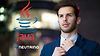
Java Foundations: The Complete Course with Java 21 Updatesudemy
Category: Java
Duration 88 hours 37 minutes 5 seconds
Course
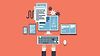
Hibernate and Java Persistence API (JPA) Fundamentalsudemy
Category: Java, Hibernate ORM
Duration 7 hours 24 minutes 19 seconds
Course
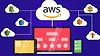
AWS Serverless REST APIs for Java Developers. CI/CD includedudemy
Category: AWS, Java
Duration 14 hours 34 minutes 16 seconds
Course
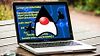
Java Programming Masterclass covering Java 11 & Java 17udemy
Category: Java
Duration 80 hours 13 minutes 14 seconds
Course
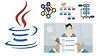
Java Data Structures and Algorithms Masterclassudemy
Category: Java
Duration 44 hours 58 minutes 57 seconds
Course
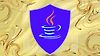
Java Programming Bootcamp: Zero to Masteryzerotomastery.io
Category: Java
Duration 9 hours 15 minutes 36 seconds
Course
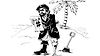
Data Structures in Javajavaspecialists.eu
Category: Java
Duration 8 hours 3 minutes 54 seconds
Course