Rust: The Complete Developer's Guide
Welcome to the most comprehensive and practical course for learning Rust from scratch! Rust is changing the approach to systems programming with its focus on memory safety, parallelism, and high performance. However, its unique concepts and syntax can be a challenging task for beginners. This course offers a structured and clear path to mastering Rust.
Read more about the course
What sets this course apart?
We focus on a strong understanding of Rust's key concepts. No unnecessary information or skipped steps—only the important knowledge that will help you successfully use Rust in any project.
The course covers the most challenging aspects of Rust:
- Ownership model in Rust? Explained in detail!
- Lifetimes and borrowing? It's all here!
- Traits and generics? You'll learn how to write flexible code using them.
The course is for those who want to truly understand Rust, not just copy code.
Whether you code in JavaScript, Python, or another language, you're in for a welcoming introduction to Rust's unique principles.
For seven consecutive years, Rust has been the "most loved programming language" according to the Stack Overflow developer survey. And it's not just a trend—major companies like Microsoft, Google, and Amazon are actively adopting Rust for critical systems. By mastering Rust, you're not only learning a language but also securing your career for the future.
What you will learn in this course:
- Fundamentals of Rust's type system and how it ensures memory safety
- Mastering pattern matching and destructuring for elegant and expressive code
- Using error handling with Result and Option types
- Organizing projects with Rust's module system
- Implementing standard data structures and algorithms in Rust style
- Managing dependencies and building projects using Cargo
- In-depth understanding of Rust syntax and core concepts
- Ability to write safe, efficient, and idiomatic code
- Readiness for real-world projects in Rust and contributing to the ecosystem
- Code optimization for performance and memory efficiency
How this course works:
This is not just another "follow-along" course. We have organized the learning process so you can truly master Rust:
- Introduction to Concepts: Clear and concise explanations of each Rust feature
- Live Programming: Implementing concepts in real-time with thought process explanations
- Assignments: Test your understanding with carefully designed exercises
- Project Work: Apply skills in practice by creating increasingly complex projects
- Best Practices: Learn idiomatic Rust and standard code patterns
This is the course I wish I had when learning Rust. A course where the focus is on the most challenging parts, clear explanations, and discussion of the pros and cons of various solutions. Sign up and join us on the path to mastering Rust!
Watch Online Rust: The Complete Developer's Guide
# | Title | Duration |
---|---|---|
1 | 1 - Introduction | 01:02 |
2 | 2 - Rust Installation | 02:08 |
3 | 4 - Creating and Running Rust Projects | 03:22 |
4 | 7 - Representing Data with Structs | 08:51 |
5 | 8 - Adding Functionality to Structs | 06:19 |
6 | 9 - Arrays vs Vectors | 06:12 |
7 | 12 - Implicit Returns | 03:31 |
8 | 13 - Installing External Crates | 05:50 |
9 | 14 - Using Code from Crates | 07:56 |
10 | 15 - Shuffling a Slice | 04:17 |
11 | 17 - Project Review | 04:02 |
12 | 18 - Project Overview | 03:10 |
13 | 19 - Defining Structs | 03:38 |
14 | 20 - Adding Inherent Implementations | 04:54 |
15 | 21 - A Mysterious Error | 04:19 |
16 | 22 - Unexpected Value Updates | 09:20 |
17 | 23 - The Goal of Ownership and Borrowing | 06:06 |
18 | 24 - The Basics of Ownership | 06:18 |
19 | 25 - Visualizing Ownership and Moves | 12:31 |
20 | 26 - Exercise Overview | 01:16 |
21 | 27 - Exercise Solution | 01:39 |
22 | 28 - Another Quick Exercise | 01:49 |
23 | 29 - A Quick Exercise Solution | 03:41 |
24 | 30 - Writing Useful Code with Ownership | 04:39 |
25 | 31 - Introducing the Borrow System | 03:47 |
26 | 32 - Immutable References | 08:54 |
27 | 33 - Exercise On References | 01:23 |
28 | 34 - References Exercise Solution | 02:04 |
29 | 35 - Mutable References | 07:50 |
30 | 36 - Exercise on Mutable Refs | 01:06 |
31 | 37 - Solution on Mutable Refs | 06:23 |
32 | 38 - Copyable Values | 04:08 |
33 | 39 - Basics of Lifetimes | 07:27 |
34 | 40 - Deciding on Argument Types | 04:52 |
35 | 41 - Back to the Bank Impl | 02:29 |
36 | 42 - Implementing Deposits and Withdrawals | 06:24 |
37 | 43 - Accounts and Bank Implementation | 06:58 |
38 | 44 - Project Wrapup | 01:45 |
39 | 45 - Project Overview | 01:26 |
40 | 46 - Defining Enums | 06:08 |
41 | 47 - Declaring Enum Values | 01:21 |
42 | 48 - Adding Implementations to Enums | 08:28 |
43 | 49 - Pattern Matching with Enums | 03:45 |
44 | 50 - When to Use Structs vs Enums | 03:00 |
45 | 51 - Adding Catalog Items | 03:49 |
46 | 52 - Unlabeled Fields | 05:53 |
47 | 53 - The Option Enum | 05:33 |
48 | 54 - Option From Another Perspective | 13:10 |
49 | 55 - Replacing Our Custom Enum with Option | 02:51 |
50 | 56 - Other Ways of Handling Options | 09:37 |
51 | 57 - Excercise Overview | 01:39 |
52 | 58 - Exercise Solution | 03:15 |
53 | 59 - Modules Overview | 06:05 |
54 | 60 - Rules of Modules | 02:55 |
55 | 61 - Refactoring with Multiple Modules | 08:20 |
56 | 62 - Project Overview | 01:35 |
57 | 63 - Reading a File | 02:18 |
58 | 64 - The Result Enum | 05:14 |
59 | 65 - The Result Enum in Action | 06:42 |
60 | 66 - Types of Errors | 03:10 |
61 | 67 - Matching on Results | 03:05 |
62 | 68 - Empty OK Variants | 07:36 |
63 | 69 - Exercise Around the Result Enum | 01:30 |
64 | 70 - Exercise Solution | 02:51 |
65 | 71 - Using a Result When Reading Files | 03:55 |
66 | 72 - Tricky Strings | 03:47 |
67 | 73 - The Stack and Heap | 05:21 |
68 | 74 - Strings String Refs and String Slices | 06:27 |
69 | 75 - When to Use Which String | 10:53 |
70 | 76 - Finding Error Logs | 07:49 |
71 | 77 - Understanding the Issue | 11:15 |
72 | 78 - Fixing Errors Around String Slices | 05:07 |
73 | 79 - Writing Data to a File | 04:14 |
74 | 80 - Alternatives to Nested Matches | 06:33 |
75 | 81 - The Try Operator | 10:04 |
76 | 82 - When to Use Each Technique | 07:28 |
77 | 83 - Project Overview | 02:02 |
78 | 84 - Basics of Iterators | 07:12 |
79 | 85 - Using For Loops with Iterators | 04:36 |
80 | 86 - Iterator Consumers | 04:33 |
81 | 87 - Iterator Adaptors | 04:47 |
82 | 88 - Vector Slices | 09:28 |
83 | 89 - Reminder on Ownership and Borrowing | 04:47 |
84 | 90 - Iterators with Mutable Refs | 04:39 |
85 | 91 - Mutable Vector Slices | 01:15 |
86 | 92 - Collecting Elements from an Iterator | 06:00 |
87 | 93 - How Collect Works | 08:56 |
88 | 94 - Moving Ownership With IntoIter | 06:45 |
89 | 95 - Inner Maps | 04:13 |
90 | 96 - Reminder on Lifetimes | 07:07 |
91 | 97 - Iterators Wrapup | 05:46 |
92 | 98 - Collect Excercise | 01:06 |
93 | 99 - Exercise Solution | 00:55 |
94 | 100 - The Filter Method | 00:46 |
95 | 101 - Filter Solution | 01:07 |
96 | 102 - Lifetime Annotations | 02:59 |
97 | 103 - A Missing Annotation | 04:52 |
98 | 104 - A Review of Borrowing Rules | 05:24 |
99 | 105 - What Lifetime Annotation Are All About | 05:48 |
100 | 106 - Common Questions Around Lifetimes | 06:21 |
101 | 107 - Lifetime Elision | 07:34 |
102 | 108 - Common Lifetimes | 05:44 |
103 | 109 - Project Setup | 04:31 |
104 | 110 - Issues with Number Types | 05:16 |
105 | 111 - The Basics of Generics | 07:18 |
106 | 112 - Trait Bounds | 04:13 |
107 | 113 - Multiple Generic Types | 02:28 |
108 | 114 - Super Solve Flexibility | 01:45 |
109 | 115 - App Overview | 03:46 |
110 | 116 - Building the Basket | 06:01 |
111 | 117 - Generic Structs | 05:20 |
112 | 118 - More on Generic Structs | 05:47 |
113 | 119 - Implementing a trait | 05:30 |
114 | 120 - Generic Trait Bounds | 02:34 |
Similar courses to Rust: The Complete Developer's Guide
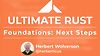
Ultimate Rust: Foundations - Next Stepsardanlabs.com
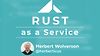
Rust as a Serviceardanlabs.com
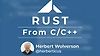
Rust from C/C++ardanlabs.com
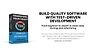
Transform Your Craft with TDD: Master clean code and testingDaniel Moka
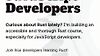
Rust for JavaScript DevelopersSidhartha Chatterjee
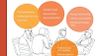
Learn Rust in a Month of LunchesDave MacLeod
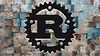
Rust Programming: The Complete Developer's Guidezerotomastery.io
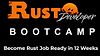
Rust Developer Bootcampletsgetrusty.com
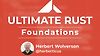
Ultimate Rust Foundationsardanlabs.com
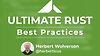