OAuth 2.0 in Spring Boot Applications
This video course is for beginner Java developers who are interested in learning how to secure OAuth 2.0 Resources in Spring Security 5. The course covers only the new OAuth 2.0 stack in Spring Security 5. This is a step-by-step video course that explains how to use OAuth 2 from the very beginning. If you do not have experience with OAuth and would like to learn how to use it in Spring Boot Web Applications, then this video course is for you.
Read more about the course
You will learn how to:
Perform each OAuth 2 authorization flow,
Authorization Code,
PKCE-enhanced authorization code,
Client credentials,
Password credentials.
Startup and configure the Keycloak server,
Configure OAuth 2 Resource Server,
Startup multiple Resource Servers on random port numbers,
Configure Spring Cloud API Gateway,
Configure and use Eureka Registry and Discovery Service,
Build a simple Spring MVC Web Application that fetches data from a protected Resource Server running behind Spring Cloud API Gateway.
Implement a simple JavaScript application that uses PKCE-Enhanced authorization code to acquire JWT access tokens and communicate with protected Resource Server,
Learn how to refresh an expired JWT Access token,
Learn to implement Scope-base access control,
Learn how to implement Role-based access control,
OAuth social login with Facebook, Google, and Okta accounts,
Implement Keycloak Remote User Authentication(User Storage SPI)
Watch Online OAuth 2.0 in Spring Boot Applications
# | Title | Duration |
---|---|---|
1 | Introduction | 04:25 |
2 | Introduction to OAuth 2 | 07:20 |
3 | OAuth 2.0 Roles | 03:17 |
4 | Current State of OAuth 2.0 in Spring Security 5 | 04:19 |
5 | OAuth 2.0 Client Types | 05:01 |
6 | OAuth Access Token | 07:46 |
7 | OAuth2 and OpenID Connect (OIDC) | 03:41 |
8 | Introduction | 04:27 |
9 | Authorization Code. Introduction. | 09:46 |
10 | Authorization Code Demo. Initial Request. | 07:37 |
11 | Authorization Code Demo. Exchange code for Access token. | 04:22 |
12 | PKCE-enhanced Authorization Code | 05:38 |
13 | Generating PKCE Code Verifier | 01:14 |
14 | Generating PKCE Code Challenge | 01:30 |
15 | PKCE Demo. Requesting Authorization Code | 07:21 |
16 | PKCE Demo. Exchanging Code for Access Token | 04:44 |
17 | Client Credentials | 01:42 |
18 | Client Credentials Grant Type Demo | 02:39 |
19 | The Password Credentials Flow | 02:14 |
20 | The Password Credentials Flow: Demo | 03:02 |
21 | Introduction | 05:05 |
22 | Requesting Refresh Token that never expires | 02:26 |
23 | Refreshing Access Token. Demonstration. | 03:14 |
24 | Introduction | 04:08 |
25 | Starting up Standalone Authorization Server Keycloak | 02:03 |
26 | Starting and Stopping Keycloak Server | 03:41 |
27 | Creating an Initial Admin User | 01:21 |
28 | Creating a new Realm | 03:04 |
29 | Creating a new user | 02:56 |
30 | Creating a new OAuth client application | 04:53 |
31 | Configuring Client Application Secrets | 01:06 |
32 | Requesting Access Token and Refresh Token | 03:56 |
33 | Enable/Disable OAuth 2.0 Authorization Flow | 02:16 |
34 | OAuth 2.0 Client Scopes | 04:56 |
35 | Introduction | 03:01 |
36 | Creating a new project | 04:05 |
37 | Import project into Spring Tool Suite IDE | 00:57 |
38 | Starting Resource Server on different port number | 01:36 |
39 | Creating a Rest Controller Class | 04:50 |
40 | Access Token Validation URIs | 02:58 |
41 | Accessing endpoints with an Access Token | 03:53 |
42 | Accessing Principal and JWT Claims | 04:17 |
43 | Demo - Accessing JWT Claims | 03:38 |
44 | Introduction | 09:15 |
45 | Create WebSecurityConfigurerAdapter and enable Web Security | 01:58 |
46 | Override the default HttpSecurity configuration | 02:46 |
47 | Configure Scope-based access control | 02:25 |
48 | Demo - without using proper Scope | 03:14 |
49 | Demo - using proper Scope | 01:06 |
50 | Introduction | 05:35 |
51 | Creating User Role | 01:15 |
52 | Securing Endpoints to a Specific Role | 02:22 |
53 | Creating Role Converter class | 02:09 |
54 | Decoding JWT to find user roles | 02:12 |
55 | Converting Roles into GrantedAuthority objects | 01:32 |
56 | Register JwtAuthenticationConverter with HttpSecurity | 01:08 |
57 | Trying how it works | 03:40 |
58 | Introduction | 04:56 |
59 | Enable Method Level Security | 02:20 |
60 | @Secured annotation example | 05:55 |
61 | @PreAuthorized annotation | 04:00 |
62 | Reading UserId from JWT Access Token | 05:39 |
63 | Trying how the @PreAuthorized annotation works | 03:01 |
64 | Creating getUser() to be used with @PostAuthorize | 03:32 |
65 | @PostAuthorized annotation | 03:09 |
66 | Trying how to the @PostAuthorized annotation works | 04:07 |
67 | Introduction | 06:02 |
68 | Creating API Gateway Project | 04:18 |
69 | Import API Gateway to Spring Tool Suite | 00:37 |
70 | Configuring API Gateway Routes | 06:27 |
71 | Trying how it works | 03:46 |
72 | Albums & Photos Resource Servers | 03:45 |
73 | Routing to multiple Resource Servers | 03:05 |
74 | Trying how it works | 04:10 |
75 | Introduction | 04:40 |
76 | Creating Eureka Discovery Service Project | 03:24 |
77 | Configuring Eureka project | 03:50 |
78 | Eureka Client Dependency | 04:14 |
79 | @EnableDiscoveryClient and configuration properties | 02:46 |
80 | Trying how it works | 00:51 |
81 | Exercise | 01:10 |
82 | Solution overview | 04:47 |
83 | Load Balancing - Introduction | 02:08 |
84 | Starting Resource Servers on Random IP Address | 04:13 |
85 | Eureka and Resource Server Instance Id | 07:01 |
86 | Configuring API Gateway as Eureka Client | 04:38 |
87 | Configure API Gateway Routes | 03:25 |
88 | Return the running port number | 02:18 |
89 | Trying how it works | 03:47 |
90 | OAuth 2.0 in MVC Web App - Introduction | 01:43 |
91 | Creating a new Spring Boot Project | 02:29 |
92 | Creating Controller class | 04:13 |
93 | Returning list of albums | 04:55 |
94 | Displaying Albums in HTML Page | 04:22 |
95 | Adding OAuth2 Client Dependency | 01:20 |
96 | Configuring OAuth2 Client properties | 05:31 |
97 | Configuring OAuth2 Provider properties | 02:40 |
98 | Reading ID Token | 06:26 |
99 | Reading JWT Access Token | 06:12 |
100 | HTTP Request to an Internal Resource Server | 07:40 |
101 | Trying how it works | 02:09 |
102 | A different approach to adding access token | 01:28 |
103 | Adding WebClient Dependency | 01:16 |
104 | Creating a WebClient Bean | 04:53 |
105 | Using WebClient in a Controller class | 02:41 |
106 | Trying how it works | 01:42 |
107 | Introduction | 01:55 |
108 | Creating a new project | 02:16 |
109 | Protected Resource Controller class | 04:04 |
110 | Protected Resource HTML Page | 03:05 |
111 | Creating public page | 03:10 |
112 | Configure HTTP Security | 03:05 |
113 | OAuth 2 Client and Provider configurations | 04:54 |
114 | Facebook: Client Id and Client Secret | 02:07 |
115 | Trying how Facebook login works | 01:31 |
116 | Google Client Id and Client Secret | 07:45 |
117 | Trying how Google sign-in works | 01:06 |
118 | Register a new Okta app | 03:51 |
119 | Configure Okta properties | 03:41 |
120 | Trying how sign-in with Okta works | 01:00 |
121 | Configure logout functionality | 03:44 |
122 | Configure the Logout link | 01:13 |
123 | Trying how logout link works | 02:45 |
124 | OpenID Connect End Session Endpoint | 03:52 |
125 | Implementing OidcClientInitiatedLogoutSuccessHandler | 02:56 |
126 | Trying how Logout from Okta works | 01:08 |
127 | Introduction | 02:09 |
128 | Creating a new Public client in Keycloak | 05:33 |
129 | Creating a new Spring Boot client application | 01:26 |
130 | Creating Index.html | 01:46 |
131 | Import jQuery | 01:09 |
132 | Generating Random State value | 04:12 |
133 | Generating Code Verifier value | 02:29 |
134 | Generating Code Challenge value | 03:27 |
135 | Requesting PKCE-Enhanced Authorization Code | 05:11 |
136 | Creating Auth Code Reader HTML Page | 01:00 |
137 | Reading authorization code from the Redirect URI | 03:43 |
138 | Validating "state" request parameter | 02:17 |
139 | Exchange Code for Access Token | 05:46 |
140 | Finding Refresh Token and ID Token | 02:11 |
141 | Sending Request to Resource Server | 05:23 |
142 | Configure CORS Access on API Gateway | 04:54 |
143 | Configure CORS in Resource Server | 10:05 |
144 | Introduction | 04:05 |
145 | Creating a new project | 03:10 |
146 | Creating RemoteUserStorageProvider class | 04:47 |
147 | Creating RemoteUserStorageProviderFactory class | 04:04 |
148 | Adding Keycloak Core Dependency | 01:26 |
149 | Solution overview | 03:28 |
150 | Remote Users Webservice Overview | 09:16 |
151 | RESTEasy HTTP Client Dependency | 01:44 |
152 | Implementing Users Api Service | 05:55 |
153 | Creating User Class | 03:06 |
154 | Adding the verifyUserPassword to UsersApiService | 03:57 |
155 | Building RESTEasy HTTP Client | 04:46 |
156 | Implementing getUserByUsername() method | 05:39 |
157 | Verifying User Password | 03:48 |
158 | Packaging UserStorageProvider | 04:17 |
159 | Deploying User Storage SPI | 08:46 |
160 | Trying how it works | 04:42 |
161 | Introduction | 03:33 |
162 | Creating a new project | 04:02 |
163 | Register an OAuth Client | 08:45 |
164 | Configure Authorization Server | 05:31 |
165 | Spring Security Configuration | 03:40 |
166 | A request for Authorization Code | 04:59 |
167 | Exchange Authorization Code for JWT Access Token | 06:07 |
168 | Creating and Configure Resource Server | 03:52 |
169 | Resource Server API Endpoint | 04:19 |
170 | Consume protected API Endpoints | 03:39 |
171 | Project Overview | 03:41 |
172 | OAuth2 Client-related configuration | 04:42 |
173 | Reading the JWT Access Token | 02:53 |
174 | Sending HTTP Request | 03:44 |
175 | Trying how it works | 01:39 |
Similar courses to OAuth 2.0 in Spring Boot Applications
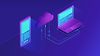
Spring Boot Microservices and Spring Cloududemy
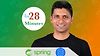
Master Java Unit Testing with Spring Boot & Mockitoudemy
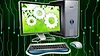
Java Spring Tutorial Masterclass - Learn Spring Framework 5udemy
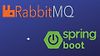
RabbitMQ : Messaging with Java, Spring Boot And Spring MVCudemy
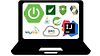
Spring Boot 3 Thymeleaf REAL-TIME Web Application - Blog Appudemy
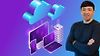
Spring Boot Microservices and Spring Cloud. Build & Deploy.udemy
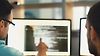
Go Full Stack with Spring Boot and Reactudemy
![[NEW] Spring Boot 3, Spring 6 & Hibernate for Beginners](https://cdn.courseflix.net/courses/100x56/new-spring-boot-3-spring-6-hibernate-for-beginners.jpg?d=1745430708588)