Python 3: Deep Dive (Part 4 - OOP)
Read more about the course
MAIN COURSE TOPICS
what are classes and instances
class data and function attributes
properties
instance, class and static methods
polymorphism and the role special functions play in this
single inheritance
slots
the descriptor protocol and its relationship to properties and functions
enumerations
exceptions
metaprogramming (including metaclasses)
COURSE PREREQUISITES
Please note this is NOT a beginner level course. You must have a strong working knowledge of functional Python programming as well as some practical experience developing Python applications in order to fully benefit from this course.
In-depth functional Python programming
functions, closures, scopes, decorators (using and writing them)
zip, sorted, any, all, and the itertools module in general
sequences, iterables, iterators and generators (what they are and how to implement the corresponding protocols)
generators, yield, and context managers
mapping types, hashing and relation to object equality
some prior knowledge of basic OOP concepts
know how to work with Python virtual environments and pip install
available Jupyter Notebook (freely available) to follow along with the course notebooks
how to use git
[Please note that this is not a cookbook style course - I don't show you how to solve specific problems, but rather a broad and in-depth look at how OOP works in the context of Python, that will allow you to apply these concepts and techniques to your own problems.]
- Strong knowledge of functional Python
- Closures and Decorators
- Iterators, Iterables and Generators
- Mapping Types, Hashing
- Some exposure to basic OOP
- Experienced intermediate Python developers who want a more in-depth understanding of Python OOP
What you'll learn:
- Python Object Oriented Concepts
- Classes
- Methods and Binding
- Instance, Class and Static Methods
- Properties
- Property Decorators
- Single Inheritance
- Slots
- Descriptors
- Enumerations
- Exceptions
- Metaprogramming
Watch Online Python 3: Deep Dive (Part 4 - OOP)
# | Title | Duration |
---|---|---|
1 | Introduction | 05:56 |
2 | Prerequisites | 08:36 |
3 | Introduction | 02:28 |
4 | Objects and Classes - Lecture | 10:50 |
5 | Objects and Classes - Coding | 04:04 |
6 | Class Attributes - Lecture | 13:09 |
7 | Class Attributes - Coding | 12:17 |
8 | Callable Class Attributes - Lecture | 02:24 |
9 | Callable Class Attributes - Coding | 03:08 |
10 | Classes are Callables - Lecture | 03:19 |
11 | Classes are Callables - Coding | 06:30 |
12 | Data Attributes - Lecture | 05:21 |
13 | Data Attributes - Coding | 11:30 |
14 | Function Attributes - Lecture | 12:36 |
15 | Function Attributes - Coding | 20:16 |
16 | Initializing Class Instances - Lecture | 06:17 |
17 | Initializing Class Instances - Coding | 05:51 |
18 | Creating Attributes at Run-Time - Lecture | 05:36 |
19 | Creating Attributes at Run-Time - Coding | 20:47 |
20 | Properties - Lecture | 16:50 |
21 | Properties - Coding | 23:32 |
22 | Property Decorators - Lecture | 12:08 |
23 | Property Decorators - Coding | 31:07 |
24 | Read-Only and Computed Properties - Lecture | 06:49 |
25 | Read-Only and Computed Properties - Coding | 24:58 |
26 | Deleting Properties - Lecture | 06:10 |
27 | Deleting Properties - Coding | 05:14 |
28 | Some Questions on the Property Class | 03:05 |
29 | Class and Static Methods - Lecture | 11:08 |
30 | Class and Static Methods - Coding | 31:00 |
31 | Python Builtin and Standard Types | 03:25 |
32 | Class Body Scope - Lecture | 05:19 |
33 | Class Body Scope - Coding | 14:37 |
34 | Quick Recap | 04:07 |
35 | Project Description | 10:54 |
36 | Project Solution - TimeZone | 15:30 |
37 | Project Solution - Transaction Numbers | 08:57 |
38 | Project Solution - Account Numbers, Names | 14:49 |
39 | Project Solution - Preferred TimeZone | 04:09 |
40 | Project Solution - Account Balance | 04:16 |
41 | Project Solution - Interest Rate | 08:29 |
42 | Project Solution - Transaction Codes | 02:49 |
43 | Project Solution - Confirmation Codes | 28:18 |
44 | Project Solution - Transactions | 23:04 |
45 | Project Solution - Testing with unittest | 50:53 |
46 | Introduction | 04:58 |
47 | __str__ and __repr__ Methods - Lecture | 03:48 |
48 | __str__ and __repr__ Methods - Coding | 08:24 |
49 | Arithmetic Operators - Lecture | 04:53 |
50 | Arithmetic Operators - Coding | 37:02 |
51 | Rich Comparisons - Lecture | 01:30 |
52 | Rich Comparisons - Coding | 18:03 |
53 | Hashing and Equality - Lecture | 01:54 |
54 | Hashing and Equality - Coding | 08:33 |
55 | Booleans - Lecture | 01:39 |
56 | Booleans - Coding | 08:27 |
57 | Callables - Lecture | 01:53 |
58 | Callables - Coding | 37:44 |
59 | The __del__ Method - Lecture | 03:55 |
60 | The __del__ Method - Coding | 15:09 |
61 | The __format__ Method - Lecture | 02:01 |
62 | The __format__ Method - Coding | 08:02 |
63 | Project Description | 06:37 |
64 | Project Solution | 31:42 |
65 | Introduction | 01:15 |
66 | Single Inheritance - Lecture | 18:23 |
67 | Single Inheritance - Coding | 11:20 |
68 | The object Class - Lecture | 04:48 |
69 | The object Class - Coding | 09:58 |
70 | Overriding - Lecture | 11:43 |
71 | Overriding - Coding | 14:00 |
72 | Extending - Lecture | 00:56 |
73 | Extending - Coding | 16:39 |
74 | Delegating to Parent - Lecture | 12:29 |
75 | Delegating to Parent - Coding | 27:21 |
76 | Slots - Lecture | 07:14 |
77 | Slots - Coding | 05:17 |
78 | Slots and Single Inheritance - Lecture | 08:08 |
79 | Slots and Single Inheritance - Coding | 15:09 |
80 | Project 3 - Description | 08:33 |
81 | Project Solution - Approach and Setup | 12:56 |
82 | Project Solution - Validators and tests | 21:44 |
83 | Project Solution - Resources class and tests | 40:05 |
84 | Project Solution - CPU class and tests | 09:09 |
85 | Project Solution - Storage class and tests | 01:59 |
86 | Project Solution - HDD and SSD classes and tests | 05:09 |
87 | Introduction | 01:15 |
88 | Descriptors - Lecture | 08:23 |
89 | Descriptors - Coding | 09:19 |
90 | Getters and Setters - Lecture | 08:16 |
91 | Getters and Setters - Coding | 21:43 |
92 | Using as Instance Properties - Lecture | 06:26 |
93 | Using as Instance Properties - Coding | 22:09 |
94 | Strong and Weak References - Lecture | 07:31 |
95 | Strong and Weak References - Coding | 20:07 |
96 | Back to Instance Properties - Lecture | 03:37 |
97 | Back to Instance Properties - Coding | 35:35 |
98 | The __set_name__ Method - Lecture | 03:05 |
99 | The __set_name__ Method - Coding | 17:41 |
100 | Property Lookup Resolution - Lecture | 04:43 |
101 | Property Lookup Resolution - Coding | 11:45 |
102 | Properties and Descriptors - Lecture | 02:39 |
103 | Properties and Descriptors - Coding | 21:26 |
104 | Application - Example 1 | 11:20 |
105 | Application - Example 2 | 34:25 |
106 | Functions and Descriptors - Lecture | 01:50 |
107 | Functions and Descriptors - Coding | 16:59 |
108 | Project Description | 03:22 |
109 | Solution - Part 1 | 42:36 |
110 | Solution - Part 2 | 08:08 |
111 | Introduction | 01:01 |
112 | Making the case for Enumerations | 08:12 |
113 | Enumerations - Lecture | 09:48 |
114 | Enumerations - Coding | 19:51 |
115 | Aliases - Lecture | 04:49 |
116 | Aliases - Coding | 09:20 |
117 | Customizing/Extending Enums - Lecture | 03:51 |
118 | Customizing/Extending Enums - Coding | 36:31 |
119 | Automatic Values - Lecture | 04:51 |
120 | Automatic Values - Coding | 21:57 |
121 | Project Description | 04:28 |
122 | Project Solution | 11:43 |
123 | Introduction | 01:20 |
124 | Python Exceptions - Lecture | 13:35 |
125 | Python Exceptions - Coding | 20:41 |
126 | Handling Exceptions - Lecture | 17:42 |
127 | Handling Exceptions - Coding | 56:21 |
128 | Raising Exceptions - Lecture | 06:19 |
129 | Raising Exceptions - Coding | 16:30 |
130 | Custom Exceptions - Lecture | 07:47 |
131 | Custom Exceptions - Coding | 54:12 |
132 | Project Description | 05:23 |
133 | Project Solution | 30:14 |
134 | Introduction | 08:51 |
135 | Decorators and Descriptors - Review | 08:51 |
136 | The __new__ Method - Lecture | 10:27 |
137 | The __new__ Method - Coding | 24:06 |
138 | How Classes are Created - Lecture | 07:12 |
139 | How Classes are Created - Coding | 12:42 |
140 | Inheriting from type - Lecture | 03:01 |
141 | Inheriting from type - Coding | 11:02 |
142 | Metaclasses - Lecture | 05:54 |
143 | Metaclasses - Coding | 08:15 |
144 | Class Decorators - Lecture | 04:43 |
145 | Class Decorators - Coding | 54:11 |
146 | Decorator Classes - Lecture | 04:05 |
147 | Decorator Classes - Coding | 18:23 |
148 | Metaclass vs Class Decorator - Lecture | 01:55 |
149 | Metaclass vs Class Decorator - Coding | 14:49 |
150 | Metaclass Parameters - Lecture | 02:45 |
151 | Metaclass Parameters - Coding | 13:17 |
152 | The __prepare__ Method - Lecture | 02:55 |
153 | The __prepare__ Method - Coding | 21:53 |
154 | Classes, Metaclasses, and __call__ | 12:36 |
155 | Metaprogramming Application 1 | 33:40 |
156 | Metaprogramming Application 2 | 23:11 |
157 | Metaprogramming Application 3 | 31:03 |
158 | Attribute Read Accessors - Lecture | 10:06 |
159 | Attribute Read Accessors - Coding | 37:18 |
160 | Attribute Write Accessors - Lecture | 03:34 |
161 | Attribute Write Accessors - Coding | 11:39 |
162 | Accessors - Application | 19:21 |
Similar courses to Python 3: Deep Dive (Part 4 - OOP)
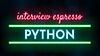
Python Interview Espressointerviewespresso (Aaron Jack)
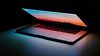
2022 Python for Machine Learning & Data Science Masterclassudemy
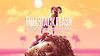
Fullstack Flask: Build a Complete SaaS App with Flaskfullstack.io
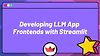
Developing LLM App Frontends with Streamlitzerotomastery.io
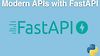
Modern APIs with FastAPI and Python CourseTalkpython
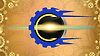
The Automation Bootcamp: Zero to Masteryzerotomastery.io
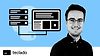
Web Developer Bootcamp with Flask and Python in 2022udemy
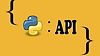
API Testing with Python 3 & PyTest, Backend Automation 2022udemy
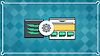
Build a Backend REST API with Python & Django - Advancedudemy
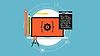