NestJS Zero to Hero - Modern TypeScript Back-end Development
NestJS is a Node.js back-end development framework built upon Express, leveraging the power of TypeScript. NestJS leverages the incredible popularity and robustness of JavaScript as a language and Node.js as a technology. It is inspired by common libraries and frameworks such as Angular, React and Vue which improve developer productivity and experience. Even considering the amount of superb libraries, helpers and tools that exist for server-side Node.js, none of them effectively solve the main problem - the architecture of an application.
More
NestJS provides an out-of-the-box application architecture which allows developers and teams to create highly testable, scalable, loosely coupled and easily maintainable applications.
Recently, the NestJS framework is gaining extreme popularity due to its incredible features;
Leverages TypeScript - strongly typed language which is a super-set of JavaScript
Simple to use, easy to learn and easy to master
Powerful Command Line Interface (CLI) tool that boosts productivity and ease of development
Detailed, well-maintained documentation
Active codebase development and maintenance
Open-source (MIT license)
Supports dozens nest-specific modules that help you easily integrate with common technologies and concepts such as TypeORM, Mongoose, GraphQL, Logging, Validation, Caching, Websockets and much more
Easy of unit-testing applications
Made for Monoliths and Micro-services (entire section in the documentation regarding the Microservice type of a NestJS application, as well as techniques and recipes).
In this course I am going to guide you through the process of planning, developing and deploying a fully-featured back-end application, based on my experience developing and maintaining systems that support dozens of millions of concurrent users at scale.
- Having a basic understanding of JavaScript and/or NodeJS
- Having basic knowledge of TypeScript is recommended, but not required
- Intermediate JavaScript developers who want to dive into back-end development
- Any developers willing to apply TypeScript on the back-end
- Developers eager to learn how to develop performant, secure and production-ready REST APIs following best practices
- Developers who want to learn how to deploy their application to the cloud (Amazon Web Services)
- Developers who want to follow building a practical, real-world application from zero to production
What you'll learn:
- Becoming familiar with the NestJS framework and its components
- Designing and developing REST APIs performing CRUD operations
- Authentication and Authorization for back-end applications
- Using TypeORM for database interaction
- Security best practices, password hashing and storing sensitive information
- Persisting data using a database
- Deploying back-end applications at a production-ready state to Amazon Web Services
- Writing clean, maintainable code in-line with industry standards
- Utilising the NestJS Command Line Interface (CLI)
- Using Postman for testing back-end services
- Using pgAdmin as an interface tool to manage PostgreSQL databases
- Implement efficient logging in a back-end application
- Environment-based configuration management and environment variables
- Implementing data validation and using Pipes
- Guarding endpoints for authorized users using Guards
- Modelling entities for the persistence layer
- TypeScript best practices
- Handling asynchronous operations using async-await
- Using Data Transfer Objects (DTO)
- Hands-on experience with JSON Web Tokens (JWT)
- Unit testing NestJS applications
- Using GraphQL with NestJS
- Database persistence with MongoDB
Watch Online NestJS Zero to Hero - Modern TypeScript Back-end Development
# | Title | Duration |
---|---|---|
1 | Welcome to the course! | 01:12 |
2 | Installing the NestJS CLI | 00:58 |
3 | (Optional) Installing VSCode and Extensions | 02:56 |
4 | Project Overview | 05:18 |
5 | Creating our project via the NestJS CLI | 02:05 |
6 | NestJS Project Structure | 06:37 |
7 | Introduction to NestJS Modules | 02:55 |
8 | Creating a Tasks Module | 03:33 |
9 | Introduction to NestJS Controllers | 03:47 |
10 | Creating at Tasks Controller | 03:38 |
11 | Introduction to NestJS Providers and Services | 05:04 |
12 | Creating a Tasks Service | 05:55 |
13 | Feature: Getting All Tasks | 07:31 |
14 | Creating a Postman Collection | 01:44 |
15 | Defining a Task Model | 05:02 |
16 | Feature: Creating a Task (Part 1 - Controller) | 05:00 |
17 | Feature: Creating a Task (Part 2 - Service) | 06:37 |
18 | Intro to Data Transfer Objects (DTO) | 05:37 |
19 | Implementing CreateTaskDto | 04:59 |
20 | Feature: Getting a Task by ID | 05:44 |
21 | Challenge: Deleting a Task | 00:53 |
22 | Solution: Deleting a Task | 04:24 |
23 | Challenge: Update Task Status | 01:20 |
24 | Solution: Update Task Status | 05:28 |
25 | Feature: Searching and Filtering | 08:50 |
26 | Introduction to NestJS Pipes | 04:15 |
27 | ValidationPipe: Creating a Task | 04:32 |
28 | Error Handling: Getting a non-existing Task | 04:17 |
29 | Error Handling: Deleting a non-existing Task | 01:10 |
30 | Validation: Update Task Status | 03:28 |
31 | Challenge: Validating Task Filtering and Search | 02:43 |
32 | Introduction to Persistence | 00:31 |
33 | Running PostgreSQL via Docker | 04:03 |
34 | Setting up pgAdmin | 01:46 |
35 | Creating a Database using pgAdmin | 00:55 |
36 | Introduction to TypeORM | 02:58 |
37 | Setting up a Database Connection | 04:30 |
38 | Creating a Task Entity | 03:52 |
39 | Active Record VS Data Mapper Patterns | 03:41 |
40 | Creating a Tasks Repository | 03:34 |
41 | Refactoring for Tasks Service | 03:45 |
42 | Persistence: Getting a Task by ID | 08:34 |
43 | Persistence: Creating a Task | 07:32 |
44 | (Challenge) Persistence: Deleting a Task | 01:23 |
45 | (Solution) Persistence: Deleting a Task | 04:59 |
46 | Persistence: Update Task Status | 02:41 |
47 | Small Change Needed | 01:41 |
48 | Persistence: Getting All Tasks | 12:23 |
49 | Intro to Authentication and Authorization | 01:13 |
50 | Setting up AuthModule, User Entity and User Repository | 05:06 |
51 | Feature: Signing Up | 08:27 |
52 | Validation: Credentials and Password Strength | 04:13 |
53 | Error Handling: Username Conflicts | 06:43 |
54 | Securely Storing Passwords | 07:23 |
55 | Password Hashing With Bcrypt | 04:26 |
56 | Feature: Signing In | 04:43 |
57 | Intro to JSON Web Tokens (JWT) | 05:55 |
58 | Setting up the JWT Module and Passport.js | 03:35 |
59 | Signing a JWT Token on Sign In (Authentication) | 07:09 |
60 | Implementing JWT Validation | 11:39 |
61 | Custom @GetUser Decorator | 03:15 |
62 | Guarding the Tasks Routes | 02:01 |
63 | Tasks and Users - Database Relation | 03:59 |
64 | Make Users Own Tasks | 04:37 |
65 | Serialize User Data | 03:40 |
66 | Restricting Getting All Tasks | 03:31 |
67 | BUG FIX: Getting All Tasks | 02:35 |
68 | Restricting Getting a Task By ID | 03:51 |
69 | Restricting Status Updates | 00:51 |
70 | Restricting Deleting A Task | 01:24 |
71 | THANK YOU! (+ Promotion) | 01:34 |
72 | Introduction to Logging | 02:54 |
73 | Implementing Logs in our NestJS app | 14:55 |
74 | Introduction to Configuration | 02:11 |
75 | Quick Intro to Environment Variables | 03:51 |
76 | Setting up ConfigModule | 09:19 |
77 | TypeORM Configuration | 09:38 |
78 | Config Schema Validation | 06:39 |
79 | JWT Secret Configuration | 04:08 |
80 | Front-end Application | 05:36 |
81 | Signing up to Heroku | 01:07 |
82 | Creating a Heroku Application | 01:00 |
83 | Installing the the Heroku CLI | 01:12 |
84 | Postgres on Heroku | 03:00 |
85 | Changes in our NestJS App | 05:12 |
86 | Deploying NestJS to Heroku | 09:49 |
87 | Deploying Front-end to GitHub Pages | 08:21 |
88 | Unit Testing Crash Course: Basics | 01:47 |
89 | Unit Testing Crash Course: First Tests | 06:39 |
90 | IMPORTANT: Fixing import paths | 01:37 |
91 | Testing TasksService - Part 1 | 18:44 |
92 | Testing TasksService - Part 2 | 07:30 |
93 | GraphQL + MongoDB: Section Introduction | 01:06 |
94 | Project Overview: School Management | 02:39 |
95 | Robo 3T - Connecting to the MongoDB Database | 01:15 |
96 | Project setup | 02:05 |
97 | Ensure NestJS 7 Installation | 00:56 |
98 | Installing GraphQL Dependencies | 03:03 |
99 | Creating the Lesson Module | 01:27 |
100 | Defining the Lesson GraphQL Type | 03:14 |
101 | Creating the Lesson Resolver | 04:04 |
102 | Using the GraphQL Playground | 03:45 |
103 | Persistence: TypeORM, MongoDB and our Lesson Entity | 06:35 |
104 | LessonService and createLesson method | 08:06 |
105 | Create Lesson GraphQL Mutation | 05:02 |
106 | getLesson GraphQL Query with MongoDB | 03:41 |
107 | Validation: Create Lesson Input | 07:16 |
108 | Challenge: Get All Lessons GraphQL Query | 04:08 |
109 | Creating the Student Module | 01:45 |
110 | Challenge: Defining the Student Entity | 02:54 |
111 | Challenge: Create Student Mutation | 10:29 |
112 | Challenge: Get All Students GraphQL Query | 02:38 |
113 | Challenge: Get Student by ID Query | 02:45 |
114 | Assign Students To Lesson GraphQL Mutation | 10:27 |
115 | Improvement: Assign Students Upon Lesson Creation | 03:15 |
116 | Resolve "students" Field in Lesson | 08:14 |
Similar courses to NestJS Zero to Hero - Modern TypeScript Back-end Development
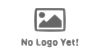
Vue 3, Nuxt.js and NestJS: A Rapid Guide - Advanced
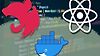
React and NestJS: A Practical Guide with Docker
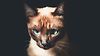
NestJS GraphQL - Schema-first approach
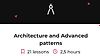
NestJS. Architecture and Advanced Patterns
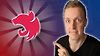
NestJS - Building Real Project API From Scratch
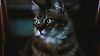
NestJS Microservices
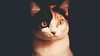
NestJS Authentication and Authorization
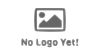
NestJS: The Complete Developer's Guide
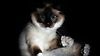
NestJS Advanced Concepts
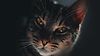