Modern Python Projects
There's a long way from writing your first print("hello world") to shipping a Python application. Installing new packages without breaking others, choosing the best tools that will still be maintained in a few years, figuring out what's the "Pythonic" code that everyone keeps talking about, moving files around to avoid import errors, writing tests even when you don't have time, or making sure that your documentation is up to date - those are some of the struggles that you might encounter.
Read more about the course
This course helps you solve those problems. It takes you through all the steps of a typical Python project - from setting up a good project structure, managing dependencies, adding tests, writing documentation, setting up continuous integration, and finally - deploying it.
This course explains how to improve your code with various static analysis tools. It gives you the tools for installing new packages or updating the Python version without messing it up. It shows you how to build popular types of Python projects like a CLI application or Python package. It even helps you configure the VS Code editor for the best experience when coding in Python!
This course covers everything you need to know to develop, run, and deploy full web apps on top of FastAPI. Just some of the topics include:
- VS Code + Python: A current and growing Python code editor
- Manage Python and packages in the real world
- Create a Python project with cookiecutter templates
- Managing project dependencies with tools like pip, pip-tools, poetry, and more
- See what "good" Python code should look like
- Test your Python code with pytest
- Documenting your code with Sphinx
- Perform some automatic checks on your code with continuous integration
- How to build a CLI application that you can run in your terminal
- Create a Python module/library that can be published on PyPI
- Make a standalone executable application that you can send to someone without Python dependencies
- Deploying your application to a Platform as a Service tool called Heroku
- Create a simple Docker image and deploy it to one of the popular Infrastructure
- And much more
Watch Online Modern Python Projects
# | Title | Duration |
---|---|---|
1 | 01-welcome | 01:30 |
2 | 02-what-you-will-learn | 06:25 |
3 | 03-what-you-need-to-know | 00:42 |
4 | 04-meet-your-instructor | 00:44 |
5 | 05-disclaimer-about-tools-and-services-in-this-course | 00:43 |
6 | 01-python | 00:47 |
7 | 02-code-editor | 00:33 |
8 | 03-source-code | 00:25 |
9 | 01-choosing-a-code-editor | 04:36 |
10 | 02-installing-vscode | 00:25 |
11 | 03-installing-Python-extension | 05:55 |
12 | 04-pylance-and-a-language-server-protocol | 02:53 |
13 | 05-quick-overview-of-vs-code | 10:48 |
14 | 06-running-python-code | 03:08 |
15 | 07-debugging-python-code | 03:56 |
16 | 08-testing-python-code | 04:53 |
17 | 09-snippets | 04:28 |
18 | 10-plugins | 08:22 |
19 | 01-dont-change-system-python | 01:40 |
20 | 02-pyenv | 01:10 |
21 | 03-installing-pyenv | 03:21 |
22 | 04-installing-pyenv-win-Windows | 00:58 |
23 | 05-installing-new-python-version-with-pyenv | 05:18 |
24 | 06-3-levels-of-pyenv | 03:48 |
25 | 07-troubleshooting-pyenv | 01:41 |
26 | 08-bonus-how-pyenv-works | 02:40 |
27 | 09-bonus-is-there-pyenv-for-node-go-etc | 00:45 |
28 | 10-the-problem-with-pip | 05:20 |
29 | 11-what-are-virtual-environments | 01:28 |
30 | 12-creating-a-virtual-environment | 02:14 |
31 | 13-using-a-virtual-environment | 01:28 |
32 | 14-typical-workflow-with-virtual-environment | 02:28 |
33 | 15-virtualenvwrapper-a-virtual-environments-management-tool | 10:04 |
34 | 16-isolate-global-packages-with-pipx | 02:53 |
35 | 17-pipx-in-action | 05:11 |
36 | 18-pipx-packages-in-vs-code | 02:34 |
37 | 19-summary | 03:49 |
38 | 01-starting-a-new-project-can-be-hard | 03:34 |
39 | 02-what-is-cookiecutter | 00:36 |
40 | 03-using-cookiecutter-to-generate-a-project | 08:01 |
41 | 04-tip-how-i-use-cookiecutter | 01:19 |
42 | 05-you-can-build-your-own-template | 02:24 |
43 | 06-summary | 02:35 |
44 | 01-what-is-project-management-in-python | 00:34 |
45 | 02-how-python-imports-modules | 03:28 |
46 | 03-import-errors | 02:34 |
47 | 04-simple-project | 02:58 |
48 | 05-medium-project | 01:39 |
49 | 06-advanced-projects | 01:46 |
50 | 07-makefiles-simple-tasks-management | 05:52 |
51 | 08-dependencies-of-your-project | 00:32 |
52 | 09-requirements-txt-conventions | 03:16 |
53 | 10-separate-requirements-files | 01:15 |
54 | 11-pin-your-dependencies | 01:02 |
55 | 12-pip-tools | 04:12 |
56 | 13-python-project-chores | 01:11 |
57 | 14-pipenv-or-poetry | 01:00 |
58 | 15-poetry-in-action | 04:48 |
59 | 16-publishing-a-package-with-poetry | 01:28 |
60 | 17-other-tools | 03:34 |
61 | 18-my-favorite-tool | 02:02 |
62 | 01-how-to-not-write-good-python-code | 01:44 |
63 | 02-pep8-and-pep257 | 02:01 |
64 | 03-black | 04:22 |
65 | 04-linters | 02:10 |
66 | 05-pylint | 07:00 |
67 | 06-flake8 | 03:11 |
68 | 07-flake8-plugins | 05:20 |
69 | 08-multiple-linters-in-vs-code | 02:05 |
70 | 09-other-static-code-analyzers | 02:56 |
71 | 10-sourcery | 03:09 |
72 | 11-different-python-repl | 01:15 |
73 | 12-ipython | 02:40 |
74 | 13-bpython | 00:54 |
75 | 14-ptpython | 01:00 |
76 | 01-unittest-vs-pytest | 00:42 |
77 | 02-converting-unittest-test-to-pytest | 04:57 |
78 | 03-running-pytest-tests | 03:50 |
79 | 04-pytest-options | 06:58 |
80 | 05-configuration-file | 02:16 |
81 | 06-fixtures | 02:16 |
82 | 07-mocking-and-monkeypatching | 01:18 |
83 | 08-parametrized-tests | 03:39 |
84 | 09-marks | 03:15 |
85 | 10-testing-code-examples-in-the-documentation | 01:21 |
86 | 11-extending-pytest-with-plugins | 01:43 |
87 | 12-useful-pytest-plugins | 03:28 |
88 | 13-moving-from-unittest-to-pytest | 01:49 |
89 | 14-summary | 02:03 |
90 | 01-sphinx | 00:42 |
91 | 02-example-code-for-this-chapter | 01:44 |
92 | 03-set-up-sphinx | 03:02 |
93 | 04-reStructuredText | 02:01 |
94 | 05-adding-more-documentation | 02:03 |
95 | 06-how-to-write-good-documentation | 02:04 |
96 | 07-generating-API-documentation | 04:10 |
97 | 08-show-source-code-in-the-documentation | 00:43 |
98 | 09-testing-your-documentation | 02:41 |
99 | 10-read-the-docs | 00:58 |
100 | 11-mkdocs | 01:16 |
101 | 12-rest-api-documentation | 02:35 |
102 | 01-building-projects-is-not-just-coding | 00:37 |
103 | 02-tox-automate-python-tasks-on-your-computer | 02:31 |
104 | 03-source-code-for-this-chapter | 00:30 |
105 | 04-tox-in-action | 06:59 |
106 | 05-pre-commit | 03:50 |
107 | 06-pre-commit-in-action | 05:34 |
108 | 07-continuous-integration | 01:31 |
109 | 08-github-actions | 03:03 |
110 | 09-github-actions-in-action | 10:15 |
111 | 10-ci-server-vs-your-computer | 00:41 |
112 | 11-gitlab-ci | 00:36 |
113 | 12-gitlab-ci-in-action | 11:30 |
114 | 13-when-to-use-which-tool | 03:15 |
115 | 01-time-for-practice | 00:37 |
116 | 02-cli-applications | 00:40 |
117 | 03-our-cli-application | 02:29 |
118 | 04-technical-considerations | 02:05 |
119 | 05-first-version-of-uptimer | 13:36 |
120 | 06-daemon-mode-and-multiple-urls | 03:45 |
121 | 07-adding-tests | 11:07 |
122 | 08-adding-documentation | 07:01 |
123 | 09-possible-improvements | 03:07 |
124 | 01-lets-build-a-python-package | 00:40 |
125 | 02-technical-considerations | 00:40 |
126 | 03-start-with-a-cookiecutter-template | 02:37 |
127 | 04-remove-unnecessary-files | 05:15 |
128 | 05-add-code | 06:01 |
129 | 06-install-package-from-setup-py | 02:25 |
130 | 07-add-tests | 03:50 |
131 | 08-add-documentation | 06:35 |
132 | 09-publishing-on-pypi | 08:59 |
133 | 10-flit | 01:36 |
134 | 11-private-pypi | 01:09 |
135 | 01-executable-applications | 02:23 |
136 | 02-gui-for-the-uptimer | 06:13 |
137 | 03-warning-building-executable-applications-is-tough | 00:57 |
138 | 04-create-mac-app-with-pyinstaller | 07:14 |
139 | 05-will-it-work-on-a-brand-new-macos-installation | 01:36 |
140 | 06-create-a-windows-exe-file | 09:09 |
141 | 07-will-it-work-on-a-brand-new-windows-installation | 01:33 |
142 | 08-windows-and-a-virus-warning | 01:16 |
143 | 09-cross-platform-python-applications | 01:22 |
144 | 01-deploying-python-application | 01:28 |
145 | 02-virtual-servers-paas-containers | 01:14 |
146 | 03-virtual-private-server | 00:59 |
147 | 04-platform-as-a-service | 01:24 |
148 | 05-docker-containers-and-kubernetes | 03:21 |
149 | 06-uptimer-website | 07:35 |
150 | 07-deploying-to-heroku | 08:23 |
151 | 08-using-docker | 01:43 |
152 | 09-lets-write-a-dockerfile | 04:00 |
153 | 10-building-a-docker-image | 05:06 |
154 | 11-publishing-on-docker-hub | 03:03 |
155 | 12-free-docker-playground | 03:53 |
156 | 13-deploy-docker-image-on-digitalocean | 03:28 |
157 | 14-auto-reboot-and-auto-update | 06:40 |
158 | 15-docker-compose | 02:44 |
159 | 01-thats-all-folks | 00:21 |
160 | 02-vs-code | 01:04 |
161 | 03-pyenv | 01:02 |
162 | 04-virtual-environments | 00:37 |
163 | 05-pipx | 00:59 |
164 | 06-cookiecutter | 01:20 |
165 | 07-growing-python-projects | 00:57 |
166 | 08-pinning-dependencies | 01:09 |
167 | 09-poetry-and-friends | 00:40 |
168 | 10-static-analysis | 01:59 |
169 | 11-pytest | 00:47 |
170 | 12-documentation | 01:04 |
171 | 13-ci | 02:18 |
172 | 14-building-a-cli-application | 00:30 |
173 | 15-building-a-python-package | 00:56 |
174 | 16-building-an-executable-application | 00:39 |
175 | 17-deployment | 01:27 |
176 | 18-thanks-and-bye | 00:23 |
Similar courses to Modern Python Projects
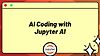
AI Coding with Jupyter AIzerotomastery.io
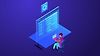
Complete Backend (API) Development with Python A-Zudemy
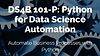
DS4B 101-P: Python for Data Science AutomationBusiness Science University
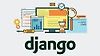
Django 2.1 & Python | The Ultimate Web Development Bootcampudemy
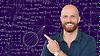
Mathematical Foundations of Machine Learningudemy
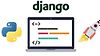
Python and Django Full Stack Web Developer Bootcampudemy
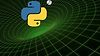
Python 3: Deep Dive (Part 1 - Functional)udemy
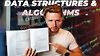
Master Data Structure & Algorithms & Crack the Coding InterviewINTERNET MADE CODER (Tuomas Kivioja)
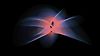
Complete linear algebra: theory and implementationudemy
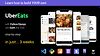