Python 3: Deep Dive (Part 2 - Iteration, Generators)
I will show you exactly how iteration works in Python - from the sequence protocol, to the iterable and iterator protocols, and how we can write our own sequence and iterable data types. We'll go into some detail to explain sequence slicing and how slicing relates to ranges. We look at comprehensions in detail as well and I will show you how list comprehensions are actually closures and have their own scope, and the reason why subtle bugs sometimes creep in to list comprehensions that we might not expect.
Read more about the course
Part 2 of this Python 3: Deep Dive series is an in-depth look at:
sequences
iterables
iterators
generators
comprehensions
context managers
generator based coroutines
We'll take a deep dive into the itertools module and look at all the functions available there and how useful (but overlooked!) they can be.
We also look at generator functions, their relation to iterators, and their comprehension counterparts (generator expressions).
Context managers, an often overlooked construct in Python, is covered in detail too. There we will learn how to create and leverage our own context managers and understand the relationship between context managers and generator functions.
Finally, we'll look at how we can use generators to create coroutines.
Each section is followed by a project designed to put into practice what you learn throughout the course.
This course series is focused on the Python language and the standard library. There is an enormous amount of functionality and things to understand in just the standard CPython distribution, so I do not cover 3rd party libraries - this is a Python deep dive, not an exploration of the many highly useful 3rd party libraries that have grown around Python - those are often sufficiently large to warrant an entire course unto themselves! Indeed, many of them already do!
Please note that this is a relatively advanced Python course, and a strong knowledge of some topics in Python is required.
In particular you should already have an in-depth understanding of the following topics:
functions and function arguments
packing and unpacking iterables and how that is used with function arguments (i.e. using *)
closures
decorators
Boolean truth values and how any object has an associated truth value
named tuples
the zip, map, filter, sorted, reduce functions
lambdas
importing modules and packages
You should also have a basic knowledge of the following topics:
various data types (numeric, string, lists, tuples, dictionaries, sets, etc)
for loops, while loops, break, continue, the else clause
if statements
try...except...else...finally...
basic knowledge of how to create and use classes (methods, properties) - no need for advanced topics such as inheritance or meta classes
understand how certain special methods are used in classes (such as __init__, __eq__, __lt__, etc)
- This is a relatively advanced course, so you should already be familiar with basic Python concepts, as well as some in-depth knowledge as described in the prerequisites in the course description. Please be sure you check those and make sure!
- You will need Python 3.6 or above, and a development environment of your choice (command line, PyCharm, Jupyter, etc.)
- Python developers who want a deeper understanding of sequences, iterables, iterators, generators and context managers.
What you'll learn:
- You'll be able to leverage the concepts in this course to take your Python programming skills to the next level.
- Sequence Types and the sequence protocol
- Iterables and the iterable protocol
- Iterators and the iterator protocol
- List comprehensions and their relation to closures
- Generator functions
- Generator expressions
- Context managers
- Creating context managers using generator functions
- Using Generators as Coroutin
Watch Online Python 3: Deep Dive (Part 2 - Iteration, Generators)
# | Title | Duration |
---|---|---|
1 | Course Overview | 06:31 |
2 | Pre-Requisites | 06:05 |
3 | Python Tools Needed | 03:04 |
4 | Introduction | 01:24 |
5 | Sequence Types - Lecture | 17:11 |
6 | Sequence Types - Coding | 27:24 |
7 | Mutable Sequence Types - Lecture | 07:19 |
8 | Mutable Sequence Types - Coding | 18:07 |
9 | Lists vs Tuples | 21:51 |
10 | Index Base and Slice Bounds - Rationale | 15:15 |
11 | Copying Sequences - Lecture | 29:26 |
12 | Copying Sequences - Coding | 23:29 |
13 | Slicing - Lecture | 32:09 |
14 | Slicing - Coding | 14:43 |
15 | Custom Sequences - Part 1 - Lecture | 10:41 |
16 | Custom Sequences - Part 1 - Coding | 34:01 |
17 | In-Place Concatenation and Repetition - Lecture | 05:35 |
18 | In-Place Concatenation and Repetition - Coding | 07:28 |
19 | Assignments in Mutable Sequences - Lecture | 07:04 |
20 | Assignments in Mutable Sequences - Coding | 10:20 |
21 | Custom Sequences - Part 2 - Lecture | 09:18 |
22 | Custom Sequences - Part 2A - Coding | 17:56 |
23 | Custom Sequences - Part 2B - Coding | 34:50 |
24 | Custom Sequences - Part 2C - Coding | 21:11 |
25 | Sorting Sequences - Lecture | 17:53 |
26 | Sorting Sequences - Coding | 25:53 |
27 | List Comprehensions - Lecture | 17:56 |
28 | List Comprehensions - Coding | 47:17 |
29 | Project Description | 07:33 |
30 | Project Solution: Goal 1 | 40:33 |
31 | Project Solution: Goal 2 | 12:14 |
32 | Introduction | 02:54 |
33 | Iterating Collections - Lecture | 11:20 |
34 | Iterating Collections - Coding | 20:19 |
35 | Iterators - Lecture | 06:22 |
36 | Iterators - Coding | 11:45 |
37 | Iterators and Iterables - Lecture | 11:23 |
38 | Iterators and Iterables - Coding | 28:04 |
39 | Example 1 - Consuming Iterators Manually | 26:32 |
40 | Example 2 - Cyclic Iterators | 31:34 |
41 | Lazy Iterables - Lecture | 03:45 |
42 | Lazy Iterables - Coding | 15:00 |
43 | Python's Built-In Iterables and Iterators - Lecture | 02:25 |
44 | Python's Built-In Iterables and Iterators - Coding | 14:22 |
45 | Sorting Iterables | 08:52 |
46 | The iter() Function - Lecture | 06:27 |
47 | The iter() Function - Coding | 14:00 |
48 | Iterating Callables - Lecture | 04:43 |
49 | Iterating Callables - Coding | 15:54 |
50 | Example 3 - Delegating Iterators | 07:42 |
51 | Reversed Iteration - Lecture | 09:50 |
52 | Reversed Iteration - Coding | 20:01 |
53 | Caveat: Using Iterators as Function Arguments | 18:47 |
54 | Project Description | 03:30 |
55 | Project Solution: Goal 1 | 05:52 |
56 | Project Solution: Goal 2 | 07:43 |
57 | Introduction | 01:22 |
58 | Yielding and Generator Functions - Lecture | 17:39 |
59 | Yielding and Generator Functions - Coding | 17:34 |
60 | Example - Fibonacci Sequence | 15:32 |
61 | Making an Iterable from a Generator - Lecture | 07:00 |
62 | Making an Iterable from a Generator - Coding | 06:41 |
63 | Example - Card Deck | 11:05 |
64 | Generator Expressions and Performance - Lecture | 09:18 |
65 | Generator Expressions and Performance - Coding | 30:20 |
66 | Yield From - Lecture | 02:37 |
67 | Yield From - Coding | 12:30 |
68 | Project Description | 04:16 |
69 | Project Solution: Goal 1 | 41:47 |
70 | Project Solution: Goal 2 | 15:58 |
71 | Introduction | 04:23 |
72 | Aggregators - Lecture | 10:06 |
73 | Aggregators - Coding | 26:29 |
74 | Slicing - Lecture | 03:19 |
75 | Slicing - Coding | 11:34 |
76 | Selecting and Filtering - Lecture | 10:03 |
77 | Selecting and Filtering - Coding | 15:08 |
78 | Infinite Iterators - Lecture | 05:30 |
79 | Infinite Iterators - Coding | 18:50 |
80 | Chaining and Teeing - Lecture | 08:41 |
81 | Chaining and Teeing - Coding | 18:53 |
82 | Mapping and Reducing - Lecture | 15:55 |
83 | Mapping and Reducing - Coding | 18:17 |
84 | Zipping - Lecture | 03:16 |
85 | Zipping - Coding | 06:55 |
86 | Grouping - Lecture | 10:01 |
87 | Grouping - Coding | 27:02 |
88 | Combinatorics - Lecture | 09:31 |
89 | Combinatorics - Coding (Product) | 21:27 |
90 | Combinatorics - Coding (Permutation, Combination) | 20:50 |
91 | Project - Description | 11:50 |
92 | Project Solution: Goal 1 | 43:51 |
93 | Project Solution: Goal 2 | 38:42 |
94 | Project Solution: Goal 3 | 07:18 |
95 | Project Solution: Goal 4 | 50:39 |
96 | Introduction | 08:03 |
97 | Context Managers - Lecture | 22:47 |
98 | Context Managers - Coding | 37:11 |
99 | Caveat when used with Lazy Iterators | 03:50 |
100 | Not just a Context Manager | 07:34 |
101 | Additional Uses - Lecture | 06:05 |
102 | Additional Uses - Coding | 36:04 |
103 | Generators and Context Managers - Lecture | 10:47 |
104 | Generators and Context Managers - Coding | 13:14 |
105 | The contextmanager Decorator - Lecture | 09:43 |
106 | The contextmanager Decorator - Coding | 24:27 |
107 | Nested Context Managers | 34:29 |
108 | Project - Description | 07:18 |
109 | Project Solution: Goal 1 | 17:51 |
110 | Project Solution: Goal 2 | 11:02 |
111 | Introduction | 07:42 |
112 | Coroutines - Lecture | 25:36 |
113 | Coroutines - Coding | 17:12 |
114 | Generator States - Lecture | 03:12 |
115 | Generator States - Coding | 06:48 |
116 | Sending to Generators - Lecture | 14:49 |
117 | Sending to Generators - Coding | 20:05 |
118 | Closing Generators - Lecture | 08:28 |
119 | Closing Generators - Coding | 27:21 |
120 | Sending Exceptions to Generators - Lecture | 07:54 |
121 | Sending Exceptions to Generators - Coding | 24:18 |
122 | Using Decorators to Prime Coroutines - Lecture | 05:42 |
123 | Using Decorators to Prime Coroutines - Coding | 08:47 |
124 | Yield From - Two-Way Communications - Lecture | 10:30 |
125 | Yield From - Two-Way Communications - Coding | 15:13 |
126 | Yield From - Sending Data - Lecture | 05:57 |
127 | Yield From - Sending Data - Coding | 26:56 |
128 | Yield From - Closing and Return - Lecture | 06:24 |
129 | Yield From - Closing and Return - Coding | 14:17 |
130 | Yield From - Throwing Exceptions - Lecture | 02:48 |
131 | Yield From - Throwing Exceptions - Coding | 25:31 |
132 | Application - Pipelines - Lecture | 04:35 |
133 | Application - Pipelines - Pulling Data | 11:28 |
134 | Application - Pipelines - Pushing Data | 09:05 |
135 | Application - Pipelines - Broadcasting Data | 32:39 |
136 | Project Description | 01:50 |
137 | Project Solution | 14:19 |
Similar courses to Python 3: Deep Dive (Part 2 - Iteration, Generators)
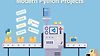
Modern Python ProjectsTalkpython
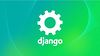
The Ultimate Django Series: Part 2codewithmosh (Mosh Hamedani)
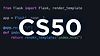
CS50's Web Programming with Python and JavaScriptHarvardX (Harvard University)
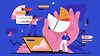
Python Data Analysis & Visualization Masterclassudemy
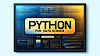
Python for Data ScienceLunarTech
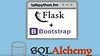
Building data-driven web apps with Flask and SQLAlchemyTalkpython
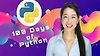
100 Days of Code - The Complete Python Pro Bootcamp for 2023udemy
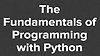
The Fundamentals of Programming with Pythontechwithtim.net (Tim Ruscica)
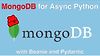
MongoDB with Async PythonTalkpython
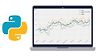