Modern JavaScript From The Beginning 2.0
36h 42m 18s
English
Paid
This is a 37+ hour in-depth course that will take you from the absolute beginning of JavaScript, learning about data types, functions and loops to learning DOM manipulation, asynchronous JS with promises, async/await and much more. You will even learn how to write unit tests for algorithms. We go into how JavaScript works under the hood including execution context, the call stack, event loop, etc. We learn about Webpack tooling and how to create a modern development environment. At the end, we build a Node.js/Express API with a custom Webpack frontend.
Read more about the course
What you'll learn
- All of the fundamentals, including variables, data types, functions, scope, etc
- DOM Manipulation, events and creating dynamic UIs
- Under the hood topics, like execution context and the call stack
- Asynchrounous JS including callbacks, promises, async/await
- Web APIs like geolocation, audio/video, canvas and speech
- OOP including classes and prototypes
- Modern environments with with Webpack tooling
- Bonus: Complete full-stack application with Node.js/Express & Webpack
Watch Online Modern JavaScript From The Beginning 2.0
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Welcome To The Course | 02:40 |
2 | Course Outline | 07:50 |
3 | Premium Docs | 03:06 |
4 | What Is JavaScript? | 07:43 |
5 | Tools & Setup | 05:25 |
6 | Running JavaScript In The Browser | 07:03 |
7 | Section Intro | 00:52 |
8 | The Sandbox Files | 02:58 |
9 | Using The Console | 10:07 |
10 | Comments & Shortcuts | 07:01 |
11 | Variables & Constants | 11:28 |
12 | Data Types | 12:55 |
13 | Primitive vs Reference Types | 07:08 |
14 | Type Conversion | 10:07 |
15 | Operators | 09:39 |
16 | Type Coercion | 04:01 |
17 | Working With Strings | 15:59 |
18 | Capitalize Challenge | 06:50 |
19 | Working With Numbers | 05:46 |
20 | The Math Object | 05:57 |
21 | Number Challenge | 08:30 |
22 | Dates & Times | 08:13 |
23 | 17 - Date Methods & DateTimeFormat API | 08:59 |
24 | Section Intro | 00:42 |
25 | Creating Arrays | 07:25 |
26 | Basic Array Methods | 10:26 |
27 | Nesting, Concat & The Spread Operator | 10:13 |
28 | Array Challenges | 07:05 |
29 | Object Literals | 08:07 |
30 | Object Spread Operator & Methods | 11:59 |
31 | Destructuring & Naming | 06:57 |
32 | JSON Intro | 09:02 |
33 | Object Challenges | 06:22 |
34 | Section Intro | 01:33 |
35 | Creating A Function | 06:08 |
36 | More on Arguments & Parameters | 10:21 |
37 | Global & Function Scope | 05:26 |
38 | Block Scope | 05:15 |
39 | Nested Scope | 02:57 |
40 | Declaration vs Expression | 04:15 |
41 | Arrow Functions | 07:37 |
42 | Immediately Invoked Function Expressions (IIFE) | 05:03 |
43 | Function Challenges | 12:20 |
44 | Execution Context | 09:19 |
45 | Execution Context In Action | 04:00 |
46 | The Call Stack | 04:53 |
47 | Section Intro | 01:09 |
48 | If Statements | 06:54 |
49 | Else-If & Nesting | 07:10 |
50 | Switches | 04:58 |
51 | Calculator Challenge | 03:21 |
52 | Truthy & Falsy | 12:17 |
53 | Logical Operators | 08:08 |
54 | Logical Assignment | 06:11 |
55 | Ternary Operator | 10:18 |
56 | Section Intro | 00:57 |
57 | For Loop | 11:26 |
58 | Break & Continue | 02:52 |
59 | While & Do While Loops | 07:00 |
60 | FizzBuzz Challenge | 08:01 |
61 | For...of Loop | 05:05 |
62 | For...in Loop | 03:08 |
63 | Array.forEach Method | 08:35 |
64 | Array.filter Method | 11:33 |
65 | Array.map Method | 15:31 |
66 | Array.reduce Method | 08:50 |
67 | Array Method Challenges | 10:27 |
68 | Section Intro | 01:17 |
69 | Intro To The DOM | 11:05 |
70 | Document Element Properties | 14:20 |
71 | DOM Selectors - Single Elements | 14:14 |
72 | DOM Selectors - Multiple Elements | 09:17 |
73 | Traversing The DOM - Elements | 11:27 |
74 | Traversing The DOM - All Nodes | 13:48 |
75 | Create & Append Elements | 06:16 |
76 | InnerHTML vs createElement() | 08:52 |
77 | Refactor To Multiple Functions | 04:10 |
78 | Insert Elements, Text & HTML | 09:25 |
79 | Custom insertAfter() Challenge | 04:48 |
80 | Replace Elements | 09:47 |
81 | Remove Elements | 07:47 |
82 | Manipulating Styles & Classes | 09:38 |
83 | Section Intro | 00:52 |
84 | Event Listeners | 13:01 |
85 | Mouse Events | 10:42 |
86 | The Event Object | 13:01 |
87 | Keyboard Events & Key Properties | 10:28 |
88 | KeyCode Mini-Project | 14:52 |
89 | Input Events | 11:48 |
90 | Form Submission & FormData Object | 10:44 |
91 | Event Bubbling | 05:39 |
92 | Event Delegation & Multiple Events | 05:46 |
93 | Page Loading & Window Events | 10:37 |
94 | Project Intro | 03:32 |
95 | Add Items To List (DOM Only) | 11:41 |
96 | Setting Up Git & Github (Optional) | 15:25 |
97 | Remove & Clear Items | 07:53 |
98 | Clear UI State | 09:53 |
99 | Filter Items | 09:56 |
100 | Local Storage Crash Course | 05:32 |
101 | Add Items To Local Storage | 08:05 |
102 | Display Items From Local Storage | 06:16 |
103 | Remove Items From Local Storage | 09:01 |
104 | Set Item To Edit | 08:01 |
105 | Update Item & Reset State | 06:11 |
106 | Prevent Duplicate Items | 03:47 |
107 | Deploy To Netlify | 04:59 |
108 | Section Intro | 00:49 |
109 | Under The Hood: Thread Of Execution | 02:40 |
110 | Under The Hood: How Async JS Works | 08:40 |
111 | setTimeout & clearTimeout Functions | 04:40 |
112 | setInterval & clearInterval Functions | 08:52 |
113 | Callbacks | 09:57 |
114 | Crash Course On HTTP Requests | 07:14 |
115 | DevTools Network Tab | 04:51 |
116 | AJAX & XHR Object | 11:29 |
117 | Joke Generator Project Challenge | 09:41 |
118 | Callback Hell | 06:53 |
119 | Promises | 10:03 |
120 | Callback To Promise Refactor | 04:59 |
121 | Promise Chaining | 04:16 |
122 | Promises vs Callback Hell | 06:02 |
123 | Handling Multiple Promises with promise.all() | 06:05 |
124 | Section Intro | 01:27 |
125 | Fetch Basics | 09:03 |
126 | Random User Mini-Project | 12:53 |
127 | Fetch Options - Method, Body Headers | 14:56 |
128 | Typicode Todos Mini-Project - Part 1 | 17:11 |
129 | Typicode Todos Mini-Project - Part 2 | 13:30 |
130 | Fetch API Error Handling | 13:18 |
131 | Async & Await | 09:26 |
132 | Try...Catch Statements | 04:42 |
133 | Error Handling With Async & Await | 06:43 |
134 | Multiple Promises With Async & Await | 12:27 |
135 | Project Intro | 04:23 |
136 | Theme Overview & Prep | 07:15 |
137 | API Overview & API Key | 04:51 |
138 | Page Router & Active Link | 09:52 |
139 | Display Popular Movies | 13:35 |
140 | Spinner & Popular TV Shows | 07:22 |
141 | Movie Details Page | 13:15 |
142 | Details Page Backdrop | 04:53 |
143 | TV Show Details Page | 07:07 |
144 | Swiper Slider | 13:29 |
145 | Search Functionality | 19:13 |
146 | Display Search Results | 11:35 |
147 | Add Pagination For Search | 16:38 |
148 | Section Intro | 01:57 |
149 | GeoLocation API | 11:47 |
150 | Show Location on a Map | 07:30 |
151 | Canvas Element & API | 13:48 |
152 | requestAnimationFrame() Method | 08:11 |
153 | Animated Clock - Part 1 | 25:08 |
154 | Animated Clock - Part 2 | 11:09 |
155 | Web Audio API | 08:26 |
156 | Music Player Project | 20:03 |
157 | Drum Machine Project | 09:07 |
158 | Video API | 06:21 |
159 | Video Player Project | 12:44 |
160 | Web Animations API - Ball Project | 10:26 |
161 | Speech Recognition API - Color Say Project | 11:39 |
162 | Speech Synthesis API - Text To Speech | 13:22 |
163 | Section Intro | 02:01 |
164 | What Is OOP? | 09:18 |
165 | 4 Basic Principles of OOP | 06:54 |
166 | More on Object Literals & this Keyword | 05:02 |
167 | Constructor Functions | 07:07 |
168 | Literals vs Built-in Constructors | 09:48 |
169 | Working With Object Properties | 07:29 |
170 | Prototypes & The Prototype Chain | 04:55 |
171 | Adding Methods to the Prototype | 04:38 |
172 | Using Object.create() | 04:56 |
173 | Prototypical Inheritance & call() | 10:12 |
174 | OOP Game Challenge | 07:44 |
175 | Classes | 07:30 |
176 | Class Inheritance | 06:29 |
177 | Static Methods | 02:54 |
178 | bind() & Defining this | 05:21 |
179 | Getters & Setters with Classes | 09:31 |
180 | Getters & Setters with defineProperty() | 10:56 |
181 | Private Property Underscore Convention | 12:14 |
182 | ES2022 Private Class Fields | 04:43 |
183 | Property Flags & Descriptors | 09:13 |
184 | Sealing & Freezing Objects | 07:26 |
185 | Project Intro | 04:17 |
186 | UI Theme Setup | 04:43 |
187 | Project Planning & Diagram | 05:04 |
188 | Base Tracker, Meal & Workout Class | 09:56 |
189 | Display Tracker Stats | 14:46 |
190 | Progress Bar & Calorie Alert | 10:01 |
191 | App Class, New Meal & Workout | 13:11 |
192 | Refactor to Single _newItem Method | 04:31 |
193 | Display New Meal & Workout | 07:36 |
194 | Remove Meal & Workout | 10:01 |
195 | Filter & Reset | 10:52 |
196 | Set Calorie Limit | 05:17 |
197 | Storage Class & Calorie Limit Persist | 05:47 |
198 | Persist Total Calories To Local Storage | 04:41 |
199 | Save Meals To Local Storage | 07:40 |
200 | Save Workouts To Local Storage | 03:44 |
201 | Remove Meals & Workouts From LocalStorage | 05:53 |
202 | Clear Storage Items | 04:15 |
203 | Section Intro | 02:07 |
204 | What Are Modules? | 07:04 |
205 | Installing & Using Node.js | 06:17 |
206 | CommonJS Modules | 09:18 |
207 | NPM Packages/Modules | 11:21 |
208 | ES Modules | 07:27 |
209 | Module Bundlers | 05:45 |
210 | Webpack Basic Setup | 11:18 |
211 | CSS & Style Loaders | 04:40 |
212 | HTML Webpack Plugin | 08:17 |
213 | Webpack DevServer Plugin | 04:40 |
214 | Babel Setup | 05:06 |
215 | CSS Minify Extract Plugin | 03:01 |
216 | Tracalorie Refactor To Use Webpack | 16:29 |
217 | Deploy Tracalorie To Netlify | 04:39 |
218 | Section Intro | 01:25 |
219 | Symbols | 10:24 |
220 | Iterators | 09:13 |
221 | Generators | 05:09 |
222 | Profile Scroller Project | 07:53 |
223 | Sets | 05:14 |
224 | Maps | 06:17 |
225 | Poll Project | 12:04 |
226 | Stacks | 13:31 |
227 | Queues | 08:45 |
228 | Linked Lists | 25:18 |
229 | Intro & What Are Algorithms? | 02:44 |
230 | What is Unit Testing? | 03:24 |
231 | Getting Started with Jest | 05:46 |
232 | Grouping Tests Together | 10:53 |
233 | Reverse String Algorithm | 09:50 |
234 | Palindrome Algorithm | 09:59 |
235 | Array Chunking | 06:12 |
236 | Anagram Algorithm | 11:58 |
237 | Get Elements By Tag - jsdom | 17:53 |
238 | Has Duplicate IDs - jsdom | 15:45 |
239 | Section Intro | 02:19 |
240 | fs (filesystem) Module | 13:56 |
241 | path Module | 07:57 |
242 | os Module | 11:05 |
243 | url & querystring Modules | 07:23 |
244 | http Module | 18:21 |
245 | Project Intro | 03:34 |
246 | Express Setup & Basic API | 16:05 |
247 | Nodemon & Route Clean Up | 06:06 |
248 | Handle POST Requests - Add Idea | 08:21 |
249 | PUT & DELETE Requests - Update & Remove Ideas | 06:18 |
250 | What Is MongoDB? | 04:47 |
251 | MongoDB Atlas Setup | 05:52 |
252 | Mongoose Connect & Dotenv | 08:54 |
253 | Mongoose Schema & Model | 08:54 |
254 | Database Queries | 15:15 |
255 | Fullstack Workflow | 03:01 |
256 | Client Folder Setup | 09:16 |
257 | Modal Component | 08:31 |
258 | IdeaForm Component | 12:01 |
259 | IdeaList Component | 12:05 |
260 | API Service - Fetch Ideas | 11:58 |
261 | Create Idea via Form | 07:26 |
262 | Save Username to Local Storage | 04:57 |
263 | Add Username Validation To Server | 07:21 |
264 | Delete Ideas | 12:57 |
265 | Deploying A Fullstack App | 08:37 |
Similar courses to Modern JavaScript From The Beginning 2.0
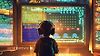
Fast and Furious Game Development with JavaScript and AIudemy
Category: JavaScript, ChatGPT
Duration 45 hours 58 minutes 32 seconds
Course
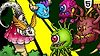
Build Animated Physics Game with JavaScriptudemy
Category: JavaScript
Duration 3 hours 29 minutes 27 seconds
Course
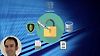
Web security: Injection Attacks with Java & Spring Bootudemy
Category: JavaScript, Spring Boot, Spring Data, Ethical Hacking / Penetration Testing
Duration 8 hours 44 minutes 36 seconds
Course
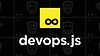
DevOps.js Conference 2021devopsjsconf.com
Category: JavaScript, Other (Devops), Conferences
Duration 7 hours 51 minutes 4 seconds
Course
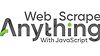
Web Scrape Anything With JavaScriptinterviewespresso (Aaron Jack)
Category: JavaScript
Duration 6 hours 9 seconds
Course
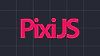
Up and Running With PixiJStutspluscom
Category: JavaScript
Duration 2 hours 8 minutes 52 seconds
Course
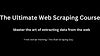
The Ultimate Web Scraping CourseAdrian Horning (The Web Scraping Guy)
Category: JavaScript, Other (Frontend)
Duration 10 hours 39 minutes 26 seconds
Course