Testing JavaScript with Kent C. Dodds
14h 11m 26s
English
Paid
May 18, 2023
This course will apply the four-layer testing method to a React application, but this foundational strategy can be applied across any JavaScript framework: Angular, Vue, legacy Backbone apps, even frameworks and libraries we haven’t met yet.
Watch Online Testing JavaScript with Kent C. Dodds
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Intro to Fundamentals of Testing in JavaScript | 00:33 |
2 | Throw an Error with a Simple Test in JavaScript | 01:42 |
3 | Abstract Test Assertions into a JavaScript Assertion Library | 01:36 |
4 | Encapsulate and Isolate Tests by building a JavaScript Testing Framework | 02:28 |
5 | Support Async Tests with JavaScripts Promises through async await | 01:06 |
6 | Provide Testing Helper Functions as Globals in JavaScript | 00:55 |
7 | Verify Custom JavaScript Tests with Jest | 00:38 |
8 | Intro to JavaScript Mocking Fundamentals | 02:41 |
9 | Override Object Properties to Mock with Monkey-patching in JavaScript | 02:05 |
10 | Ensure Functions are Called Correctly with JavaScript Mocks | 03:21 |
11 | Restore the Original Implementation of a Mocked JavaScript Function with jest.spyOn | 02:13 |
12 | Mock a JavaScript module in a test | 03:39 |
13 | Make a shared JavaScript mock module | 01:58 |
14 | Intro to Static Analysis Testing JavaScript Applications | 01:00 |
15 | Lint JavaScript by Configuring and Running ESLint | 04:41 |
16 | Use the ESLint Extension for VSCode | 01:18 |
17 | Use pre-built ESLint Configuration using extends | 02:01 |
18 | Run ESLint with npm Scripts | 02:19 |
19 | Format Code by Installing and Running Prettier | 02:56 |
20 | Configure Prettier | 02:13 |
21 | Use the Prettier Extension for VSCode | 01:47 |
22 | Disable Unnecessary ESLint Stylistic Rules with eslint-config-prettier | 02:26 |
23 | Validate All Files are Properly Formatted with Prettier | 03:11 |
24 | Avoid Common Errors by Installing and Configuring TypeScript | 05:30 |
25 | Make ESLint Support TypeScript Files | 05:29 |
26 | Validate Code in a pre-commit git Hook with husky | 02:47 |
27 | Auto-format All Files and Validate Relevant Files in a precommit Script with lint-staged | 04:19 |
28 | Run Multiple npm Scripts in Parallel with npm-run-all | 01:17 |
29 | Intro to Use DOM Testing Library to test any JS framework | 02:02 |
30 | Use DOM Testing Library with React | 02:02 |
31 | Use DOM Testing Library with Preact | 02:14 |
32 | Use DOM Testing Library with jQuery | 00:40 |
33 | Use DOM Testing Library with Dojo | 01:26 |
34 | Use DOM Testing Library with HyperApp | 01:33 |
35 | Use DOM Testing Library with AngularJS | 01:03 |
36 | Use DOM Testing Library with Angular | 01:29 |
37 | Use DOM Testing Library with VueJS | 00:57 |
38 | Use DOM Testing Library with Mithril | 01:09 |
39 | Use DOM Testing Library with from-html | 01:08 |
40 | Use DOM Testing Library with Svelte | 01:39 |
41 | Intro to Configure Jest for Testing JavaScript Applications | 02:24 |
42 | Install and Run Jest | 02:48 |
43 | Compile Modules with Babel in Jest Tests | 02:54 |
44 | Configure Jest’s Test Environment for Testing Node or Browser Code | 02:40 |
45 | Support Importing CSS files with Jest’s moduleNameMapper | 03:42 |
46 | Support using Webpack CSS Modules with Jest | 03:39 |
47 | Generate a Serializable Value with Jest Snapshots | 08:37 |
48 | Test an Emotion Styled UI with Custom Jest Snapshot Serializers | 02:22 |
49 | Support Custom Module Resolution with Jest moduleDirectories | 02:29 |
50 | Configure Jest to Run Setup for All Tests with Jest setupFilesAfterEnv | 02:54 |
51 | Support a Test Utilities File with Jest moduleDirectories | 10:58 |
52 | Use Jest Watch Mode to Speed Up Development | 07:15 |
53 | Step through Code in Jest using the Node.js Debugger and Chrome DevTools | 04:15 |
54 | Configure Jest to Report Code Coverage on Project Files | 04:41 |
55 | Analyze Jest Code Coverage Reports | 04:36 |
56 | Set a Code Coverage Threshold in Jest to Maintain Code Coverage Levels | 04:27 |
57 | Report Jest Test Coverage to Codecov through TravisCI | 01:42 |
58 | Run Jest Watch Mode by Default Locally with is-ci-cli | 02:10 |
59 | Run Tests with a Different Configuration using Jest’s --config Flag and testMatch Option | 06:34 |
60 | Support Running Multiple Configurations with Jest’s Projects Feature | 04:19 |
61 | Run ESLint with Jest using jest-runner-eslint | 04:38 |
62 | Test Specific Projects in Jest Watch Mode with jest-watch-select-projects | 02:25 |
63 | Filter which Tests are Run with Typeahead Support in Jest Watch Mode | 02:24 |
64 | Run Only Relevant Jest Tests on Git Commit to Avoid Breakages | 04:10 |
65 | What's changed in Test React Components with Jest and React Testing Library | 05:12 |
66 | Intro to Test React Components with Jest and React Testing Library | 01:43 |
67 | Render a React Component for Testing | 02:29 |
68 | Use Jest DOM for Improved Assertions | 03:08 |
69 | Use DOM Testing Library to Write More Maintainable React Tests | 04:19 |
70 | Use React Testing Library to Render and Test React Components | 01:32 |
71 | Debug the DOM State During Tests using React Testing Library’s debug Function | 01:11 |
72 | Test React Component Event Handlers with fireEvent from React Testing Library | 03:09 |
73 | Improve Test Confidence with the User Event Module | 01:30 |
74 | Test Prop Updates with React Testing Library | 01:05 |
75 | Assert That Something is NOT Rendered with React Testing Library | 02:01 |
76 | Test Accessibility of Rendered React Components with jest-axe | 03:18 |
77 | Test componentDidCatch Handler Error Boundaries with React Testing Library | 06:18 |
78 | Hide console.error Logs when Testing Error Boundaries with jest.spyOn | 02:56 |
79 | Ensure Error Boundaries Can Successfully Recover from Errors | 03:58 |
80 | Mock react-transition-group in React Component Tests with jest.mock | 04:56 |
81 | Use React Testing Library’s Wrapper Option to Simplify using rerender | 01:11 |
82 | Mock HTTP Requests with jest.mock in React Component Tests | 06:44 |
83 | Mock HTTP Requests with MSW | 06:45 |
84 | Test Drive the Development of a React Form with React Testing Library | 03:11 |
85 | Test Drive the Submission of a React Form with React Testing Library | 01:38 |
86 | Test Drive the API Call of a React Form with React Testing Library | 06:50 |
87 | Test Drive Mocking react-router’s Redirect Component on a Form Submission | 05:57 |
88 | Test Drive Assertions with Dates in React | 03:46 |
89 | Use Generated Data in Tests with tests-data-bot to Improve Test Maintainability | 03:25 |
90 | Test Drive Error State with React Testing Library | 05:23 |
91 | Write a Custom Render Function to Share Code between Tests and Simplify Tests | 02:56 |
92 | Test React Components that Use the react-router Router Provider with createMemoryHistory | 03:59 |
93 | Initialize the `history` Object with a Bad Entry to Test the react-router no-match Route | 01:14 |
94 | Create a Custom render Function to Simplify Tests of react-router Components | 05:16 |
95 | Test a Redux Connected React Component | 03:01 |
96 | Test a Redux Connected React Component with Initialized State | 01:36 |
97 | Create a Custom Render Function to Simplify Tests of Redux Components | 04:02 |
98 | Test a Custom React Hook with React’s Act Utility and a Test Component | 03:17 |
99 | Write a Setup Function to Reduce Duplication of Testing Custom React Hooks | 03:27 |
100 | Test a Custom React Hook with renderHook from React Hooks Testing Library | 01:28 |
101 | Test Updates to Your Custom React Hook with rerender from React Hooks Testing Library | 01:00 |
102 | Test React Portals with within from React Testing Library | 04:17 |
103 | Test Unmounting a React Component with React Testing Library | 04:39 |
104 | Write React Application Integration Tests with React Testing Library | 07:40 |
105 | Improve Reliability of Integration Tests using find* Queries from React Testing Library | 02:03 |
106 | Improve Reliability of Integration Tests Using the User Event Module | 01:51 |
107 | What's changed in Install, Configure, and Script Cypress for JavaScript Web Applications | 02:01 |
108 | Intro to Install, Configure, and Script Cypress for JavaScript Web Applications | 01:40 |
109 | Install and Run Cypress | 03:20 |
110 | Write the First Cypress Test | 04:58 |
111 | Configure Cypress in cypress.json | 02:43 |
112 | Installing Cypress Testing Library | 03:03 |
113 | Script Cypress for Local Development and Continuous Integration | 05:08 |
114 | Debug a Test with Cypress | 04:23 |
115 | Test User Registration with Cypress | 04:43 |
116 | Cypress Driven Development | 02:20 |
117 | Simulate HTTP Errors in Cypress Tests | 03:17 |
118 | Test User Login with Cypress | 01:28 |
119 | Create a User with cy.request from Cypress | 02:20 |
120 | Keep Tests Isolated and Focused with Custom Cypress Commands | 02:09 |
121 | Use Custom Cypress Command for Reusable Assertions | 01:38 |
122 | Run Tests as an Authenticated User with Cypress | 01:21 |
123 | Use cy.request from Cypress to Authenticate as a New User | 01:46 |
124 | Use a Custom Cypress Command to Login as a User | 00:40 |
125 | Combine Custom Cypress Commands into a Single Custom Command | 01:38 |
126 | Install React DevTools with Cypress | 03:09 |
127 | Intro to Test Node.js Backends | 04:24 |
128 | Test Pure Functions Overview | 01:29 |
129 | Write Unit Tests for a Simple Pure Function | 02:47 |
130 | Improve Error Messages by Generating Test Titles | 04:47 |
131 | Use jest-in-case to Reduce Duplication and Improve Test Titles | 04:07 |
132 | Create a Casify Function to Generate Cases for jest-in-case | 04:14 |
133 | Test Node Middleware Overview | 01:57 |
134 | Write a Unit Test for Handling an UnauthorizedError | 06:20 |
135 | Write a Unit Test for Handling headersSent in an Error Middleware | 02:04 |
136 | Write a Unit Test for Status 500 Error Middleware Fallback | 02:21 |
137 | Improve Test Maintainability using the Test Object Factory Pattern | 02:58 |
138 | Use a Test Object Factory utils/generate | 03:40 |
139 | Test Node Controllers Overview | 02:52 |
140 | Write a Unit Test for a Controller by Mocking the Database | 06:27 |
141 | Simplify Assertions on Error Messages with toMatchInlineSnapshot | 05:36 |
142 | Unit Test Business Logic of a Controller’s Middleware | 05:29 |
143 | Test Controller 404 Edge Case where Resource is Not Found | 03:10 |
144 | Test Controller Unauthorized Case | 03:39 |
145 | Test getListItems for Getting Multiple Mock Objects | 05:58 |
146 | Test the Happy Path of a Creation Flow | 06:09 |
147 | Testing an Error Case for createListItem | 03:35 |
148 | Testing the Happy Path for updateListItem | 04:50 |
149 | Testing Resource Deletion | 02:28 |
150 | Test Authentication API Routes Overview | 04:15 |
151 | Start a Node Server and Fire a Request to an HTTP API Endpoint | 04:13 |
152 | Make Assertions on HTTP API Responses for Registration | 01:37 |
153 | Test the Login Endpoint for a Node Server | 01:58 |
154 | Test the User’s Resource Endpoint | 01:58 |
155 | Create a Pre-configured axios Client for the baseURL | 01:48 |
156 | Improve Error Messages with an axios Interceptor | 06:56 |
157 | Ensure a Unique Server Port | 05:03 |
158 | Use Snapshots to Assert on Server Error Responses | 03:01 |
159 | Interact Directly with the Database | 01:39 |
160 | Test all the Edge Cases | 03:39 |
161 | Test CRUD API Routes Overview | 01:19 |
162 | Write an Integration Test for a Resource Create Endpoint | 03:50 |
163 | Write an Integration Test for a Resource Read Endpoint | 01:43 |
164 | Integration Test a Resource Update Endpoint | 01:42 |
165 | Integration Test a Resource Delete Endpoint | 04:01 |
166 | Snapshot the Error Message with Dynamic Data | 01:54 |
167 | Practical testing with Wes Bos and Scott Tolinski | 22:04 |
168 | a11y with Marcy Sutton | 34:23 |
169 | Static Types with Jessica Kerr | 28:42 |
170 | Testing Practices with J.B. Rainsberger | 33:27 |
171 | Visual regression testing with Angie Jones | 26:46 |
172 | Snapshots and Reason with Jared Forsyth | 35:02 |
173 | Testing culture with Justin Searls | 46:15 |
174 | Ministry of testing with Rosie Sherry | 27:42 |
175 | Craftmanship with Kent Beck | 32:56 |
176 | Testing Levels with Mattias Johansson | 38:32 |
Similar courses to Testing JavaScript with Kent C. Dodds
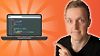
Build Fullstack Trello clone: WebSocket, Socket IO
Duration 8 hours 49 minutes 48 seconds
Course
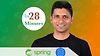
Master Java Unit Testing with Spring Boot & Mockito
Duration 3 hours 56 minutes 12 seconds
Course
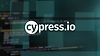
Cypress: Web Automation Testing from Zero to Hero
Duration 10 hours 39 minutes 49 seconds
Course
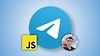
Build Telegram Bots with JavaScript: The Complete Guide
Duration 5 hours 28 minutes 48 seconds
Course
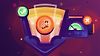
Test with Jest
Duration 52 minutes 39 seconds
Course
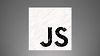
JavaScript: Understanding the Weird Parts
Duration 12 hours 10 minutes 48 seconds
Course
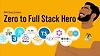
Zero to Full Stack Hero
Duration 101 hours 29 minutes 59 seconds
Course
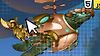
Learn HTML Canvas - Pixels, Particles & Physics
Duration 2 hours 15 minutes 38 seconds
Course
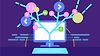
100 Days Of Code: The Complete Web Development Bootcamp 2022
Duration 78 hours 51 minutes 55 seconds
Course
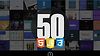
50 Projects In 50 Days - HTML, CSS & JavaScript
Duration 18 hours 13 minutes 45 seconds
Course