MERN 2024 Edition - MongoDB, Express, React and NodeJS
19h 7m
English
Paid
November 13, 2024
Welcome to the immersion in MERN Stack! This course will help you master all aspects of creating a full-featured "Jobify" application using MongoDB, Express, React, and Node.js, regardless of your skill level.
More
Main Topics of the Course:
- Frontend with React: Create a React frontend application from scratch using VITE, add global styles, and create Landing, Error, Register, and Dashboard pages.
- Visual Design: Master creating professional and attractive interfaces using thoughtfully designed images and layouts.
- Routing with React Router 6.4+: Manage transitions and nested pages with React Router.
- Creating a Server Application: Dive into backend development with ES6 modules and use "nodemon" for rapid development.
- Database Management: Set up a cloud database with MongoDB using Atlas, and create routes and controllers for efficient data handling.
- Testing and Error Handling: Use Thunder Client for testing, set up error handling in Express, and use the "express-async-errors" package for simplified debugging.
- Security and Authentication: Hash passwords, implement JWT for secure authentication, and compare passwords to ensure user safety.
- Frontend and Backend Integration: Connect frontend with the server, use "concurrently" and set up a "proxy" in VITE for seamless integration.
- Advanced React Techniques: Implement protected routes, set up navigation with React Router 6, and logout functionalities.
- API Management: Use Axios to interact with APIs, set up JWT tokens, and various Axios configurations for efficient data retrieval and management.
- CRUD and Access Control: Implement full CRUD functionality, set up access rights, and understand creating and managing test data.
- Data Visualization and UI Enhancement: Set up informative charts and cards, implement search and filtering for user convenience.
- Pagination and Deployment: Master pagination and deploy a MERN application on Render for real-world usage.
This course will be your guide to the world of full-stack development using MERN, providing skills to create reliable and scalable web applications. Join us and become a professional MERN Stack developer!
Watch Online MERN 2024 Edition - MongoDB, Express, React and NodeJS
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Course Requirements | 02:03 |
2 | Video Blur Fix | 01:36 |
3 | Course Review | 00:32 |
4 | VS Code | 06:15 |
5 | VITE - Info | 01:24 |
6 | VITE - Install | 02:54 |
7 | VITE - Folder Structure | 06:22 |
8 | Remove Boilerplate | 00:56 |
9 | Obtain Assets | 04:03 |
10 | Global CSS | 03:19 |
11 | Title and Favicon | 02:16 |
12 | Install All Libraries (Optional) | 02:05 |
13 | React Router - Info | 02:31 |
14 | React Router - Initial Setup | 04:00 |
15 | Create Pages | 07:04 |
16 | Setup index.js | 05:52 |
17 | Link Component | 03:49 |
18 | Nested Routes | 07:37 |
19 | Error Page | 04:42 |
20 | Styled Components - Intro | 05:12 |
21 | Wrapper | 03:36 |
22 | Landing Page | 08:04 |
23 | Landing Page - CSS (optional) | 11:08 |
24 | Logo Component | 03:02 |
25 | Logo and Images | 01:39 |
26 | Error Page - JSX | 03:55 |
27 | Error Page - CSS (optional) | 03:09 |
28 | Register Page - Setup | 07:48 |
29 | FormRow Component | 07:19 |
30 | Login Page | 04:44 |
31 | Login and Register CSS (optional) | 04:24 |
32 | Dashboard Setup | 03:40 |
33 | Dashboard Structure | 05:12 |
34 | Dashboard CSS (optional) | 03:20 |
35 | Dashboard Context | 09:15 |
36 | React Icons | 02:23 |
37 | Navbar Structure | 04:46 |
38 | Navbar CSS (optional) | 08:04 |
39 | Links Data | 04:35 |
40 | Sidebar Structure | 05:08 |
41 | Sidebar Functionality | 07:35 |
42 | Sidebar CSS (optional) | 11:28 |
43 | NavLinks Component | 02:51 |
44 | Big Sidebar | 05:33 |
45 | Big Sidebar CSS (optional) | 09:53 |
46 | Logout Container | 07:54 |
47 | Logout Container CSS (optional) | 05:55 |
48 | Theme Toggle | 03:48 |
49 | Theme Toggle CSS (optional) | 01:50 |
50 | Dark Theme Logic | 10:15 |
51 | Dark Theme Logic - Bug Fix | 01:34 |
52 | Dark Theme CSS (optional) | 07:49 |
53 | Folder Structure | 05:44 |
54 | ES6 Modules | 05:28 |
55 | Install Server Packages | 03:26 |
56 | Express and Nodemon | 05:24 |
57 | Thunder Client | 03:25 |
58 | JSON Middleware | 04:51 |
59 | Morgan and Dotenv | 10:40 |
60 | New Node Features (optional) | 07:31 |
61 | Get All Jobs | 09:45 |
62 | Create Job | 07:45 |
63 | Get Single Job | 06:34 |
64 | Edit Job | 04:38 |
65 | Delete Job | 04:16 |
66 | Not Found and Error Route | 04:23 |
67 | Not Found vs Error Route | 04:35 |
68 | Controller and Router | 11:53 |
69 | MongoDB | 00:45 |
70 | Atlas Account | 06:10 |
71 | Mongoose | 01:06 |
72 | Connect DB | 03:13 |
73 | Job Model | 05:49 |
74 | Create Job Controller | 07:00 |
75 | Async Errors | 06:01 |
76 | Get All Jobs and Get Single Job | 05:52 |
77 | Delete and Update Job | 06:44 |
78 | Status Codes | 02:31 |
79 | Custom Error | 12:40 |
80 | More Custom Errors | 01:49 |
81 | Validation Layer - Intro | 03:11 |
82 | Express Validator - Setup | 11:12 |
83 | Validation Middleware | 08:16 |
84 | Constants | 05:03 |
85 | Validate Job Input | 09:55 |
86 | Validate ID Params | 06:44 |
87 | Validate Job | 10:29 |
88 | User Model | 03:25 |
89 | User Controller and Router | 04:55 |
90 | Create User | 02:11 |
91 | Validate Register User | 08:50 |
92 | Admin User | 03:22 |
93 | Hash Password | 06:14 |
94 | Hash Utils | 02:58 |
95 | Validate Login | 03:28 |
96 | Login Logic | 07:04 |
97 | JWT | 07:44 |
98 | JWT ENV | 01:55 |
99 | Http Only Cookie | 08:19 |
100 | Authenticate User Setup | 06:17 |
101 | Verify Cookie | 05:01 |
102 | Verify JWT | 05:56 |
103 | Add User to Job Routes | 04:46 |
104 | Validate Owner | 07:13 |
105 | Logout Controller | 03:27 |
106 | User Routes | 08:28 |
107 | Get Current User | 05:09 |
108 | Update User | 07:17 |
109 | Get Application Stats | 06:48 |
110 | Proxy Setup | 09:17 |
111 | Concurrently | 04:12 |
112 | Axios | 07:06 |
113 | React Router Action Intro | 02:13 |
114 | First Action | 07:32 |
115 | Register User Complete | 14:28 |
116 | Navigation State | 03:31 |
117 | React Toastify | 05:41 |
118 | Login User | 07:51 |
119 | UseActionData Hook | 05:49 |
120 | Loaders | 04:29 |
121 | Get Current User | 06:43 |
122 | Logout | 03:01 |
123 | Add Job Structure | 07:35 |
124 | Select Input | 08:15 |
125 | Create Job Functionality | 08:43 |
126 | Add Job CSS (optional) | 05:52 |
127 | All Jobs Structure | 06:30 |
128 | All Jobs Context | 02:05 |
129 | Jobs Container JSX | 03:42 |
130 | Jobs Container CSS (optional) | 02:48 |
131 | Job Component | 10:49 |
132 | Job Component CSS (optional) | 11:35 |
133 | Edit Job - Setup | 06:40 |
134 | Route Params | 05:13 |
135 | Edit Job Loader | 02:57 |
136 | Edit Job JSX | 05:17 |
137 | Edit Job Action | 03:03 |
138 | Delete Job | 07:24 |
139 | Admin Page Setup | 06:43 |
140 | Admin Page Complete | 05:31 |
141 | Admin CSS (optional) | 07:28 |
142 | Avatar Images | 01:10 |
143 | Public Folder | 05:14 |
144 | User Schema Update | 02:08 |
145 | Profile Page Structure | 09:47 |
146 | Profile Action | 07:06 |
147 | Setup Multer | 05:58 |
148 | Cloudinary | 04:58 |
149 | Update User Functionality | 12:17 |
150 | Submit Button Component | 06:30 |
151 | Create Test User | 04:54 |
152 | Restrict Access to Test User | 07:21 |
153 | Mockaroo | 04:38 |
154 | Populate DB | 08:54 |
155 | Stats Route Setup | 07:53 |
156 | Group by Job Status | 09:41 |
157 | Group by Monthly Applications | 09:44 |
158 | Stats Page Setup | 06:33 |
159 | Stats Container | 06:41 |
160 | BarChart Container | 03:14 |
161 | Charts | 08:06 |
162 | Charts Container CSS (optional) | 01:37 |
163 | Query Params | 05:46 |
164 | Search Param | 06:19 |
165 | Job Status and Job Type | 03:46 |
166 | Sort | 05:41 |
167 | Pagination | 11:00 |
168 | Search Form Setup | 09:09 |
169 | Loader and Query Params | 05:45 |
170 | Controlled Inputs | 09:04 |
171 | Debounce | 07:33 |
172 | Pagination Setup | 07:29 |
173 | Render Buttons | 07:11 |
174 | Pagination Logic - First Approach | 08:32 |
175 | Pagination Logic - Complex Approach | 13:57 |
176 | Button Container CSS (optional) | 07:09 |
177 | Local Build | 07:52 |
178 | Render Intro | 00:35 |
179 | Deploy App | 05:30 |
180 | Build Front-End Programmatically | 08:51 |
181 | Update User - Fix | 12:24 |
182 | Global Loading | 04:46 |
183 | React Query - Install | 07:03 |
184 | Page ErrorElement | 07:53 |
185 | First Query with React Query | 11:55 |
186 | React Query in Stats Loader | 09:28 |
187 | React Query - Current User | 04:41 |
188 | Invalidate Queries | 05:30 |
189 | React Query - All Jobs | 09:57 |
190 | React Query - Edit Job | 04:13 |
191 | Axios Interceptors | 06:02 |
192 | Security Packages | 05:49 |
Similar courses to MERN 2024 Edition - MongoDB, Express, React and NodeJS
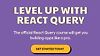
React Query (OLD)ui.dev (ex. Tyler McGinnis)
Duration 7 hours 1 minute 30 seconds
Course
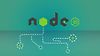
NodeJS - The Complete Guide (incl. MVC, REST APIs, GraphQL)udemyAcademind Pro
Duration 38 hours 51 minutes 43 seconds
Course
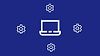
Microservices with NodeJS, React, Typescript and Kubernetesudemy
Duration 95 hours 13 minutes 4 seconds
Course
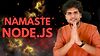
Namaste Node.jsAkshay Saini (NamasteDev.com)
Duration 35 hours 39 minutes 27 seconds
Course

Instagram Clone Coding 3.0Nomad Coders
Duration 20 hours 48 minutes 39 seconds
Course
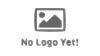
Nodejs Express - unit testing/integration tests with Jestudemy
Duration 2 hours 48 minutes 43 seconds
Course
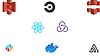
Build a React & Redux App w CircleCI CICD, AWS & Terraformudemy
Duration 25 hours 45 minutes 21 seconds
Course
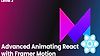
Advanced Animating React with Framer Motionleveluptutorials
Duration 2 hours 57 minutes 30 seconds
Course
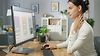
Complete DApp - Solidity & React - Blockchain Developmentudemy
Duration 15 hours 21 minutes 24 seconds
Course