Mastering NestJS - 2024
8h 52m 2s
English
Paid
Discover the dynamic world of server-side web development with this meticulously designed course on Nest.js, created for both beginners and experienced developers aiming to master server-side TypeScript. This course offers a progressive guide that immerses you in practical learning of Nest.js and its modern approaches to development in TypeScript.
Read more about the course
In the course, you will learn how to properly approach the execution of real projects, acquiring skills that can be directly applied in a professional environment.
Key topics of the course:
- Basics of NestJS
- Controllers
- Pipes
- Guards
- Interceptors
- Middleware
- CRUD with MySQL
- TypeORM
- REST API
- Cookies and sessions
- Authentication with JWT
- Encryption with BcryptJS
- Working with MongoDB
- Mongoose
- Practical assignments and much more...
Skills learned:
- Basics of NestJS: Learn fundamental principles such as controllers, pipes, guards, and middleware.
- CRUD operations with MySQL and MongoDB: Master techniques for creating reliable web applications and APIs based on relational and NoSQL databases.
- Interceptors: Learn to use interceptors to handle incoming and outgoing requests, modify request and response data, execute additional logic, or perform global error handling.
Upon completing the course, you will be able to:
- Create scalable and easily maintainable Node.js applications
- Effectively use TypeScript in NestJS projects
- Master key concepts of NestJS architecture
- Develop reliable RESTful APIs
- Implement advanced features such as middleware, pipes, interceptors, and guards
- Write comprehensive tests for your code
Watch Online Mastering NestJS - 2024
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Introduction to NEST JS | 02:26 |
2 | Setting up the project | 02:23 |
3 | Understanding Project Structure | 06:11 |
4 | Understanding Controllers | 05:11 |
5 | Controller Fundamentals(@Get Handler) | 04:22 |
6 | Controller Fundamentals(Headers) | 03:36 |
7 | Controller Fundamentals (@Post Handler) | 08:58 |
8 | Controller Fundamentals (@Post Handler) - Pt 2 with Modules | 06:57 |
9 | Controller Fundamentals (Fetching Params) | 06:45 |
10 | Controller Fundamentals (@Put Handler) | 10:19 |
11 | Controller Fundamentals (@Patch Handler) | 04:09 |
12 | Controller Fundamentals (@Delete Handler) | 03:25 |
13 | Request Object | 04:43 |
14 | Response Object | 02:07 |
15 | Fetching Queries | 03:25 |
16 | @HttpCode Vs. @Res Decorator | 03:23 |
17 | HTTP Response Status | 02:54 |
18 | Introduction To Pipes | 02:00 |
19 | Built-in Pipes(ParseIntPipe) | 02:41 |
20 | Built-in Pipes(ParseFloatPipe) | 01:08 |
21 | Built-in Pipes(ParseBooleanPipe) | 02:26 |
22 | Built-in Pipes(ParseArrayPipe) | 03:00 |
23 | Built-in Pipes (ParseUUIDPipe) | 04:13 |
24 | Built-in Pipes (ValidationPipe) | 05:53 |
25 | Validating empty fields | 03:35 |
26 | Validating Field Length | 03:05 |
27 | Custom Validation Messages | 03:03 |
28 | Validating Field Using @IsEnum() Validator | 04:08 |
29 | Validating Dates In NestJS | 02:32 |
30 | Validating Optional Fields | 02:34 |
31 | Validating Regex Pattern | 03:12 |
32 | Creating A Custom Pipe | 06:40 |
33 | Understanding ArgumentMetadata (metadata.type) | 03:41 |
34 | Assignment - Custom Pipe (Handling different “type” arguments) | 03:57 |
35 | Understanding ArgumentMetadata (metadata.metatype) | 05:02 |
36 | Understanding ArgumentMetadata (metadata.data) | 03:39 |
37 | Implementing Global Pipes | 02:32 |
38 | Introduction to Nest Middlewares | 03:47 |
39 | Implementing Middleware | 03:46 |
40 | Registering a Middleware | 05:21 |
41 | Route-Specific Middleware | 05:49 |
42 | Assignment - Checking Content-type With Middleware | 04:01 |
43 | Handling Route Wildcards | 03:20 |
44 | Middleware For Specific Route Handlers | 04:39 |
45 | Excluding Routes | 03:50 |
46 | Controller-Driven Route Middleware | 02:16 |
47 | Understanding Functional Middleware | 04:27 |
48 | Applying Multiple Middlewares | 06:52 |
49 | Applying Global Middlewares | 03:53 |
50 | Assignment - Password Encryption Middleware | 10:20 |
51 | Introduction To Guards | 04:15 |
52 | Understanding Guard | 05:34 |
53 | Understanding ExecutionContext | 06:51 |
54 | Accessing Arguments With getArgs() | 03:43 |
55 | Limiting Controller Access With Guard | 04:07 |
56 | Understanding switchToHttp() method | 05:00 |
57 | Assignment - API Key Authorization | 06:11 |
58 | Applying Multiple Guards | 03:15 |
59 | Defining Custom Metadata | 05:07 |
60 | Setting Custom Metadata - A Better Way | 03:26 |
61 | Applying Role For Specific Handlers | 10:04 |
62 | Applying Multiple Roles | 01:36 |
63 | Applying Global Guards | 04:47 |
64 | Introduction To Interceptors | 03:01 |
65 | Understanding Interceptor | 05:55 |
66 | Assignment - Transforming Response Data | 04:43 |
67 | Assignment - Modifying Request Headers | 03:50 |
68 | Assignment - Hiding Sensitive Information | 05:51 |
69 | Exception Mapping | 03:24 |
70 | Data Validation With Interceptor | 05:38 |
71 | Authentication and Authorization | 04:25 |
72 | Applying Global Interceptors | 02:40 |
73 | Connecting To MySQL Database Using TypeORM | 05:25 |
74 | Creating Entity With TypeORM | 07:03 |
75 | Inserting Product Data(CRUD) | 08:10 |
76 | Fetching the Product Data(CRUD) | 03:58 |
77 | Updating Product Record(CRUD) | 06:23 |
78 | Deleting Product Record(CRUD) | 03:27 |
79 | Introduction to MyStore Application | 02:44 |
80 | Rendering Template On Server | 04:42 |
81 | Creating Navbar With “includes” | 03:32 |
82 | Creating Home Interface | 09:07 |
83 | Conditional Rendering - No Product Found | 02:27 |
84 | Configuring Add Product Route | 02:30 |
85 | Creating “Add Product” Interface | 05:52 |
86 | Configuring Edit Product Route | 01:50 |
87 | Interface & Functionality - Edit Product | 04:11 |
88 | Fetching The Products | 03:12 |
89 | Adding Product To Database - Part 1 | 02:53 |
90 | Adding Product To Database - Part 2 | 07:54 |
91 | Updating The Product | 07:14 |
92 | Deleting The Product | 01:58 |
93 | Section Introduction | 02:34 |
94 | Setting Up The Sign-up Route | 02:25 |
95 | Designing the Sign-Up Form | 02:54 |
96 | Implementing Show/Hide Password Functionality | 04:41 |
97 | Validating Password | 02:33 |
98 | Registering User To The Database | 06:51 |
99 | Configuring Login Page | 02:13 |
100 | Validating User & Sending Cookie | 04:42 |
101 | Reading A Cookie | 02:14 |
102 | Rendering DOM Based On Login Status | 03:49 |
103 | Implementing Logout | 01:21 |
104 | Configuring Express-Session | 02:55 |
105 | Sending And Reading Session Cookie | 03:41 |
106 | Storing Sessions In MySQL | 03:45 |
107 | Destroying The Session - Logout | 01:43 |
108 | Optimizing Session Storage | 04:16 |
109 | Implementing JWT Token | 06:52 |
110 | Validating Request With Middleware | 05:01 |
111 | Conditional Rendering With Token | 06:15 |
112 | Hashing The Password On SignUp | 03:52 |
113 | Validating Login Credentials | 02:16 |
114 | Finalizing MyStore Application | 04:21 |
115 | Connecting To MongoDB Database | 03:33 |
116 | Creating A Schema | 03:27 |
117 | Registering The Schema | 02:08 |
118 | Create & Save Product To MongoDB | 05:25 |
119 | Fetching Documents From MongoDB | 03:59 |
120 | Updating A Document In MongoDB | 03:30 |
121 | Deleting A Document From MongoDB | 01:52 |
122 | Connecting MyStore With MongoDB Database | 02:28 |
123 | Creating User Schema | 02:04 |
124 | Injecting The User Schema | 01:51 |
125 | Creating Product Schema | 01:47 |
126 | Injecting The Product Schema | 03:20 |
127 | Creating MongoDB Session Store | 04:53 |
Similar courses to Mastering NestJS - 2024
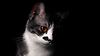
NestJS GraphQL - Code-first approachlearn.nestjs.com
Category: NestJS, GraphQL
Duration 1 hour 55 minutes 15 seconds
Course
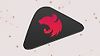
NestJS Zero to Hero - Modern TypeScript Back-end Developmentudemy
Category: NestJS
Duration 8 hours 44 minutes 8 seconds
Course
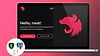
NestJs - Build Modern APIs in NestJs with Unit Testingudemy
Category: NestJS
Duration 9 hours 19 minutes 27 seconds
Course
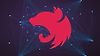
NestJS Microservices: Build & Deploy a Scaleable Backendudemy
Category: NestJS
Duration 9 hours 2 minutes 2 seconds
Course
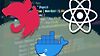
React and NestJS: A Practical Guide with Dockerudemy
Category: React.js, Docker, NestJS
Duration 6 hours 54 minutes 20 seconds
Course
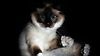
NestJS Advanced Conceptslearn.nestjs.com
Category: NestJS
Duration 1 hour 59 minutes 39 seconds
Course
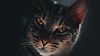
NestJS Fundamentalslearn.nestjs.com
Category: NestJS
Duration 5 hours 17 minutes 22 seconds
Course
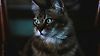
NestJS Microserviceslearn.nestjs.com
Category: NestJS
Duration 1 hour 38 minutes 2 seconds
Course
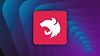
NestJS Masterclass - NodeJS Framework Backend Developmentudemy
Category: NestJS
Duration 24 hours 26 minutes 30 seconds
Course
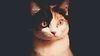
NestJS Authentication and Authorizationlearn.nestjs.com
Category: NestJS
Duration 2 hours 17 minutes 12 seconds
Course