Master Go
A few years ago, I discovered Go and immediately fell in love with this language. I loved how the incredibly clean design of the language, as well as the awesome toolchain, suddenly made coding a breeze. Still, every language able to produce production-level code has some inherent complexity, and soon I found myself dragging pieces of information together, from various tutorials, blogs, forums, books, and other parts of the internet. You bet that these information bits were often incomplete, targeted at a different level than I needed, or even contradicted each other.
More
I really wished I had everything in one place, carefully collected and assembled into a sane curriculum, presented in a pleasant way, with consistent style, easy to digest.
And so I built Master Go, to give you the course that I wish I had. A course designed to be efficient, intuitive, and complete.
Watch Online Master Go
# | Title | Duration |
---|---|---|
1 | Welcome! | 05:29 |
2 | Intro to section 1 | 01:12 |
3 | Installing Git | 00:54 |
4 | Installing Go | 03:49 |
5 | Installing Visual Studio Code | 05:24 |
6 | Get the code | 02:09 |
7 | Your First Go Program | 04:24 |
8 | Go Documentation on golang.org | 06:24 |
9 | The Go Playground | 02:49 |
10 | Intro to section 2 | 00:47 |
11 | Variables | 05:08 |
12 | Control Structures 1 - if | 02:22 |
13 | Control Structures 2 - switch | 01:48 |
14 | Control Structures 3 - for | 04:55 |
15 | A Quick Intro to Using Libraries | 04:38 |
16 | Input and Output 1 - Printing | 03:15 |
17 | Input and Output 2 - Scanning | 04:08 |
18 | Input and Output 3 - Command Line | 01:46 |
19 | Input and Output 4 - Flags | 03:51 |
20 | Exercise: Build Your First Little Commandline Tool! | 02:09 |
21 | Strings 1 - Basics | 05:38 |
22 | Strings 2 - Unicode | 04:40 |
23 | Strings 3 - Literals | 01:58 |
24 | Numeric Data Types 1 - Integers and Booleans | 06:57 |
25 | Numeric Data Types 2 - Floating Point Numbers | 04:02 |
26 | Numeric Data Types 3 - Floating Point Tips | 05:46 |
27 | Numeric Data Types 4 - Complex Numbers | 02:24 |
28 | Constants | 07:27 |
29 | Pointers | 05:57 |
30 | Functions 1 - Declaring Functions | 04:26 |
31 | Functions 2 - Function Behavior (recursion, deferred functions, scope) | 04:19 |
32 | Functions 3 - Function Values and Closures | 06:31 |
33 | Functions 4 - Functions and Pointers | 02:34 |
34 | Error Handling | 08:54 |
35 | Error Inspection | 06:39 |
36 | Packages and Libraries 1 - Using Third-Party Packages | 04:34 |
37 | Packages and Libraries 2 - Creating Custom Packages | 05:37 |
38 | Packages and Libraries 3 - Publish Your Package | 05:14 |
39 | Packages and Libraries 4 - Sub-Packages | 03:16 |
40 | Modules 1 - Definition | 02:06 |
41 | Modules 2 - Creating a Module | 03:06 |
42 | Modules 3 - Add Version Information | 02:34 |
43 | Modules 4 - Using Modules | 06:01 |
44 | Modules 5 - Local Development | 07:20 |
45 | Modules 6 - Dependency Maintenance | 08:22 |
46 | Modules 8 - How Go Selects a Module Version | 08:25 |
47 | Exercise: Bank Account | 06:46 |
48 | Intro to section 3 | 00:46 |
49 | Arrays | 05:19 |
50 | Slices | 06:38 |
51 | Pass-By-Value Semantics seem to break! (Or do they?) | 02:20 |
52 | Byte Slices and Strings | 03:42 |
53 | Maps | 07:31 |
54 | Type Declarations | 03:28 |
55 | Type Aliases | 04:28 |
56 | Struct Basics | 07:20 |
57 | Struct embedding and anonymous fields | 03:09 |
58 | Struct field tags and JSON | 02:38 |
59 | Methods | 08:36 |
60 | Method Sets | 02:46 |
61 | Interfaces 1 - Representing Behavior | 07:44 |
62 | Interfaces 2 - Interfaces as Parameters | 05:36 |
63 | Interfaces 4 - Internals (and a gotcha) | 01:33 |
64 | Generics 1 - Type Parameters | 05:36 |
65 | Generics 2 - Type Constraints | 07:06 |
66 | Generics 3 - Generic Interface Functions | 05:52 |
67 | Exercise: write a Web service - part 1: the Web server | 03:29 |
68 | Exercise: write a Web service - part 2: routing | 02:03 |
69 | Exercise: write a Web service - part 3: handling requests | 03:13 |
70 | Exercise: write a Web service - part 4: the data store | 05:38 |
71 | Intro to section 4 | 01:09 |
72 | Compiling with go run, go build, and go install | 04:38 |
73 | Conditional compilation | 05:37 |
74 | The Go Module Proxy and the Go Sum DB | 05:41 |
75 | Configuring Proxy and Sum Servers | 05:15 |
76 | Formatting your code with go fmt | 02:26 |
77 | Testing with go test | 08:24 |
78 | Testing - Good test design, and how to verify test coverage | 03:27 |
79 | Testing - Subtests, parallel tests, and table-driven tests | 06:41 |
80 | Testing - Fuzzing | 12:27 |
81 | Testing - Benchmarking with go test | 04:31 |
82 | Documentation - Create documentation from code | 03:29 |
83 | Documentation - godoc and go doc | 02:46 |
84 | Intro to section 5 | 00:32 |
85 | Reflection | 09:52 |
Similar courses to Master Go
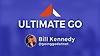
Ultimate Goardanlabs.com
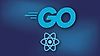
Working with React and Go (Golang)udemy
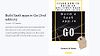
Build SaaS apps in Go (2nd edition)Dominic St-Pierre
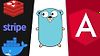
Angular and Golang: A Rapid Guide - Advancedudemy
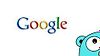
Golang (Google go) udemy
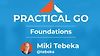
Practical Go Foundationsardanlabs.com
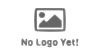
For the Love of Go: Book/Video BundleJohn Arundel
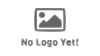