How to develop a productive HTTP client in Golang (Go)
Have you ever called a REST API from your Go program? Did you implemented your own HTTP client or did you ended up using some of the thousand libraries out there? Do you know what your HTTP client is doing in the background? In this course we're starting from scratch! We're going to remember how a basic HTTP call looks like by digging into the request & response objects. We're going to write a basic HTTP client to perform HTTP requests and then use it in productive applications.
Read more about the course
What issues do we have? Can we scale our applications by following this approach? Of course not!
That's why we're creating an HTTP client library that provides:
Fast, reliable and friction-free HTTP connections.
Support for all HTTP methods: GET, POST, PUT, DELETE, PATCH and more!
A Concurrency-Safe HTTP client that you can use without worrying about performance.
Content type management and optimization.
Mocking features out of the box.
A clean interface in case you want to unit test your code without relying on integration testing features.
A robust implementation so you won't need any external dependency whatsoever.
Completely customizable interface: timeouts, transport layer, custom HTTP client and lots of useful features.
A library that is PRODUCTION-READY!
If you're looking to integrate a 3rd party REST APIs in your code, you'll need to perform an HTTP call to it. Make sure you take a look at this course before even considering alternatives out there that will force you to use different dependencies for running, testing and extending your code! As Robert Pike says: "A little copying is much better than a little dependency". In this course we're not only getting rid of the dependencies but we're also getting rid of the copying. We're not using anything more than the Go's standard library to design & develop our own HTTP client.
This client will the baseline for all of the applications we're going to build later, making our business scale and grow as fast as we can Go.
Watch Online How to develop a productive HTTP client in Golang (Go)
# | Title | Duration |
---|---|---|
1 | Introduction | 01:35 |
2 | Welcome! | 02:51 |
3 | The reason for this course | 08:43 |
4 | What we're going to build | 03:15 |
5 | How an HTTP call looks like | 13:23 |
6 | Connections and timeouts | 08:32 |
7 | Implementing a basic HTTP GET | 06:20 |
8 | Default problems | 21:33 |
9 | The reason for a new library | 25:36 |
10 | Introduction to Go modules | 20:18 |
11 | Go basics: Structs, functions, interfaces and methods. | 16:05 |
12 | Adding basic behavior | 13:24 |
13 | Defining custom & common headers | 17:41 |
14 | Dealing with the request body | 13:22 |
15 | Testing, testing and testing! | 18:09 |
16 | Be careful with code coverage | 18:38 |
17 | Dealing with timeouts | 16:07 |
18 | Allow timeout customization | 16:28 |
19 | Allow timeout disabling | 09:05 |
20 | Builder pattern applied | 15:47 |
21 | Refactoring our builder implementation | 06:11 |
22 | Making the client concurrent-safe | 09:04 |
23 | Using our custom response implementation | 18:11 |
24 | Creating our examples | 13:16 |
25 | Should we provide mocking features? | 12:24 |
26 | Defining the Mock struct | 14:12 |
27 | Adding the mock server | 17:35 |
28 | Responding from the mock server | 13:03 |
29 | Adding a default mock | 17:20 |
30 | How to flush every active mock | 09:01 |
31 | Improving mock body and keys | 07:57 |
32 | How to publish a Go module | 08:02 |
33 | How to use our Go module | 09:27 |
34 | Easily testing API calls with our library | 18:43 |
35 | Allowing custom HTTP client | 09:31 |
36 | Clean our public interface | 14:02 |
37 | Adding documentation to our code | 08:40 |
38 | Adding more examples | 21:08 |
39 | Allow user agent definition | 07:56 |
40 | Defining common constants | 06:29 |
41 | Releasing the first stable version! | 07:44 |
42 | Cleaning our mocking interface | 19:05 |
43 | Changing how we mock requests | 23:19 |
44 | Cleaning our mock server | 07:15 |
45 | What we have done | 18:02 |
Similar courses to How to develop a productive HTTP client in Golang (Go)
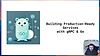
Building Production-Ready Services with gRPC and GoByteSizeGo
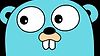
How to design & develop REST microservices in Golang (Go)udemy
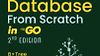
Build Your Own Database in Go From ScratchJames Smith
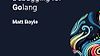
Foundations of Debugging for GolangByteSizeGoMatt Boyle
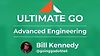
Ultimate Go: Advanced Engineering 2.0ardanlabs.com
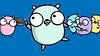
Web Authentication, Encryption, JWT, HMAC, & OAuth With Goudemy
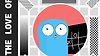
For the Love of Go: Book/Video BundleJohn Arundel
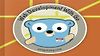