Learn and Understand NodeJS
NodeJS is a rapidy growing web server technology, and Node developers are among the highest paid in the industry. Knowing NodeJS well will get you a job or improve your current one by enabling you to build high quality, robust web applications. In this course you will gain a deep understanding of Node, learn how NodeJS works under the hood, and how that knowledge helps you avoid common pitfalls and drastically improve your ability to debug problems.
Read more about the course
In this course we'll look at how the C++ written V8 Javascript engine works and how NodeJS uses it to expand the abilities of Javascript. You'll learn how to structure your code for reuse and to be easier to understand, manage, and expand using modules and understand how modules really work.
You'll learn how asynchronous code works in Node and the Node event loop, as well as how to use the event emitter, streams, buffers, pipes, and work with files. We'll see how that leads to building a web server in Node.
We'll dive into web sites, web apps and APIs with Express and learn how Express can save us time as Node developers.
You'll also gain an understanding of npm, connecting to databases, and the MEAN stack!
During it all you'll gain a deep understanding of the Javascript concepts and other computer science concepts that power Node.
NodeJS doesn't have to be hard to learn. The biggest mistake most coding tutorials make is expecting someone to learn simply by imitating others' code. Real world situations are never exactly like the tutorial.
I believe the best way to learn is to understand how a tool works and what it does for you, look at examples, and then try it yourself. That's how this course is built, with the goal to help you both learn and understand NodeJS.
Note: In this course you'll also get downloadable source code. You will often be provided with 'starter' code, giving you the base for you to start writing your code, and 'finished' code to compare your code to.
Watch Online Learn and Understand NodeJS
# | Title | Duration |
---|---|---|
1 | Introduction and the Goal of this Course | 04:34 |
2 | Big Words and NodeJS | 01:14 |
3 | Conceptual Aside: The Command Line Interface | 09:50 |
4 | Conceptual Aside: Processors, Machine Language, and C++ | 10:08 |
5 | Javascript Aside: Javascript Engines and The ECMAScript Specification | 04:02 |
6 | V8 Under the Hood | 05:58 |
7 | Adding Features to Javascript | 15:05 |
8 | Conceptual Aside: Servers and Clients | 06:46 |
9 | What Does Javascript Need to Manage a Server? | 02:19 |
10 | The C++ Core | 05:52 |
11 | The Javascript Core | 03:05 |
12 | Let's Install and Run Some Javascript in Node | 16:48 |
13 | Conceptual Aside: Modules | 02:22 |
14 | Javascript Aside: First-Class Functions and Function Expressions | 12:04 |
15 | Let's Build a Module | 10:55 |
16 | Javascript Aside: Objects and Object Literals | 06:56 |
17 | Javascript Aside: Prototypal Inheritance and Function Constructors | 11:39 |
18 | Javascript Aside: By Reference and By Value | 05:45 |
19 | Javascript Aside: Immediately Invoked Function Expressions (IIFEs) | 07:43 |
20 | How Do Node Modules Really Work?: module.exports and require | 17:34 |
21 | Javascript Aside: JSON | 01:41 |
22 | More on require | 11:40 |
23 | Module Patterns | 19:13 |
24 | exports vs module.exports | 10:03 |
25 | Requiring Native (Core) Modules | 06:52 |
26 | Modules and ES6 | 02:37 |
27 | Web Server Checklist | 01:22 |
28 | Conceptual Aside: Events | 05:25 |
29 | Javascript Aside: Object Properties, First Class Functions, and Arrays | 05:09 |
30 | The Node Event Emitter - Part 1 | 13:59 |
31 | The Node Event Emitter - Part 2 | 11:59 |
32 | Javascript Aside: Object.create and Prototypes | 06:19 |
33 | Inheriting From the Event Emitter | 14:41 |
34 | Javascript Aside: Node, ES6, and Template Literals | 07:56 |
35 | Javascript Aside: .call and .apply | 03:41 |
36 | Inheriting From the Event Emitter - Part 2 | 09:44 |
37 | Javascript Aside: ES6 Classes | 08:44 |
38 | Inheriting From the Event Emitter - Part 3 | 06:01 |
39 | Javascript Aside: Javascript is Synchronous | 02:39 |
40 | Conceptual Aside: Callbacks | 01:30 |
41 | libuv, The Event Loop, and Non-Blocking Asynchronous Execution | 11:40 |
42 | Conceptual Aside: Streams and Buffers | 04:32 |
43 | Conceptual Aside: Binary Data, Character Sets, and Encodings | 11:10 |
44 | Buffers | 06:49 |
45 | ES6 Typed Arrays | 04:53 |
46 | Javascript Aside: Callbacks | 04:04 |
47 | Files and fs | 15:53 |
48 | Streams | 18:15 |
49 | Conceptual Aside: Pipes | 02:15 |
50 | Pipes | 15:52 |
51 | Web Server Checklist | 02:38 |
52 | Conceptual Aside: TCP/IP | 07:56 |
53 | Conceptual Aside: Addresses and Ports | 03:12 |
54 | Conceptual Aside: HTTP | 06:07 |
55 | http_parser | 06:57 |
56 | Let's Build a Web Server in Node | 17:27 |
57 | Outputting HTML and Templates | 11:44 |
58 | Streams and Performance | 05:12 |
59 | Conceptual Aside: APIs and Endpoints | 02:38 |
60 | Outputting JSON | 06:40 |
61 | Routing | 11:03 |
62 | Web Server Checklist | 02:25 |
63 | Conceptual Aside: Packages and Package Managers | 03:08 |
64 | Conceptual Aside: Semantic Versioning (semver) | 04:47 |
65 | npm and the npm registry: Other People's Code | 05:00 |
66 | init, nodemon, and package.json | 13:03 |
67 | init, nodemon, and package.json - Part 2 | 15:18 |
68 | Using Other People's Code | 01:44 |
69 | Installing Express and Making it Easier to Build a Web Server | 16:02 |
70 | Routes | 04:33 |
71 | Static Files and Middleware | 14:59 |
72 | Templates and Template Engines | 15:55 |
73 | Querystring and Post Parameters | 18:22 |
74 | RESTful APIs and JSON | 05:22 |
75 | Structuring an App | 13:46 |
76 | Conceptual Aside: Relational Databases and SQL | 03:49 |
77 | Node and MySQL | 09:29 |
78 | Conceptual Aside: NoSQL and Documents | 03:13 |
79 | MongoDB and Mongoose | 10:51 |
80 | Web Server Checklist | 01:08 |
81 | MongoDB, Express, AngularJS, and NodeJS | 06:59 |
82 | AngularJS: Managing the Client | 10:33 |
83 | AngularJS: Managing the Client (Part 2) | 08:10 |
84 | AngularJS: Managing the Client (Part 3) | 19:18 |
85 | Conceptual Aside: Angular 1, Angular 2, React, and more… | 03:07 |
86 | Working with The Full Stack (and being a Full Stack Developer) - Part 1 | 12:00 |
87 | NodeTodo: Software Requirements | 02:21 |
88 | Initial Setup | 03:39 |
89 | Setting up Mongo and Mongoose | 09:10 |
90 | Adding Seed Data | 09:32 |
91 | Creating our API | 13:34 |
92 | Testing our API | 16:18 |
93 | Adding a Front-End in Angular 2 (Part 1) | 23:23 |
Similar courses to Learn and Understand NodeJS
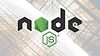
Node.js API - making it shine!udemy
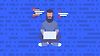
Full-Stack Web Developer Bootcamp with Real Projectsudemy
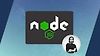
Understanding Node.js: Core Conceptsudemy
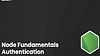
Node Fundamentals Authenticationleveluptutorials
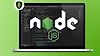
Node.js, Express, MongoDB Bootcamp 2020 - with Real Projectsudemy
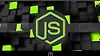
Node.js, Express & MongoDB Dev to Deploymentudemy
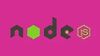
Node.js for Beginners - Become a NodeJs Developer + Projectudemy
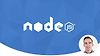
The Complete Node.js Developer Course (3rd Edition)udemy
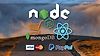