MERN Stack - React Node from Scratch Building Social Network
Welcome to Become a FullStack / MERN Stack JavaScript Developer from Scratch with React, Node JS and MongoDB. In this course you will Learn React with Node JS from Absolute Scratch – Build A Complete MERN Stack Social Network from Scratch and Deploy to the Cloud Hosting.
More
In this course you will learn:
Node JS From Scratch
Node JS API Development from Scratch
React JS from Scratch
Modern JavaScript from Scratch
A FullStack Social Network Application from Scratch
Build Rock Solid Authentication with Password Forgot/Password Reset using JWT
Authorization
Implement Social Login using JWT
CRUD, Image Upload, User Posts Relationships, follow, unfollow, likes, comments and more
Super Admin based on Role
Custom reCAPTCHA
Pagination
Deploy FullStack React Node Social Network to Digital Ocean Cloud Hosting
Full Source Code is available for each major section and lectures
Direct help from Instructor if you ever get stuck!
In depth understanding of Modern JavaScript, React and Node JS
Each line of code is explained!
Easy to understand (Course starts from absolute basic and gradually makes progress)
Follow the best practices while coding
Fully understand the code you are writing
Best way of structuring Node Js and React application so that it scales in future
Watch Online MERN Stack - React Node from Scratch Building Social Network
# | Title | Duration |
---|---|---|
1 | What is node js | 02:19 |
2 | Why learn node js | 02:21 |
3 | Installing node js | 02:40 |
4 | Javascript in browser environment | 03:16 |
5 | Javascript in node js environment | 02:27 |
6 | Getting started with node js | 02:55 |
7 | Writing functions | 03:44 |
8 | Import export | 06:27 |
9 | Using arrow functions | 05:38 |
10 | Object destructuring | 02:02 |
11 | Using node js core modules | 03:30 |
12 | Using npm packages | 08:16 |
13 | Using express | 05:29 |
14 | Node js event loop | 03:07 |
15 | Programming for event loop | 04:29 |
16 | Asynchronous programming | 08:14 |
17 | Synchronous programming | 04:25 |
18 | Functional approach | 03:47 |
19 | Secrets of understanding node js | 02:38 |
20 | Creating server with express | 07:16 |
21 | Separating routes | 05:05 |
22 | Middleware explained | 06:50 |
23 | Using controllers | 07:14 |
24 | Json and postman | 04:33 |
25 | Signup with mlab to use mongodb | 11:32 |
26 | Connecting to database using mongoose | 05:42 |
27 | Post schema | 04:53 |
28 | Creating a post | 12:59 |
29 | Validation and friendly error messages | 14:27 |
30 | Getting posts | 06:37 |
31 | Whats next and cleanup | 03:04 |
32 | User schema | 05:08 |
33 | Virtual fields and methods | 12:49 |
34 | User signup using async await | 12:16 |
35 | User signin validation and error messaging | 09:49 |
36 | User signin flow | 09:41 |
37 | User signin with jwt | 13:33 |
38 | Testing user signin | 03:43 |
39 | Signout method | 03:27 |
40 | Protecting routes | 06:39 |
41 | Handling unauthorized error | 02:59 |
42 | Implementing authorization | 05:23 |
43 | Find user by id and add to req object | 06:35 |
44 | Has authorization method | 03:59 |
45 | Apply require signin to create post | 02:01 |
46 | Showing all users | 08:49 |
47 | Showing single user | 07:49 |
48 | Update user | 10:51 |
49 | Delete user | 06:22 |
50 | User post relationship with post schema | 06:46 |
51 | Create post with image upload and user | 11:13 |
52 | Testing create post | 09:34 |
53 | Get all posts with user | 02:36 |
54 | Get all posts by user | 07:31 |
55 | Post update delete flow | 02:53 |
56 | Post by id based on route param | 03:51 |
57 | Delete post | 10:39 |
58 | Update post | 05:59 |
59 | Whats next | 03:59 |
60 | Documenting api | 09:11 |
61 | Adding cors | 03:41 |
62 | Modern javascript | 01:54 |
63 | Creating variables using const | 04:06 |
64 | Creating variables using let | 03:48 |
65 | Template strings | 05:10 |
66 | Default parameters | 03:25 |
67 | Arrow functions | 05:58 |
68 | Arrow functions and this keyword | 10:11 |
69 | Destructuring object | 08:14 |
70 | Destructuring array | 02:09 |
71 | Restructuring | 04:57 |
72 | Spread and rest operators | 07:32 |
73 | Class constructor super | 14:23 |
74 | Installing react | 05:24 |
75 | React files and folders introduction | 06:23 |
76 | Storing data in component state via ajax call | 10:59 |
77 | Rendering state data using map | 05:48 |
78 | Conditional rendering | 04:18 |
79 | Imports exports props | 09:36 |
80 | Handling click events | 07:40 |
81 | Destructuring inline styling and keys | 04:24 |
82 | Create react project | 04:45 |
83 | Using react router dom | 09:30 |
84 | Adding pages | 02:51 |
85 | Signup form | 04:25 |
86 | Handling onChange events | 08:46 |
87 | User signup | 11:02 |
88 | Code refactoring | 06:03 |
89 | Showing validation and success message | 08:48 |
90 | Code refactoring signup page | 03:57 |
91 | Signin page | 08:07 |
92 | User signin | 10:56 |
93 | Loading... | 05:05 |
94 | Menu component | 04:20 |
95 | Styling and active link | 08:49 |
96 | Signout | 13:00 |
97 | Conditional rendering of signup signin links | 08:09 |
98 | Show user name | 02:48 |
99 | Code refactoring auth logic | 05:29 |
100 | Profile page | 07:07 |
101 | Showing user info from local storage | 05:40 |
102 | Using .env variables | 03:51 |
103 | Fetch user profile | 13:46 |
104 | Code refactoring fetch user | 08:19 |
105 | Show edit profile delete profile buttons | 06:45 |
106 | Active link user profile | 02:42 |
107 | Whats next? | 01:45 |
108 | Users component | 02:30 |
109 | Populate users in state | 04:22 |
110 | Loop through users | 06:08 |
111 | Style user cards | 06:18 |
112 | Default profile image | 03:39 |
113 | All users profile page | 08:37 |
114 | Delete profile component | 02:15 |
115 | Users profile based on props change | 03:25 |
116 | Delete account prompt | 03:58 |
117 | Delete user account | 07:35 |
118 | Edit profile component | 07:09 |
119 | Pre profile edit profile form | 05:30 |
120 | Update user profile | 09:51 |
121 | Client side validation on profile update | 09:18 |
122 | Private route for authenticated users only | 08:58 |
123 | Profile photo upload | 11:00 |
124 | Loading... on edit profile | 03:53 |
125 | Node API - Update profile with image | 13:44 |
126 | File size validation | 04:22 |
127 | Node API - Get user photo with separate route | 05:45 |
128 | Display profile image in edit profile page | 05:18 |
129 | Default image and profile image on all pages | 09:21 |
130 | User about field | 04:52 |
131 | Update user info in local storage | 07:34 |
132 | Whats next? | 03:03 |
133 | Following and followers - User schema and userById method | 05:54 |
134 | Following and followers - Routes and controller methods | 09:46 |
135 | Remove following and remove followers - Routes and controller methods | 03:44 |
136 | Follow Profile Buttons Component | 04:41 |
137 | Check follow or not | 08:08 |
138 | Implement follow | 10:55 |
139 | Implement unfollow | 04:49 |
140 | Profile tabs component | 06:02 |
141 | Display followers list | 07:34 |
142 | Display following list | 05:22 |
143 | Node API - Who to follow? | 06:49 |
144 | Find people component | 08:55 |
145 | Find people and follow | 10:19 |
146 | Starting with posts | 02:58 |
147 | Create new post | 18:32 |
148 | Show all posts in home page | 08:39 |
149 | Show post's user date and excerpt | 12:38 |
150 | Node API - Post image | 03:45 |
151 | Show posts with image | 04:52 |
152 | Single post component | 03:25 |
153 | Load single post in state | 06:15 |
154 | Display single post | 06:40 |
155 | Show loading on single post and posts | 03:04 |
156 | Posts by user | 08:15 |
157 | Display posts by user | 04:29 |
158 | Show update delete buttons | 06:48 |
159 | Delete post | 07:24 |
160 | Delete post prompt | 01:52 |
161 | Update post component | 04:48 |
162 | Implement update post | 17:23 |
163 | Update post photo and error messaging | 05:36 |
164 | Whats next? | 02:13 |
165 | Node API - Implement like unlike | 09:45 |
166 | React frontend - Like Unlike methods | 03:21 |
167 | Implement like unlike in frontend | 15:28 |
168 | Like unlike styling | 04:38 |
169 | Like signin redirect | 04:46 |
170 | Comments backend | 08:56 |
171 | React comment uncomment methods | 03:21 |
172 | Adding comments | 14:54 |
173 | Render comments | 12:04 |
174 | Comment uncomment and validations | 13:04 |
175 | Deploy Node JS API - Signup and super user | 13:44 |
176 | Running API in Digital Ocean | 12:09 |
177 | Using mongoDB in Digital Ocean | 07:02 |
178 | Deploy React SPA to Digital Ocean | 10:15 |
179 | Own reCAPTCHA? | 02:18 |
180 | Super Admin Overview | 01:27 |
181 | Implementing role to users | 11:44 |
182 | Making a user admin using command line | 11:40 |
183 | Admin can update and delete anyone's post | 06:44 |
184 | Admin can update and delete any user | 12:45 |
185 | How to download source code from Github and checkout different commits | 07:30 |
Similar courses to MERN Stack - React Node from Scratch Building Social Network
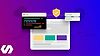
Microservices with Node JS and ReactudemyStephen Grider
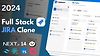
Build a Jira cloneCode With Antonio
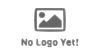
MongoDB - The Ultimate Administration and Developer's Guideudemy
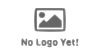
Node.js: The Complete Guide to Build RESTful APIscodewithmosh (Mosh Hamedani)
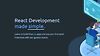
React Formula - Learn Frontend DevelopmentAlvin Zablan
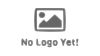
Server Side Rendering with React and ReduxudemyStephen Grider
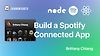
Build a Spotify Connected Appfullstack.io
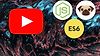
Youtube cloneNomad Coders
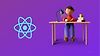