Full Stack Isomorphic JavaScript with Vue.js & Node.js
Learn to build full-stack isomorphic JavaScript web applications with Vue and Node.js along with the MEVN stack. The MEVN stack is a collection of great tools—MongoDB, Express.js, Vue.js, and Node.js—that provide a strong base for a developer to build easily maintainable web applications. With each of them a JavaScript or JavaScript-based technology, having a shared programming language means it takes less time to develop applications.
More
This course enables you to build, test, and deploy full stack isomorphic applications with Node.js, Express, Vue.js, and MongoDB. You will start by setting up the development environment and grasping some of the essential concepts for building applications. You will then build a single page application using MEVN stack. You will also develop a songs playlist application wherein you will create a new playlist and search through YouTube videos. Next, you will learn to consume data from third-party APIs within your application as well as perform CRUD operations against a locally hosted API using the HTTP client Axios. Finally, you will learn to deploy the applications on the cloud.
Watch Online Full Stack Isomorphic JavaScript with Vue.js & Node.js
# | Title | Duration |
---|---|---|
1 | The Course Overview | 01:21 |
2 | What You Should Know First | 00:58 |
3 | Single-Page Applications and Vue.js | 04:06 |
4 | NoSQL and MongoDB | 02:19 |
5 | Node.js and Express.js | 01:42 |
6 | Installing Node.js and Node Package Manager (NPM) | 01:10 |
7 | Installing Vue.js Command Line Interface (CLI) Project Generator | 00:52 |
8 | Installing MongoDB | 03:46 |
9 | Installing Integrated Development Environment (IDE) | 00:54 |
10 | Create Vue.js Application | 01:46 |
11 | Project Directory and Structure | 02:45 |
12 | Vue.js Components | 14:57 |
13 | Vue.js Router | 06:39 |
14 | Vue.js Navigation Guards | 03:57 |
15 | Vuex State Management | 10:21 |
16 | Create Server with Express.js | 08:31 |
17 | Learn to Use Express.js Router | 05:51 |
18 | Use Express.js Middleware and CORS Configuration | 06:43 |
19 | RESTful Endpoints with HTTP Controllers | 07:52 |
20 | Check HTTP Status Codes | 03:18 |
21 | Connect to MongoDB and Use MongoDB Compass GUI | 02:41 |
22 | Working with Entity Models | 04:28 |
23 | Create, Read, Update, and Delete (CRUD) | 08:09 |
24 | Encrypt Passwords with Node.js and BCrypt | 02:56 |
25 | Validate Passwords | 08:40 |
26 | JSON Web Tokens | 03:13 |
27 | Vue.js and JSON Web Tokens | 01:19 |
28 | User Authentication | 03:07 |
29 | Validating a User’s Session | 03:55 |
30 | Managing User's Session | 03:16 |
31 | Connecting Vue.js to Backend Server | 25:33 |
32 | Getting Ready for Production | 03:20 |
33 | Amazon Web Services and Amazon Elastic Compute Cloud | 03:49 |
34 | Deploying App to the Web | 05:06 |
35 | Final Thoughts | 03:50 |
36 | The Course Overview | 03:33 |
37 | First Step Towards Vue | 02:41 |
38 | Setting Up Vue Using Node.js | 04:55 |
39 | Basics, Directives, and Reactivity | 06:53 |
40 | Vue DevTools | 05:35 |
41 | Installing Vue-CLI and Creating a New Project | 03:54 |
42 | Tour of Project Files and What Are Single-File Components? | 04:13 |
43 | Using Axios to Consume a Third-Party API | 05:55 |
44 | Creating Custom Components and Importing Them | 05:23 |
45 | Installing Vuex and vue-router and Adding Them to the Vue Instance | 04:02 |
46 | Vue Core Concepts and How to Implement – Part 1 | 06:05 |
47 | Vue Core Concepts and How to Implement – Part 2 | 05:30 |
48 | How to Use vue-router and Router Links | 06:46 |
49 | Application Structure – Part 1 | 06:33 |
50 | Application Structure – Part 2 | 02:34 |
51 | Putting Together the Sample API Code and Adding to the Frontend Application | 08:43 |
52 | How to Set Up the Database | 05:54 |
53 | Demonstration of API in REST Client | 04:08 |
54 | Creating an Account and Then Authenticating /Getting a Token – Part 1 | 05:31 |
55 | Creating an Account and Then Authenticating /Getting a Token – Part 2 | 05:19 |
56 | Setup Login Page, Functionality and Adding the User – Part 1 | 05:06 |
57 | Setup Login Page, Functionality and Adding the User – Part 1 - 2 | 04:29 |
58 | Using the API to Create, Read, Update, and Delete Items – Part 1 | 08:14 |
59 | Using the API to Create, Read, Update, and Delete Items – Part 2 | 05:06 |
60 | Using the API to Create, Read, Update, and Delete Items – Part 3 | 07:12 |
61 | Using the API to Create, Read, Update, and Delete Items – Part 4 | 07:08 |
62 | Using the Vuex Store as a Single Point of Contact to the API | 04:57 |
63 | Testing | 08:01 |
64 | Security | 06:19 |
Similar courses to Full Stack Isomorphic JavaScript with Vue.js & Node.js
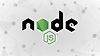
Mastering NodeJS with Interview Questions 2024udemy
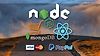
React Node FullStack - Ecommerce from Scratch to Deploymentudemy
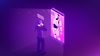