JavaScript Basics for Beginners
6h 39m 44s
English
Paid
JavaScript is one of the most popular programming languages in the world. Companies like Walmart, Netflix, and PayPal run big internal applications around JavaScript. If you're looking for a career in web or mobile app development, you MUST know JavaScript well. This course is the ideal starting point for anyone who wants to master the fundamentals of JavaScript. It breaks down modern JavaScript into digestible and easy-to-understand pieces using real-world examples, exercises and step-by-step solutions. No foo-bar-baz nonsense or fluff here!
Watch Online JavaScript Basics for Beginners
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Welcome | 00:29 |
2 | What is JavaScript? | 04:41 |
3 | Setting Up the Development | 03:10 |
4 | JavaScript in Browsers | 03:48 |
5 | Separation of Concerns | 02:05 |
6 | JavaScript in Node | 01:51 |
7 | Variables | 05:37 |
8 | Constants | 01:45 |
9 | Primitive Types | 03:11 |
10 | Dynamic Typing | 03:18 |
11 | Objects | 05:15 |
12 | Arrays | 04:19 |
13 | Functions | 04:40 |
14 | Types of Functions | 03:17 |
15 | JavaScript Operators | 00:41 |
16 | Arithmetic Operators | 03:40 |
17 | Assignment Operators | 01:45 |
18 | Comparison Operators | 02:02 |
19 | Equality Operators | 03:12 |
20 | Ternary Operators | 02:11 |
21 | Logical Operators with Non-booleans | 05:31 |
22 | Logical Operators with Non-Booleans | 05:54 |
23 | BitWise Operators | 08:29 |
24 | Operator Precedence | 01:15 |
25 | Exercise- Swapping Variables | 02:27 |
26 | If...else | 05:24 |
27 | Switch...case | 04:42 |
28 | For | 05:51 |
29 | While | 02:12 |
30 | Do...While | 03:30 |
31 | Infinite Loops | 02:43 |
32 | For...In | 04:26 |
33 | For...of | 01:16 |
34 | Break and Continue | 02:35 |
35 | Max of Two Numbers | 04:00 |
36 | Exercise- Landscape or Portrait | 02:26 |
37 | Exercise- FizzBuzz | 06:40 |
38 | Exercise- Demerit Points | 09:06 |
39 | Exercise- Even and Odd Numbers | 02:03 |
40 | Exercise- Count Truthy | 04:34 |
41 | Exercise- String Properties | 02:59 |
42 | Exercise- Sum of Multiples 3 and 5 | 02:41 |
43 | Exercise - Grade | 06:33 |
44 | Exercise - Stars | 02:34 |
45 | Exercise - Prime Numbers | 08:25 |
46 | Basics | 04:54 |
47 | Factory Functions | 05:45 |
48 | Constructor Functions | 05:49 |
49 | Dynamic Nature of Objects | 02:04 |
50 | Constructor Property | 02:25 |
51 | Functions are Objects | 04:48 |
52 | Value vs. Reference Types | 05:50 |
53 | Enumerating Properties of an Object | 05:10 |
54 | Cloning an Object | 04:22 |
55 | Garbage Collection | 01:15 |
56 | Math | 02:57 |
57 | String | 06:26 |
58 | Template Literals | 04:53 |
59 | Date | 04:01 |
60 | Exercise 1- Address Object | 01:42 |
61 | Exercise- Factory and Constructor Function | 03:03 |
62 | Exercise 3- Object Equality | 04:00 |
63 | Exercise 4- Blog Post Object | 02:16 |
64 | Exercise 5- Constructor Functions | 02:53 |
65 | Exercise 6- Price Range Objects | 03:38 |
66 | Introduction | 00:32 |
67 | Adding Elements | 03:36 |
68 | Finding Elements (Primitives) | 03:33 |
69 | Finding Elements (Reference Types) | 05:47 |
70 | Arrow Functions | 01:35 |
71 | Removing Elements | 03:04 |
72 | Emptying an Array | 04:15 |
73 | Combining and Slicing Arrays | 03:41 |
74 | The Spread Operator | 02:26 |
75 | Iterating an Array | 02:33 |
76 | Joining Arrays | 03:12 |
77 | Sorting Arrays | 06:33 |
78 | Testing the Elements of an Array | 03:45 |
79 | Filtering an Array | 02:47 |
80 | Mapping an Array | 07:48 |
81 | Reducing an Array | 06:44 |
82 | Exercise 1- Array from Range | 01:51 |
83 | Exercise 2- Includes | 01:55 |
84 | Exercise 3- Except | 02:09 |
85 | Exercise 4- Moving an Element | 06:32 |
86 | Exercise 5- Count Occurrences | 04:32 |
87 | Exercise 6- Get Max | 06:15 |
88 | Exercise 7- Movies | 04:39 |
89 | Function Declarations vs. Expressions | 03:19 |
90 | Hoisting | 01:57 |
91 | Arguments | 04:16 |
92 | The Rest Operator | 04:39 |
93 | Default Parameters | 03:41 |
94 | Getters and Setters | 05:39 |
95 | Try and Catch | 05:36 |
96 | Local vs. Global Scope | 04:45 |
97 | Let vs Var | 05:53 |
98 | The This Keyword | 07:23 |
99 | Changing This | 07:01 |
100 | Exercise 1- Sum of Arguments | 03:43 |
101 | Exercise 2- Area of Circle | 01:54 |
102 | Exercise 3- Error Handling | 02:50 |
Similar courses to JavaScript Basics for Beginners
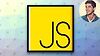
JavaScript: The Advanced Conceptsudemyzerotomastery.io
Category: JavaScript
Duration 25 hours 9 minutes 23 seconds
Course
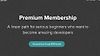
Premium Javascript (Premium membership)Watch and code
Category: JavaScript
Duration 63 hours 55 minutes 37 seconds
Course
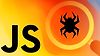
Mastering JavaScript Unit Testingcodewithmosh (Mosh Hamedani)
Category: JavaScript
Duration 3 hours 51 minutes 31 seconds
Course
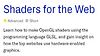
Shaders for the Websuperhi.com
Category: JavaScript, Three.js, OpenGL Shading Language (GLSL)
Duration 16 hours 3 minutes 4 seconds
Course
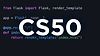
CS50's Web Programming with Python and JavaScriptHarvardX (Harvard University)
Category: JavaScript, Python
Duration 14 hours 3 minutes 25 seconds
Course
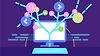
100 Days Of Code: The Complete Web Development Bootcamp 2024Academind Pro
Category: JavaScript, Sql, HTML, CSS, Node.js, Vue
Duration 78 hours 51 minutes 55 seconds
Course
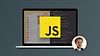
The Complete JavaScript Course 2023: From Zero to Expert!udemy
Category: JavaScript
Duration 68 hours 53 minutes 44 seconds
Course