The Complete JavaScript Course 2023: From Zero to Expert!
This is a truly complete JavaScript course, that goes beyond what other JavaScript courses out there teach you.
I will take you from a complete JavaScript beginner to an advanced developer. You will not just learn the JavaScript language itself, you will also learn how to program. How to solve problems. How to structure and organize code using common JavaScript patterns.
Come with me on a journey with the goal of truly understanding the JavaScript language. And I explain everything on the way with great detail!
Read more about the course
You will learn "why" something works in JavaScript, not just "how". Because in the modern JavaScript world of today, you need more than just knowing how something works. You need to debug code, you need to understand code, you need to be able to think about code.
To achieve our goal together, the course contains coding sessions, coding challenges, theory lectures, real-world projects and a final course exam.
This course is different because it's not just about writing code, it's also about how and why code works the way it does. Because it's the perfect mix between theory and practice. Because it focuses not only on small coding examples, but also on real-world projects and use cases.
So, by the end of the course, you will be a capable JavaScript developer, able to write, understand and debug JavaScript code using all the powerful features the language offers to us.
Here is exactly what we cover in this course:
• All the JavaScript and programming fundamentals: things like variables, data types, boolean logic, if/else statements, loops, functions, objects, arrays, and more.
• Everything you need to know in order to gain a deep understanding of how JavaScript works behind the scenes: execution contexts, hoisting, scoping, the 'this' keyword, and more.
• How to make JavaScript code interact with webpages: DOM manipulation. Learn how to select and change webpage elements, create new elements and handle DOM events.
• Complex JavaScript features such as function constructors, prototypal inheritance, first-class functions, closures, the bind and apply methods, and more.
• We are going to code 3 beautiful real-world apps to apply our knowledge and learn new concepts (I provide the starter HTML and CSS code for these projects).
• Learn how to organize and structure your code using modules and functions, how to create data privacy and encapsulation, and why that's so important.
• What's new in the most modern version of JavaScript: new features of ES6 / ES2015.
• Asynchronous JavaScript: the event loop, promises, async/await, AJAX calls and APIs.
• Modern JavaScript in 2018: Learn how to set up a modern development workflow with NPM, Webpack, Babel and ES6 modules.
Watch Online The Complete JavaScript Course 2023: From Zero to Expert!
# | Title | Duration |
---|---|---|
1 | Course Structure and Projects | 05:26 |
2 | Watch Before You Start! | 06:10 |
3 | Setting Up Our Code Editor | 08:24 |
4 | Section Intro | 00:54 |
5 | Hello World! | 05:58 |
6 | A Brief Introduction to JavaScript | 11:19 |
7 | Linking a JavaScript File | 15:56 |
8 | Values and Variables | 16:06 |
9 | Data Types | 19:20 |
10 | let, const and var | 09:59 |
11 | Basic Operators | 19:32 |
12 | Operator Precedence | 11:20 |
13 | Coding Challenge #1 | 10:29 |
14 | Strings and Template Literals | 10:59 |
15 | Taking Decisions: if / else Statements | 12:51 |
16 | Coding Challenge #2 | 06:22 |
17 | Type Conversion and Coercion | 16:41 |
18 | Truthy and Falsy Values | 10:04 |
19 | Equality Operators: == vs. === | 15:42 |
20 | Boolean Logic | 08:31 |
21 | Logical Operators | 10:38 |
22 | Coding Challenge #3 | 15:08 |
23 | The switch Statement | 13:11 |
24 | Statements and Expressions | 06:09 |
25 | The Conditional (Ternary) Operator | 10:03 |
26 | Coding Challenge #4 | 09:13 |
27 | JavaScript Releases: ES5, ES6+ and ESNext | 14:20 |
28 | Section Intro | 00:37 |
29 | Activating Strict Mode | 10:28 |
30 | Functions | 19:09 |
31 | Function Declarations vs. Expressions | 10:40 |
32 | Arrow Functions | 09:53 |
33 | Functions Calling Other Functions | 10:08 |
34 | Reviewing Functions | 15:38 |
35 | Coding Challenge #1 | 18:13 |
36 | Introduction to Arrays | 21:35 |
37 | Basic Array Operations (Methods) | 12:53 |
38 | Coding Challenge #2 | 09:37 |
39 | Introduction to Objects | 06:09 |
40 | Dot vs. Bracket Notation | 19:22 |
41 | Object Methods | 23:06 |
42 | Coding Challenge #3 | 13:00 |
43 | Iteration: The for Loop | 11:12 |
44 | Looping Arrays, Breaking and Continuing | 22:03 |
45 | Looping Backwards and Loops in Loops | 11:54 |
46 | The while Loop | 11:53 |
47 | Coding Challenge #4 | 15:36 |
48 | Pathways and Section Roadmaps | 04:09 |
49 | Section Intro | 00:40 |
50 | Setting up Prettier and VS Code | 16:23 |
51 | Installing Node.js and Setting Up a Dev Environment | 11:33 |
52 | Learning How to Code | 17:43 |
53 | How to Think Like a Developer: Become a Problem Solver! | 10:54 |
54 | Using Google, StackOverflow and MDN | 26:38 |
55 | Debugging (Fixing Errors) | 05:10 |
56 | Debugging with the Console and Breakpoints | 19:26 |
57 | Coding Challenge #1 | 15:25 |
58 | Section Intro | 00:41 |
59 | Basic HTML Structure and Elements | 09:33 |
60 | Attributes, Classes and IDs | 13:10 |
61 | Basic Styling with CSS | 12:01 |
62 | Introduction to the CSS Box Model | 20:58 |
63 | Section Intro | 00:50 |
64 | PROJECT #1: Guess My Number! | 08:48 |
65 | What's the DOM and DOM Manipulation | 06:49 |
66 | Selecting and Manipulating Elements | 08:23 |
67 | Handling Click Events | 12:51 |
68 | Implementing the Game Logic | 19:34 |
69 | Manipulating CSS Styles | 08:19 |
70 | Coding Challenge #1 | 11:41 |
71 | Implementing Highscores | 07:48 |
72 | Refactoring Our Code: The DRY Principle | 15:06 |
73 | PROJECT #2: Modal Window | 13:22 |
74 | Working With Classes | 16:56 |
75 | Handling an "Esc" Keypress Event | 14:12 |
76 | PROJECT #3: Pig Game | 17:09 |
77 | Rolling the Dice | 15:59 |
78 | Switching the Active Player | 15:17 |
79 | Holding Current Score | 24:56 |
80 | Resetting the Game | 15:39 |
81 | Section Intro | 01:32 |
82 | An High-Level Overview of JavaScript | 12:12 |
83 | The JavaScript Engine and Runtime | 13:48 |
84 | Execution Contexts and The Call Stack | 17:46 |
85 | Scope and The Scope Chain | 25:38 |
86 | Scoping in Practice | 21:14 |
87 | Variable Environment: Hoisting and The TDZ | 11:01 |
88 | Hoisting and TDZ in Practice | 14:43 |
89 | The this Keyword | 06:32 |
90 | The this Keyword in Practice | 13:12 |
91 | Regular Functions vs. Arrow Functions | 18:05 |
92 | Primitives vs. Objects (Primitive vs. Reference Types) | 16:07 |
93 | Primitives vs. Objects in Practice | 14:58 |
94 | Section Intro | 00:55 |
95 | Destructuring Arrays | 19:34 |
96 | Destructuring Objects | 19:47 |
97 | The Spread Operator (...) | 21:27 |
98 | Rest Pattern and Parameters | 19:02 |
99 | Short Circuiting (&& and ||) | 15:56 |
100 | The Nullish Coalescing Operator (??) | 03:33 |
101 | Logical Assignment Operators | 11:41 |
102 | Coding Challenge #1 | 14:58 |
103 | Looping Arrays: The for-of Loop | 07:21 |
104 | Enhanced Object Literals | 07:03 |
105 | Optional Chaining (?.) | 16:11 |
106 | Looping Objects: Object Keys, Values, and Entries | 10:10 |
107 | Coding Challenge #2 | 14:31 |
108 | Sets | 13:19 |
109 | Maps: Fundamentals | 14:04 |
110 | Maps: Iteration | 12:42 |
111 | Summary: Which Data Structure to Use? | 09:41 |
112 | Coding Challenge #3 | 09:38 |
113 | Working With Strings - Part 1 | 16:54 |
114 | Working With Strings - Part 2 | 21:46 |
115 | Working With Strings - Part 3 | 21:42 |
116 | Coding Challenge #4 | 15:16 |
117 | String Methods Practice | 16:37 |
118 | Section Intro | 00:49 |
119 | Default Parameters | 09:18 |
120 | How Passing Arguments Works: Value vs. Reference | 13:37 |
121 | First-Class and Higher-Order Functions | 05:25 |
122 | Functions Accepting Callback Functions | 15:21 |
123 | Functions Returning Functions | 06:37 |
124 | The call and apply Methods | 16:52 |
125 | The bind Method | 21:34 |
126 | Coding Challenge #1 | 18:48 |
127 | Immediately Invoked Function Expressions (IIFE) | 07:53 |
128 | Closures | 19:49 |
129 | More Closure Examples | 15:31 |
130 | Coding Challenge #2 | 05:26 |
131 | Section Intro | 00:54 |
132 | Simple Array Methods | 16:38 |
133 | The new at Method | 06:05 |
134 | Looping Arrays: forEach | 13:47 |
135 | forEach With Maps and Sets | 05:33 |
136 | PROJECT: "Bankist" App | 09:49 |
137 | Creating DOM Elements | 18:45 |
138 | Coding Challenge #1 | 08:32 |
139 | Data Transformations: map, filter, reduce | 04:41 |
140 | The map Method | 15:41 |
141 | Computing Usernames | 12:15 |
142 | The filter Method | 06:20 |
143 | The reduce Method | 20:58 |
144 | Coding Challenge #2 | 09:57 |
145 | The Magic of Chaining Methods | 19:41 |
146 | Coding Challenge #3 | 03:58 |
147 | The find Method | 06:48 |
148 | Implementing Login | 24:19 |
149 | Implementing Transfers | 20:55 |
150 | The findIndex Method | 12:36 |
151 | some and every | 15:12 |
152 | flat and flatMap | 09:33 |
153 | Sorting Arrays | 21:56 |
154 | More Ways of Creating and Filling Arrays | 20:34 |
155 | Summary: Which Array Method to Use? | 06:25 |
156 | Array Methods Practice | 32:22 |
157 | Coding Challenge #4 | 23:49 |
158 | Section Intro | 00:51 |
159 | Converting and Checking Numbers | 16:47 |
160 | Math and Rounding | 18:15 |
161 | The Remainder Operator | 10:57 |
162 | Numeric Separators | 06:58 |
163 | Working with BigInt | 11:19 |
164 | Creating Dates | 12:56 |
165 | Adding Dates to "Bankist" App | 22:22 |
166 | Operations With Dates | 15:28 |
167 | Internationalizing Dates (Intl) | 17:19 |
168 | Internationalizing Numbers (Intl) | 19:07 |
169 | Timers: setTimeout and setInterval | 13:54 |
170 | Implementing a Countdown Timer | 28:32 |
171 | Section Intro | 01:05 |
172 | PROJECT: "Bankist" Website | 08:50 |
173 | How the DOM Really Works | 10:37 |
174 | Selecting, Creating, and Deleting Elements | 20:29 |
175 | Styles, Attributes and Classes | 21:55 |
176 | Implementing Smooth Scrolling | 15:57 |
177 | Types of Events and Event Handlers | 10:34 |
178 | Event Propagation: Bubbling and Capturing | 05:04 |
179 | Event Propagation in Practice | 17:47 |
180 | Event Delegation: Implementing Page Navigation | 18:50 |
181 | DOM Traversing | 14:36 |
182 | Building a Tabbed Component | 24:09 |
183 | Passing Arguments to Event Handlers | 18:44 |
184 | Implementing a Sticky Navigation: The Scroll Event | 07:56 |
185 | A Better Way: The Intersection Observer API | 24:02 |
186 | Revealing Elements on Scroll | 12:40 |
187 | Lazy Loading Images | 18:31 |
188 | Building a Slider Component: Part 1 | 23:17 |
189 | Building a Slider Component: Part 2 | 19:36 |
190 | Lifecycle DOM Events | 09:27 |
191 | Efficient Script Loading: defer and async | 13:31 |
192 | Section Intro | 01:08 |
193 | What is Object-Oriented Programming? | 20:56 |
194 | OOP in JavaScript | 10:09 |
195 | Constructor Functions and the new Operator | 14:20 |
196 | Prototypes | 14:37 |
197 | Prototypal Inheritance and The Prototype Chain | 10:59 |
198 | Prototypal Inheritance on Built-In Objects | 14:48 |
199 | Coding Challenge #1 | 07:28 |
200 | ES6 Classes | 12:58 |
201 | Setters and Getters | 13:16 |
202 | Static Methods | 06:25 |
203 | Object.create | 10:57 |
204 | Coding Challenge #2 | 06:01 |
205 | Inheritance Between "Classes": Constructor Functions | 21:04 |
206 | Coding Challenge #3 | 10:38 |
207 | Inheritance Between "Classes": ES6 Classes | 10:47 |
208 | Inheritance Between "Classes": Object.create | 08:54 |
209 | Another Class Example | 10:45 |
210 | Encapsulation: Protected Properties and Methods | 06:48 |
211 | Encapsulation: Private Class Fields and Methods | 16:12 |
212 | Chaining Methods | 04:44 |
213 | ES6 Classes Summary | 07:13 |
214 | Coding Challenge #4 | 08:55 |
215 | Section Intro | 00:59 |
216 | Project Overview | 05:03 |
217 | How to Plan a Web Project | 17:42 |
218 | Using the Geolocation API | 08:17 |
219 | Displaying a Map Using Leaflet Library | 13:49 |
220 | Displaying a Map Marker | 19:55 |
221 | Rendering Workout Input Form | 16:43 |
222 | Project Architecture | 09:29 |
223 | Refactoring for Project Architecture | 24:06 |
224 | Managing Workout Data: Creating Classes | 16:19 |
225 | Creating a New Workout | 34:07 |
226 | Rendering Workouts | 24:20 |
227 | Move to Marker On Click | 16:33 |
228 | Working with localStorage | 25:32 |
229 | Final Considerations | 05:42 |
230 | Section Intro | 00:56 |
231 | Asynchronous JavaScript, AJAX and APIs | 17:58 |
232 | Our First AJAX Call: XMLHttpRequest | 19:11 |
233 | [OPTIONAL] How the Web Works: Requests and Responses | 13:39 |
234 | Welcome to Callback Hell | 13:53 |
235 | Promises and the Fetch API | 09:26 |
236 | Consuming Promises | 09:25 |
237 | Chaining Promises | 09:14 |
238 | Handling Rejected Promises | 16:14 |
239 | Throwing Errors Manually | 15:25 |
240 | Coding Challenge #1 | 16:33 |
241 | Asynchronous Behind the Scenes: The Event Loop | 17:54 |
242 | The Event Loop in Practice | 09:16 |
243 | Building a Simple Promise | 20:18 |
244 | Promisifying the Geolocation API | 13:27 |
245 | Coding Challenge #2 | 15:54 |
246 | Consuming Promises with Async/Await | 15:07 |
247 | Error Handling With try...catch | 10:23 |
248 | Returning Values from Async Functions | 14:40 |
249 | Running Promises in Parallel | 10:57 |
250 | Other Promise Combinators: race, allSettled and any | 13:20 |
251 | Coding Challenge #3 | 17:31 |
252 | Section Intro | 00:48 |
253 | An Overview of Modern JavaScript Development | 06:40 |
254 | An Overview of Modules in JavaScript | 15:02 |
255 | Exporting and Importing in ES6 Modules | 22:39 |
256 | Top-Level await (ES2022) | 14:45 |
257 | The Module Pattern | 10:21 |
258 | CommonJS Modules | 04:25 |
259 | A Brief Introduction to the Command Line | 12:28 |
260 | Introduction to NPM | 18:41 |
261 | Bundling With Parcel and NPM Scripts | 21:40 |
262 | Configuring Babel and Polyfilling | 18:04 |
263 | Review: Writing Clean and Modern JavaScript | 10:27 |
264 | Let's Fix Some Bad Code: Part 1 | 23:21 |
265 | Declarative and Functional JavaScript Principles | 12:26 |
266 | Let's Fix Some Bad Code: Part 2 | 38:10 |
267 | Section Intro | 00:57 |
268 | Project Overview and Planning (I) | 14:22 |
269 | Loading a Recipe from API | 24:12 |
270 | Rendering the Recipe | 22:24 |
271 | Listening For load and hashchange Events | 11:03 |
272 | The MVC Architecture | 16:33 |
273 | Refactoring for MVC | 38:54 |
274 | Helpers and Configuration Files | 21:24 |
275 | Event Handlers in MVC: Publisher-Subscriber Pattern | 15:09 |
276 | Implementing Error and Success Messages | 11:35 |
277 | Implementing Search Results - Part 1 | 25:47 |
278 | Implementing Search Results - Part 2 | 28:16 |
279 | Implementing Pagination - Part 1 | 12:19 |
280 | Implementing Pagination - Part 2 | 37:06 |
281 | Project Planning II | 03:14 |
282 | Updating Recipe Servings | 26:41 |
283 | Developing a DOM Updating Algorithm | 34:23 |
284 | Implementing Bookmarks - Part 1 | 26:01 |
285 | Implementing Bookmarks - Part 2 | 18:32 |
286 | Storing Bookmarks With localStorage | 18:23 |
287 | Project Planning III | 02:10 |
288 | Uploading a New Recipe - Part 1 | 17:44 |
289 | Uploading a New Recipe - Part 2 | 40:38 |
290 | Uploading a New Recipe - Part 3 | 19:59 |
291 | Wrapping Up: Final Considerations | 14:46 |
292 | Section Intro | 00:45 |
293 | Simple Deployment With Netlify | 11:19 |
294 | Setting Up Git and GitHub | 07:40 |
295 | Git Fundamentals | 19:37 |
296 | Pushing to GitHub | 09:32 |
297 | Setting Up Continuous Integration With Netlify | 10:07 |
298 | Where to Go from Here | 03:01 |
Similar courses to The Complete JavaScript Course 2023: From Zero to Expert!
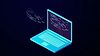
Full Stack Isomorphic JavaScript with Vue.js & Node.jsudemy
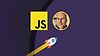
Animating with the JavaScript Web Animations APIudemy
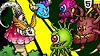
Build Animated Physics Game with JavaScriptudemy
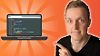
Build Fullstack Trello clone: WebSocket, Socket IOudemy
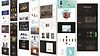
10 Mega Responsive Websites with HTML, CSS, and JavaScriptudemy
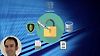
Web security: Injection Attacks with Java & Spring Bootudemy
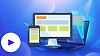
Complete Web Developer in 2023: Zero to Masteryudemyzerotomastery.io
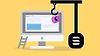