Data Structures & Algorithms - JavaScript
That means that you can actually learn more material in less time and have higher retention of the material. That is the key combination of factors to prepare you for the technical interview that lands you your dream job! I invite you to watch a few of the videos in this course to see what I mean. The difference will be noticeable right away! I spent over a year to create this course with the goal that an absolute beginner can take it and understand all of the concepts the first time through.
Read more about the course
After each line of code, an animation of the data structure or algorithm is updated to show exactly what that line of code did.
The animations provide some huge advantages to students:
Increased understanding of the concepts
Greater rate of retention
The material can be covered in a fraction of the time
Watch Online Data Structures & Algorithms - JavaScript
# | Title | Duration |
---|---|---|
1 | Introduction | 04:06 |
2 | Code Editor | 04:04 |
3 | Big O: Intro | 02:58 |
4 | Big O: Worst Case | 01:30 |
5 | Big O: O(n) | 02:06 |
6 | Big O: Drop Constants | 01:39 |
7 | Big O: O(n^2) | 03:10 |
8 | Big O: Drop Non-Dominants | 01:47 |
9 | Big O: O(1) | 01:24 |
10 | Big O: O(log n) | 04:26 |
11 | Big O: Different Terms for Input | 02:07 |
12 | Big O: Arrays | 05:36 |
13 | Big O: Wrap Up | 04:31 |
14 | Quiz Intro | 01:44 |
15 | Classes | 05:53 |
16 | Pointers | 04:36 |
17 | Linked List: Intro | 01:47 |
18 | LL: Big O | 06:13 |
19 | LL: Under the Hood | 02:40 |
20 | LL: Constructor | 07:13 |
21 | LL: Push | 05:43 |
22 | LL: Pop - Intro | 06:04 |
23 | LL: Pop - Code | 07:54 |
24 | LL: Unshift | 03:34 |
25 | LL: Shift | 05:19 |
26 | LL: Get | 03:47 |
27 | LL: Set | 04:42 |
28 | LL: Insert | 06:21 |
29 | LL: Remove | 04:57 |
30 | LL: Reverse | 05:54 |
31 | DLL: Constructor | 02:56 |
32 | DLL: Push | 03:56 |
33 | DLL: Pop | 07:08 |
34 | DLL: Unshift | 02:48 |
35 | DLL: Shift | 04:01 |
36 | DLL: Get | 04:29 |
37 | DLL: Set | 03:43 |
38 | DLL: Insert | 06:11 |
39 | DLL: Remove | 04:51 |
40 | Stack: Intro | 05:39 |
41 | Stack: Constructor | 03:01 |
42 | Stack: Push | 03:53 |
43 | Stack: Pop | 03:05 |
44 | Queue: Intro | 02:15 |
45 | Queue: Constructor | 02:00 |
46 | Queue: Enqueue | 03:13 |
47 | Queue: Dequeue | 03:25 |
48 | Trees: Intro & Terminology | 04:23 |
49 | Binary Search Trees: Example | 02:31 |
50 | BST: Big O | 06:55 |
51 | BST: Constructor | 03:39 |
52 | BST: Insert - Intro | 06:05 |
53 | BST: Insert - Code | 09:44 |
54 | BST: Contains | 08:31 |
55 | Hash Table: Intro | 05:22 |
56 | HT: Collisions | 02:39 |
57 | HT: Constructor | 04:58 |
58 | HT: Set | 04:51 |
59 | HT: Get | 04:45 |
60 | HT: Keys | 03:19 |
61 | HT: Big O | 02:06 |
62 | HT: Interview Question | 05:30 |
63 | Graph: Intro | 04:02 |
64 | Graph: Adjacency Matrix | 03:17 |
65 | Graph: Adjacency List | 01:18 |
66 | Graph: Big O | 06:17 |
67 | Graph: Add Vertex | 03:11 |
68 | Graph: Add Edge | 03:15 |
69 | Graph: Remove Edge | 03:57 |
70 | Graph: Remove Vertex | 07:00 |
71 | Recursion: Intro | 06:30 |
72 | Call Stack | 06:16 |
73 | Factorial | 08:39 |
74 | Bubble Sort: Intro | 02:25 |
75 | Bubble Sort: Code | 02:38 |
76 | Selection Sort: Intro | 02:56 |
77 | Selection Sort: Code | 04:58 |
78 | Insertion Sort: Intro | 01:24 |
79 | Insertion Sort: Code | 04:38 |
80 | Insertion Sort: Big O | 01:31 |
81 | Merge Sort: Overview | 01:45 |
82 | Merge: Intro | 01:38 |
83 | Merge: Code | 03:27 |
84 | Merge Sort: Intro | 01:19 |
85 | Merge Sort: Code | 03:40 |
86 | Merge Sort: Big O | 02:49 |
87 | Quick Sort: Intro | 02:32 |
88 | Pivot: Intro | 03:00 |
89 | Pivot: Code | 04:19 |
90 | Quick Sort: Code | 03:53 |
91 | Quick Sort: Big O | 03:36 |
92 | Tree Traversal: Intro | 01:56 |
93 | BFS (Breadth First Search): Intro | 03:38 |
94 | BFS: Code | 05:33 |
95 | DFS (Depth First Search): PreOrder - Intro | 01:20 |
96 | DFS: PreOrder - Code | 06:31 |
97 | DFS: PostOrder - Intro | 02:14 |
98 | DFS: PostOrder - Code | 04:54 |
99 | DFS: InOrder - Intro | 01:47 |
100 | DFS: InOrder - Code | 04:20 |
Similar courses to Data Structures & Algorithms - JavaScript
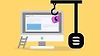
Web Components & Stencil.js - Build Custom HTML Elementsudemy
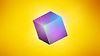
Object-oriented Programming in JavaScriptcodewithmosh (Mosh Hamedani)udemy
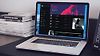
Make a Spotify Clone from Scratch: JavaScript PHP and MySQLudemy
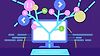
100 Days Of Code: The Complete Web Development Bootcamp 2024Academind Pro
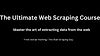
The Ultimate Web Scraping CourseAdrian Horning (The Web Scraping Guy)
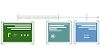