TypeScript course
6h 27m 32s
English
Paid
Types are fundamental to TypeScript, so naturally you'll need to be familiar with the base types that exist in JavaScript. We'll start the course off with some housekeeping items. You'll learn about the best strategy for getting the most out of the course as well as what you'll build during the course.
Watch Online TypeScript course
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Introduction, Philosophy, and Tips | 03:22 |
2 | Why TypeScript? | 05:34 |
3 | JavaScript Types | 08:57 |
4 | Basic TypeScript Configuration | 06:49 |
5 | Implicit Type Checking | 04:15 |
6 | Adding Type Annotations | 05:47 |
7 | Typing Function Declarations | 08:42 |
8 | (Practice) Type Annotations | 00:39 |
9 | (Solution) Type Annotations | 02:31 |
10 | `any` and `unknown` types | 05:27 |
11 | Interfaces | 11:07 |
12 | (Practice) Interfaces | 00:34 |
13 | (Solution) Interfaces | 02:24 |
14 | Enum and Tuple Types | 09:55 |
15 | Void and Never Types | 03:05 |
16 | `type` aliases | 04:35 |
17 | Union Types | 04:41 |
18 | Intersection Types | 02:50 |
19 | Literal Types | 02:39 |
20 | (Practice) Union & Literal Types | 01:25 |
21 | (Solution) Union & Literal Types | 02:54 |
22 | (Project) Starting Template | 02:46 |
23 | (Project) Adding Initial Types | 02:49 |
24 | (Project) Cell Event Handler | 01:49 |
25 | (Project) Win Condition | 08:20 |
26 | Class Definition | 07:51 |
27 | Modifiers | 08:42 |
28 | (Practice) Classes | 01:48 |
29 | (Solution) Classes | 03:24 |
30 | TypeScript Operators | 06:59 |
31 | (Bonus) Advanced Function Typing | 03:19 |
32 | Common Type Guards | 08:35 |
33 | Handling null and undefined | 06:48 |
34 | (Practice) Narrowing Types | 00:29 |
35 | (Solution) Narrowing Types | 05:29 |
36 | (Bonus) Structural vs Nominal Typing | 06:55 |
37 | Discriminating Unions | 02:31 |
38 | (Practice) Discriminating Unions | 01:03 |
39 | (Solution) Discriminating Unions | 01:47 |
40 | Assertion Signatures | 06:59 |
41 | User Defined Type Guards | 05:42 |
42 | (Practice) User Defined Type Guards | 00:33 |
43 | (Solution) User Defined Type Guards | 02:27 |
44 | Generics | 12:41 |
45 | (Bonus) Zustand Implementation | 11:11 |
46 | (Practice) Generics | 00:44 |
47 | (Solution) Generics | 01:55 |
48 | (Bonus) Thinking In Types | 04:34 |
49 | Mapped Types | 05:31 |
50 | Conditional Types | 11:00 |
51 | (Practice) Utility Types | 01:10 |
52 | (Solution) Utility Types | 11:17 |
53 | (Bonus) ES Modules In Depth | 06:57 |
54 | Modules in TypeScript | 06:14 |
55 | (Bonus) TypeScript Namespaces | 03:41 |
56 | Built-In Type Definitions | 03:12 |
57 | (Bonus) Outputting TypeScript Definitions | 02:48 |
58 | Definitely Typed and @types/ packages | 03:55 |
59 | Additional TSConfig.json options | 08:36 |
60 | (Bonus) How the `target` field works | 05:05 |
61 | Module Resolution | 12:47 |
62 | (Bonus) Configuring for Webpack Development | 04:41 |
63 | (Bonus) Configuring for Babel Development | 02:50 |
64 | (Bonus) Configuring for Modern Web Development | 05:17 |
65 | (Bonus) Configuring for Node Development | 05:09 |
66 | (Bonus) Configuring for Library Development with TSDX | 02:09 |
67 | Recursive Conditional Types | 04:28 |
68 | Template Literal Types | 09:48 |
69 | Mapped Types Key Remapping | 06:42 |
70 | Unexpected TypeScript Behavior ( | 10:48 |
71 | (Bonus) Experimental Decorators | 05:33 |
72 | (Project) Initial NodeJS Setup | 03:38 |
73 | (Project) Static Web Server | 06:08 |
74 | (Project) Multiple Routes | 04:57 |
75 | (Project) Dynamic API Route | 05:23 |
76 | Outro | 01:26 |
Similar courses to TypeScript course
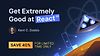
Learn React 19 with Epic React v2Kent C. Dodds
Category: TypeScript, React.js
Duration 26 hours 51 minutes 3 seconds
Course
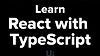
React with TypeScriptui.dev (ex. Tyler McGinnis)
Category: TypeScript, React.js
Duration 2 hours 53 seconds
Course
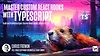
Master Custom React Hooks with TypeScriptfullstack.io
Category: TypeScript, React.js
Duration 2 hours 21 minutes 3 seconds
Course
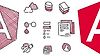
Angular Architecture. How to Build Scalable Web Applicationsudemy
Category: Angular, TypeScript, Firebase
Duration 7 hours 34 minutes 45 seconds
Course
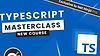
TypeScript MasterclassNet Ninja
Category: TypeScript
Duration 5 hours 21 minutes 53 seconds
Course
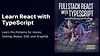
Fullstack React with Typescriptfullstack.io
Category: TypeScript, React.js
Duration 10 hours 16 minutes 46 seconds
Book
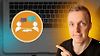
TypeScript Interview Questions - Coding Interview 2023udemy
Category: TypeScript, Preparing for an interview
Duration 2 hours 56 minutes 26 seconds
Course