Build a Backend REST API with Python & Django - Advanced
Welcome to the advanced course on how to Build a Backend REST API using Python, Django (2.0), Django REST Framework (3.9), Docker, Travis CI, Postgres and Test Driven Development! Whether you’re a freelance programmer, tech entrepreneur, or just starting out building backends - this course will help lay the foundation of your knowledge base and give you the tools to advance your skills with some of the most in-demand programming languages today.
Read more about the course
APIs are the unsung heroes behind the technologies that we all love and use religiously.
One of the most critical components for any tech-based business is an API. So knowing how to create an API from start to finish is a vital skill to have as a developer. You cannot build a successful app without a backend REST API!
In this course I’ll show you how to build an advanced API that handles creating and updating user profiles, changing passwords, creating objects, uploading images, filtering and searching objects, and more.
The best way to learn anything is to do it. So the practical application of the course -- the project that you’ll build along side me -- is an API. A recipe API, to be specific.
You will learn how to build an advanced recipe API that allows you to upload and store some of your favourite recipes from photos and the web.
You’ll learn how to create objects i.e. recipes with titles, price points, cooking times, ingredients and tags like “comfort food”, “vegan” or “dessert”. Think of it as a virtual recipe box.
By the end of this course you will have built a fully functioning REST API that can handle:
User authentication
Creating objects
Filtering and sorting objects
Uploading and viewing images
You’ll also learn, in detail how to:
Setup a project with Docker and Docker-Compose
Configure Travis-CI to automatically run linting and unit tests
Write unit tests using the Django Test Framework
Apply best practice principles including Test Driven Development
Handle uploading media files with Django
Customize the Django admin
Configure a Postgres database
This course has one singular focus: To teach you how to create an advanced API from start to finish using best practice principles and Test Driven Development.
This course is NOT FOR YOU:
If you’re looking for a course to build an API, a front end, and deployment
If you’re looking to build 10 different apps in one course
If you want to learn lots of different technologies and approaches to app development in general
This is a hands-on course, with a bit of theory and lots of opportunities to test your knowledge.
The content is challenging but rewarding. Ready for it? Let’s dive in!
**PLEASE NOTE: You cannot run Docker on Windows 10 Home edition. This is because Windows 10 Pro or Enterprise is required in order to use Hyper-V which Docker uses for virtualization. To take this course you have two options. These are covered in Lecture 6, which is free to preview before purchasing the course.
- Basic knowledge of programming and building simple applications
- Familiar with Django
- Comfortable using command line tools (Terminal/Command Prompt)
- macOS, Linux or Windows machine capable of running Docker (This excludes Windows 10 Home)
- Positive attitude and willingness to learn!
- Intermediate programmers who already have some understanding of Python and want to skill up
- Developers proficient in other languages but looking to add Python to their toolkit
What you'll learn:
- Setting up a local development server with Docker
- Writing a Python project using Test Driven Development
- Building a REST API with advanced features such as uploading and viewing images
- Creating a backend that can be used a base for your future projects or MVP
- Hands on experience applying best practice principles such as PEP-8 and unit tests
- Configure Travis-CI to automate code checks
Watch Online Build a Backend REST API with Python & Django - Advanced
# | Title | Duration |
---|---|---|
1 | Welcome to Build a Backend API with Django REST Framework - Advanced | 02:21 |
2 | Intro to the course | 02:25 |
3 | Course structure | 01:04 |
4 | How to get the most out of this course | 00:55 |
5 | How to get help | 01:26 |
6 | Python | 00:49 |
7 | Django | 00:55 |
8 | Django REST Framework | 00:47 |
9 | Docker | 00:36 |
10 | Travis-CI | 00:44 |
11 | Postgres | 00:19 |
12 | What is test driven development? | 04:01 |
13 | Setup new GitHub project | 02:51 |
14 | Add Dockerfile | 09:57 |
15 | Configure Docker Compose | 05:23 |
16 | Create Django project | 02:57 |
17 | Enable Travis-CI for project | 01:42 |
18 | Create Travis-CI configuration file | 07:16 |
19 | Writing a simple unit test | 05:48 |
20 | Writing a unit test with TDD | 05:09 |
21 | Create core app | 02:53 |
22 | Add tests for custom user model | 05:42 |
23 | Implement custom user model | 10:24 |
24 | Normalize email addresses | 03:26 |
25 | Add validation for email field | 02:37 |
26 | Add support for creating superusers | 05:21 |
27 | Add tests for listing users in Django admin | 09:31 |
28 | Modify Django admin to list our custom user model | 02:28 |
29 | Modify Django admin to support changing user model | 07:49 |
30 | Modify Django admin to support creating users | 04:57 |
31 | Add postgres to docker compose | 05:26 |
32 | Add postgres support to Dockerfile | 05:44 |
33 | Configure database in Django | 02:53 |
34 | Mocking with unittests | 01:31 |
35 | Add tests for wait_for_db command | 11:44 |
36 | Add wait_for_db command | 07:32 |
37 | Make docker compose wait for db | 02:52 |
38 | Test in browser | 03:02 |
39 | Create users app | 03:30 |
40 | Add tests for create user API | 16:44 |
41 | Add create user API | 13:53 |
42 | Add tests for creating a new token | 10:40 |
43 | Add create token API | 13:54 |
44 | Add tests for manage user endpoint | 11:51 |
45 | Add manage user endpoint | 11:25 |
46 | Create recipe app | 02:16 |
47 | Add tag model | 08:10 |
48 | Add tests for listing tags | 12:09 |
49 | Add feature to list tags | 12:13 |
50 | Add create tags feature | 07:08 |
51 | Add ingredient model | 04:09 |
52 | Add tests for listing ingredients | 10:30 |
53 | Implement feature for list ingredients | 05:05 |
54 | Implement feature for creating ingredients | 07:39 |
55 | Re-factor tags and ingredients viewsets | 07:05 |
56 | Add recipe model | 12:51 |
57 | Add tests for listing recipes | 15:17 |
58 | Implement feature for listing recipes | 08:39 |
59 | Add tests for retrieving recipe detail | 09:13 |
60 | Implement feature for retrieving recipe detail | 07:07 |
61 | Add tests for creating recipes | 11:27 |
62 | Implement feature for creating recipes | 04:23 |
63 | Add tests for updating recipes | 09:43 |
64 | Add Pillow requirement | 12:16 |
65 | Modify recipe model | 11:54 |
66 | Add tests for uploading image to recipe | 13:30 |
67 | Add feature to upload image | 11:18 |
68 | Add tests for filtering recipes | 08:58 |
69 | Implement feature to filter recipes | 09:20 |
70 | Add tests for filtering tags and ingredients | 13:00 |
71 | Implement feature for filtering tags and ingredients | 06:32 |
72 | What was covered in this course | 00:53 |
Similar courses to Build a Backend REST API with Python & Django - Advanced
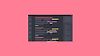
Build a Python REST API with the Django Rest Frameworkudemy
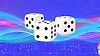
Statistics Bootcamp (with Python): Zero to Masteryzerotomastery.io
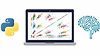
Python for Data Science and Machine Learning Bootcampudemy
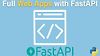
Full Web Apps with FastAPITalkpython
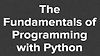
The Fundamentals of Programming with Pythontechwithtim.net (Tim Ruscica)
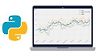
Python for Financial Analysis and Algorithmic Tradingudemy
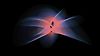
Complete linear algebra: theory and implementationudemy
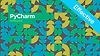
Effective PyCharm (2021 edition)Talkpython
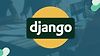
Django Masterclass : Build Web Apps With Python & Djangoudemy
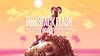