Async Techniques and Examples in Python
Python's async and parallel programming support is highly underrated. In this course, you will learn the entire spectrum of Python's parallel APIs. We will start with covering the new and powerful async and await keywords along with the underpinning module: asyncio. Then we'll move on to Python's threads for parallelizing older operations and multiprocessing for CPU bound operations. We'll close out the course with a host of additional async topics such as async Flask, task coordination, thread safety, and C-based parallelism with Cython.
Read more about the course
Source code and course GitHub repository
github.com/talkpython/async-techniques-python-course
What's this course about and how is it different?
This is the definitive course on parallel programming in Python. It covers the tried and true foundational concepts such as threads and multiprocessing as well as the most modern async features based on Python 3.7+ with async and await.
In addition to the core concepts and APIs for concurrent programming, you will learn best practices and how to choose between the various APIs as well as how to use them together for the biggest advantage.
In this course, you will:
- See how concurrency allows improved performance and scalability
- Build async-capable code with the new async and await keywords
- Add asynchrony to your app without additional threads or processes
- Work with multiple threads to run I/O bound work in Python
- Use locks and thread safety mechanisms to protect shared data
- Recognize a dead-lock and see how to prevent them in Python threads
- Take full advantage of multicore CPUs with multiprocessing
- Unify the thread and process APIs with execution pools
- Add massive speedups with Cython and Python threads
- Create async view methods in Flask web apps
- And lots more
Who is this course for?
Anyone who would like to write Python code that does more, scales better, and takes better advantage of modern, multicore CPUs. Whether you're a web developer or data scientists, you will find a host of techniques to do more faster.
Watch Online Async Techniques and Examples in Python
# | Title | Duration |
---|---|---|
1 | Course introduction | 01:27 |
2 | Async for taking full advantage of modern CPUs | 01:52 |
3 | Topics covered | 04:53 |
4 | Student prerequisites | 00:45 |
5 | Meet your instructor | 00:49 |
6 | Video player: A quick feature tour | 02:05 |
7 | Do you have Python 3? | 01:40 |
8 | Getting Python 3 | 00:39 |
9 | Recommended text editor | 00:54 |
10 | Hardware requirements | 01:14 |
11 | Get the source code | 01:02 |
12 | Async for computational speed | 03:43 |
13 | Demo: Why you need async for speed | 03:55 |
14 | An upper bound for async speed improvement | 03:53 |
15 | Async for scalability | 01:50 |
16 | Concept: Visualizing a synchronous request | 03:34 |
17 | Concept: Visualizing an asynchronous request | 02:15 |
18 | Python's async landscape | 04:25 |
19 | Why threads don't perform in Python | 02:53 |
20 | Python async landscape: asyncio | 01:16 |
21 | I/O-driven concurrency | 03:51 |
22 | Demo: Understanding basic generators | 09:05 |
23 | Demo: The producer-consumer app | 03:08 |
24 | Demo: Make the producer-consumer async | 05:36 |
25 | Demo: Make the producer-consumer async (methods) | 07:17 |
26 | Concept: asyncio | 01:18 |
27 | Performance improvements of producer consumer with asyncio | 01:47 |
28 | Faster asyncio loops with uvloop | 04:38 |
29 | Let's do some real work | 01:07 |
30 | Synchronous web scraping | 03:09 |
31 | async web scraping | 09:17 |
32 | Concept: async web scraping | 01:25 |
33 | Other async-enabled libraries | 03:42 |
34 | Python async landscape: Threads | 01:07 |
35 | Visual of thread execution | 01:13 |
36 | How to choose between asyncio and threads | 02:34 |
37 | Demo: hello threads | 05:00 |
38 | Demo: Waiting on more than one thread | 03:53 |
39 | Demo: Something productive with threads | 03:10 |
40 | Concept: Thread API | 01:42 |
41 | Concept: Tips for multiple threads | 00:42 |
42 | Cancelling threads with user input | 06:02 |
43 | Concept: Timeouts | 01:22 |
44 | Demo: Attempting to leverage multiple cores with threads | 05:46 |
45 | Python async landscape: Thread Safety landscape | 00:47 |
46 | Threads are dangerous | 01:28 |
47 | Visualizing the need for thread safety | 03:35 |
48 | Demo: An unsafe bank | 05:05 |
49 | Demo: Make the bank safe (global) | 04:35 |
50 | Demo: A missed lock in our bank (global) | 01:45 |
51 | Demo: Make the bank safe (fine-grained) | 05:50 |
52 | Demo: Breaking a deadlock | 03:45 |
53 | Concept: Basic thread safety | 01:43 |
54 | Python async landscape: multiprocessing | 01:03 |
55 | Introduction to scaling CPU-bound operations | 01:52 |
56 | Demo: Scaling CPU-bound operations with multiprocessing | 04:56 |
57 | Concept: Scaling CPU-bound operations | 01:22 |
58 | Multiprocessing return values | 02:19 |
59 | Concept: Return values | 01:00 |
60 | Python async landscape: Execution pools | 01:51 |
61 | Demo: Executor app introduction | 02:22 |
62 | Demo: Executor app (threaded-edition) | 06:45 |
63 | Demo: Executor app (process-edition) | 01:47 |
64 | Concept: Execution pools | 01:43 |
65 | Python async landscape: asyncio derivatives | 01:32 |
66 | Why do we need more libraries? | 04:32 |
67 | Introducing unsync | 02:22 |
68 | Demo: unsync app introduction | 04:22 |
69 | Demo: unsync app for mixed-mode parallelism | 05:55 |
70 | Concept: Mixed-mode parallelism with unsync | 03:11 |
71 | Introducing Trio | 01:11 |
72 | Demo: Starter code for Trio app | 01:02 |
73 | Demo: Converting from asyncio to Trio | 04:54 |
74 | Demo: Cancellation with Trio | 01:57 |
75 | Concept: Trio nurseries | 01:17 |
76 | The trio-async package | 00:56 |
77 | Python async landscape: Async web | 01:21 |
78 | Review: Request latency again | 01:32 |
79 | Demo: Introducing our Flask API | 05:02 |
80 | There is no async support for Flask | 01:51 |
81 | Demo: Introducing Quart for async Flask | 01:06 |
82 | Demo: Converting from Flask to Quart | 01:30 |
83 | Demo: Making our API async | 04:39 |
84 | Demo: An async weather endpoint | 01:34 |
85 | Concept: Flask to Quart | 02:37 |
86 | Load testing web apps with wrk | 02:01 |
87 | A note about rate limiting with external services | 03:17 |
88 | Performance results | 03:33 |
89 | Remember to run on an ASGI server | 01:42 |
90 | Python async landscape: Cython | 01:32 |
91 | C and Python are friends | 01:45 |
92 | Why Cython | 03:00 |
93 | Cython syntax compared | 02:27 |
94 | Demo: Hello Cython | 05:37 |
95 | Concept: Getting started with Cython | 01:12 |
96 | Demo: Fast threading with cython (app review) | 02:47 |
97 | Demo: Fast threading with Cython (hotspot) | 01:40 |
98 | Demo: Fast threading with Cython (conversion) | 02:20 |
99 | Demo: Fast threading with Cython (GIL-less) | 04:06 |
100 | Demo: Fast threading with Cython (int overflow issues) | 02:53 |
101 | Concept: Cython's nogil | 01:25 |
102 | The finish line | 00:35 |
103 | Review: Why async? | 02:01 |
104 | Review: asyncio | 01:04 |
105 | Review: Threads | 01:19 |
106 | Review: Thread safety | 02:17 |
107 | Review: multiprocessing | 02:14 |
108 | Review: Execution pools | 01:45 |
109 | Review: Mixed-mode parallelism | 01:59 |
110 | Review: Coordination with Trio | 01:35 |
111 | Review: Async Flask | 01:18 |
112 | Review: Cython | 01:39 |
113 | Thanks and goodbye | 00:17 |
Similar courses to Async Techniques and Examples in Python
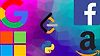
LeetCode In Python: 50 Algorithms Coding Interview Questionsudemy
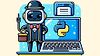
OpenAI Assistants with OpenAI Python APIudemy
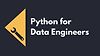
Python for Data EngineersAndreas Kretz
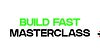
Build Fast MasterclassBuildFast Academy
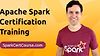
Apache Spark Certification TrainingFlorian Roscheck
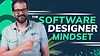
The Software Designer Mindset (COMPLETE)ArjanCodes
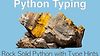
Rock Solid Python with Python Typing CourseTalkpython
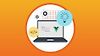
The Complete Guide to Django REST Framework and Vue JSudemy
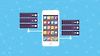
REST APIs with Flask and Pythonudemy
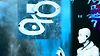