Rock Solid Python with Python Typing Course
4h 27m 54s
English
Paid
When Python was originally invented way back in 1989, it was a truly dynamic and typeless programming language. But that all changed in Python 3.5 when type "hints" were added to the language. Over time, amazing frameworks took that idea and ran with it. They build powerful and type safe(er) frameworks. Some of these include Pydantic, FastAPI, Beanie, SQLModel, and many many more. In this course, you'll learn the ins-and-outs of Python typing in the language, explore some popular frameworks using types, and get some excellent advice and guidance for using types in your applications and libraries.
Read more about the course
In this course, you will:
- Compare popular static languages with Python (such as Swift, C#, TypeScript, and others)
- See a exact clone of a dynamic Python codebase along side the typed version
- Learn how and when to create typed variables
- Understand Python's strict nullability in its type system
- Specify constant (unchangeable) variables and values
- Reduce SQL injection attacks with LiteralString
- Uses typing with Python functions and methods
- Use typing with classes and class variables
- Work with multiple numerical types with Python's numerical type ladder
- Use Pydantic to model and parse complex data in a type strict manner
- Create an API with FastAPI that exchanges data with type integrity
- Query databases with Pydantic using the Beanie ODM
- Create CLI apps using type information to define the CLI interface
- Leverage mypy for verifying the integrity of your entire codebase in CI/CD
- Add runtime type safety to your application
- Marry duck typing and static typing with Python's new Protocol construct
- Learn design patterns and guidance for using types in Python code
Watch Online Rock Solid Python with Python Typing Course
Join premium to watch
Go to premium
# | Title | Duration |
---|---|---|
1 | Welcome | 01:57 |
2 | Python Language Typing Definition | 02:41 |
3 | What We'll Cover | 02:42 |
4 | Goal: Not 100% | 01:11 |
5 | You'll Need Python 3.10 or Newer | 01:07 |
6 | git the Repo | 01:22 |
7 | Meet Your Instructor | 01:38 |
8 | Spectrum of Type Strictness | 06:37 |
9 | Running the Source Code | 03:26 |
10 | Motorcycle Class, Untyped | 05:36 |
11 | Using the Untyped Motorcycle | 02:21 |
12 | Duck Typing | 03:03 |
13 | TypeScript Motorcycles | 04:40 |
14 | C# Motorcycle and Why Types Can Detract from Readability | 05:02 |
15 | A Very Swift Motorcycle | 04:04 |
16 | Typed Python Motorcycles | 07:38 |
17 | Python Typing Introduction | 00:33 |
18 | Where Do Python Type Hints Come From? | 01:14 |
19 | Typing and Variables | 03:23 |
20 | Survey of Core Types | 03:05 |
21 | Nullable Types | 05:22 |
22 | Unions | 03:11 |
23 | If You Don't Know the Type | 02:03 |
24 | Constants | 03:37 |
25 | Avoiding Injection Attacks with LiteralString | 06:02 |
26 | Functions: Basic Typing | 05:13 |
27 | Functions: void Functions | 02:44 |
28 | Functions: Functions as Objects | 05:34 |
29 | Typing for Container Data Types | 07:22 |
30 | More Complex Containers | 08:28 |
31 | Classes and Typing | 06:27 |
32 | Externally Defining Types | 04:27 |
33 | Adding Our Own Types | 03:56 |
34 | Representing Multiple Numerical Types | 04:13 |
35 | Generics Available in Python 3.12 | 01:45 |
36 | Gradual Typing | 03:11 |
37 | Frameworks Introduciton | 00:46 |
38 | Pydantic Foundations | 02:24 |
39 | Pydantic Code Example | 01:00 |
40 | pip-tools for Adding Requirements | 03:16 |
41 | Parsing Basic Data with Pydantic | 08:57 |
42 | Data-Rich Pydantic Example | 06:03 |
43 | Web frameworks using Type Hints | 04:58 |
44 | Database Frameworks Built on Pydantic | 03:40 |
45 | CLIs with Python Types | 02:09 |
46 | Setting up Our FastAPI Example | 03:43 |
47 | FastAPI, Beanie, and Pydantic MongoDB Example | 04:27 |
48 | Setting up the DB to Run the Code Yourself | 02:08 |
49 | Tools Introduction | 00:42 |
50 | Editors (Round 2) | 02:26 |
51 | Full Project Inspection | 06:12 |
52 | Static Type Checkers | 01:32 |
53 | mypy in Action | 05:14 |
54 | Runtime Type Checking with Beartype | 01:56 |
55 | Getting Started with Beartype | 06:43 |
56 | Beartype Speed Test | 07:01 |
57 | Orthogonal/Structural Typing Introduction | 01:06 |
58 | Inheritance Gone Wrong, an Example | 06:11 |
59 | Static duck typing with Protocols | 08:24 |
60 | Structural Typing Visualized | 01:19 |
61 | Patterns Introduction | 01:11 |
62 | Types on the Boundary | 02:05 |
63 | Public Packages | 01:30 |
64 | Autocomplete | 01:10 |
65 | To Optional or Not | 03:11 |
66 | Versions of Python | 04:19 |
67 | Minimalism Overview | 04:12 |
68 | Minimalism Code | 01:45 |
69 | Refactoring Motivation | 01:31 |
70 | Refactoring with Types | 02:37 |
71 | Point of No Return | 07:39 |
72 | Collection Advice | 02:27 |
73 | Conclusion | 05:05 |
Similar courses to Rock Solid Python with Python Typing Course
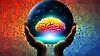
Mastering OpenAI Python APIs: Unleash ChatGPT and GPT4udemy
Category: Python, ChatGPT
Duration 13 hours 4 minutes 58 seconds
Course
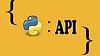
API Testing with Python 3 & PyTest, Backend Automation 2022udemy
Category: Python
Duration 13 hours 59 minutes 14 seconds
Course
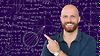
Mathematical Foundations of Machine Learningudemy
Category: Python, Data processing and analysis
Duration 16 hours 25 minutes 26 seconds
Course
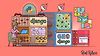
Make a Location-Based Web App With Django and GeoDjangorealpython.com
Category: Python, Django
Duration 56 minutes 48 seconds
Course
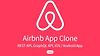
Airbnb App CloneNomad Coders
Category: Python, Other (Mobile Apps Development)
Duration 17 hours 50 minutes 5 seconds
Course
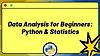
Data Analysis for Beginners: Python & Statisticszerotomastery.io
Category: Python, Data processing and analysis
Duration 6 hours 34 minutes 20 seconds
Course
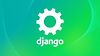
The Ultimate Django Series: Part 1codewithmosh (Mosh Hamedani)
Category: Python
Duration 4 hours 49 minutes 19 seconds
Course
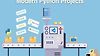
Modern Python ProjectsTalkpython
Category: Python
Duration 8 hours 45 minutes 6 seconds
Course
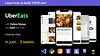
Create UberEats with Python/Django and Swift 3Code4Startup (coderealprojects)
Category: Python, Django, Swift
Duration 19 hours 13 minutes 29 seconds
Course
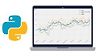
Python for Financial Analysis and Algorithmic Tradingudemy
Category: Others, Python
Duration 16 hours 54 minutes 20 seconds
Course