REST APIs with Flask and Python
Are you tired of boring, outdated, incomplete, or incorrect tutorials? I say no more to copy-pasting code that you don’t understand. Welcome to one of the best resources online on creating REST APIs. I'm Jose, and I'm a software engineer; here to help you truly understand and develop your skills in web and REST API development with Python, using Flask.
More
Production-ready REST APIs with Flask
This course will guide you in creating simple, intermediate, and advanced REST APIs including authentication, deployments, caching, and much more.
We'll start with a Python refresher that will take you from the very basics to some of the most advanced features of Python—that's all the Python you need to complete the course.
Using Flask and popular extensions Flask-RESTful, Flask-JWT, and Flask-SQLAlchemy we will dive right into developing complete, solid, production-ready REST APIs.
We will also look into essential technologies Git, Heroku, and nginx.
You'll be able to...
Create resource-based, production-ready REST APIs using Python, Flask, and popular Flask extensions;
Handle secure user registration and authentication with Flask.
Using SQLAlchemy and Flask-SQLAlchemy to easily and efficiently store resources to a database; and
Understand the complex intricacies of deployments and the performance of Flask REST APIs.
But what is a REST API anyway? Put simply, a REST API is an application that accepts data from clients and returns data back. With the data, it can do many things. For example, a REST API we build in this course accepts text data from the client, processes it and stores it in a database, and then returns some data back so the client can show something to the user.
When working with REST APIs, the client is usually a web app or mobile app. That's in contrast to web apps, where the client is usually the user themselves.
I pride myself on providing excellent support and feedback to every single student. I am always available to guide you and answer your questions.
I'll see you on the inside. Let's take another step toward REST API mastery!
- Some prior programming experience in any programming language will help. The course includes a full Python refresher course.
- All software used in the course is provided, and completely free
- Complete beginners may wish to take a beginner Python course first, and then transition to this course afterwards
- Students wanting to extend the capabilities of mobile and web applications by using server-side technologies
- Software developers looking to expand their skill-set by learning to develop professional grade REST APIs
- Those looking to learn Python while specifically catering to web services
What you'll learn:
- Connect web or mobile applications to databases and servers via REST APIs
- Create secure and reliable REST APIs which include authentication, logging, caching, and more
- Understand the different layers of a web server and how web applications interact with each other
- Handle seamless user authentication with advanced features like token refresh
- Handle log-outs and prevent abuse in your REST APIs with JWT blacklisting
- Develop professional-grade REST APIs with expert instruction
Watch Online REST APIs with Flask and Python
# | Title | Duration |
---|---|---|
1 | Introduction to this section | 01:02 |
2 | Variables in Python | 08:27 |
3 | Solution to coding exercise: Variables | 02:01 |
4 | String formatting in Python | 06:27 |
5 | Getting user input | 05:17 |
6 | Writing our first Python app | 03:20 |
7 | Lists, tuples, and sets | 06:32 |
8 | Advanced set operations | 04:40 |
9 | Solution to coding exercise: Lists, tuples, sets | 04:41 |
10 | Booleans in Python | 05:01 |
11 | If statements | 08:18 |
12 | The 'in' keyword in Python | 02:03 |
13 | If statements with the 'in' keyword | 08:19 |
14 | Loops in Python | 11:08 |
15 | Solution to coding exercise: Flow control | 03:09 |
16 | List comprehensions in Python | 07:25 |
17 | Dictionaries | 08:32 |
18 | Destructuring variables | 08:29 |
19 | Functions in Python | 10:42 |
20 | Function arguments and parameters | 07:41 |
21 | Default parameter values | 03:55 |
22 | Functions returning values | 07:20 |
23 | Solution to coding exercise: Functions | 02:31 |
24 | Lambda functions in Python | 07:53 |
25 | Dictionary comprehensions | 04:02 |
26 | Solution to coding exercise: Dictionaries | 06:17 |
27 | Unpacking arguments | 10:25 |
28 | Unpacking keyword arguments | 08:45 |
29 | Object-Oriented Programming in Python | 15:53 |
30 | Magic methods: __str__ and __repr__ | 06:26 |
31 | Solution to coding exercise: Classes and objects | 05:05 |
32 | @classmethod and @staticmethod | 14:04 |
33 | Solution to coding exercise: @classmethod and @staticmethod | 05:55 |
34 | Class inheritance | 08:33 |
35 | Class composition | 06:09 |
36 | Type hinting in Python 3.5+ | 05:09 |
37 | Imports in Python | 09:34 |
38 | Relative imports in Python | 08:54 |
39 | Errors in Python | 12:48 |
40 | Custom error classes | 05:05 |
41 | First-class functions | 07:53 |
42 | Simple decorators in Python | 07:13 |
43 | The 'at' syntax for decorators | 03:34 |
44 | Decorating functions with parameters | 02:25 |
45 | Decorators with parameters | 04:51 |
46 | Mutability in Python | 06:04 |
47 | Mutable default parameters (and why they're a bad idea) | 04:28 |
48 | Overview of the project we'll build | 04:01 |
49 | Initial set-up for a Flask app | 04:37 |
50 | Your first REST API endpoint | 04:06 |
51 | What is JSON? | 03:44 |
52 | How to interact with and test your REST API | 04:30 |
53 | How to create stores in our REST API | 05:38 |
54 | How to create items in each store | 07:56 |
55 | How to get a specific store and its items | 04:40 |
56 | What are Docker containers and images? | 13:29 |
57 | How to run a Flask app in a Docker container | 11:16 |
58 | Data model improvements for our API | 15:41 |
59 | General improvements to our first REST API | 07:08 |
60 | New endpoints for our first REST API | 09:06 |
61 | How to run the API in Docker with automatic reloading and debug mode | 06:27 |
62 | How to use Blueprints and MethodViews in Flask | 10:26 |
63 | How to write marshmallow schemas for our API | 04:02 |
64 | How to perform data validation with marshmallow | 04:32 |
65 | Decorating responses with Flask-Smorest | 04:40 |
66 | Overview and why use SQLAlchemy | 02:08 |
67 | How to code a simple SQLAlchemy model | 04:46 |
68 | How to write one-to-many relationships using SQLAlchemy | 09:43 |
69 | How to configure Flask-SQLAlchemy with your Flask app | 07:55 |
70 | How to insert data into a table using SQLAlchemy | 07:22 |
71 | How to find models in the database by ID or return a 404 | 03:59 |
72 | How to update models with SQLAlchemy | 05:11 |
73 | How to retrieve list of all models | 00:50 |
74 | How to delete models with SQLAlchemy | 01:12 |
75 | Conclusion of this section | 03:54 |
76 | Changes in this section | 02:48 |
77 | One-to-many relationship between stores and tags | 10:54 |
78 | Many-to-many relationship between items and tags | 11:24 |
79 | Who uses the JWT? | 10:16 |
80 | How to set up Flask-JWT-Extended with our app | 04:17 |
81 | Coding the User model and schema | 02:08 |
82 | How to add a register endpoint to the REST API | 09:36 |
83 | How to add a login endpoint to the REST API | 08:39 |
84 | Protect endpoints by requiring a JWT | 06:01 |
85 | JWT claims and authorization | 05:56 |
86 | How to add logout to the REST API | 06:20 |
87 | Request chaining with Insomnia | 04:33 |
88 | Token refreshing with Flask-JWT-Extended | 09:31 |
89 | How to add Flask-Migrate to our Flask app | 01:39 |
90 | Initialize your database with Flask-Migrate | 05:43 |
91 | Change SQLAlchemy models and generate a migration | 05:02 |
92 | Manually review and modify database migrations | 03:20 |
93 | What are Git repositories and commits? | 09:44 |
94 | Initialize a Git repository for our project | 08:28 |
95 | Writing Markdown for documents and commits | 02:37 |
96 | Remote repositories and how to use them | 05:28 |
97 | Git branches and merging | 06:18 |
98 | Merge conflicts and how to resolve them | 05:31 |
99 | Overview of the final e-book chapters | 01:39 |
100 | Creating a Render.com web service | 05:55 |
101 | How to run Flask with gunicorn in Docker | 06:14 |
102 | Get a deployed PostgreSQL database | 01:52 |
103 | Use PostgreSQL locally and in production | 17:46 |
104 | Test the finished production app | 01:39 |
105 | How to send emails with Python and Mailgun | 04:48 |
106 | How to send emails when users register | 09:38 |
107 | What is a task queue and setting up a Redis database | 05:06 |
108 | How to Populate and consume the task queue with rq | 06:27 |
109 | How to process background tasks with the rq worker | 05:25 |
110 | How to send HTML emails using Mailgun and Python | 10:18 |
111 | How to deploy a background worker to render.com | 05:40 |
Similar courses to REST APIs with Flask and Python
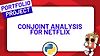
Conduct a Choice-Based Conjoint Analysis for Netflix with Python
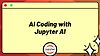
AI Coding with Jupyter AI
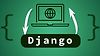
Python Django - The Practical Guide
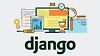
Django 2.1 & Python | The Ultimate Web Development Bootcamp
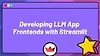
Developing LLM App Frontends with Streamlit
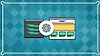
Build a Backend REST API with Python & Django - Advanced
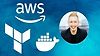
DevOps Deployment Automation with Terraform, AWS and Docker
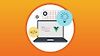
The Complete Guide to Django REST Framework and Vue JS
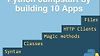
Python Jumpstart by Building 10 Apps
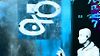